Round a Double to Two Decimal Places in Java
When working with financial or mathematical data in Java, it’s often necessary to truncate or round floating-point numbers to a specific number of decimal places. In many cases, we might want to truncate a double
to just two decimal places for display or storage purposes. This article will explore several approaches to achieve this task in Java.
1. Using DecimalFormat(“0.00”)
Java’s DecimalFormat
class provides a straightforward way to format numeric values. We can use it to truncate a double
to two decimal places.
public class TruncateDoubleWithDecimalFormat { public static void main(String[] args) { double number = 123.456789; DecimalFormat decimalFormat = new DecimalFormat("0.00"); System.out.println("Truncated number: " + decimalFormat.format(number)); decimalFormat.setRoundingMode(RoundingMode.DOWN); System.out.println("Truncated number with RoundingMode.DOWN: "+ decimalFormat.format(number)); decimalFormat.setRoundingMode(RoundingMode.UP); System.out.println("Truncated number with RoundingMode.UP: "+ decimalFormat.format(number)); } }
In this approach, we create a DecimalFormat
object with the pattern "0.00"
, which specifies that we want to keep exactly two decimal places. The 0
represents a required digit, so if there are fewer digits than the format specifies, leading zeroes will be added. This means that even if the input number has fewer than two decimal places, it will still be formatted to have two decimal places. Then, we use format()
method to format the double
number.
We set the rounding mode of the DecimalFormat
object to RoundingMode.DOWN
, which specifies that we want to truncate the decimal part without rounding up. We also set the rounding mode of the DecimalFormat
object to RoundingMode.UP
, which specifies that we want to round up the decimal part if it’s equal to or greater than 0.5.
The output from running the above code is:
Truncated number: 123.46 Truncated number with RoundingMode.DOWN: 123.45 Truncated number with RoundingMode.UP: 123.46
2 Using DecimalFormat(“#.##”)
We can also use a different pattern in DecimalFormat
to achieve the same result with a slightly different behaviour.
double number = 123.456789; DecimalFormat decimalFormat = new DecimalFormat("#.##"); System.out.println("Truncated Number using Pattern #.## is: " + decimalFormat.format(number));
In this approach, the pattern "#.##"
specifies that we want to keep up to two decimal places, but unlike "0.00"
, the #
represents an optional digit. This means that if the input number has fewer than two decimal places, they will not be displayed in the output.
2.1 Explanation of the Differences Between 0.00 and #.##
The key difference between these two approaches lies in how they handle numbers with fewer than two decimal places.
- With
DecimalFormat("0.00")
, the output always contains exactly two decimal places, potentially adding trailing zeroes if the input number has fewer than two decimal places. - With
DecimalFormat("#.##")
, the output only includes decimal places if they are present in the input number, resulting in a more compact representation but potentially losing precision if the input number has more than two decimal places.
Example showing the differences
double number1 = 123.4; double number2 = 123.40; DecimalFormat decimalFormat = new DecimalFormat("0.00"); DecimalFormat decimalFormat2 = new DecimalFormat("#.##"); System.out.println("123.4 output with 0.00: " + decimalFormat.format(number1)); System.out.println("123.4 output with #.##: " + decimalFormat2.format(number1)); System.out.println("123.40 output with 0.00: " + decimalFormat.format(number2)); System.out.println("123.40 output with #.##: " + decimalFormat2.format(number2));
Output is:
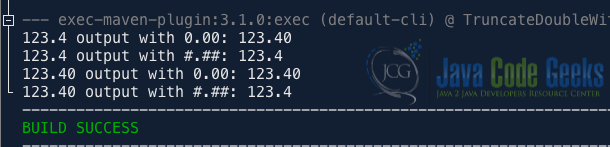
3. Using BigDecimal Class
BigDecimal
provides precise numerical operations and is suitable for financial calculations. We can use it to truncate a double
to two decimal places like this:
public class BigDecimalExample { public static void main(String[] args) { double number = 123.456789; BigDecimal bigDecimal = new BigDecimal(number); BigDecimal truncated = bigDecimal.setScale(2, BigDecimal.ROUND_DOWN); BigDecimal truncated2 = bigDecimal.setScale(2, BigDecimal.ROUND_UP); System.out.println("Truncated number using BigDecimal.ROUND_DOWN: " + truncated); System.out.println("Truncated number using BigDecimal.ROUND_UP: " + truncated2); } }
In this approach, we create a BigDecimal
object from the double
number, then use setScale()
method to specify the scale (number of decimal places) and BigDecimal.ROUND_DOWN
rounding mode to truncate the number.
Output:
Truncated number using BigDecimal.ROUND_DOWN: 123.45 Truncated number using BigDecimal.ROUND_UP: 123.46
4. Using Math.round
Another approach is to use the Math.round()
method to round the number to two decimal places. This method leverages multiplication and division to achieve truncation.
public class TruncateDoubleWithMaths { public static void main(String[] args) { double number = 123.456789; double truncated = Math.round(number * 100.0) / 100.0; System.out.println("Truncated number: " + truncated); } }
Here, we multiply the number by 100 to shift the decimal point two places to the right, then round it using Math.round()
, and finally divide by 100.0 to shift the decimal point back two places to the left.
5. Using String.format()
This approach uses string manipulation for formatting. Java’s String.format()
method allows us to format a string representing a double
with a specified number of decimal places like this:
public class TruncateDoubleWithStringManipulation { public static void main(String[] args) { double number = 123.456789; String truncated = String.format("%.2f", number); double result = Double.parseDouble(truncated); System.out.println("String Manipulation truncated number: " + result); } }
In this approach, %.2f
specifies that we want to format the number with two decimal places using floating-point format.
Output:
String Manipulation truncated number: 123.46
6. Conclusion
Truncating a double
to two decimal places in Java can be achieved using various approaches as shown in this article. Each approach has its advantages and may be more suitable depending on the specific requirements of your application. Choose the approach that best fits your needs in terms of performance, readability, and maintainability.
7. Download the Source Code
This was an example of how to Round a Double to Two Decimal Places in Java.
You can download the full source code of this example here: Round a Double to Two Decimal Places in Java