Java String Replace and ReplaceAll String Methods
In this example, we are going to see how to use the String Replace and ReplaceAll String class API Methods in Java. With replaceAll
, you can replace all character sequences and single characters from a String
instance.
As you know, String
objects are immutable. This means, that every time you try to change its value, a new String
object is created, holding the new value. So replaceAll will create a new String object and return a reference pointing to it.
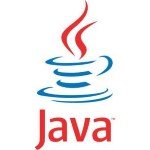
Let’s see some examples on Java String Replace and ReplaceAll String Methods.
1. Replacing single characters
Here is how you can replace all instances of a single character inside a String
.
public class StringReplaceAllExample { public static void main(String[] args) { String str = "This is a String to use as an example to present raplaceAll"; // replace all occurrences of 'a' with '@' String newStr = str.replaceAll("a", "@"); System.out.println(newStr); // replace all occurrences of 'e' with '3' newStr = newStr.replaceAll("e", "3"); System.out.println(newStr); // replace all occurrences of 't' with 'T' newStr = newStr.replaceAll("t", "T"); System.out.println(newStr); // remove all occurrences of 'o' newStr = newStr.replaceAll("o", ""); System.out.println(newStr); // replace all occurrences of 't' with 'That' newStr = newStr.replaceAll("T", "That"); System.out.println(newStr); } }
This will output:
This is @ String to use @s @n ex@mple to present r@pl@ceAll This is @ String to us3 @s @n 3x@mpl3 to pr3s3nt r@pl@c3All This is @ STring To us3 @s @n 3x@mpl3 To pr3s3nT r@pl@c3All This is @ STring T us3 @s @n 3x@mpl3 T pr3s3nT r@pl@c3All Thathis is @ SThatring That us3 @s @n 3x@mpl3 That pr3s3nThat r@pl@c3All
As you can see from the above example, replacement is a case sensitive operation, For example, when we replaced all occurrences of ‘a’, we didn’t replace ‘A’ in the original String
. Additionally, you can use replaceAll
to completely remove a character from the String
by replacing it with ""
. It’s also worth noting that if no occurrences of the targeted character are found, as a result replaceAll
will return the exact same String
, no new String
gets created.
The difference between replace and replaceAll is that the replace method either takes a pair of char or a pair CharSequence’s . The replace method will replace all occurrences of a char or CharSequence’s . Using the wrong function can lead to subtle bugs.
Here we can see a replace method example.
public class Replace { public static void main(String[] args) { String Str = new String("The dog plays with the ball."); System.out.println(Str.replace('a', 'T')); } }
The output is:
The dog plTys with the bTll.
2. Replacing char sequences character
Here is how you can replace all char sequences inside a String
with another char sequence or with a single character.
public class StringReplaceAllExample { public static void main(String[] args) { String str = "This is a String to use as an example to present raplaceAll"; // replace all occurrences of 'This' with 'That' String newStr = str.replaceAll("This", "That"); System.out.println(newStr); // replace all occurrences of 'String' with 'big String' newStr = str.replaceAll("String", "big String"); System.out.println(newStr); // remove all occurrences of 'is' newStr = str.replaceAll("is", ""); System.out.println(newStr); // remove all occurrences of 'replaceAll' newStr = str.replaceAll("raplaceAll", ""); System.out.println(newStr); } }
This will output:
That is a String to use as an example to present raplaceAll This is a big String to use as an example to present raplaceAll Th a String to use as an example to present raplaceAll This is a String to use as an example to present
3. Using regular expressions
This is a great feature of replaceAll
. You can use a regular expression to replace all occurrences of matching char sequences inside a String
.
public class StringReplaceAllExample { public static void main(String[] args) { String str = "This 1231 is 124 a String 1243 to 34563 use 5455"; // remove all numbers String newStr = str.replaceAll("[0-9]+", ""); System.out.println(newStr); // remove all words with 'Java' newStr = str.replaceAll("[a-zA-Z]+", "Java"); System.out.println(newStr); } }
This will output:
This is a String to use Java 1231 Java 124 Java Java 1243 Java 34563 Java 5455
Another example is:
public class ReplaceAllex { public static void main(String[] args) { String s="That is a String to use as an example to present raplaceAll" ; System.out.println(s); String str = s.replaceAll(" {2,}", " "); System.out.println(str); } }
The output is:
That is a String to use as an example to present raplaceAll That is a String to use as an example to present raplaceAll
The last example:
public class ReplaceAllDollar { public static void main(String[] args) { String s="The difference between dollars and euros is 0.09 dollars."; System.out.println(s); String str = s.replaceAll("dollars", "\\$"); System.out.println(str); } }
The output is:
The difference between dollars and euros is 0.09 dollars. The difference between $ and euros is 0.09 $.
4. Download the Source Code
You can download the full source code of this example here: Java String Replace and ReplaceAll String Methods
Last updated on May 12th, 2020
I want to replace Name with name in the Text but if i use replace method it is replacing LastName also with Lastname. But i want only Name only should be replaced.