In this post, we feature a Char to String Java Example and how to convert String to Character Array in Java. We will see how to convert a Character to String and a String to a StringArray.
Char to String Java Example
A character is a unit of information that roughly corresponds to a grapheme, grapheme-like unit, or symbol, such as in an alphabet or syllabary in the written form of a natural language. A string is traditionally a sequence of characters, either as a literal constant or as some kind of variable.
In the first Java program we convert a Character to String. Basically there are two ways to do that:
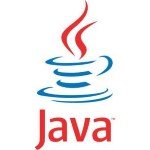
- the
Character.toString(char )
method of theCharacter
class - the
String.valueOf(char )
method of theString
Class.
To be precise the Character.toString(char )
method internally uses String.valueOf(char )
method. So you are be better off with String.valueOf(char)
.
CharToString.java
package com.javacodegeeks.java.core; public class CharToString { public static void main(String[] args) { char ch = 'J'; String string1 = Character.toString(ch); String string2 = String.valueOf(ch); System.out.println("character is : " + ch + ". String using String.valueOf(char c): " + string2); System.out.println("character is : " + ch + ". String using Character.toString(char c): " + string1); } }
Output
character is : J. String using String.valueOf(char c): J character is : J. String using Character.toString(char c): J
Now we are going to use String.toCharArray()
to convert a string into an array of characters.
StringToCharArray.java
package com.javacodegeeks.java.core; import java.util.Arrays; public class StringToCharArray { public static void main(String[] args) { String string = "JavaCodeGeeks"; char[] charArray = string.toCharArray(); System.out.println("String is:" + string + " Character Array : " + Arrays.toString(charArray)); } }
As you can see I am using Arrays.toString(char[])
in order to print the character array in a more readable way. So the output will be:
String is:JavaCodeGeeks Character Array : [J, a, v, a, C, o, d, e, G, e, e, k, s]
This was a Char to String Java Example & How to convert String to Character Array in Java.
Last updated on Aug. 14th, 2019
The Title should say: How to convert char to String and a String to char Array in Java