String length Java Example
In this example, we are going to see how you can find out the length of a String in Java. By length string, we mean the number of Unicode characters or Code Units.
1. What is String in Computer Programming
In computer programming, a string is traditionally sequence of characters, either as a literal constant or as some kind of variable. The latter may allow its elements to be mutated and the length changed, or it may be fixed (after creation). A string is generally considered as a data type and is often implemented as an array data structure of bytes (or words) that stores a sequence of elements, typically characters, using some character encoding. String may also denote more general arrays or other sequence (or list) data types and structures.
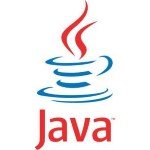
2. String length Java Example
String
class has a very convenient method of doing so: length()
.
Here is how you can use it:
StringLengthExample.java
package com.javacodegeeks.core.string; public class StringLengthExample { public static void main(String[] args) { String s1 = "Java Code Geeks are awesome!"; int strLength = s1.length(); System.out.println("The length of the string s1 : "+strLength); System.out.println("The length of this string is : ".length()); } }
This will output:
The length of the string s1 : 28
31
Take care when using length
with words of languages that have multi-character letters length
will count the number of characters of the String
, not lexicographical length.
There is also another way to count the number of characters in a String
, but “unpaired surrogates within the text range count as one code point”:
StringFromatExample.java:
package com.javacodegeeks.core.string; public class StringLengthExample { public static void main(String[] args) { String s1 = "Java Code Geeks are awesome!"; System.out.println(s1.codePointCount(0, s1.length())); } }
This will output: 28
This was a String length Java Example.
3. Download the Source Code
You can download the full source code of this example here: String Length Java Example