StringBuilder Java Example
In this post, we feature a comprehensive StringBuilder Java Example. StringBuffer in Java is the same as StringBuilder, but the first is thread-safe. At the end of this article, we will create an abstract TestBase
class which shows StringBuffer ‘s common methods.
1. Introduction
StringBuilder object seems like a String
object but with the characteristics of an array. Every object of this type is like a sequence of characters that can be modified. The StringBuilder
class provides many methods for changing the content and/or the length of the sequence, for initializing the capacity etc. StringBuilder
class is mostly used when we want to concatenate many strings continuously and/or treat them like variable-length arrays.
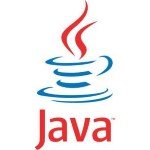
In this example, as you can expect, we are going to show how to use basic operations of StringBuilder
class.
- Four constructors of
StringBuilder
StringBuilder
common methods:append
,delete
, andinsert
- The comparison between
StringBuilder
andString
2. Technologies Used
The example code in this article was built and run using:
- Java 11
- Maven 3.3.9
- Eclipse Oxygen
- Junit 4.12
3. Maven Project
3.1 Dependencies
I will include Junit
in the pom.xml
.
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>jcg.zheng.demo</groupId> <artifactId>java-strringbuilder-demo</artifactId> <version>0.0.1-SNAPSHOT</version> <build> <sourceDirectory>src</sourceDirectory> <plugins> <plugin> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.0</version> <configuration> <release>11</release> </configuration> </plugin> </plugins> </build> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> </dependency> </dependencies> </project>
3.2 Read File Application
In this step, I will create a FeadFileApp
class which reads all the lines of a file and append them into a string builder, separated by ‘@’ character, until the end of the file. Finally, it calls toString()
method in order to take the string representation of the string builder. In conclusion, I use it to show that StringBuilder
class offers a better performance.
ReadFileApp.java
package jcg.zheng.demo; import java.io.BufferedReader; import java.io.FileNotFoundException; import java.io.FileReader; import java.io.IOException; public class ReadFileApp { public static void main(String[] args) { String fileName = "C:/MaryZheng/jcgFile.txt"; try { BufferedReader br = new BufferedReader(new FileReader(fileName)); StringBuilder sbFile = new StringBuilder(); String line = br.readLine(); while (line != null) { sbFile.append(line); sbFile.append('@'); line = br.readLine(); } String readFile = sbFile.toString(); br.close(); System.out.println("from file: " + readFile); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } } }
Output of the Java application
from file: Hello@How are you@
3.3 POJO Example
In this step, I will create a POJOExample
class which is used to append an object argument to a StringBuilder
object.
POJOExample.java
package jcg.zheng.demo; public class POJOExample { public POJOExample(String name, int id) { super(); this.name = name; this.id = id; } private String name; private int id; @Override public String toString() { return "POJOExample [name=" + name + ", id=" + id + "]"; } }
4. JUnit Test
4.1 TestBase
As we stated at the beginning of the article, StringBuffer in Java is the same as StringBuilder, but the first is thread-safe. In this step, I will create an abstract TestBase
class which shows StringBuffer ‘s common methods:
append
– adds a specified parameter to a string builder. In this step, I create test methods for various data types:boolean
,char
,char[]
,double
,POJOExample
,String
, andStringBuilder
.insert
– inserts the string representation of a data type in a specific offset of the sequence.delete
– removes a specific portion of the string builder.length
– returns the current number of characters in the string builder sequence.capacity
– returns the current capacity which indicates the allocation of character space of the string builder.
A StringBuilder
object will be created in a separated test class which extends from TestBase
.
TestBase.java
package jcg.zheng.demo; import static org.junit.Assert.assertEquals; import org.junit.Test; public abstract class TestBase { private static final String HELLO_EVERYONE_FROM_JCG = "Hello Everyone from JCG"; private static final String EVERYONE = "Everyone "; private static final String HELLO_FROM_JCG = "Hello from JCG"; protected StringBuilder sb; public TestBase() { super(); } protected void clear() { if (sb.length() >= 1) { sb.delete(0, sb.length()); assertEquals(0, sb.length()); } } @Test public void test_append_boolean() { sb.append(true); sb.append(false); assertEquals("truefalse", sb.toString()); } @Test public void test_append_char() { sb.append('|'); assertEquals("|", sb.toString()); } @Test public void test_append_charArray() { char[] abc = {'a', 'b', 'c'}; sb.append(abc); assertEquals("abc", sb.toString()); } @Test public void test_append_double() { sb.append(123.456); assertEquals("123.456", sb.toString()); } @Test public void test_append_object() { sb.append(new POJOExample("Mary", 1)); assertEquals("POJOExample [name=Mary, id=1]", sb.toString()); } @Test public void test_append_string() { sb.append(HELLO_FROM_JCG); assertEquals(HELLO_FROM_JCG, sb.toString()); } @Test public void test_append_anotherSB() { StringBuilder sb2 = new StringBuilder("New Value"); sb.append(sb2); assertEquals("New Value", sb.toString()); } @Test public void test_delete() { sb.append(HELLO_FROM_JCG); sb.delete(6, 11); assertEquals("Hello JCG", sb.toString()); } @Test public void test_insert() { sb.append(HELLO_FROM_JCG); sb.insert(6, EVERYONE); assertEquals(HELLO_EVERYONE_FROM_JCG, sb.toString()); } }
4.2 Default Constructor
In this step, I will create a ConstructorStingBuilder1Test
which extends from TestBase
and creates a StringBuilder
object from the default constructor. The created object has a default capacity of 16 which means 16 empty characters.
ConstructStringBuilder1Test.java
package jcg.zheng.demo; import static org.junit.Assert.assertEquals; import org.junit.Before; public class ConstructStringBuilder1Test extends TestBase { @Before public void setup() { sb = new StringBuilder(); assertEquals(16, sb.capacity()); assertEquals(0, sb.length()); clear(); } }
Output of mvn test -Dtest=ConstructStringBuilder1Test
------------------------------------------------------- T E S T S ------------------------------------------------------- Running jcg.zheng.demo.ConstructStringBuilder1Test Tests run: 9, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.168 sec Results : Tests run: 9, Failures: 0, Errors: 0, Skipped: 0
4.3 Constructor with Capacity
In this step, I will create a ConstructorStingBuilder2Test
which extends from TestBase
and creates a StringBuilder
object with a capacity number. Here is the capacity constructor signature:
/*Constructs a string builder with no characters in it and an initial capacity specified by the capacity argument. capacity - the initial capacity.*/ StringBuilder(int capacity)
ConstructStringBuilder2Test.java
package jcg.zheng.demo; import static org.junit.Assert.assertEquals; import org.junit.Before; public class ConstructStringBuilder2Test extends TestBase { @Before public void setup() { // initialized capacity sb = new StringBuilder(15); assertEquals(15, sb.capacity()); assertEquals(0, sb.length()); clear(); } }
Output of mvn test -Dtest=ConstructStringBuilder2Test
------------------------------------------------------- T E S T S ------------------------------------------------------- Running jcg.zheng.demo.ConstructStringBuilder2Test Tests run: 9, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.162 sec Results : Tests run: 9, Failures: 0, Errors: 0, Skipped: 0
4.4 Constructor with CharSequence
In this step, I will create a ConstructorStingBuilder3Test
which extends from TestBase
and creates a StringBuilder
object from a CharSequence
. Here is the CharSequence
constructor signature:
/*Constructs a string builder that contains the same characters as the specified CharSequence. The initial capacity of the string builder is 16 plus the length of the CharSequence argument. seq - the sequence to copy.*/ StringBuilder(CharSequence seq)
The created object has a capacity of 72.
ConstructStringBuilder3Test.java
package jcg.zheng.demo; import static org.junit.Assert.assertEquals; import org.junit.Before; public class ConstructStringBuilder3Test extends TestBase { @Before public void setup() { // initialized capacity sb = new StringBuilder('H'); assertEquals(72, sb.capacity()); assertEquals(0, sb.length()); clear(); } }
Output of mvn test -Dtest=ConstructStringBuilder3Test
------------------------------------------------------- T E S T S ------------------------------------------------------- Running jcg.zheng.demo.ConstructStringBuilder3Test Tests run: 9, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.211 sec Results : Tests run: 9, Failures: 0, Errors: 0, Skipped: 0
4.5 Constructor with String
In this step, I will create a ConstructorStingBuilder4Test
which extends from TestBase
and creates a StringBuilder
object from a String
object. Here is the string constructor signature:
/*Constructs a string builder initialized to the contents of the specified string. str - the initial contents of the buffer.*/ StringBuilder(String str)
The created object has a capacity of 21.
ConstructStringBuilder4Test.java
package jcg.zheng.demo; import static org.junit.Assert.assertEquals; import org.junit.Before; public class ConstructStringBuilder4Test extends TestBase { @Before public void setup() { // initialized capacity sb = new StringBuilder("Hello"); assertEquals(21, sb.capacity()); assertEquals(5, sb.length()); clear(); } }
Output of mvn test -Dtest=ConstructStringBuilder4Test
------------------------------------------------------- T E S T S ------------------------------------------------------- Running jcg.zheng.demo.ConstructStringBuilder4Test Tests run: 9, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.165 sec Results : Tests run: 9, Failures: 0, Errors: 0, Skipped: 0
4.6 Comparison with String
In this step, I will create a StingBuilder_StringTest
which demonstrates that StringBuilder
is mutable and String
is immutable.
testString
– it creates amsg
String
variable and uses+
to append a string. You can confirm that each + operation return a newString
object by viewing themsg
‘s ID at debugging.testStringBuilder
– it changes theStringBuilder
object –msg
– by usingappend
method.
StringBuilder_StringTest.java
package jcg.zheng.demo; import static org.junit.Assert.assertEquals; import org.junit.Test; public class StringBuilder_StringTest { @Test public void testString() { String msg = "Hello"; msg = msg + " JCG"; msg = msg + " World"; assertEquals("Hello JCG World", msg); } @Test public void testStringBuilder() { StringBuilder msg = new StringBuilder(); msg.append("Hello"); msg.append(" JCG"); msg.append(" World"); assertEquals("Hello JCG World", msg.toString()); } }
Output of mvn test -DTest=StringBuilder_StringTest
------------------------------------------------------- T E S T S ------------------------------------------------------- Running jcg.zheng.demo.StringBuilder_StringTest Tests run: 2, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.14 sec Results : Tests run: 2, Failures: 0, Errors: 0, Skipped: 0
5. StringBuilder Java Example – Summary
In this example, I demonstrated what the StringBuilder
class offers in order to have better performance. We showed its four constructors and several of StringBuffer ‘s common methods in Java, like: append
, delete
, insert
, etc which change the content.
6. Download the Source Code
This example consists of a Maven project which contains several Junit tests to demonstrate the usage of the StringBuilder
class.
You can download the full source code of this example here: StringBuilder Java Example
Last updated on Jan. 22nd, 2020
StringBuilder that is the string object whose value can be changed . It is very important concept in java. Many thanks for sharing.