Java String Methods from Java 8 to Java 14
In this article, we will look at the new string methods added to the Java API from versions 8 to 14.
1. Java String Methods – Java 8
The newly added method in Java 8 is join for joining up the various strings
. Let us see the methods with an example:
public class StringDemo { public static void main(String[] args) { String sep = " "; System.out.println(String.join(sep, "first", "second", "third")); List<String> elements = Arrays.asList(new String[]{"first", "second", "third"}); System.out.println(String.join(sep, elements)); } }
- The join method is used to specifying a new
string
which acts as the separator between the specified strings. - Running the example above produces the following output:
first second third first second third
2. Java String Methods – Java 9
The two new methods added as part of Java 9 are chars and codePoints. They are very similar to each other except that they exhibit different behavior when surrogate pairs are used. Refer to this documentation to understand in detail about the Unicode and surrogate pairs. codePoints combines the surrogate pairs available in the sequence while chars treat them as separate characters. This can be explained with an example:
String value = MAX_HIGH_SURROGATE + "" + MAX_SURROGATE; value.chars().forEach(character -> { System.out.print(character + " "); }); System.out.println(); value.codePoints().forEach(character -> { System.out.print(character + " "); });
56319 57343 1114111
Using a string like “Hello world” would have no perceivable difference between the two methods.
72 101 108 108 111 32 87 111 114 108 100 72 101 108 108 111 32 87 111 114 108 100
3. Java String Methods – Java 10
There were no new methods added in Java 10.
4. Java String Methods – Java 11
A whole set of 6 methods were added in Java 11 to extend the capability of String
class. Let us look at the methods with an example.
isBlank – This is different from isEmpty
Method. isEmpty
checks if the string
has at least one character or not. isBlank
checks for at least one non-white space character. In our case isBlank
returns true whereas isEmpty
returns false.
String value = " "; System.out.println(value.isBlank()); System.out.println(value.isEmpty());
lines – This is used to break the entire string
into a set of lines. It breaks up the string into lines if it encounters any of these line terminators – \n (or) \r (or) \r\n. The typical use case is when the entire string is read from a file, and we have to process them line by line. The implementation is much faster than doing a split
.
String fileContent = "This is line1\nThis is line2"; fileContent.lines().forEach(line -> System.out.println(line));
We have broken the string into various lines, and we iterate through the lines to print the result:
This is line1 This is line2
repeat – It is used to repeat a string the specified number of times. In the below example we repeat the hyphen 20 times which is printed to the console.
System.out.println("-".repeat(20));
--------------------
- strip – used to remove leading and trailing white spaces in a
string
- stripLeading – Removes only the leading white space characters from the
string
- stripTrailing – Removes only the trailing white space characters from the
string
String variableWithSpaces = " Space remove "; System.out.println(variableWithSpaces+"d"); System.out.println(variableWithSpaces.strip()+"d"); System.out.println(variableWithSpaces.stripLeading()+"d"); System.out.println(variableWithSpaces.stripTrailing()+"d");
Space remove d Space removed Space remove d Space removed
Character d is added to signify the end character. Here the first example is the unchanged string
. Subsequent examples shows the behavior of each strip method.
You can also check the Java 11 String Class New Methods Example for further knowledge.
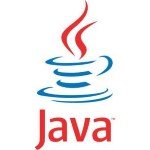
5. Java String Methods – Java 12
Four new methods were added as part of Java 12 String class. The describeConstable
and resolveConstantDesc
methods were introduced to support JEP 334 (JVM Constants API). These methods implement the Constable, and ConstantDesc interfaces, respectively. (The JVM Constants API allows loadable constants to be symbolically referenced. This change is technical in nature and is beneficial for programs that work with the constant pool table. For a short explanation on the Constants API, view the following article: Java 12’s JVM Constants API explained in 5 minutes.)
The first method we are going to look is describeConstable
. It creates an optional instance with the value of the string
specified in it.
String value = "value"; Optional optionalValue = value.describeConstable(); System.out.println(optionalValue.orElse("Empty"));
It is representation in alignment with java’s functional operators. The above program produces the following result.
value
String resolvedValue = value.resolveConstantDesc(MethodHandles.publicLookup()); System.out.println(resolvedValue);
This prints the string as it is. The introduction of this method is for the Constants API as explained above.
indent
method is used to insert the specified number of spaces before the string.
String indentedValue = value.indent(10); System.out.print(indentedValue);
The above example inserts 10 space characters before the string. Another hidden aspect of this method, it inserts a newline character at the end which can be observed in the print statement.
value
This takes the string and then allows it to be transformed to a new type or other functional transformations on top of it. This is another step towards leveraging lambda programming in java.
String out = resolvedValue.transform(x -> x.repeat(2)); System.out.println(out);
The above code runs the repeat transformation on the string to repeat the string twice.
valuevalue
You can also check the Java 12 String Methods Example for further knowledge.
6. Java String Methods – Java 13
There were 3 methods introduced in String Class to specifically handle text blocks. A text block is a multi-line string literal that avoids the need for most escape sequences, automatically formats the string predictably, and gives the developer control over format when desired. This is a preview language feature and even is marked deprecated in Java 13. For further details on text blocks refer to JEP 355.
We will see an example that combines all three methods.
String html = " \n" + " \n" + "Hello, \\t world from Java %d
\n" + " \n" + "\n"; System.out.println(html.formatted(13).stripIndent().translateEscapes());
formatted
– used to specify the appropriate value for the format string in the input. This is very similar to behaviour ofFormatter
class.stripIndent
– Removes the unnecessary whitespace in the input. In the above example, it removes the extra whitespace character in first line afterhtml
tag.translateEscapes
– it Strips the escape character such as \\ and applies the actual formatting. In the example above, it applies \t to the string.
<html> <body> <p>Hello, world from Java 13</p> </body> </html>
7. Download the Source code
You can download the full source code of this example here: Java String Methods from Java 8 to Java 14