Java Constructor Example (with video)
In this example, we are going to explain the use of the Java constructor for java classes.
In most cases, we need to create an object of a class, so as to use it. This can be done by using the constructors
that are provided within the class, which will create a new object of it and also assign initial values to its fields.
You can also check this tutorial in the following video:
1. Calling Constructor
We can create an object, simply by calling a constructor with the use of the operator new
: Person personObject = new Person();
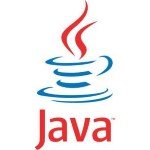
This line of code creates a new object of the class Person.
2. Declaring Constructors
Constructors have the same name as the class and they have no return type, since they return an instance of the class. We can use the access modifier, which can be public
, protected
, private
or “default”, so as to manage the accessibility of other classes to it.
Moreover constructors may, or may not have any arguments. A no-argument constructor
is used to initialize the fields with default, predefined values.
We can have more than one constructors, depending on the number and type of arguments which they require in their declaration.
We are not allowed to create more than one constructors with the same number and type of arguments for each class, since the compiler cannot distinguish them and a compilation error will occur.
Let’s have a look at the following example:
Person.java:
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
|
package com.javacodegeeks.core.constructor; public class Person { private String name; private int age; public Person() { // no-argument constructor, which assigns predefined values this .name = "Unknown" ; this .age = 0 ; } public Person(String Name) { // constructor which assigns one predefined value an one based on the input value this .name = Name; this .age = 0 ; } public Person(String Name, int Age) { // constructor which assigns the given values this .name = Name; this .age = Age; } public String getName() { return name; } public int getAge() { return age; } public static void main(String args[]) { // We create three different objects, one with each constructor provided. Person person1 = new Person(); Person person2 = new Person( "Jim" ); Person person3 = new Person( "John" , 28 ); // We print their data. System.out.println( "person1: " + person1.getName() + " is " + person1.getAge() + " years old." ); System.out.println( "person2: " + person2.getName() + " is " + person2.getAge() + " years old." ); System.out.println( "person3: " + person3.getName() + " is " + person3.getAge() + " years old." ); } } |
Output:
1
2
3
|
person1: Unknown is 0 years old. person2: Jim is 0 years old. person3: John is 28 years old. |
3. Declaring Constructor in subclass
The previous example demonstrated the use of constractors withing a single class. When it comes to class Inheritance, we can call the constructor of the superclass, within the subclass by using super
.
The following simple example illustrates how we can declare the constractor of a subclass. In this case, class Employee extends Person by adding the job of the person as extra information.
Employee.java:
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
|
package com.javacodegeeks.core.constructor; public class Employee extends Person { private String job; public Employee() { super (); // executes constructor Person(), line 7 in Person.java this .job = "Unknown" ; } public Employee(String Name) { super (Name); // executes constructor Person(String Name), line 13 in Person.java this .job = "Unknown" ; } public Employee(String Name, int Age, String Job) { super (Name, Age); // executes constructor Person(String Name), line 19 in Person.java this .job = Job; } private String getJob() { return job; } public static void main(String args[]) { // We create three different objects, one with each constructor provided above. Employee employee1 = new Employee(); Employee employee2 = new Employee( "Tom" ); Employee employee3 = new Employee( "George" , 40 , "Teacher" ); System.out.println( "employee1: " + employee1.getName() + " is " + employee1.getAge() + " years old " + employee1.getJob()); System.out.println( "employee2: " + employee2.getName() + " is " + employee2.getAge() + " years old " + employee2.getJob()); System.out.println( "employee3: " + employee3.getName() + " is " + employee3.getAge() + " years old " + employee3.getJob()); } } |
Output:
1
2
3
|
employee1: Unknown is 0 years old Unknown employee2: Tom is 0 years old Unknown employee3: George is 40 years old Teacher |
4. The Default Constructor
In case we do not provide a constructor
for our class at all, the java compiler provides us with a default constructor. This default constructor is not visible in our code and has no input arguments.
The following class has no constructor. We create an object of it and we print the values of its two variables. Notice that no error is generated and the variables have been initialized. This is due to the utilization of the default constructor.
DefaultConstructorExample.java:
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
20
21
|
package com.javacodegeeks.core.constructor; public class DefaultConstructorExample { private int num; private String str; public int getNum() { return num; } public String getStr() { return str; } public static void main(String args[]) { // we create a new object of the class DefaultConstructorExample obj = new DefaultConstructorExample(); System.out.println( "num = " + obj.getNum()); System.out.println( "str = " + obj.getStr()); } } |
Output:
1
2
|
num = 0 str = null |
5. More articles
- Java Tutorial for Beginners
- What is Java used for
- Best Way to Learn Java Programming Online
- 150 Java Interview Questions and Answers
6. Download the Source Code
This was an example of using Constructors in Java.
Download the Eclipse project of this example: Java Constructor Example
Last updated on May 05th, 2021