1. Introduction
Due to the content of this article, in the first section, I will start with a brief introduction about Spring and Spring-Boot framework, what are these frameworks and where to use them. Millions of developers are using Spring and Spring-Boot widely.
1.1. Spring
Firstly, let’s start with a brief introduction to Spring frameworks which is a programming and configuration model to develop modern enterprise applications.
This was firstly launched in 2002 by Rod Johnson and later on in 2003, Spring was moved under the Apache license 2.0.
Spring offers powerful infrastructure support at the application level. The most used features in Spring framework are:
- Core technologies like dependencies injection, events, resources, validation, and data binding.
- Classes for testing your code like Mocking Objects, Spring MVC Test, and WebTestClient.
- Data access: transaction, DAO support, JDBC, ORM, and Marshalling XML.
- Spring MVC and Spring WebFlux, web frameworks.
- JMS, JCA (J2EE Connector Architecture), JMX, Email, Tasks, Scheduling, and Cache.
- Supported languages are Kotlin, Groovy, and dynamic languages.
If you want to start learning Spring, you can check out the official documentation.
1.2. Spring Boot
Secondly, we will briefly introduce you to the Spring-boot framework. Spring-Boot is an open-source Java-based framework to be able to create microservices applications.
This framework helps you to easily create a web service application just with some annotation, ready to run in just a few minutes. Most Spring-Boot applications need some basic configuration of the Spring framework. Therefore Spring-Boot has many features that complete the benefits of the Spring framework without the need for an entire Spring configuration setup.
The most important features are:
- Be able to create a stand-alone Spring application.
- Embed Tomcat, Jetty, or Undertow directly without needing to deploy WAR files.
- The most important ‘starter’ dependencies to simplify your build configuration.
- Easily configure Spring and 3rd party libraries whenever possible.
- Similar to the Spring framework, there is no need for code generation and no requirements for XML configuration.
- It has support for auto-configuration.
- Spring boot initializer.
For more information about the Spring-Boot profile, you can find it in the official documentation.
2. Questions
In this section, we will present you with the most asked Spring-Boot questions at the technical interview. These questions are filtered by the latest asked questions in the technical interview from multiple websites. Further, we will go through the Top 20 Spring-Boot interview questions.
2.1. What is Spring-Boot and the needs of it?
It is a Spring module that helps you to rapidly develop a microservice application. Spring-Boot framework comes with auto-dependency, embedded HTTP servers, auto-configuration, management endpoints, and Spring Boot CLI. This framework helps you to improve your productivity and also provides a lot of conveniences to write your business logic. It can be used to develop stand-alone microservices It is a Spring module that helps you to rapidly develop a microservice application. Spring-Boot framework comes with auto-dependency, embedded HTTP servers, auto-configuration, management endpoints, and Spring Boot CLI. This framework helps you to improve your productivity and also provides a lot of conveniences to write your business logic. It can be used to develop stand-alone microservices applications very fast. In short, it’s now the standard way to develop Java applications with the Spring framework.
2.2. Advantages of Spring-Boot
- Easy to understand and develop Spring applications.
- Increase productivity and reduce development time.
- No need to write XML configuration, just a few annotations are required to do the configuration.
- Spring-boot provides CLI tools to develop and test applications.
- It comes with embedded tomcat, servlet containers jetty without the need of WAR files.
- Many APIs help you to monitor and manage applications in dev and prod environments.
2.3. Describe in a few words how Spring-Boot works internally?
Behind the scenes it automatically configures your application based on the dependencies added in the project and after that wrap-up all the classes into a JAR and launch a Tomcat server (in case you are developing a web application). The entry point of the Spring-Boot application is the class annotated with @SpringBootApplication annotation, in that class should be the main method. @ComponentScan annotation automatically scans all components from the project.
2.4. Why do you choose Spring-Boot over Spring?
Some points about the Spring framework.
Firstly, I wanted to note that this framework is used for developing web applications based on Java that provides tools and libraries to create customized web applications.
Secondly, the Spring framework is more complex than Spring-Boot in that it takes an un-opinionated view (leaves lots of flexibility to the developer to do the right choice).
About Spring-Boot.
Firstly, I wanted to point out that this framework is a module of Spring used to create a Spring application project which can just run and execute.
Secondly, Spring-Boot is less complex than Spring and offers an opinionated view.
2.5. What is auto-config in Spring-Boot, what are the benefits? Why is Spring Boot called opinionated?
The auto-config in Spring-Boot configures a lot of things that the developer doesn’t need to do much configuration and easily start and execute the application. This will configure beans, controllers, view resolvers etc, hence it helps a lot in creating a Java application.
That is to say, let’s discuss why the Spring-Boot is considered opinionated.
Firstly, opinionated is a software design pattern that decides and guides you into their way of doing things.
Secondly, Spring-Boot is considered as opinionated because it follows the opinionated default configuration that reduces developer efforts to configure the application.
2.6. What is the difference between @SpringBootApplication and @EnableAutoConfiguration annotation?
@SpringBootApplication annotation is present in the main class or bootstrapper class. This annotation is a combination of @Configuration, @ComponentScan and @EnableAutoConfiguration annotations with their default attributes. This annotation helps the developer to use just one annotation instead of the three annotations.
The annotation @EnableAutoConfiguration is used to enable auto-configuration and component scanning in the project. It is a combination of @Configuration and @ComponentScan annotations. This makes Spring Boot look for auto-configuration beans on its classpath and automatically apply them.
2.7. What is the need of @ComponentScan annotation?
For the classes that are in the same package hierarchy (or below) with the main class the spring will automatically scan all the classes and the beans/components will be added in the IoC at the start-up.
On the other hand in case you have other packages with beans/components that are not in the same hierarchy, which are not sub-package of the main, you will have to manually configure that package to scan and use the annotation @ComponentScan. This annotation will be added in the main class and the attribute of the @ComponentScan annotation should have that package path.
2.8. What is the entry point for Spring-Boot application?
The entry point of the Spring-Boot application is the main method which is annotated with @SpringBootApplication or equivalent of this annotation. This main method will initialize all the required beans and the defined components to start-up the application.
@SpringBootApplication
public class PaymentWebservice {
public static void main(String[] args) {
SpringApplication.run(PaymentWebservice.class);
}
}
2.9. What is a Spring actuator? What are the advantages?
It is a nice feature of Spring-Boot that provides a lot of information about the running application. This allows you to monitor and manage your application. It is easy to control your application just by using the HTTP endpoints. The actuator dependency figures out the metrics and makes them available as endpoints.
The most important built-in endpoints that Actuator provides:
- env exposes environment properties
- health shows application health information
- httptrace displays HTTP trace information
- info displays arbitrary application information
- metrics shows metrics information
- loggers shows the configuration of loggers in the application
- mappings displays a list of all @RequestMapping paths
2.10. How to deploy web applications such as JAR and WAR in Spring-Boot?
In the old style, we deploy web applications in a war file and deploy it on a server. In this way, we can arrange multiple applications on the same server. This was an approach when CPU and memory were limited, and that was the way to save it.
Further, we will present both cases to deploy JAR and WAR in Spring-Boot with the build tool: Maven and Gradle.
Firstly, we will present the maven dependency to add in the pom.xml:
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
This plugin spring-boot-maven-plugin
provides the ability to package a web application as an executable JAR. With this plugin in place, we will get a fat JAR after executing the package task. This jar will contain all the dependencies including an embedded server. In the pom.xml file, we should also define this line: <packaging>jar</packaging>
in case you want to build a JAR file. In case you don’t provide that in the pom.xml the default value is JAR.
For deploying a war file the only thing we should do is to change the previous value to war, it will be something like this: <packaging>jar</packaging>
.
Secondly, we will discuss deploying a war or jar with the build tool Gradle. In contrast with the previous tool for pom.xml for Gradle we have:
plugins {
id "org.springframework.boot"
}
If we want to deploy a JAR file we have to add in the plugins: apply plugin: 'java'
. For war, the only thing to change is: apply plugin: 'war'
.
In Spring-Boot 2.x we have to do different tasks to deploy an application just by calling the gradle
task which are: ./gradlew bootJar
or ./gradlew bootWar
.
2.11. How can you change the default Embedded tomcat server in Spring Boot?
Spring-Boot supports as servers to run web applications the following: Tomcat, Jetty, and Undertow.
To change the default embedded server you have to add the dependency with the new embedded server and exclude the default one. For this example, we will choose to change to the Undertow web server to run our application. What you have to do are the following in your build tool (Maven or Gradle add the dependency and exclude the default).
Maven dependency:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-undertow</artifactId>
</dependency>
#exclude the default embedded server
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<exclusions>
<exclusion>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
</exclusion>
</exclusions>
</dependency>
Gradle dependency:
implementation ('org.springframework.boot:spring-boot-starter-web') { exclude module: 'spring-boot-starter-tomcat' } implementation 'org.springframework.boot:spring-boot-starter-undertow'
In the logs you will see something like this:
2022-04-23 12:56:05.915 INFO --- [main] io.undertow - starting server: Undertow - 2.2.3.Final
2022-04-23 12:56:05.934 INFO --- [main] org.xnio - XNIO version 3.8.0.Final
2022-04-23 12:56:05.960 INFO --- [main] org.xnio.nio - XNIO NIO Implementation Version 3.8.0.Final
2022-04-23 12:56:06.065 INFO --- [main] org.jboss.threads - JBoss Threads version 3.1.0.Final
2022-04-23 12:56:06.123 INFO --- [main] o.s.b.w.e.undertow.UndertowWebServer - Undertow started on port(s) 8443 (http)
2.12. How to disable a specific auto-configuration class in Spring-Boot?
If you want to exclude a specific class from auto-configuration you can use the attribute: exclude from the annotation @EnableAutoConfiguration. So that class will not apply for auto-configuration.
@EnableAutoConfiguration(exclude={className})
2.13. Why do you use profiles?
The most important feature of using profiles is the fact that you can configure multiple environments with different properties on each environment. While developing applications you will need multiple environments such as: Dev, QA, Prod. For example, we might be using an authorization model with a password for dev but for prod, we might have a different password. This also applies to DBMS even if it is the same across the environment, the URLs will be different.
2.14. Minimum requirements for using Spring-boot framework.
Spring Boot 2.6.7 requires Java 8 with an extension to the Java 17 and a Spring Framework 5.3.19 or above is required.
On the other hand from the perspective of the Build Tool is required Maven 3.5+ and Gradle the following versions 6.8.x, 6.9.x, and 7.x.
2.15. What is spring-boot-starter-parent?
It is a special starter which helps Gradle or Maven dependency-management to easily add the jars to your classpath
, providing default configurations and a complete dependency tree to quickly build the application.
2.16. How can you access a value defined in the application? What are properties files in Spring Boot?
There are three possible ways of accessing a value defined in the property file.
- Using @Value annotation with the key from the property file.
- With a class annotating with @ConfigurationProperties with the key.
- Environment object provides a method called: getProperty() where you can provide as parameter a key from properties.
Above all, a quick mention regarding properties file, that is related to the configuration that you can overwrite in the Spring-Boot, but also to define your own properties that you can use in the code. This file is located in the src/main/resources.
2.17. How can you control logging in Spring Boot?
Spring-Boot uses Commons Logging for all internal logging, and you can change log levels by adding the following lines in the application.properties file:
logging.level.org.springframework=DEBUG
logging.level.com.demo=INFO
Spring-Boot loads the application.properties file if it exists in the classpath
and can be used to configure both Spring-Boot properties and some constants that are used in application code.
2.18. What is the default port for Tomcat Spring-Boot?
The default port for Tomcat embedded server is 8080.
2.19. How to change the port of the embedded Tomcat server in Spring Boot?
To change the default port in Spring-boot, you will have to overwrite this property in the application.properties:
server.port=8443
There is also another way of changing the default port. This second option is to provide this parameter as a variable to the run command:
java -jar <your_jar_with_dependencies>.jar --server.port=8443
2.20. What is dependency injection in spring boot?
Is the process of injecting the dependent bean objects into target bean objects. In other words, the ability of an object to supply dependencies to another object.
Type of dependency injection:
- Setter injection. The IoC container will inject the dependent bean into the target bean object by calling the setter method.
- Constructor injection. The IoC will inject the dependent bean object into the target bean object by calling the target bean constructor.
- Field injection. The IoC will inject the dependent bean object into the target bean by Reflection API.
Spring framework it’s also known as Inversion of Control. Inversion of Control is the base principle on which Dependency Injection is made. So basically it is used to invert different kinds of additional responsibilities of a class rather than the main responsibility.
2.21. Bonus section.
In this section, we will show you how Spring-Boot modules look internally.
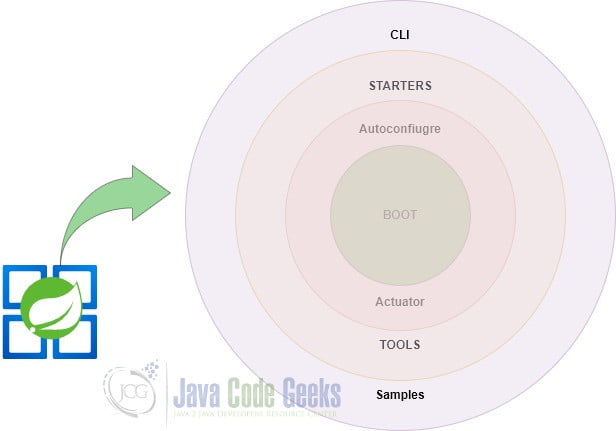
The main concept behind Spring-Boot starters is to reduce the dependencies definition and to simplify the project build dependencies. In case of developing a Spring application we have to define in the pom.xml the following dependencies: Spring core JAR file, Spring Web JAR file, MVC jar file and JAR file. On the other hand, this framework starters provides the easy way to add only one JAR file spring-boot-starter-web.
The concept behind Spring-Boot autoconfiguration is to minimize the programmer’s effort to define thousands of XML configurations. The autoconfiguration will manage all the XML configurations with annotation.
Spring-Boot CLI provides a tool to run and test Spring-Boot applications from the command line. This is referring to command line arguments. To execute the application, Spring-Boot CLI uses Spring-Boot starter and Autoconfiguration to resolve all dependencies.
Spring-Boot actuator is a tool to provide some HTTP endpoints to monitor the application. We can manage our production application using these HTTP endpoints.
3. Conclusion
In this article, you will get familiar with the most asked questions during the interview. If you are already familiar with the Spring-Boot it will be helpful for you to prepare for the technical interview. Besides that even if you are a beginner with Spring-Boot, this article will help you to better understand the concept of Spring-Boot and the power of this framework.
To sum up this article it is useful for people who are preparing for technical interviews but also for beginners who want to better understand the concept with a summarization for the entire framework.