Static vs Non-Static in Java
In this article, we will explain Static vs Non-Static definitions in Java.
1. Introduction
In Java, there are around 57 reserved keywords that cannot be used as an identifier. One of them is the static keyword.
Static keyword in Java is used for memory management. It can be used with variables, methods, blocks, and nested classes. Static keyword is actually used for keeping the same copy of variables or methods for every instance of a class. If a member of a class is static, it can be accessed before any objects are created for that class and also without any object reference. In Java, static keyword is a non-access modifier and can be used with the following :
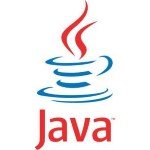
- Variables
- Methods
- Blocks
- Inner classes
2. Static vs Non-Static variables in Java
In Java, at the class level, we can have either a static or a non-static variable. The static variable is also called a class variable as it can be accessed without an object reference. Static variables are stored in a class area in the memory. On the other hand, non-static variables or an instance variable are created in the heap memory for all the instances of the class individually. Let us try to understand the differences between the two types with the below programs.
2.1 Example for Non Static variable
We will now see the use of a non-static variable with the following code snippet.
public class NonStaticEmployee { String name; int empid; String company; public NonStaticEmployee(int empid, String name, String company) { this.empid = empid; this.name = name; this.company = company; } public void display() { System.out.println("The details of the employee is employee id: "+empid+", employee name: "+name+", company:"+company); } public static void main(String[] args) { NonStaticEmployee emp1 = new NonStaticEmployee(1001,"Aakash Pathak","Webcodegeeks"); NonStaticEmployee emp2 = new NonStaticEmployee(1002,"Robert Bernes","Webcodegeeks"); emp1.display(); emp2.display(); } }
Output
The details of the employee is employee id: 1001, employee name: Aakash Pathak, company:Webcodegeeks The details of the employee is employee id: 1002, employee name: Robert Bernes, company:Webcodegeeks
If we look at the above code the class level field ‘company’ is having the same value i.e. ‘webcodegeeks’ for all the instances of the class Employee. Hence if we keep the field ‘company’ as a non-static variable, then it would be a wastage of memory as for each object created it would allocate memory for the company variable for each of the instances created and further assign the given value ‘webcodegeeks’ in it. Hence to avoid the situation we can declare the field company as a static variable and assign it the value ‘webcodegeeks’ in the class definition so it would independent of the no. of instances created thereby saving memory. Let us see the modified code for the same program.
2.2 Example for Static variable
We will now see the use of a static variable with the following code snippet.
public class StaticEmployee { String name; int empid; static String company="webcodegeeks"; public StaticEmployee(int empid, String name) { this.empid = empid; this.name = name; } public void display() { System.out.println("The details of the employee is employee id: "+empid+", employee name: "+name+", company:"+company); } public static void main(String[] args) { StaticEmployee emp1 = new StaticEmployee(1001,"Aakash Pathak"); StaticEmployee emp2 = new StaticEmployee(1002,"Robert Bernes"); emp1.display(); emp2.display(); } }
Output
The details of the employee is employee id: 1001, employee name: Aakash Pathak, company:Webcodegeeks The details of the employee is employee id: 1002, employee name: Robert Bernes, company:Webcodegeeks
3. Static vs Non-Static Method in Java
Static methods are utility methods in a class which can be exposed to other classes without having to create an instance of the owner class to call the method. Hence from a memory management perspective, static methods are less memory intensive compared to non-static methods. In Java, many of the utility classes like Wrapper classes, System class, Collections classes have static methods. On the other hand, a non-static method is an instance-based method that needs to be called by specific instances of the class and the behavior of such methods completely depends on the state of the objects which calls them. Let us see some of the differences between the nature of static and non-static methods.
Sl. No. | Key | Static Method | Non-static method |
1. | Access | A static method can access only static members and can not access non-static members. | A non-static method can access both static as well as non-static members. |
2. | Binding | Static method uses complie time binding or early binding. | Non-static method uses run time binding or dynamic binding. |
3 | Overriding | A static method cannot be overridden being compile time binding. | A non-static method can be overridden being dynamic binding. |
4 | Memory allocation | Static method occupies less space and memory allocation happens once. | A non-static method may occupy more space. Memory allocation happens when method is invoked and memory is deallocated once method is executed completely. |
5 | Keyword | A static method is declared using static keyword. | A normal method is not required to have any special keyword. |
3.1 Example for Non-static method
We will now see the use of a non-static method with the following code snippet.
package Methods; public class NonStaticEmployee { String name; int empid; String company; public NonStaticEmployee(int empid,String name,String company) { this.name = name; this.empid = empid; this.company = company; } public void display() { System.out.println("The details of the employee is"+" employee id="+empid+", emlployee name="+name+", company="+company); } public static void main(String[] args) { NonStaticEmployee emp1 = new NonStaticEmployee(1001,"Akash Pathak","WebcodeGeeks"); emp1.display(); NonStaticEmployee emp2 = new NonStaticEmployee(1002,"Robert Bernes","WebcodeGeeks"); emp2.display(); } }
Output
The details of the employee is employee id=1001, emlployee name=Akash Pathak, company=WebcodeGeeks The details of the employee is employee id=1002, emlployee name=Robert Bernes, company=WebcodeGeeks
3.2 Example of a Static Method
We will now see the use of a static method with the following code snippet.
package Methods; public class StaticEmployee { String name; int empid; String company; public StaticEmployee(int empid,String name,String company) { this.name = name; this.empid = empid; this.company = company; } public void display() { System.out.println("The details of the employee is"+" employee id="+empid+", emlployee name="+name+", company="+company); } public static double calcSal(double basic,double HRA, double Tax, double PI) { return basic+HRA+PI-Tax/100*basic; } public static void main(String[] args) { StaticEmployee emp1 = new StaticEmployee(1001,"Akash Pathak","WebcodeGeeks"); emp1.display(); System.out.println("The Salary for "+emp1.empid+" is: "+calcSal(12000,10000,9,5000)); StaticEmployee emp2 = new StaticEmployee(1002,"Robert Bernes","WebcodeGeeks"); emp2.display(); System.out.println("The Salary for "+emp2.empid+" is: "+calcSal(10000,8000,8,4000)); } }
Output
The details of the employee is employee id=1001, emlployee name=Akash Pathak, company=WebcodeGeeks The Salary for 1001 is: 25920.0 The details of the employee is employee id=1002, emlployee name=Robert Bernes, company=WebcodeGeeks The Salary for 1002 is: 21200.0
4. Static Initialization block vs initialization block
In Java, a block is a set of statements enclosed within curly braces which are used as a compound statement or a single unit of code. Blocks can be used in many ways in Java e.g. Methods, if-else statements, loops, and lambdas, etc. Independent blocks, i.e. blocks which are not used by other Java constructs like loops or if-else statements or methods, etc., are broad of two types namely i. static initializer blocks and ii. initializer blocks. Let us understand the difference between the two types of blocks from the given comparison below.
Sl. No. | Static Initialization Block | Instance Initialization Block |
1 | static keyword is used to define a static initialization block. | An instance initialization block can be defined without using any special keyword. |
2 | A static initialization block gets loaded as soon as a class gets loaded in the memory and is not associated with a call to the constructor of a class during object creation. | An instance initialization block is only executed when there is a call to the constructor during object creation. |
3 | Static block can only access static members of its class i.e. static variables and static methods. | An instance initialization block can both static as well as non-static members of its class i.e. static and non-static variables and methods. |
4 | The superclass constructor is not called automatically from the static initialization block. | An automatic call to super class constructor is made by using the super() before executing any statement in the instance initialization block. |
5 | Static block is called just once during the entire execution of the program when the class loads. | Instance initialization block is called as many times as a call to the constructor of the class is made. |
4.1 Static Initializer block
A static initialization block is used as a static initializer for a class i.e to initialize the static variables of a class. This block of code is executed only once when the class is loaded into memory by the ClassLoader component of JVM and is even called before the main method.
4.1.1 Example of a Static Initialization block
We will now see the use of a static initialization block with the following code snippet.
package blocks; public class StaticInitializationEmployee { int empid; String name; static String company; static { System.out.println("Static Initialization block called"); company = "WebCodeGeeks"; } public StaticInitializationEmployee(int empid,String name) { this.empid = empid; this.name = name; } public void display() { System.out.println("The details of the employee are: employee Id:"+empid+", employee name= "+name+", company name="+company); } public static void main(String[] args) { System.out.println("Main method called"); StaticInitializationEmployee emp1 = new StaticInitializationEmployee(1001,"Akash Pathak"); emp1.display(); StaticInitializationEmployee emp2 = new StaticInitializationEmployee(1002,"Robert Bernes"); emp2.display(); } }
Output
Static Initialization block called Main method called The details of the employee are: employee Id:1001, employee name= Akash Pathak, company name=WebCodeGeeks The details of the employee are: employee Id:1002, employee name= Robert Bernes, company name=WebCodeGeeks
In the above example, we can see that the static Initialization block has been called even before the main method.
4.1.2 Example of Initialization block
We will now see the use of an initialization block with the following code snippet.
package blocks; public class InitializationEmployee { int empid; String name; String company; { System.out.println("Initialization block called"); company = "WebCodeGeeks"; } public InitializationEmployee(int empid,String name) { this.empid = empid; this.name = name; } public void display() { System.out.println("The details of the employee are: employee Id:"+empid+", employee name= "+name+", company name="+company); } public static void main(String[] args) { System.out.println("Main method called"); InitializationEmployee emp1 = new InitializationEmployee(1001,"Akash Pathak"); emp1.display(); InitializationEmployee emp2 = new InitializationEmployee(1002,"Robert Bernes"); emp2.display(); } }
Output
Static Initialization block called Main method called Initialization block called The details of the employee are: employee Id:1001, employee name= Akash Pathak, company name=WebCodeGeeks Initialization block called The details of the employee are: employee Id:1002, employee name= Robert Bernes, company name=WebCodeGeeks
In the above example, we can see that the instance initialization block has been called even as many times as the no. of objects created.
5. Static Inner class vs Inner class
In Java, we are able to define a class inside another class. Such a class is called nested class or inner class. For e.g.
class OuterClass { ... class NestedClass { ... } }
Nested classes are divided into two categories: static and non-static. Nested classes that are declared static
are called static nested classes. Non-static nested classes are called inner classes.
class OuterClass { ... static class StaticNestedClass { ... } class InnerClass { ... } }
A nested class is a member of its enclosing class. Non-static nested classes (inner classes) have access to other members of the enclosing class, even if they are declared private. Static nested classes do not have access to other members of the enclosing class. As a member of the OuterClass, a nested class can be declared private, public, protected, or package-private. (Recall that outer classes can only be declared public or package-private.)
5.1 Why Use Nested Classes?
Compelling reasons for using nested classes include the following:
- It is a way of logically grouping classes that are only used in one place: If a class is useful to only one other class, then it is logical to embed it in that class and keep the two together. Nesting such as “helper classes” makes their package more streamlined.
- It increases encapsulation: Consider two top-level classes, A and B, where B needs access to members of A that would otherwise be declared
private
. By hiding class B within class A, A’s members can be declared private and B can access them. In addition, B itself can be hidden from the outside world. - It can lead to more readable and maintainable code: Nesting small classes within top-level classes places the code closer to where it is used.
5.1.1 Static Nested Classes
As with class methods and variables, a static nested class is associated with its outer class. And like static class methods, a static nested class cannot refer directly to instance variables or methods defined in its enclosing class: it can use them only through an object reference. A static nested class interacts with the instance members of its outer class (and other classes) just like any other top-level class. In effect, a static nested class is behaviorally a top-level class that has been nested in another top-level class for packaging convenience.
Static nested classes are accessed using the enclosing class name:
OuterClass.StaticNestedClass
For example, to create an object for the static nested class, use this syntax:
OuterClass.StaticNestedClass nestedObject = new OuterClass.StaticNestedClass();
5.1.2 Inner Classes
As with instance methods and variables, an inner class is associated with an instance of its enclosing class and has direct access to that object’s methods and fields. Also, because an inner class is associated with an instance, it cannot define any static members itself.
Objects that are instances of an inner class exist within an instance of the outer class. Consider the following classes:
class OuterClass { ... class InnerClass { ... } }
An instance of InnerClass
can exist only within an instance of OuterClass
and has direct access to the methods and fields of its enclosing instance.
To instantiate an inner class, you must first instantiate the outer class. Then, create the inner object within the outer object with this syntax:
OuterClass.InnerClass innerObject = outerObject.new InnerClass();
5.2 Difference between Static Nested class and Inner class
Sl. No | Inner Class | Static Nested Class |
1. | Without an outer class object existing, there cannot be an inner class object. That is, the inner class object is always associated with the outer class object. | Without an outer class object existing, there may be a static nested class object. That is, static nested class object is not associated with the outer class object. |
2. | Inside normal/regular inner class, static members can’t be declared. | Inside static nested class, static members can be declared. |
3. | As main() method can’t be declared, regular inner class can’t be invoked directly from the command prompt. | As main() method can be declared, the static nested class can be invoked directly from the command prompt. |
4. | Both static and non static members of outer class can be accessed directly. | Only a static member of outer class can be accessed directly. |
5.2.1 Example of Static Inner Class
We will now see the use of a static nested class with the following code snippet.
package Classes; class OuterClass { static int x = 10; int y = 20; private static int z = 30; static class StaticNestedClass { void display() { // can access static member of outer class System.out.println("outer_x = " + x); // can access display private static member of outer class System.out.println("outer_private = "+ z); // The following statement will throw a compilation error as static inner classes cannot access not static members //System.out.println("outer_y = " + y); } } } public class StaticNestedClassDemo { public static void main(String[] args) { // accessing a static nested class OuterClass.StaticNestedClass obj = new OuterClass.StaticNestedClass(); obj.display(); } }
Output
outer_x = 10 outer_private = 30
5.2.2 Example of Inner Class
We will now see the use of an inner class with the following code snippet.
package Classes; class NonStaticOuterClass { static int x = 10; int y = 20; private int z = 30; // inner class class InnerClass { void display() { // can access static member of outer class System.out.println("outer_x = " + x); // can also access non-static member of outer class System.out.println("outer_y = " + y); // inner class can also access a private member of the outer class System.out.println("outer_private = " + z); } } } // Driver class public class InnerClassDemo { public static void main(String[] args) { // accessing an inner class NonStaticOuterClass obj = new NonStaticOuterClass(); NonStaticOuterClass.InnerClass innerObject = obj.new InnerClass(); innerObject.display(); } }
Output
outer_x = 10 outer_y = 20 outer_private = 30
6. Summary
In this tutorial, we understood the about static and non-static members of a class like static and non-static fields and methods. Further, we also understood about static and non-static blocks and static and non-static inner classes.
7. References
- https://docs.oracle.com/javase/tutorial/java/javaOO/nested.html
- https://www.careerride.com/java-static-and-non-static-fields-of-class.aspx
- https://www.javatpoint.com/static-nested-class
- https://beginnersbook.com/2013/05/static-vs-non-static-methods/
- https://www.java67.com/2012/10/nested-class-java-static-vs-non-static-inner.html
7. Download the source code
The following code shows the usage of static and non-static variables, methods, blocks, and nested classes.
You can download the full source code of this example here: Static vs Non-Static in Java
I’m not sure about the waste of memory of this piece of code:
NonStaticEmployee emp1 = new NonStaticEmployee(1001,”Aakash Pathak”,”Webcodegeeks”);
NonStaticEmployee emp2 = new NonStaticEmployee(1002,”Robert Bernes”,”Webcodegeeks”);
Does Java not use String Constant Pool in this situation?