Java Variables Tutorial
1. Introduction
In this article, we will look at one of the basic concepts of Java known as variables. We will see what java variable types mean, memory storage, types of variables, and some examples.
2. What is a Variable?
In Java, a variable is a container to hold data values during execution of a java program. All variables have a data type associated with it. The data type associated with a variable determines:
- the memory it requires.
- how it is stored in memory.
- the range of values that that variable can hold.
- the operations or methods that can be applied to it.
We need to at least declare the variable in Java. Only then we can use it. Depending on the datatype, it may be auto initialized. The basic syntax of declaring a variable in java is as follows:
<data-type> <variable_name> [ = value][, <variable_name> [ = value] ...] ;
We declare variables one on each line or multiple in a single line. Both syntaxes are valid.
Some examples of variables are
int myNumber = 10;
float b= 0.0, char c = ‘a’ , int num =10;
Here the int, char and float are the data-types. myNumber, b and num are variable names and 10 and 0.0 are the values.
3. Types of Variables
There are 4 types of variables in Java
- Instance variables (Non-Static Fields): Non-static fields/variables declared by objects are instance variables. They are unique to the object that creates them.
- Class variables (Static Fields): Static fields/variables declared by a class. There will only always be a single copy of this variable during the execution of a java program, no matter the instances the class has. Usually, such variables are also final i.e. they are constants.
- Local variables: Another name for local variables is method variables. All the variables that a method uses are local variables. These variables are visible only inside the method that created them.
- Parameters: The variables that a method uses to pass the value(s) to another method are Parameters. They are available only to the method that passes them.
- Constants: These are usually class variables that are static and final. We cannot change a constant’s value once assigned.
4. How does Java store variables?
A Java Program uses the Heap and stack memories. Java stores variables either on the stack or the heap depending on their type.
- Local variables go to the stack.
- Instance variables live on the heap memory along with the object that created them.
- Static or class variables live on the heap memory.
- Parameters go to on the heap memory along with the method.
5. Naming conventions
Developers need to follow certain rules or naming conventions for variables. They are as follows:
- Variables can be an unlimited sequence of Unicode characters and letters. However, it is the convention to give short names for variables.
- Variable names can start with the dollar symbol i.e $ and the underscore symbol i.e. _ . However, this is discouraged. In Java, by convention variables should start with letters, and the use of the $ is discouraged altogether.
- Single character variable names should be avoided unless the variables are temporary variables like the for-loop variable etc. In general, the variable should be such that it is self-explanatory.
- Variable names with just one word should be all lowercase. If the variable name contains more words, then the first character of the next word should be capitalized. Eg: int number=10; int minValue =0;.
- Constant variable names should all be uppercase. If the variable is more than one word, then it should be connected using underscores. E.g. static final double PI= 3.1415926536; static final MIN_AGE=18;
6. Examples of Java Variables
Let us look at examples of the various types of variables to see how they work.
6.1 Local variables
Local variables are the variables that methods use. Local variables need an initial value. If there is no initial value, the java program throws a compilation error. It will run only when we give it a suitable value.
VariablesMain.java
public class VariablesMain{ public static void main(String[] args){ //This is a local variable. It needs to be initialised. //If below line is written as below the code fails: //int sum; int sum = 10; System.out.print("The value of sum is: " + sum); } }
The output of this program is as follows:
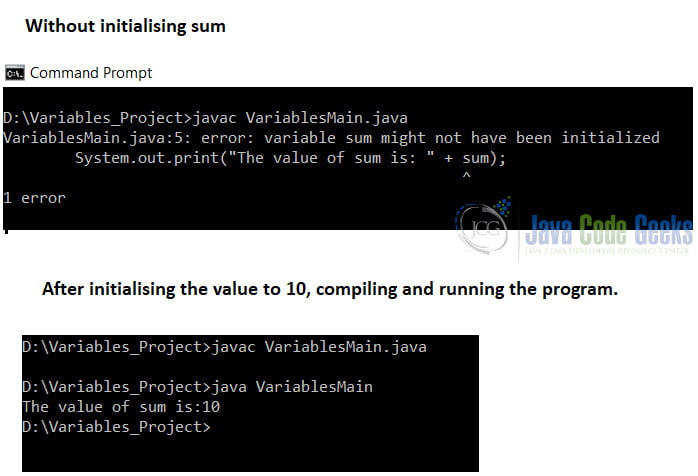
Local variables do not have to be given direct values. They are also used for getting the “return” value of a method call. An example of this is the variable result in the code below.
VariablesMain.java
public class VariablesMain{ public static void main(String[] args){ int number1 = 10,number2 = 30; int result = calculateSum(number1,number2); System.out.println("The value of sum is: " + result); } public static int calculateSum(int num1,int num2){ int result = num1+num2; return result; } }
The result of this program is that the sum is 40.
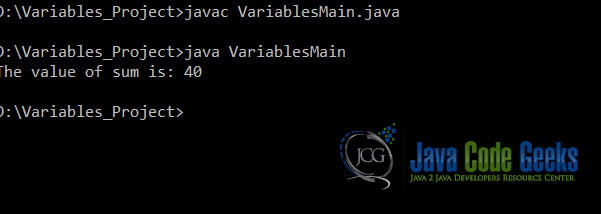
6.2 Parameters
Parameters are the variables that methods use to pass values from one method to another. Since the parameters are used inside the method during runtime, it is required to initialize them beforehand. One other possibility is that the parameters get their actual value during runtime and are initialized with their default values.
As an example, as we saw in the previous example:
VariablesMain.java
public class VariablesMain{ public static void main(String[] args){ int number1 = 10,number2 = 30; int result = calculateSum(number1,number2); System.out.println("The value of sum is: " + result); } public static int calculateSum(int num1,int num2){ int result = num1+num2; return result; } }
Here the number1 and number2 are passed as parameters to the calculateSum() method. Here, as we see the parameter names in the calculateSum method definition are different i.e. num1 and num2 which is valid. Parameters are just the copies of the values to be passed to the method. In the above code, the String[] args is also an example of a parameter of the method main. The String[] args is initialized with the default value of “null”. The actual value can be passed during runtime as follows:
MainParameters.java
public class MainParameters{ public static void main(String[] args){ if(args !=null && args.length !=0){ System.out.println("The file name is:: " + args[0]); }else{ System.out.println("No file name given!!"); } } }
While executing this program we can give a filename as the argument. If it is not mentioned, then the output “No filename is given!!”. If the code above is not written then if no filename is set then the code will fail with the “Index out of bounds” exception. Edited source code i.e. with no if…else
MainParameters.java
public class MainParameters{ public static void main(String[] args){ System.out.println("The file name is:: " + args[0]); } }
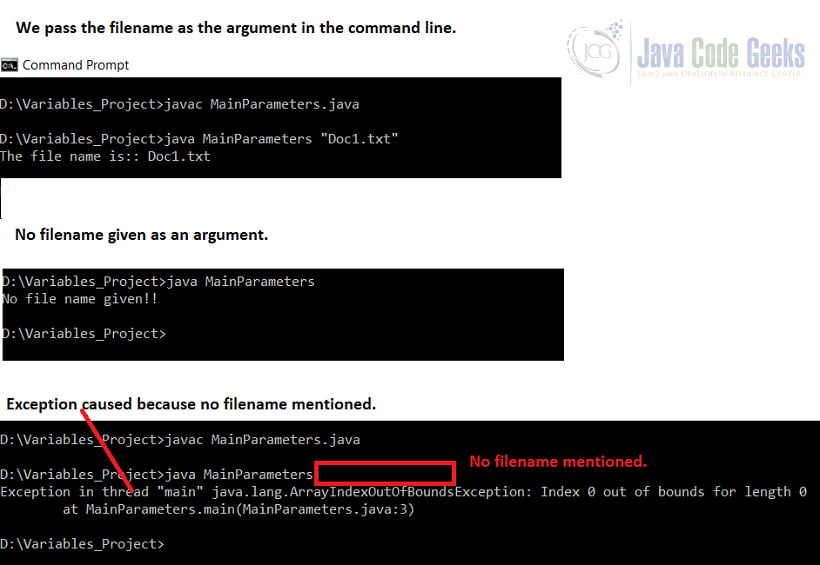
6.3 Instance variables
Instance variables are variables that are declared in a class and are not inside any method. These instance variables need not be initialized. They are created and destroyed by the object that creates them.
As an example, we have a Car class that has the instance variables: full capacity, carColor and type. We also have getter-setters for each variable which we will use to change and display their value. The MainClass.java is a car sales dealer class. The inventory is displayed.
MainClass.java
public class MainClass { public static void main(String[] args) { Car car1 = new Car(); car1.setColor("black"); car1.setFuelCapacity(55); car1.setType("corolla"); System.out.println("Car1 specs are: " + car1.getColor() +" " + car1.getType()+ " with a fuel capacity of " + car1.getFuelCapacity()); Car car2 = new Car(); car2.setColor("silver"); car2.setFuelCapacity(50); car2.setType("camry"); System.out.println("Car2 specs are: " + car2.getColor() +" " + car2.getType()+ " with a fuel capacity of " + car2.getFuelCapacity()); Car car3 = new Car(); car3.setColor("grey"); car3.setFuelCapacity(70); car3.setType("innova"); System.out.println("Car 3 specs are: " + car3.getColor() +" " + car3.getType()+ " with a fuel capacity of " + car3.getFuelCapacity()); } }
Car.java
public class Car { //instance variables. private int fuelCapacity; private String carColor; private String type="car"; public int getFuelCapacity(){ return this.fuelCapacity; } public void setFuelCapacity(int fuelCapacity){ this.fuelCapacity = fuelCapacity; } public String getColor(){ return this.carColor; } public void setColor(String color){ this.carColor = color; } public String getType(){ return this.type; } public void setType(String type){ this.type = type; } }
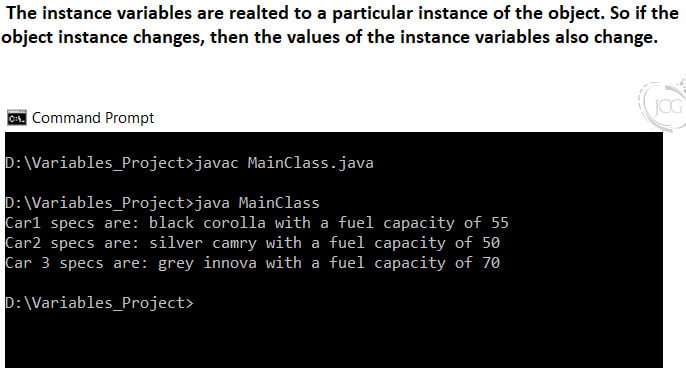
6.4 Static variables
Class fields or variables marked with the keyword static are static variables. There is only one copy of static variables across all the instances of the class. To understand this concept, we modify the code in the earlier example of Cars. We will introduce a new variable called max_gears. Max_gears is a static variable. For using this variable, we do not use the instance variables car1, car2, and car3. We use the Class name i.e. Car.
Car.java
public class Car { static int max_gears = 10; //instance variables. private int fuelCapacity; private String carColor; private String type="car"; public int getFuelCapacity(){ return this.fuelCapacity; } public void setFuelCapacity(int fuelCapacity){ this.fuelCapacity = fuelCapacity; } public String getColor(){ return this.carColor; } public void setColor(String color){ this.carColor = color; } public String getType(){ return this.type; } public void setType(String type){ this.type = type; } }
MainClass.java
public class MainClass { public static void main(String[] args) { Car car1 = new Car(); car1.setColor("black"); car1.setFuelCapacity(55); car1.setType("corolla"); Car car2 = new Car(); car2.setColor("silver"); car2.setFuelCapacity(50); car2.setType("camry"); Car car3 = new Car(); car3.setColor("grey"); car3.setFuelCapacity(70); car3.setType("innova"); //To use the max_gears we use the class name i.e “Car” System.out.println("The original value of the max_gears is: " + Car.max_gears); } }
There is only one copy of the static variable. To see this in action we will change the value of the max_gears variable and see the value of the variable for every car instance. The Car class remains the same here.
MainClass.java
public class MainClass { public static void main(String[] args) { Car car1 = new Car(); car1.setColor("black"); car1.setFuelCapacity(55); car1.setType("corolla"); Car car2 = new Car(); car2.setColor("silver"); car2.setFuelCapacity(50); car2.setType("camry"); Car car3 = new Car(); car3.setColor("grey"); car3.setFuelCapacity(70); car3.setType("innova"); System.out.println("The original value of the max_gears is: " + Car.max_gears); //Setting the static variable to a new value. Car.max_gears = 40; //Affects all the class instances since there is one copy of the variable. //This method of referencing static variables is not correct, this is just as an example. System.out.println("The original value of the max_gears for car1 is: " + car1.max_gears); System.out.println("The original value of the max_gears for car2 is: " + car2.max_gears); System.out.println("The original value of the max_gears for car3 is: " + car3.max_gears); } }
As we see in the output the value of the max_gears does not change with every instance of the car object.
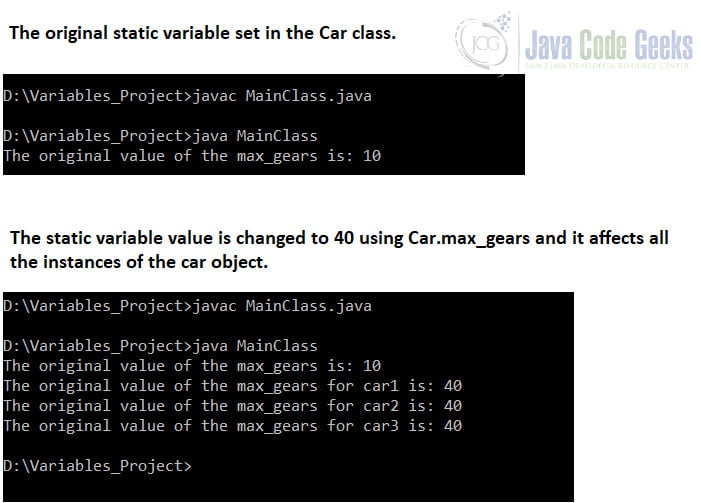
6.5 Constant variables
Usually, static variables are also constant in value. Just marking a variable as static is not enough since static variables can still be changed. To make a constant we use the final keyword. We write constant variables in all caps and separate words using underscores. Changing the final variable results in an exception. To see this action, we have modified the earlier example of cars and the sales agency to make the max_gears variable into a final variable.
Cars.java
public class Car { //constant variable static final int MAX_GEARS = 10; //static variable //static int max_gears = 10; //instance variables. private int fuelCapacity; private String carColor; private String type="car"; public int getFuelCapacity(){ return this.fuelCapacity; } public void setFuelCapacity(int fuelCapacity){ this.fuelCapacity = fuelCapacity; } public String getColor(){ return this.carColor; } public void setColor(String color){ this.carColor = color; } public String getType(){ return this.type; } public void setType(String type){ this.type = type; } }
We have changed the max_gears variables to MAX_GEARS as it is constant. Just as static variables, there is only one variable copy for all class instances. So, the following still works.
MainClass.java
public class MainClass { public static void main(String[] args) { Car car1 = new Car(); car1.setColor("black"); car1.setFuelCapacity(55); car1.setType("corolla"); Car car2 = new Car(); car2.setColor("silver"); car2.setFuelCapacity(50); car2.setType("camry"); Car car3 = new Car(); car3.setColor("grey"); car3.setFuelCapacity(70); car3.setType("innova"); // System.out.println("The original value of the max_gears is: " + Car.max_gears); //Setting the static variable to a new value. //This is valid //Car.max_gears = 40; //Affects all the class instances since there is one copy of the variable. //This method of referencing static variables is not correct, this is just as an example. System.out.println("The original value of the max_gears for car1 is: " + car1.MAX_GEARS); System.out.println("The original value of the max_gears for car2 is: " + car2.MAX_GEARS); System.out.println("The original value of the max_gears for car3 is: " + car3.MAX_GEARS); } }
However, if we try to change the final value, it results in an exception. Here the Cars.java remains the same.
MainClass.java
public class MainClass { public static void main(String[] args) { Car car1 = new Car(); car1.setColor("black"); car1.setFuelCapacity(55); car1.setType("corolla"); Car car2 = new Car(); car2.setColor("silver"); car2.setFuelCapacity(50); car2.setType("camry"); Car car3 = new Car(); car3.setColor("grey"); car3.setFuelCapacity(70); car3.setType("innova"); // System.out.println("The original value of the max_gears is: " + Car.max_gears); //Setting the static variable to a new value. //This is valid //Car.max_gears = 40; //Affects all the class instances since there is one copy of the variable. // //This method of referencing static variables is not correct, this is just as an example. // System.out.println("The original value of the max_gears for car1 is: " + car1.MAX_GEARS); // System.out.println("The original value of the max_gears for car2 is: " + car2.MAX_GEARS); // System.out.println("The original value of the max_gears for car3 is: " + car3.MAX_GEARS); //Trying to Set a final variable with a new value will result in an exception. Car.MAX_GEARS = 40;
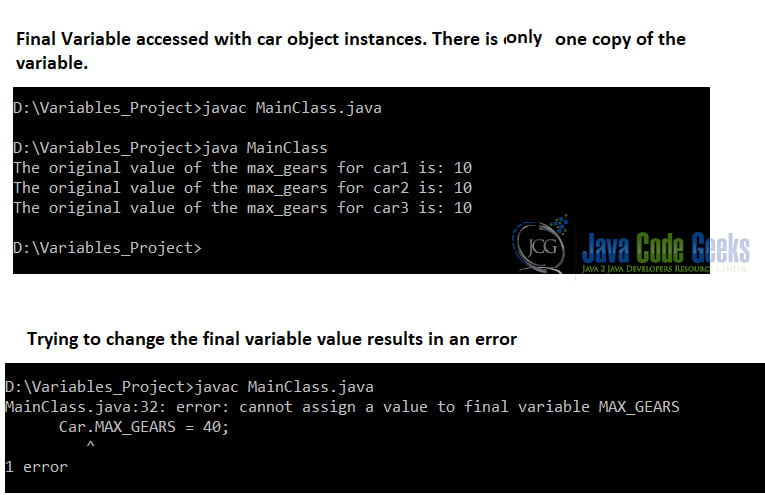
7. Summary
Java variable types form an integral part of Java Programs. Variables store data that is used later. We saw how variables are declared, how they are stored in memory, and also how they are initialized. We also saw that variables require datatypes that control what type of values get stored in variables.
8. Download the Source Code
These were examples of Java variables and java variable types.
You can download the full source code of this example here: Java Variables Tutorial