Java Print Example
1. Introduction
Java print, Java println and Java System.out.println method is a convenient method found both within the java.io.PrintStream and java.io.PrintWriter class and is used to display a text on the console. The text is passed as a parameter to this method in the form of a String. After printing the text on the console, it places the cursor at the end of the text. The most common function call is “System.out.println”. The next printing takes place from there. It has various overloaded forms as shown below:
public void print(boolean b)
public void print(char c)
public void print(int i)
public void print(long l)
public void print(float f)
public void print(double d)
public void print(char[] s)
public void print(String s)
public void print(Object obj)
Note: print() and java system.out.println() method under the PrintStream class is used to print bytes to the output stream whereas the print() method within the PrintWriter class is used to display the characters to the output stream. In this tutorial, we will refer to the print() method belonging to the PrintWriter class for all our examples and descriptions.
2. print Method in Java
Table displaying the details of the various overloaded forms of the print() method.
Method Syntax | Description | Parameters |
public void print(boolean b) | Prints a boolean value. The string produced by is translated into bytes according to the platform’s default character encoding, and these bytes are written in exactly the manner of the method. | b – The boolean to be printed |
public void print(char c) | Prints a character. The character is translated into one or more bytes according to the platform’s default character encoding, and these bytes are written in exactly the manner of the method. | c – The char to be printed |
public void print(int i) | Prints an integer. The string produced by is translated into bytes according to the platform’s default character encoding, and these bytes are written in exactly the manner of the method. | i – The int to be printed |
public void print(long l) | Prints a long integer. The string produced by is translated into bytes according to the platform’s default character encoding, and these bytes are written in exactly the manner of the method. | l – The long to be printed |
public void print(float f) | Prints a floating-point number. The string produced by is translated into bytes according to the platform’s default character encoding, and these bytes are written in exactly the manner of the method. | f – The float to be printed |
public void print(double d) | Prints a double-precision floating-point number. The string produced by is translated into bytes according to the platform’s default character encoding, and these bytes are written in exactly the manner of the method. | d – The double to be printed |
public void print(char[] s) | Prints an array of characters. The characters are converted into bytes according to the platform’s default character encoding, and these bytes are written in exactly the manner of the method. | s – The array of chars to be printed |
public void print(String s) | Prints a string. If the argument is null then the string "null" is printed. Otherwise, the string’s characters are converted into bytes according to the platform’s default character encoding, and these bytes are written in exactly the manner of the method. | s – The String to be printed |
public void print(Object obj) | Prints an object. The string produced by the method is translated into bytes according to the platform’s default character encoding, and these bytes are written in exactly the manner of the method. | obj – The Object to be printed |
2.1 Java print Example
The following example shows the usage of different forms of the print() method.
PrintDemo.java
public class PrintDemo { public static void main(String[] args) { boolean b=true; char c='i'; int i=30; long l=20000L; float f = 23.45f; double d = 200.34; char[] name= {'S','A','M'}; String str="Carl Jackson"; Object obj = new Object(); //print a boolean value System.out.print(b); //print a character value System.out.print(c); //print an integer value System.out.print(i); //print an long value System.out.print(l); //print a float value System.out.print(f); //print a double value System.out.print(d); //print a character array System.out.print(name); //print a String System.out.print(str); //print an object System.out.print(obj); } }
After running the above code in any IDE of your choice you’ll receive the following output:
Output
truei302000023.45200.34SAMCarl Jacksonjava.lang.Object@2ff4acd0
As we can see in the above output for the given code, the output for each print statement is printed just after the previous output and there is no blank space between the two outputs for two consecutive print statements.
3. Introduction to println method and difference with the print method in Java
The java system.out.println() method is another convenient method within the PrintWriter class which is used for displaying text on the console. The only difference between print() and java System.out.println() method is that the latter positions the cursor onto the next line after printing the desired text while the former method leaves the cursor on the same line. The different overloaded forms of java System.out.println() method are as follows:
public void println()
public void println(boolean x)
public void println(char x)
public void println(int x)
public void println(long x)
public void println(float x)
public void println(double x)
public void println(char[] x)
public void println(String x)
public void println(Object x)
3.1 System.out.println() Method in Java
Table displaying the details of the various overloaded forms of System.out,println() method in Java.
Syntax | Description | Parameters |
public void println() | Terminates the current line by writing the line separator string. The line separator string is defined by the system property line. separator, and is not necessarily a single newline character (‘\n’). | |
public void println(boolean x) | Prints a boolean and then terminate the line. This method behaves as though it invokes print(boolean) and then println(). | x – The boolean to be printed |
public void println(char x) | Prints a character and then terminate the line. This method behaves as though it invokes and then . | x – The char to be printed. |
public void println(int x) | Prints an integer and then terminate the line. This method behaves as though it invokes and then . | x – The int to be printed. |
public void println(long x) | Prints a long and then terminate the line. This method behaves as though it invokes and then . | x – a The long to be printed. |
public void println(float x) | Prints a float and then terminate the line. This method behaves as though it invokes and then . | x – The float to be printed. |
public void println(double x) | Prints a double and then terminate the line. This method behaves as though it invokes and then . | x – The double to be printed. |
public void println(char[] x) | Print an array of characters and then terminate the line. This method behaves as though it invokes print(char[]) and then println(). | x – an array of chars to print. |
public void println(String x) | Prints a String and then terminate the line. This method behaves as though it invokes and then . | x – The String to be printed. |
public void println(Object x) | Prints an Object and then terminate the line. This method calls at first String.valueOf(x) to get the printed object’s string value, then behaves as though it invokes and then . | x – The Object to be printed. |
3.2 Java System.out.println Example
The following example shows the usage of different forms of the java System.out.println() method.
PrintlnDemo.java
class PrintlnDemo { public static void main(String[] args) { boolean b=false; char ch='C'; int i=20; long l= 2000L; float f=20.34f; double d=20.23; char[] name= {'S','A','M'}; String str="Carl Jackson"; Object obj = new Object(); // using simple println() method System.out.println(); // printing boolean value System.out.println(b); // printing char value System.out.println(ch); // printing int value System.out.println(i); // printing long value System.out.println(l); // printing float value System.out.println(f); // printing double value System.out.println(d); // printing char array value System.out.println(name); // printing String value System.out.println(str); // printing Object value System.out.println(obj); } }
After running the above code in any IDE of your choice you’ll receive the following output:
Output
false C 20 2000 20.34 20.23 SAM Carl Jackson java.lang.Object@2ff4acd0
In the above output, we can see that output generated from each of the println() method ends with a new line i.e. the output for the next statement is printed in a new line.
4. Introduction to Printf() method
The printf() method is a convenience method under the PrintWriter class to write a formatted string to the writer object using the specified format string and arguments. If automatic flushing is enabled, calls to this method will flush the output buffer. printf() provides string formatting similar to the printf function in C. The different overloaded forms of printf() method are as follows:
- public PrintWriter printf(String format, Object… args)
- public PrintWriter printf(Locale l, String format,Object… args)
4.1 printf() method in Java
Table displaying the details of the various overloaded forms of printf() method.
Syntax | Description | Parameters | Returns | Throws |
public PrintWriter printf(String format, Object… args) | A convenience method to writing a formatted string to this writer using the specified format string and arguments. If automatic flushing is enabled, calls to this method will flush the output buffer. An invocation of this method of the form out.printf(format, args) behaves in the same way as the invocation out.format(format, args) | format – A format string as described in Format string syntax. args – Arguments referenced by the format specifiers in the format string. If there are more arguments than format specifiers, the extra arguments are ignored. The number of arguments is variable and may be zero. The maximum number of arguments is limited by the maximum dimension of a Java array as defined by The Java™ Virtual Machine Specification . The behavior on a null argument depends on the conversion. | This writer | IllegalFormatException – If a format string contains an illegal syntax, a format specifier that is incompatible with the given arguments, insufficient arguments are given the format string or other illegal conditions. For the specification of all possible formatting errors, see the Details section of the formatter class specification. NullPointerException – If the format is null |
public PrintWriter printf(Locale l, String format, Object… args) | A convenience method to writing a formatted string to this writer using the specified format string and arguments. If automatic flushing is enabled, calls to this method will flush the output buffer. An invocation of this method of the form out.printf(l, format, args) behaves in the same way as the invocation out.format(l, format, args) | l – The locale to apply during formatting. If l is null then no localization is applied. format – A format string as described in Format string syntax. args – Arguments referenced by the format specifiers in the format string. If there are more arguments than format specifiers, the extra arguments are ignored. The number of arguments is variable and may be zero. The maximum number of arguments is limited by the maximum dimension of a Java array as defined by The Java™ Virtual Machine Specification . The behavior on a null argument depends on the conversion. | This writer | IllegalFormatException – If a format string contains an illegal syntax, a format specifier that is incompatible with the given arguments, insufficient arguments are given the format string or other illegal conditions. For the specification of all possible formatting errors, see the Details section of the formatter class specification. NullPointerException – If the format is null |
4.2 Java printf Example
The following example shows the usage of different forms of the printf() method.
PrintfDemo.java
import java.util.Date; import java.util.Locale; public class PrintfDemo { public static void main(String[] args) { // Number Formatting int x = 10; System.out.printf("Formatted output is: %d %d%n", x, -x); // Precision formatting float y = 2.28f; System.out.printf("Precision formatting upto 4 decimal places %.4f\n",y); float z = 3.147293165f; System.out.printf("Precision formatting upto 2 decimal places %.2f\n",z); // Filling with Zeroes System.out.printf("'%05.2f'%n", 2.28); System.out.printf("'%010.2f'%n", 2.28); System.out.printf("'%010.2f'%n", -2.28); System.out.printf("'%010.2f'%n", 1234567.89); System.out.printf("'%010.2f'%n", -1234567.89); // Right and Left alignment System.out.printf("'%10.2f'%n", 2.28); System.out.printf("'%-10.2f'%n", 2.28); // Using comma and locale System.out.printf(Locale.US, "%,d %n", 5000); // String formatting System.out.printf("%s %s!%n","Hello","World"); System.out.printf("%s\f%s!%n","Hello","World!"); System.out.printf("%s\\%s!%n","Hello","World!"); // Uppercase System.out.printf("%s %S!%n","Hello","World"); // Boolean Formatting System.out.printf("%b%n", false); System.out.printf("%b%n", 0.5); System.out.printf("%b%n", "false"); // Time Formatting Date date = new Date(); System.out.printf("%tT%n", date); System.out.printf("H : %tH, M: %tM, S: %tS%n",date,date,date); System.out.printf("%1$tH:%1$tM:%1$tS %1$Tp GMT %1$tz %n", date); // Date Formatting System.out.printf("%s %tB %<te, %<tY", "Current date: ", date); System.out.printf("%1$td.%1$tm.%1$ty %n", date); System.out.printf("%s %tb %<te, %<ty", "Current date: ", date); } }
Output
Formatted output is: 10 -10 Precision formatting upto 4 decimal places 2.2800 Precision formatting upto 2 decimal places 3.15 '02.28' '0000002.28' '-000002.28' '1234567.89' '-1234567.89' ' 2.28' '2.28 ' 5,000 Hello World! HelloWorld!! Hello\World!! Hello WORLD! false true true 16:21:20 H : 16, M: 21, S: 20 16:21:20 PM GMT +0530 Current date: March 17, 202017.03.20 Current date: Mar 17, 20
5. Conclusion
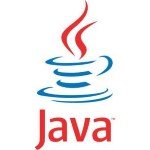
In this article, we saw the usage of the following Java methods print, println and printf from the PrintStream class. We also saw their differences with each other and how they can be used in different situations for printing different types of outputs to the console.
6. References
- https://docs.oracle.com/javase/7/docs/api/java/io/PrintWriter.html#PrintWriter(java.io.OutputStream,%20boolean)
- https://www.journaldev.com/28692/java-printf-method
7. Download the source code
That was a Java print Example.
You can download the full source code of this example here: Java Print Example