Java Long Class Example
In this article, we will see examples of the Long class in the java programming language.
1. Introduction
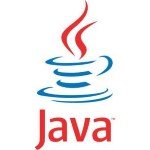
The Long class is simply a wrapper class for the primitive type long. It wraps the long primitive value to an object. An object of type Long contains a single field whose type is long. So what would be the benefit if wraps around the primitive data type to an object? Well, basically we would be able to access all the methods that are readily available in the Long class which is very useful.
In addition, this class provides several methods for converting a long to a String and a String to a long, as well as other constants and methods useful when dealing with a long.
2. Long Class Declaration
public final class Long extends Number implements Comparable<Long>
3. Long Class Fields
Following are the fields for java.lang.Long class:
- static long MAX_VALUE − This is a constant holding the maximum value a long can have, 2^63-1.
- static long MIN_VALUE − This is a constant holding the minimum value a long can have, -2^63.
- static int SIZE − This is the number of bits used to represent a long value in two’s complement binary form.
- static Class<Long> TYPE − This is the class instance representing the primitive type long.
4. Long Class Constructors
There two constructor available to Long class which we can use to instantiate a Character object:
Constructor | Description |
Long(long value) | Constructs a newly allocated Long object that represents the specified long argument. |
Long(String s) | Constructs a newly allocated Long object that represents the long value indicated by the String parameter. |
Creating the Long wrapper class object.
class A { public static void main(String... ar) { Long b1 = new Long(10); //Boxing primitive long to a Long object. Long b2 = new Long("20"); //Passing primitive long as a String } }
Reading the Long wrapper class object.
There are two ways to read a Long object:
- By directly printing the Long object.
- Calling method, longValue() of Long wrapper class, which returns the primitive long equivalent.
public class A { public static void main(String... ar) { Long b1 = new Long(10); //Boxing primitive long in a wrapper Long object. Long b2 = new Long("20"); //Passing primitive long as a String to be boxed in a Long object. //Unboxing a wrapper Long object. System.out.println(b1); //Unboxing an Integer object using longValue() method System.out.println(b2.longValue()); } }
5. Long Class Methods in Java
Some important methods of Long wrapper class:
Methods | Description |
int compareTo(Long b) | – Returns a zero if the invoking Long object contains value same as b. – Returns a positive value if invoking Long object’s value is > b. – Returns a negative value if invoking Long object’s value is < b. |
boolean equals (Object ob) | Returns a true if invoking Long object has same value as referred by ob, else false. |
static long parseLong(String s) | Returns a primitive long value if String, s could be converted to a valid long value. |
static Long valueOf(long b) | Returns a Long object after converting it from primitive long value, b. |
static Long valueOf(String s) | Returns a Long object after converting it from a String, s. |
short shortValue() | Returns an primitive short value after converting it from an invoked Long object. |
Long byteValue() | Returns a primitive byte value after converting it from an invoked Long object. |
int intValue() | Returns a primitive int value after converting it from an invoked Long object. |
long longValue() | Returns a primitive long value after converting it from an invoked Long object. |
float floatValue() | Returns a primitive float value after converting it from an invoked Long object. |
double doubleValue() | Returns an primitive double value after converting it from an invoked Long object. |
Using compareTo() method to compare values in two Long objects.
Method compareTo(Long l) takes Long class object and it:
- Returns a zero if the invoked Long object contains the value same as l.
- Returns 1 if the invoked Long object contains value larger than l.
- Returns -1 if the invoked Long object contains value smaller than l.
public class A { public static void main(String... ar) { Long l1 = new Long("10"); //Constructor accepting String value Long l2 = new Long(10); //Constructor accepting primitive int value System.out.println("Value in l1 = "+ l1); System.out.println("Value in l2 = "+ l2); System.out.println("Invoking l1 to compare with l2 : "+ l1.compareTo(l2)); Long l3 = new Long("15"); Long l4 = new Long(20); System.out.println("Value in l3 = "+l3); System.out.println("Value in l4 = "+l4); System.out.println("Invoking l3 to compare with l4 : "+ l3.compareTo(l4)); System.out.println("Invoking l4 to compare with l3 : "+ l4.compareTo(l3)); } }
6. Summary
As mentioned earlier, the Long class wraps a value of the primitive type long in an object. An object of type Long contains a single field whose type is long. In addition, this class provides several methods for converting a long to a String and a String to a long, as well as other constants and methods useful when dealing with a long.
This article helps to enhance your knowledge about the Java Long Class along with examples of constructors and methods.
7. Download the Source Code
You can download the full source code of this example here: Java Long Class Example