Java print to console Example
In this post, we feature a comprehensive article about the Java print to console Example. We will have a look at the System.console, the methods provided and its differences.
1. Java Console class
The java.io.Console class provides methods to access the character-based console device. If the virtual machine has a console then it is represented by a unique instance of this class which can be obtained by invoking the System.console() method. If no console device is available then an invocation of that method will return null.
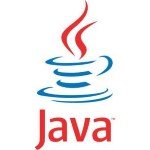
The Java console class is used to get inputs from the console. It provides methods to read texts and passwords. Passwords read using the Console class will not be visible to the naked eye.
You can also check our Java Console Application Tutorial in the following video:
2. Methods of Console class
The following are some of the methods of the Console class. Each method will be explained with an example program.
2.1 writer() method
Retrieves the PrintWriter object associated with this console.
ConsoleWriterExample.java
package com.javacodegeeks.basics; import java.io.Console; import java.io.PrintWriter; public class ConsoleWriterExample { public static void main(String[] args) { Console console = System.console(); if(console != null) { PrintWriter pw = console.writer(); pw.println("Console class writer() method example"); }else { System.out.println("Console is null"); } } }
Output in eclipse:
Console is null
Output in command prompt:
Console class writer() method example
There is no console when executing in eclipse possibly because most IDEs are using javaw.exe instead of java.exe to run Java code. One of the ways of bringing console in eclipse is starting the application in remote debugging mode.
2.2 reader() method
Retrieves the unique Reader object associated with the console.
ConsoleReaderExample.java
package com.javacodegeeks.basics; import java.io.Console; import java.util.Scanner; public class ConsoleReaderExample { public static void main(String[] args) { Console console = System.console(); if(console != null) { Scanner sc = new Scanner(console.reader()); System.out.println("Enter your name - "); String input = sc.nextLine(); System.out.println("Hello "+input); }else { System.out.println("Console is null"); } } }
Output in command prompt:
Enter your name - John Doe Hello John Doe
The bulk read operations read(char[..].) and read(java.nio.CharBuffer) on the returned object will not read in characters beyond the line bound for each invocation, even if the destination buffer has space for more characters. A line bound is considered to be any one of a line feed (‘\n’), a carriage return (‘\r’), a carriage return followed immediately by a linefeed, or an end of the stream.
2.3 readLine() method
For simple applications requiring only line-oriented reading, use readLine() or readLine(String, Object….).
ConsoleReadLineExample.java
package com.javacodegeeks.basics; import java.io.Console; public class ConsoleReadLineExample { public static void main(String[] args) { Console console = System.console(); if(console != null) { String input = console.readLine("Enter Input: "); System.out.println("Input from console - "+input); }else { System.out.println("Console is null"); } } }
Output in command prompt:
Enter Input: I am John Doe Input from console - I am John Doe
This method returns the string containing the line read from the console, not including any line termination character, or null if an end of the stream has been reached.
2.4 readPassword() method
Reads a password or passphrase from the console which is not visible to the user.
ConsoleReadPasswordExample.java
package com.javacodegeeks.basics; import java.io.Console; public class ConsoleReadPasswordExample { public static void main(String[] args) { Console console = System.console(); if(console != null) { char[] input = console.readPassword("Enter Password: "); System.out.println("The password is - "+new String(input)); }else { System.out.println("Console is null"); } } }
Output in command prompt:
Enter Password: The password is - Java123
Returns a character array containing the password or passphrase read from the console, not including any line-termination characters, or null if an end of the stream has been reached.
2.5 format() and printf() methods
Writes a formatted string to the console’s output stream using the specified format string and arguments.
ConsoleFormatExample.java
package com.javacodegeeks.basics; import java.io.Console; public class ConsoleFormatExample { public static void main(String[] args) { Console console = System.console(); if(console != null) { String name="John Doe"; String age = "29"; console.format("My name is %s and I am %s years old %n",name,age ); console.printf("My name is " +name+" and I am "+age+ " years old"); }else { System.out.println("Console is null"); } } }
Output in command prompt:
My name is John Doe and I am 29 years old My name is John Doe and I am 29 years old
The program throws an IllegalFormatException when the format string has an incorrect syntax or incompatible format with the argument or insufficient arguments, etc.
The printf() method is used here to write a formatted string to the console’s output stream using the specified format string and arguments.
2.6 flush() method
Flushes the console and forces any buffered output to be written immediately.
ConsoleFlushExample.java
package com.javacodegeeks.basics; import java.io.Console; public class ConsoleFlushExample { public static void main(String[] args) { Console console = System.console(); if(console != null) { String input = console.readLine("Enter Input: "); System.out.println("The entered input is - "+input); console.flush(); }else { System.out.println("Console is null"); } } }
Output in command prompt:
Enter Input: I am a programmer The entered input is - I am a programmer
The above output is written to the console immediately.
3. System.in, System.out, System.err
The 3 streams System.in, System.out, System.err are also common sources or destinations of data. Most commonly used is probably the System.out for writing output to the console from console programs.
These 3 streams are initialized by the Java runtime when a JVM starts up, so you don’t have to instantiate any streams yourself (although you can exchange them at runtime).
3.1 System.in
The “standard” input stream. This stream is already open and ready to supply input data. Typically this stream corresponds to keyboard input or another input source specified by the host environment or user. A typical way of using System.in is:
FileInputStream fis = new FileInputStream("inputfile.txt"); // set input stream System.setIn(fis); char c = (char) System.in.read();
3.2 System.out
The “standard” output stream. This stream is already open and ready to accept output data. Typically this stream corresponds to display output or another output destination specified by the host environment or user.
For Java applications, a typical way to write a line of output data is:
System.out.println(data)
3.3 System.err
The “standard” error output stream. This stream is already open and ready to accept output data.
Typically this stream corresponds to display output or another output destination specified by the host environment or user. By convention, this output stream is used to display error messages or other information that should come to the immediate attention of a user even if the principal output stream, the value of the variable out, has been redirected to a file or other destination that is typically not continuously monitored.
System.err.println()
4. Download the Java print to console Example
This was an example of Java print to console.
You can download the full source code of this example here: Java print to console Example