Java Operator Precedence Example
In this article, we will cover the details of Java operator precedence table relative to each other.
1. What are operators in Java?
Operator in java is a symbol that is used to perform operations. For example: +, -, *, / etc.
There are many types of operators in Java listed below,
- Unary Operator,
- Arithmetic Operator,
- Shift Operator,
- Relational Operator,
- Bitwise Operator,
- Logical Operator,
- Ternary Operator and
- Assignment Operator
2. What is operator precedence in Java?
Operator precedence determines the order in which the operators in an expression are evaluated.
Java operator precedence comes into play while evaluating an expression in java which contains multiple operators belonging to multiple types as shown above. In order for always reaching to the same solution for the expression with multiple operators, rules of operator precedence are established to avoid ambiguity.
3. Java Operator Precedence Table
In this section, we will present a tabular structure representing the relative precedence order of various java operator in Fig 1 below.
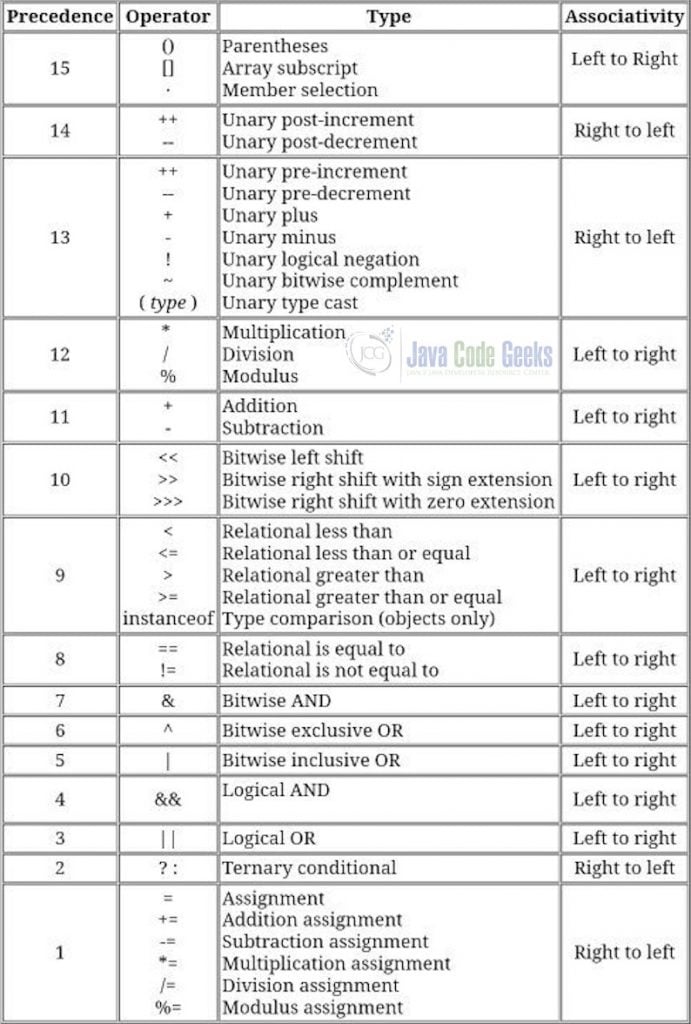
4. Examples of Java Operator Precedence
In this section we will discuss some code examples which present the Operator precedence in action.
First example we will discuss will contain pre/post unary operator.
Precedence.java
class Precedence {
public static void main(String[] args) {
System.out.println("Evaluating Operator Precedence with pre and post addition unary operator");
int a = 10, b = 5, c = 1, result;
result = a - ++c - ++b;
System.out.print("Result is ");
System.out.println(result);
}
}
So in the above example of Precedence.java
we have used the post-addition and pre-addition unary operator to evaluate an expression. First b will be incremented to 6 from 5, then c will be incremented to 2 from 1 and then a is set to 10 in the order of associativity. Afterwards, 2 will subtracted from 10 and 2 will be subtracted from the result of (10-2) making final result to 2, as shown in the snapshot in Fig.2 below,

Next example we will discuss will be of Leap Year calculation.
LeapYear.java
public class LeapYear {
public static void main(String[] args) {
int year = 2019;
System.out.println("Evaluating Leap year");
printLeapYear(year);
}
public static void printLeapYear(int year) {
boolean a = (((year % 4) == 0) && ((year % 100) != 0)) || ((year % 400) == 0); // most clear due to Parenthesis
boolean b = (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0); // less clear expression
boolean c = (year % 4 == 0 && year % 100 != 0) || year % 400 == 0; // less clear expression
boolean d = ((year % 4 == 0) && (year % 100 != 0)) || (year % 400 == 0); // less clear expression
boolean e = year % 4 == 0 && year % 100 != 0 || year % 400 == 0; // least clear expression
System.out.println(a);
System.out.println(b);
System.out.println(c);
System.out.println(d);
System.out.println(e);
}
}
In the above code snippet, we have tried to highlight the readability of the same java expression using the parenthesis. With parenthesis, we can make out the smaller expressions within the larger expressions clearly, which in turn improves the readability of the code. In this example we have used the binary(%) and logical operators (!,&&, ||). Output of the code snippet is shown in the Fig. 3 below,
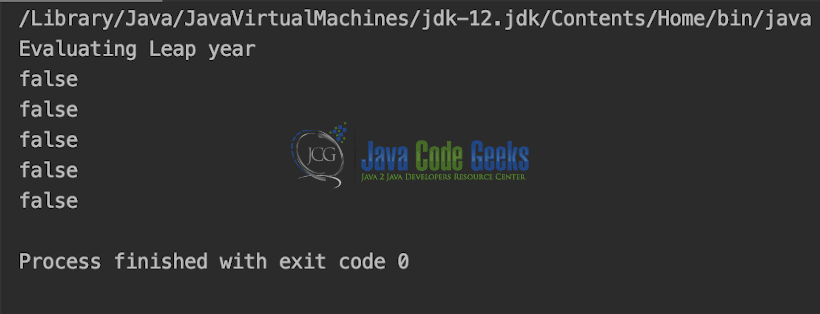
In this example we will discuss the Temperature Conversion.
TemperatureConversion.java
public class TemperatureConversion
{
public static void main(String arg[])
{
System.out.println("Evaluating the Temperature Conversion");
double fahrenheit = 98.4;
double celsius = ( 5.0 * (fahrenheit - 32.0) ) / 9.0; // clear expression because of the Parenthesis
System.out.println(fahrenheit + " F is same as " + celsius + " C.");
}
}
In this example, we have again emphasised the use of parenthesis to increase the readability of the expression. In this example, we have used multiple binary operators including multiplication, division and subtraction. First we will solve the expression within the parenthesis, followed by the outer expression from left to right to maintain the rules of associativity.
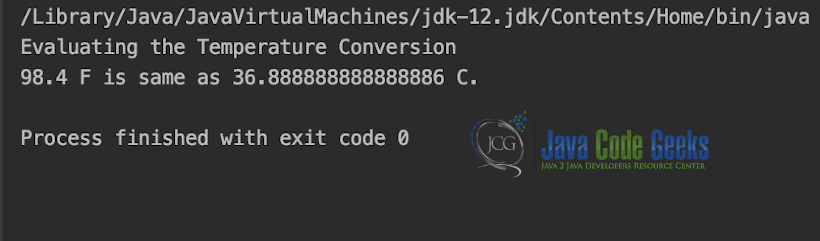
5. Summary
To Summarise, we have covered the basics of operator precedence, including their relation of associativity with each other via some code examples. Some more example and details regarding the java operators precedence and associativity can be find here.
6. Download the source code
You can download the full source code of this example here: Java Operator Precedence Example