String charAt() Java Example
In this post, we feature a comprehensive String charAt Java Example. This method in Java returns a char at the specified index.
1. Introduction
The java.lang.CharSequence interface provides read-only access to many different kinds of char sequence. It has provided a charAt
method since version 1.4 to return the character value at the specified index. java.lang.String
class implements java.lang.CharSequence
. The string indexes start from zero to the string’s length()
minus one.
Here is the method syntax:
char charAt(int index)
Parameters: index
– the index of the char value to be returned.
Returns: the specified char value at the specified index. If the character value specified by the index is a surrogate, the surrogate value is returned.
Throws: IndexOutOfBoundsException if the index value is negative, equals to, or greater than the length()
value.
2. Technologies Used
The example code in this article was built and run using:
- Java 11
- Eclipse Oxygen
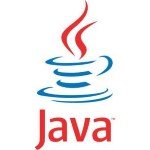
3. Application
In this step, I will create a stand-alone Java application that defines a test string and prints out its character value at the index. I will demonstrate the exception when the index value is out of range as well as the string includes a surrogate value.
loopString_charAt
– create afor
loop, starts from zero and ends with theorgString.length()
minus one. Print out its char value for all the indexes.charAt_exception
– create twotry..catch
statements to show theIndexOutOfBoundException
.chatAt_surrogate
– demonstrate a String withUTF-16
encoding with high-low surrogate pair values.main
– InvokesloopString_charAt
,loopString_surrogate
, andcharAc_exception
.
DemoApp.java
package jcg.zheng.demo; import java.io.UnsupportedEncodingException; public class DemoApp { public static void main(String[] args) { String testString = "Mary Rocks!"; System.out.println("Test String: " + testString); loopString_charAt(testString); charAt_exception(testString); charAt_surrogate(); } private static void loopString_charAt(String orgString) { for (int pos = 0; pos < orgString.length(); ++pos) { char c = orgString.charAt(pos); System.out.println(orgString + "[" + pos + "]=" + c); } } private static void charAt_exception(String orgString) { try { orgString.charAt(-1); } catch (IndexOutOfBoundsException e) { System.out.println(e.getMessage()); } try { orgString.charAt(orgString.length()); } catch (IndexOutOfBoundsException e) { System.out.println(e.getMessage()); } } private static void charAt_surrogate() { byte[] data = { 0, 0x41, // A (byte) 0xD8, 1, // High surrogate (byte) 0xDC, 2, // Low surrogate 0, 0x42, // B (byte) 0xd800, (byte) 0xdfff, }; try { String text = new String(data, "UTF-16"); System.out.println("Origal String with surrogate, its length=" + text.length()); System.out.println(text.charAt(0) + " " + text.codePointAt(0)); System.out.println(text.charAt(1) + " " + text.codePointAt(1)); System.out.println(text.charAt(2) + " " + text.codePointAt(2)); System.out.println(text.charAt(3) + " " + text.codePointAt(3)); System.out.println(text.charAt(4) + " " + text.codePointAt(4)); } catch (UnsupportedEncodingException e) { e.printStackTrace(); } } }
4. Demo
In Eclipse, right-click DemoApp
and click Run As Java Application. Capture the output here.
Output
Test String: Mary Rocks! Mary Rocks![0]=M Mary Rocks![1]=a Mary Rocks![2]=r Mary Rocks![3]=y Mary Rocks![4]= Mary Rocks![5]=R Mary Rocks![6]=o Mary Rocks![7]=c Mary Rocks![8]=k Mary Rocks![9]=s Mary Rocks![10]=! String index out of range: -1 String index out of range: 11 Origal String with surrogate, its length=5 A 65 ? 66562 ? 56322 B 66 ÿ 255
5. String charAt() Java Example – Summary
In this example, I demonstrated how to use the charAt
method to return a character value at the string’s specified index in three ways:
- When the parameter
index
is within the given string’s range. - When the parameter
index
is out of range of the giving string value. - When
String
has a surrogate value.
6. Download the Source Code
This example consists of a project which uses the charAt
method to return String’s character value at a specified index.
You can download the full source code of the String charAt Java Example here: String charAt() Java Example
Last updated on Jan. 14th, 2020
Hi Mary how ya doing can you please explain your byte array set up? i mean { 0, 0x41, // A
(byte) 0xD8, 1, // High surrogate
(byte) 0xDC, 2, // Low surrogate
0, 0x42, // B
(byte) 0xd800, (byte) 0xdfff,
};
Why in 2 bytes x character? is this mandatory for utf-16 i think? and in the surrogate what 1,2 means and leading zero in the A,B and the setup for the last character? please.
good question. Java defaults to UTF-16 and it requires 2 bytes for a character. you can checkout this thread https://stackoverflow.com/questions/5903008/what-is-a-surrogate-pair-in-java