Modules of Spring Architecture
In this article, we’ll look at the Spring Framework architecture, how its modules works, and the benefits of using this powerful environment.
1. Introduction
Since Spring Framework is a Java based platform, it provides a comprehensive and solid software infrastructure while we can focus on the aspects of our application. In other words, Spring Framework handle all the base of the application and the developer can works more comfortably, without concern about specific technical stuff.
The next section we going to discuss the most important modules and how they work together to support our application.
2. Modules
Below we can see a chart that shows us the principal modules in Spring Framework:
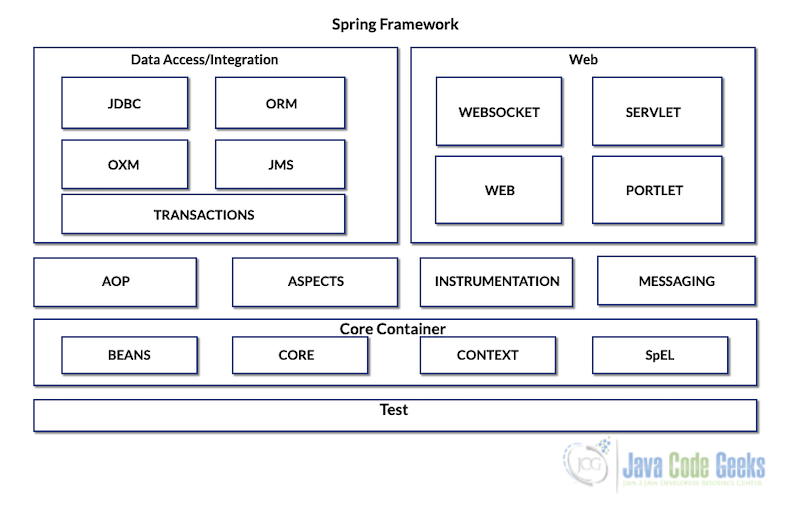
As we notice, Spring Framework divides its modules in these layers:
- Core Container – having the fundamental parts of the framework.
- Data Access/Integration – the layer to access databases and transaction modules.
- Web – responsible to deal with web-oriented integration such as HTTP connections, Model-View-Controller (MVC) structures and REST Web services.
- Miscellaneous – a few other modules but important to know about:
- AOP – Aspect-Oriented Programming
- Aspects
- Instrumentation
- Messaging
- Test
In summary, Spring Framework has about 20 modules and sub-modules in his architecture. In the following sessions, we’ll dive in all of them and see their usability.
3. Core Container
We should call the Core Container layer as the “heart” of Spring Framework. To clarify, this module owns the most used implementations of the Spring Framework that will be used across the entire application for sure.
This layer consists of the modules: spring-core
, spring-beans
, spring-context
and spring-expression
(Spring Expression Language).
3.1 Core and Beans modules
Inversion of Control (IoC) also known as Dependency Injection (DI) is present in spring-core
module. The org.springframework.beans
and org.springframework.context
packages are the basis for Spring Framework’s IoC container.
In Spring, the objects that form the backbone of our application and that are managed by the Spring IoC container are called beans. A bean is an object that is instantiated, assembled, and managed by a Spring IoC container. Otherwise, a bean is simply one of many objects in your application. Beans, and the dependencies among them, reflect in the configuration metadata used by a container.
More details about Core Container and Beans can be find here.
3.2 Context module
The Core and Beans modules provide objects to the Context module and it access any objects defined and configured. The org.springframework.context.ApplicationContext
interface is the focal point of the Context module.
This module inherits its features from the Beans module and adds support for internationalization (using, for example, resource bundles), event propagation, resource loading, and the transparent creation of contexts by, for example, a Servlet container.
3.3 Spring Expression Language (SpEL)
The Spring Expression Language (SpEL) is a powerful expression language that supports querying and manipulating an object graph at runtime.
While there are several other Java expression languages available — OGNL, MVEL, and JBoss EL, to name a few — Spring community creates the Spring Expression Language to provide a single well supported expression language that can be used across all the products in the Spring portfolio.
The language supports setting and getting property values, property assignment, method invocation, accessing the content of arrays, collections and indexers, logical and arithmetic operators, named variables, and retrieval of objects by name from Spring’s IoC container. It also supports list projection and selection as well as common list aggregations.
4. Data Access/Integration
The Data Access/Integration layer consists of the JDBC, ORM, OXM, JMS, and Transaction modules. In other words, all access to data and method to retrieve and transform it are find in this module. Let’s see its modules below.
4.1 JDBC
Spring JDBC Framework (spring-jdbc
) takes care of all the low-level details starting from opening the connection, prepare and execute the SQL statement, process exceptions, handle transactions and finally close the connection.
JdbcTemplate is the classic Spring JDBC approach and the most popular. This “lowest level” approach and all others use a JdbcTemplate under the covers.
The org.springframework.jdbc.core
package contains the JdbcTemplate class and its various callback interfaces, plus a variety of related classes. See more details in Data Access with JDBC documentation.
4.2 ORM
This module (spring-orm
) provides the most popular object-relational mapping APIs including JPA, JDO and Hibernate. Certainly, the major goal of Spring’s ORM is clear the application layering, with any data access and transaction technology, and for loose coupling of application objects.
Spring adds significant enhancements to the ORM layer of our choice when creating data access applications. Further, we can use much of the ORM support as we would in a library, regardless of technology, because everything is designed as a set of reusable JavaBeans.
Examples of ORM Framework can be find here.
4.3 OXM
Object/XML Mapping, or O/X mapping for short (spring-oxm
), is the act of converting an XML document to and from an object. This conversion process is also known as XML Marshalling, or XML Serialization.
4.3.1 Marsheller and Unmarsheller
A marshaller serializes an object to XML, and an unmarshaller deserializes XML stream to an object. The interfaces used to do it are org.springframework.oxm.Marshaller
and org.springframework.oxm.Unmarshaller
.
These abstractions allow you to switch O/X mapping frameworks with relative ease, with little or no changes required on the classes that do the marshalling. Additionally, this approach has the benefit of making it possible to do XML marshalling with a mix-and-match approach (e.g. some marshalling performed using JAXB, other using XMLBeans) in a non-intrusive fashion, leveraging the strength of each technology.
4.4 Transaction Management
The spring-tx
module supports programmatic and declarative transaction management for classes that implement special interfaces and for all our POJOs (Plain Old Java Objects).
Basically, developers have had two choices for transaction management: global or local transactions, both of which have profound limitations.
4.4.1 Global and Local Transactions
Global transactions enable you to work with multiple transactional resources, typically relational databases and message queues. In addition, the application server manages global transactions through the JTA (Java Transaction API), which is a heady API to use (partly due to its exception model).
Local transactions are resource-specific, such as a transaction associated with a JDBC connection. Therefore it is easier to use, still have significant disadvantages: they cannot work across multiple transactional resources.
Spring resolves these disadvantages, enabling developers to use a consistent programming model in any environment. You write your code once, and it can benefit from different transaction management strategies in different environments.
More details about how Spring use Transaction Management here.
4.5 JMS
Java Messaging Service (spring-jms
), JMS for short, is the module that contains features for producing and consuming messages. Since Spring Framework 4.1, it provides integration with the spring-messaging
module.
JMS can be roughly divided into two areas of functionality, namely the production and consumption of messages. The JmsTemplate
class is used for message production and synchronous message reception. For asynchronous reception similar to Java EE’s message-driven bean style, Spring provides a number of message listener containers that are used to create Message-Driven POJOs (MDPs).
The JmsTemplate
class is the central class in the JMS core package. In other words, it simplifies the use of JMS since it handles the creation and release of resources when sending or synchronously receiving messages.
5. Web
The Web layer consists of the spring-web
, spring-webmvc
, spring-websocket
, and spring-webmvc-portlet
modules.
5.1 Web MVC and Servlet Framework
The spring-web
module provides basic web-oriented integration features such as multipart file-upload functionality and the initialization of the IoC container using servlet listeners and a web-oriented application context.
The Spring Web model-view-controller (MVC) framework is designed around a DispatcherServlet
that dispatches requests to handlers, with configurable handler mappings, view resolution, locale, time zone and theme resolution as well as support for uploading files.
The default handler is based on the @Controller
and @RequestMapping
annotations, offering a wide range of flexible handling methods. With the introduction of Spring 3.0, the @Controller
mechanism also allows you to create RESTful Web sites and applications, through the @PathVariable
annotation and other features.
5.2 WebSocket
The Web Socket protocol RFC 6455 defines an important new capability for web applications: full-duplex, two-way communication between client and server.
It is a new capability on the heels of a long history of techniques to make the web more interactive including Java Applets, XMLHttpRequest, Adobe Flash, ActiveXObject and others.
Spring Framework 4 includes a new spring-websocket
module with comprehensive WebSocket support. Also, it is compatible with the Java WebSocket API standard (JSR-356) and also provides additional value-add as explained in the rest of the introduction.
The best fit for WebSocket is in web applications where the client and server need to exchange events at high frequency and with low latency. Prime candidates include: applications in finance, games, collaboration, and others.
Take a look in the documentation right here.
5.3 Portlet
The spring-webmvc-portlet
module is an additional support to the conventional (servlet-based) Web development, implementing JSR-286 Portlet development.
The Portlet MVC framework is a mirror image of the Web MVC framework, and also uses the same underlying view abstractions and integration technology.
The main way in which portlet workflow differs from servlet workflow is that the request to the portlet can have two distinct phases: the action phase and the render phase.
Read details about this module in the documentation here.
6. Miscellaneous
Here we’ll see some modules that make part of Spring Architecture.
6.1 Aspect-Oriented Programming
Aspect-Oriented Programming (AOP) complements Object-Oriented Programming (OOP) by providing another way of thinking about program structure. The key unit of modularity in OOP is the class, whereas in AOP the unit of modularity is the aspect.
While the Spring IoC container does not depend on AOP, meaning you do not need to use AOP if you don’t want to, AOP complements Spring IoC to provide a very capable middleware solution.
6.1.1 AOP Terminologies
AOP has some specific terminologies that we can see below:
- Aspect – This is a module that has a set of APIs providing cross-cutting requirements. For example, a logging module would be called the AOP aspect for logging. An application can have any number of aspects depending on the requirement.
- Join point – This represents a point in your application where you can plug-in the AOP aspect. To clarify, it is the current place in the application where an action will be taken using the Spring AOP framework.
- Advice – This is the actual action to be taken either before or after the method execution. Therefore, it is the piece of code that is invoked during the program execution by the Spring AOP framework.
- Pointcut – This is a set of one or more join points where advice should be executed. We can specify “pointcuts” using expressions or patterns as we will see in our AOP examples.
- Introduction – An introduction allows you to add new methods or attributes to the existing classes.
- Target object – The object being advised by one or more aspects. This object will always be a proxied object, also referred to as the advised object.
- Weaving – Weaving is the process of linking aspects with other application types or objects to create an advised object. This can be done at compile time, load time, or at runtime.
6.1.2 Advice types
Still talking about Advices, we have these types that Spring Framework can use:
- before – Run advice before the method execution.
- after – Run advice after the method execution, regardless of its outcome.
- after-returning – Run advice after the method execution only if the method completes successfully.
- after-throwing – Run advice after the method execution only if the method exits by throwing an exception.
- around – Run advice before and after the advised method is invoked.
See AOP documentation to more details.
6.2 Instrumentation
The Instrumentation module (spring-instrument
) provides class instrumentation support and classloader implementations to be used in certain application servers.
The spring-instrument-tomcat
module contains Spring’s instrumentation agent for Tomcat.
6.3 Messaging
The Spring Integration Message (spring-message
) is a generic container for data. Any object can be provided as the payload, and each Message
instance includes headers containing user-extensible properties as key-value pairs.
While the interface Message
plays the crucial role of encapsulating data, it is the MessageChannel
that decouples message producers from message consumers.
6.4 Test
The spring-test
module supports the unit testing and integration testing of Spring components with JUnit or TestNG. It provides consistent loading of Spring ApplicationContext
s and caching of those contexts. It also provides mock objects that you can use to test your code in isolation.
7. Summary
In this article, we saw the most important and used Spring Framework modules. Further, we could have an idea of each module’s usability and understand how they work together in the Spring environment.
This article was based on Spring Framework official documentation that can be found in this link.