Simple Do While loop Java Example
1. Introduction
A do-while loop in Java is a control flow statement that executes a block of code at least once, and then repeatedly executes the block, or not, depending on a given boolean condition at the end of the block.
The do while construct consists of a process symbol and a condition. First, the code within the block is executed, and then the condition is evaluated. If the condition is true the code within the block is executed again. This repeats until the condition becomes false.
You can also check Loops in Java in the following video:
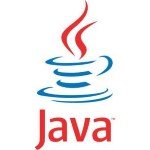
Because do while loops check the condition after the block is executed, the control structure is often also known as a post-test loop. In contrast with the while loop, which tests the condition before the code within the block is executed, the do-while loop is an exit-condition loop. This means that the code must always be executed first and then the expression or test condition is evaluated. If it is true, the code executes the body of the loop again. This process is repeated as long as the expression evaluates to true. If the expression is false, the loop terminates and control transfers to the statement following the do-while.
2. Do While loop Java Example
With this example, we are going to demonstrate how to use a simple do while
statement. The do while
statement continually executes a block of statements while a particular condition is true. The difference between do while and while
is that do-while
evaluates its expression at the bottom of the loop instead of the top. Therefore, the statements within the do
block are always executed at least once. In short, to use a simple do-while
statement you should:
Create your do-while
block and write the code to be executed in the do
statement and then the expression to be checked in the while block.
Here is the syntax:
do{ //code } while(condition);
Let’s take a look at the code snippet that follows:
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
20
|
package com.javacodegeeks.snippets.basics; public class SimpleDoWhileExample { public static void main(String[] args) { int i = 0 ; int max = 5 ; System.out.println( "Counting to " + max); do { i++; System.out.println( "i is : " + i); } while (i < max); } } |
Output:
Counting to 5
i is : 1
i is : 2
i is : 3
i is : 4
i is : 5
3. Download the Source Code
This was an example of how to use a simple do-while
statement in Java.
You can download the full source code of this example here: Simple Do While loop Java Example
Last updated on Jan. 29th, 2020