Java List add() and addAll() Methods
1. Introduction
In every programming language, data structures are very important and it needs to pay more attention to what type of structure you use depending on your use case. There are multiple type of data structure like:
- Array. Arrays stores items at the adjoining memory location. The items from an array can be easily accessed by an index. Length of an array can be fixed or flexible length.
- Stack. A stack stores items in a linear order. The type of operations to process a Stack is: Last In First Out(LIFO).
- Queue. This stores items like a stack. The operation order will be First In First Out (FIFO).
- Linked list. This list stores items in a linear order. Each element of a list contains a data item as well as a reference or link to the next item in the list.
- Tree. This type of structure is a bit different then the previous ones. A tree store collection or items in an abstract hierarchical way. Each node has a key value with the parent node linked to child nodes. The structure of a tree is composed by a root node and the ancestor of all nodes in the tree.
- And other types.
For more information about data structure you can find here.
2. What is a List in Java
Lists in Java are collections of items to maintain an ordered collection. The List is an interface that is present in the java.util
package and inherits the Collection interface. The implementation of List
interface is present in the following classes: ArrayList, LinkedList, Stack and many more. The most important lists in the Java programming language are ArrayList
and LinkedList
.
Declaration of the interface:
public interface List<E> extends Collection<E>
This interface exposes multiple methods like: add(), addAll(), clear(), get(), equals(), hashcode()
and many more. These methods have their own implementation in the class which implements List.
Two of the important methods are add()
and addAll()
which will be presented in the next sections with some examples.
3. add() method
One of the important methods in the List interface is add()
, which will add a new item at the end of the list. There are two add methods in the List Interface which will add an element at the end of the list. In contrast with the original method, add followed by an index will insert that value at the given index. The index will be the place in the list where that new element will be stored. All the existing items will be moved/shifted to the right. You can append values only with the same type as the declaration of the List.
3.1. Different Usages
You can declare different types of list: String, Integer and Objects and many more. The method add
can be used when you want to add just a single item into the list.
4.1. Example
As we mentioned before, List is an interface. To create a list you need to instantiate a class that implements the List interface. For the purpose of this example we will use the ArrayList implementation class.
public static void main(String[] args) {
final List<String> test = new ArrayList<>();
test.add("new Item" );
test.add("second item");
test.add("third item");
test.add("forth item");
test.add(2, "additional item");
final List<Integer> listOfIntegers = new ArrayList<>();
listOfIntegers.add(1);
listOfIntegers.add(2);
listOfIntegers.add(3);
listOfIntegers.add(4);
listOfIntegers.add(1,7);
final List<UserDTO> user = new ArrayList<>();
user.add(new UserDTO("test", "test@test.com", "NY", "+4"));
user.add(new UserDTO("jhon", "jhon@test.com", "NY", "+4"));
}
public class UserDTO {
private String username;
private String email;
private String place;
private String phone;
// Getters and Setters
public UserDTO(String username, String email, String place, String phone) {
this.username = username;
this.email = email;
this.place = place;
this.phone = phone;
}
@Override
public String toString() {
return "UserDTO{" + "username='" + username + '\'' + ", email='" + email + '\'' + ", place='" + place + '\'' + ", phone='" + phone + '\''
+ '}';
}
}
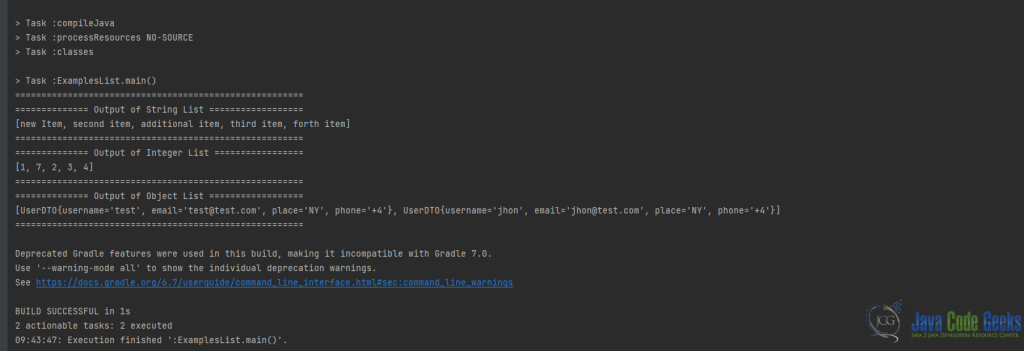
5. addAll() method
This will allow you to add/append a list of items over another list at the end of it. Similarly, with the simple add
method, another method was overridden from the original addAll method. You will have the possibility to give an index where the existing collections will add/append to the existing list. All the existing items will be moved/shifted to the right. Same approach as add()
method that you will be able to append items of the same type.
5.1. Different Usages
The usage of this method is to allow you to combine items from two lists. You can use this approach when you want to use the current one instead of returning a new one.
5.2. Example
For this example, we will use another implementation which is LinkedList.
public static void main(String[] args) {
final List<String> test1 = new LinkedList<>();
test1.add("new Item" );
test1.add("second item");
test1.add("third item");
test1.add("forth item");
test1.add(2, "additional item");
final List<String> test2 = new LinkedList<>();
test2.add("value1");
test2.add("value2");
test2.add("value3");
final List<String> test3 = new LinkedList<>();
test3.add("test1");
test3.add("test2");
test3.add("test3");
test1.addAll(test2);
test3.addAll(1, test1)
}
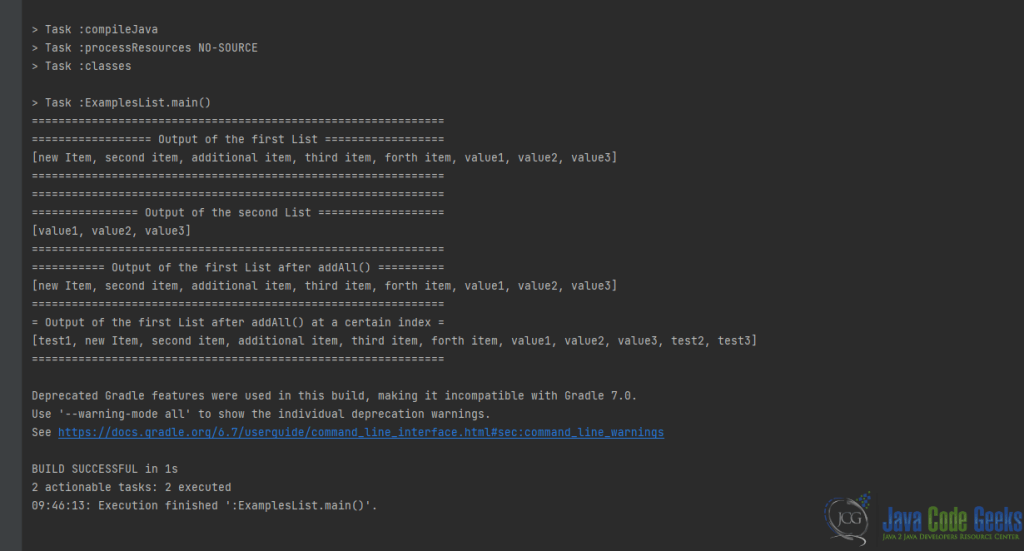
6. Common Errors that could occur
The most common errors that could occur can be:
IndexOutOfBoundsException
. This error can occur when you want to get an item from a list and the given index does not exist.ClassCastException
. It will throw this error in case the class of the elements is incompatible with the specified elements (most likely this error will appear in the ArrayList or other implementation).NullPointerException
. For some implementations ofLists
the null value is not supported. In case you provide a null value it will throw aNullPointerException
.IllegalArgumentException
. When you initialize the ArrayList with a negative value, a type of this error will be thrown.
7. Conclusion
Lists are one of the primary types of data structure that is widely used in programming. This type makes it the most used data structure from the point of usability and easy to send it between services.
In this article we covered List data structure and some of the methods that Java exposed for one of their implementations. The methods that we presented in this article are add() and addAll() with some examples. Besides these two methods we will show the common errors that occur in the List class implementation.