Java Print Array Example
In this post, we feature a comprehensive article about how to print a Java array.
1. Introduction
Each variable holds values, but the array variable holds multiple values of the same type. In other words, the group of similar types of data grouped under one variable is called Array.
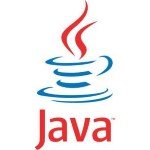
- You can access array values using its index.
- An Array is ordered means it keeps its element sorted.
- It can contain primitive data types as well as objects of the class.
You can watch the following video and learn how to use arrays in Java:
Now we will see what are all the options to print the values of arrays. Based on your needs, you can select any option. Come let’s dive into different options and examples.
2. Java Print Array Examples
2.1 Using For loop
The general form of for
loop works in all java versions. Enhanced for
loop introduced in java 5. If you need read-only array without modifying the values, then you can choose enhanced for
loop.
Code Example1
private void printUsingForLoop() { int[] intArray = {1, 2, 3, 4, 5}; for (int i = 0; i < intArray.length; i++) { System.out.print(intArray[i] + " "); } } private void printUsingEnhancedForLoop() { int[] intArray = {1, 2, 3, 4, 5}; for (int i : intArray) { System.out.print(i + " "); } }
Output
1 2 3 4 5
In the above code, for
loop internally maintains counter and iterate using index till the last element of the array. Enhanced for
traverse each element one by one and also the code is more readable. intArray[i]
gives the value of the array on respective index. for (int i : intArray)
iterates each value of the array. Both options will give the same output.
2.2 Using Arrays.toString
This method returns the String representation of the values of an array.
Code Example2
private void printStringArray() { String[] array = new String[] {"Java", "Code", "Geek"}; System.out.println(Arrays.toString(array)); } private void printIntArray() { int[] intArray = { 1, 2, 3, 4, 5 }; System.out.println(Arrays.toString(intArray)); }
Output
[Java, Code, Geek] [1, 2, 3, 4, 5]
In the above code, Arrays.toString
used. Whatever array you pass to this method, it will read all the values of the array without any additional loop. Arrays.toString(intArray)
gives the value of the int array and Arrays.toString(array)
gives the values of String array. This option is recommended but it is available only from Java 5.
2.3 Using String.join
This method concatenates all the values of an array using delimiter.
Code Example3
private void printArrayUsingJoin() { String[] strArray = {"Java", "Code", "Geek"}; String delimiter = " "; System.out.println(String.join(delimiter, strArray)); }
Output
Java Code Geek
In the above code, String.join(delimiter, strArray)
method accepts delimiter and the array, for which you want to print the values. Basically it is reading the values from the array and applying delimiter and concatenating it.
2.4 Using Arrays.deepToString
This method returns the string representation of all values of a multidimensional array.
Code Example4
private static void printTwoDimentionArray() { String[][] multiArray = new String[][] {{"Java", "Code", "Geek"}, {"Hello", "World", "Program"}}; System.out.println(Arrays.deepToString(multiArray)); System.out.println(Arrays.toString(multiArray)); }
Output
[[Java, Code, Geek], [Hello, World, Program]] [[Ljava.lang.String;@15db9742, [Ljava.lang.String;@6d06d69c]
In the above code, Arrays.deepToString(multiArray)
used. It will print the values of the multidimensional but Arrays.toString()
will just print the object name as shown in the output. This option is recommended but it is available only from Java 5.
2.5 Using Stream
This method is available from Java 8.
Arrays.Stream
returns a sequential Stream with the specified array as its source.- Stream.of() method invokes the
Arrays.stream()
method for non-primitive data types. It is like a wrapper for theArrays.stream()
method. This method accepts primitive as well as non-primitive data types - All the below code will give the same output
Code Example5
private static void printUsingStreamWithForeach() { String[] strArray = new String[]{"Welcome", "to", "Java", "Code", "Geek"}; Arrays.stream(strArray).forEach(System.out::println); } private static void printUsingAsListStreamWithForeach() { String[] strArray = new String[]{"Welcome", "to", "Java", "Code", "Geek"}; Arrays.asList(strArray).stream().forEach(s -> System.out.println(s)); } private static void printUsingStreamOf() { String[] strArray = new String[]{"Welcome", "to", "Java", "Code", "Geek"}; Stream.of(strArray).forEach(System.out::println); }
Output
Welcome to Java Code Geek
In the above code, Arrays.stream(strArray)
and forEach(System.out::println)
are used. forEach
performs against each element of the Stream. Stream.of(strArray).forEach(System.out::println)
also gives the same output.
2.6 Using Stream and flatMap
This method uses the Map
method and flattens the result. For Multidimensional array, you can make use of flatMap
Code Example6
private void printUsingFlatMapForStringMultiArray() { String[][] multiArray = new String[][] { { "Java", "language" }, { "Hello", "World" } }; Arrays.stream(multiArray).flatMap(x -> Arrays.stream(x)).forEach(System.out::println); } private void printUsingFlatMapForIntMultiArray() { int[][] multiArray = new int[][] { { 10, 20 }, { 30, 40 } }; Arrays.stream(multiArray).flatMapToInt(x -> Arrays.stream(x)).forEach(System.out::println); }
Output
Java language Hello World 10 20 30 40
In the above code,Arrays.stream(multiArray).flatMap
accepts the multidimensional array and the flatMap method used along with the lambda expression to print each element.flatMapToInt
method is used to accept the integer array.
2.7 Using Arrays.asList
Arrays have the feature to convert the String array to the list.
Code Example7
private static void printArrayUsingasList() { String[] strArray = { "Java", "Code", "Geek"}; List listArray = Arrays.asList(strArray ); System.out.println(listArray); }
Output
[Java, Code, Geek]
In the above code, Arrays.asList(strArray)
converted the String array into List
and used println
to print the values of the array. It is one of the easiest way but unwantedly conversion occurs so it is not much recommended
2.8 Using GSON library
GSON is the open-source java library used to serialize/deserialize the java objects from/to JSON. Prerequisite is gson-2.8.2.jar
Code Example8
private void printUsingGson() { String[] strArray = { "Java", "Code", "Geek"}; Gson gson = new Gson(); System.out.println(gson.toJson(strArray)); }
Output
["Java","Code","Geek"]
In the above code, I used the Gson method gson.toJson(strArray)
to get the array values. If your project is already using this library then it is wise to use this option to print the arrays..
2.9 Using Jackson library
Jackson is the open-source java library used to process the JSON.
Prerequisite is
- jackson-annotations-2.10.0.pr3.jar
- jackson-core-2.10.0.pr3.jar
- jackson-databind-2.10.0.pr3.jar
Code Example9
private void printUsingJackson() throws JsonProcessingException { String[] strArray = { "Java", "Code", "Geek"}; ObjectWriter objwriter = new ObjectMapper().writer().withDefaultPrettyPrinter(); System.out.println(objwriter.writeValueAsString(strArray)); }
Output
[ "Java", "Code", "Geek" ]
In the above code, ObjectWriter objwriter = new ObjectMapper().writer().withDefaultPrettyPrinter();
methods used from the Jackson library. objwriter.writeValueAsString(strArray)
gives the value in string representation. In a same way, you can use any type of data.
2.10 Using StringBuilder
StringBuilder will append the string and insert it to accept data of any type. It is also one of the options to print the array with the help of for
loop.
Code Example10
private static void printArrayUsingStringBuilder() { boolean flag = true; StringBuilder strBuilder = new StringBuilder(); String[] strArray = new String[] { "Java", "Code", "Geek" }; for (String eachString : strArray) { if (!flag) strBuilder.append(' '); strBuilder.append(eachString); flag = false; } System.out.println(strBuilder.toString()); }
Output
Java Code Geek
In the above code, StringBuilder
used to build the String
along with for
loop. strBuilder.toString()
method returns all the appended values of the array.
3. Conclusion
This article listed out all the possible options to print the java array. According to me, the best option is Arrays.toString()
for single dimension array and Arrays.deepToString()
for multi-dimensional array. Hope this article helps you !!!
4. Download the Source Code
This was an example of printing the Java array in 10 different options.
You can download the full source code of this example here: Java Print Array Example