Simple while loop Java Example (with video)
1. Introduction
With this example we are going to demonstrate how to use a simple while loop Java statement. The while
statement continually executes a block of statements while a particular condition is true. In short, to use a simple while
loop you should:
- Create a
while
statement that evaluates an expression, which must return a boolean value and has a statement. - If the expression evaluates to true, the while statement executes the statement in the while block.
- The
while
statement continues testing the expression and executing its block until the expression evaluates to false.
You can also check Loops in Java in the following video:
Let’s take a look at the code snippet that follows:
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
|
package com.javacodegeeks.snippets.basics; public class SimpleWhileLoopExample { public static void main(String[] args) { int i = 0 ; int max = 5 ; System.out.println( "Counting to " + max); while (i < max) { i++; System.out.println( "i is : " + i); } } } |
Output
Counting to 5
i is : 1
i is : 2
i is : 3
i is : 4
i is : 5
2. Control Flow Diagram
In this flow given below, as part of while loop execution, at first, the test expression which evaluates to boolean value is executed. If the boolean condition evaluates to True, then the statements inside the body of the loop will be executed. Once the execution of statement(s) inside the loop gets over, then the test expression is again evaluated. Only when the condition evaluates to False, then the execution of the loop terminates.
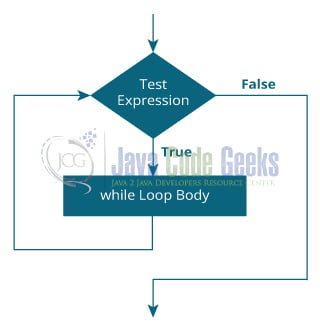
3. While loop with assignment
In this section let us get to know a program which utilizes the while loop with assignment
public class WhileWithAssignment { public static void main(String[] args) { boolean var=true; while(var=getNext()) { System.out.println("Inside while"); var=true; } System.out.println("Outside while, value of var is: "+var); } public static boolean getNext() { return false; } }
Output
Outside while, value of var is: false
First, the variable var is initialized with true. In the parenthesis of while, getNext() method is called. It returns false and assigns false to the variable var. Finally, the statement after while loop is executed.
4. More articles
5. Download the Source Code
This is an example of Simple While Loop in Java.
You can download the full source code of this example here: Simple while loop Java Example
Last updated on Apr. 29th, 2021