Continue Java Statement
1. Continue Java Statement
In this example, we shall show you how to use the continue keyword statement in Java.
The continue statement skips the current iteration of a for, while, or do-while loop and it continues the current flow of the program. In case of an inner loop, the continue statement continues only the inner loop.
In this example, the continue statement is used when checking an array’s elements, as described below:
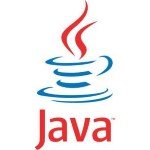
- Create a for statement with an int index from 0 up to an int array length, that checks the elements of the array
- When an element divided to 2 returns 0, then the continue statement is called that skips the current iteration
As described in the code snippet below:
public class ContinueStatement { public static void main(String[] args) { int array[] = new int[] { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10}; System.out.println("Printing all odd numbers"); for (int i = 0; i < array.length; i++) { if (array[i] % 2 ==0) continue; else System.out.print(array[i] + " "); } } }
Output
Printing all odd numbers
1 3 5 7 9
3. Labeled continue
Let’s say you have the below example:
public class Continue { public static void main(String[] args) { for (int i = 0; i <10 ; i++) { for (int j = 0; j <5 ; j++) { if(j%2==0){ System.out.println("i="+i+" and j="+j); continue; } } } } }
If we just write the continue statement, then this will print 0 and 0, 0 and 0 and 2 etc. But if we wanted to continue the outer loop we could do the following.
public class ContinueLabeled { public static void main(String[] args) { outerloop: for (int i = 0; i <10 ; i++) { for (int j = 0; j <5 ; j++) { if(j%2==0){ System.out.println("i="+i+" and j="+j); continue outerloop; } } } } }
Now the output will be 0 and 0, 1 and 0, 2 and 0 etc.
This was an example of how to use the continue statement, which is an essential part of the Java syntax.
2. Download the source code
That was an example of how to use the continue keyword in Java.
You can download the full source code of this example here: Continue Java Statement
Last updated on Apr. 22nd, 2020