@Override Java Annotation Example
In this article, we will speak about the override annotation, which is used as @Override in a Java program. Firstly, we will talk about what is the override in OOP in general and why we use it. Then we will analyze why we do overrides in methods and last but not least we will do some examples.
1. Introduction
In Java, the method overriding is one of many ways that achieve the Run Time Polymorphism. Overriding is a capability of Java which allow a child class or subclass to provide a specific implementation of a method that is provided already by a parent class or super-class. In other words when a method of a subclass has the same name, parameters and return type with a method of super-class then we can say that the method of the subclass overrides the method of the super-class.
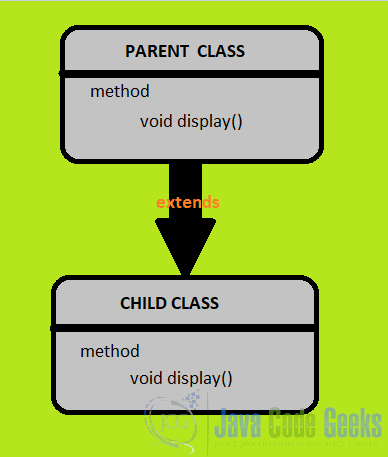
2. Technologies used
The example code in this article was built and run using:
- Java 1.8.231(1.8.x will do fine)
- Eclipse IDE for Enterprise Java Developers-Photon
3. Why override methods in your program
The reason that we use override methods is that a child class can give its own implementation to a method that is already created by the parent class or super-class. In this case, the method in the child class is called overriding method and the method in the parent class is called overridden method. Here is an example:
Animal.java
public class Animal { public void sleep() { System.out.println("Sleeping"); } }
Lion.java
public class Lion extends Animal{ public void sleep(){ System.out.println("Lion is sleeping"); } public static void main( String args[]) { Lion obj = new Lion(); obj.sleep(); } }
The output is:
Lion is sleeping
In this example, we can see that there are two methods with the same signature but at the output, it prints the sentence of the method of the lion class. This is happens because of the new object “obj” that is declared as Lion object.
4. What does @Override annotation do and why you should use it in Java
In Java, the Override annotation is used when we override a method in a subclass. The reason that we use the @Override annotation is:
- If the programmer makes any mistake such a wrong parameter type or a wrong method name then he would get a compile-time error. With the annotation, you instruct the compiler that you are overriding the method so if you don’t use it then the subclass method would behave as a new method.
- It will improve the readability of the code. For example, if you have many classes then the annotation would help you to identify the classes that require changes when you change the signature of a method.
Here you can see an example of the @Override annotation:
Parent.java
public class Parent { public void display(String message){ System.out.println(message); } }
Parent.java
public class Child extends Parent{ @Override public void display(String message){ System.out.println("The message is: "+ message); } public static void main(String args[]){ Child obj = new Child(); obj.display("Hello world!!"); } }
The output is:
The message is: Hello world!!
5. How to declare a method that it should never be overridden
When we want a method not to be overridden we can use the final modifier. The final methods have the ability that it cannot be overridden. So you cannot modify a final method from a subclass. The reason that we use final methods is that we don’t want to change by any outsider. Here is an example:
FinalExample.java
public class FinalExample { public final void display(){ System.out.println("FinalExample method"); } public static void main(String args[]){ new FinalExample().display(); } }
Finalex.java
public class Finalex extends FinalExample { public void display(){ System.out.println("Finalex method"); } }
In this example at Finalex class, the Eclipse will show this message “Cannot override the final method from FinalExample”.
6. Download the Source Code
This was the example of the Override Java Annotation.
You can download the full source code of this example here: @Override Java Annotation Example