A complete KeyListener Java Example
In this article,we will talk about java key listener and we will demonstrate a complete KeyListener Java example.
1. Introduction
Java key listener is an interface used in Java Swing to monitor keyboard-related events that take place in your applications. This is particularly useful when you want to perform input validation on form fields or add shortcut functionality to your application.
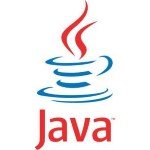
2. KeyListener Java API
The java keyboard listener interface extends the EventListener
interface and has the following method signatures:
void keyPressed(KeyEvent e)
This method is invoked when a key has been pressed.void keyReleased(KeyEvent e)
This method is invoked when a key has been released.void keyTyped(KeyEvent e)
This method is invoked when a key has been typed.
3. A Simple KeyListener Java Class
In this section, we will see the implementation of KeyListenerExample.java
. The steps to implement the class are as follows:
- Create a new
KeyListener
object. - Override the methods that correspond to the key events you want to monitor e.g
keyPressed
,keyReleased
,keyTyped
. - Create a
JTextField
component - Use it’s
addKeyListener
method to add to it theKeyListener
you’ve created.
Let’s take a closer look at the code snippet that follows:
KeyListenerExample.java
package com.javacodegeeks.snipppets.desktop; import java.awt.BorderLayout; import java.awt.Container; import java.awt.event.KeyEvent; import java.awt.event.KeyListener; import javax.swing.JFrame; import javax.swing.JTextField; public class KeyListenerExample { public static void main(String args[]) { JFrame frame = new JFrame("Key Listener"); Container contentPane = frame.getContentPane(); KeyListener listener = new KeyListener() { @Override public void keyPressed(KeyEvent event) { printEventInfo("Key Pressed", event); } @Override public void keyReleased(KeyEvent event) { printEventInfo("Key Released", event); } @Override public void keyTyped(KeyEvent event) { printEventInfo("Key Typed", event); } private void printEventInfo(String str, KeyEvent e) { System.out.println(str); int code = e.getKeyCode(); System.out.println(" Code: " + KeyEvent.getKeyText(code)); System.out.println(" Char: " + e.getKeyChar()); int mods = e.getModifiersEx(); System.out.println(" Mods: " + KeyEvent.getModifiersExText(mods)); System.out.println(" Location: " + keyboardLocation(e.getKeyLocation())); System.out.println(" Action? " + e.isActionKey()); } private String keyboardLocation(int keybrd) { switch (keybrd) { case KeyEvent.KEY_LOCATION_RIGHT: return "Right"; case KeyEvent.KEY_LOCATION_LEFT: return "Left"; case KeyEvent.KEY_LOCATION_NUMPAD: return "NumPad"; case KeyEvent.KEY_LOCATION_STANDARD: return "Standard"; case KeyEvent.KEY_LOCATION_UNKNOWN: default: return "Unknown"; } } }; JTextField textField = new JTextField(); textField.addKeyListener(listener); contentPane.add(textField, BorderLayout.NORTH); frame.pack(); frame.setVisible(true); } }
4. KeyListenerLoginFormExample
In this section, we will see an example implementation where the KeyListener
class is used to perform validation on a user name input field.
KeyListenerLoginFormExample.java
package com.javacodegeeks.snipppets.desktop; import javax.swing.*; import javax.swing.border.Border; import java.awt.*; import java.awt.event.KeyEvent; import java.awt.event.KeyListener; public class KeyListenerLoginFormExample { public static void main(String args[]) { JPanel inputPanel = new JPanel(); JFrame frame = new JFrame("Login Form"); Container pane = frame.getContentPane(); JLabel userNameInvalidLabel = new JLabel(); JLabel userNameLabel = new JLabel("User Name"); JTextField userNameTextField = new JTextField( 15); KeyListener listener = new KeyListener() { @Override public void keyPressed(KeyEvent event) { validateUserName(event); } @Override public void keyReleased(KeyEvent event) { } @Override public void keyTyped(KeyEvent event) { validateUserName(event); } private void validateUserName(KeyEvent event) { int num = event.getKeyChar(); if(!Character.isDigit(event.getKeyChar())) { event.consume(); } if(event.getKeyChar()==KeyEvent.VK_ENTER) { userNameInvalidLabel.setText("Your username is valid!!"); userNameTextField.setText(""); event.consume(); } else { userNameInvalidLabel.setText("");; } } }; userNameTextField.addKeyListener(listener); inputPanel.add(userNameLabel); inputPanel.add(userNameTextField); inputPanel.add(userNameInvalidLabel); pane.add(inputPanel, BorderLayout.PAGE_START); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(700,500); frame.setVisible(true); } }
In the above class, we see the usage of the keyTyped
and keyPressed
methods in the KeyListener implementation. The event.consume() statement in lines 35 and 40, will reset the value typed into the text field. Thus, through the validateUserName()
method, we are able to make sure that userNameTextField
only accepts numbers.
5. Summary
In this article, we were able to show 2 different usages and implementation of KeyListener
in Java.
6. Download the Source Code
This was a complete KeyListener Java Example.
You can download the full source code of this example here: A Complete KeyListener Java Example
Last updated on Jun. 11th, 2020
Awesome! thanks