java.io.InputStream – InputStream Java Example
In this example, we are going to talk about a very important Java class, InputStream. If you have even the slightest experience with programming in Java, chances are that you’ve already used InputStream
in one of your programs, or one of its subclasses, like FileInputStream
or BufferedInputStream
.
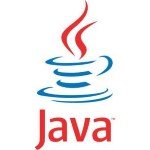
You see, java io inputstream is an abstract class, that provides all the necessary API methods you can use in order to read data from a source. That source can be anything : a console, a file, a socket, a pipe, and even an array of bytes that resides on memory. The truth is that most of the times, when reading data from source, your program is actually reading a stream of bytes that resides on memory.
But what you need to know is that a concrete java io inputstream is connected to one of the aforementioned data resources. It’s main purpose is to read data from that source an make them available for manipulation from inside your program.
1. Simple InputStream Java Examples
Ok so let’s see a simple java io inputstream example on how you can read bytes form the console.
InputStreamExample.java
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
|
package com.javacodegeeks.core.io.inputstream; import java.io.IOException; public class InputStreamExample { public static void main(String[] args){ try { System.out.println( "Available bytes :" +System.in.available()); System.out.print( "Write something :" ); int b = System.in.read(); System.out.println( "Available bytes :" +System.in.available()); System.out.println( "Input was :" +b); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } } |
Let’s clear things up: System.in
is an InputStream
that is connected to the standard input. This means that its can be used to read data from the console. In this snippet we’ve used two InputStream
API methods :
read()
: This methods reads the next byte from the input stream and returns it as an integer from 0 to 255. If theInputStream
has no more data or if it is closed,read()
will return -1.read()
is a blocking IO method. This means that it either waits until the byte is read, or returns -1 if the stream has no more data or is closed. It also throws anIOException
, that has to be handled.available()
: This method will return an estimation of the number of available bytes that you can read from theInputStream
without blocking.
If you run the program, it will output:
Available bytes :0 Write something :wepfokpd]asdfooefe02423-=VF2VWVVESAaf Available bytes :38 Input was :119
So as you can see when we initially call System.in.available()
the available bytes to read are 0, so consequently we are going to block to the next read()
call. Then we type an arbitrary string. As you can see, this string consists of 39 bytes (included is the byte of ‘\n’, because you have to press “Return/Enter” to make the typed string available to the InputStream
). When we call read()
we just read the first byte of the InputStream
, which is evaluated to 119 (read returns the byte an integer from 0 to 255). Then, you see that the output says 38 available bytes. Remember that we’ve already read one byte in the previous line, when read()
returned.
You can also choose to read a number of bytes in a byte array, instead of reading just one byte, To do that you can use public int read(byte[] b)
:
InputStreamExample.java
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
package com.javacodegeeks.core.io.inputstream; import java.io.IOException; import java.util.Arrays; public class InputStreamExample { public static void main(String[] args){ byte [] bytes = new byte [ 30 ]; try { System.out.println( "Available bytes :" +System.in.available()); System.out.print( "Write something :" ); int bytesread = System.in.read(bytes); System.out.println( "I've read :" +bytesread + " bytes from the InputStream" ); System.out.println(Arrays.toString(bytes)); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } } |
If you run the program, it will output:
Available bytes :0 Write something :sdjfsjdflksjdlfjksjdf I've read :23 bytes from the InputStream [115, 100, 106, 102, 115, 106, 100, 102, 108, 107, 115, 106, 100, 108, 102, 106, 107, 115, 106, 100, 102, 13, 10, 0, 0, 0, 0, 0, 0, 0]
As you can see I’ve read 23 bytes form the stream and placed it a byte array. An important thing to notice here is that while my byte array was 30 bytes log, it is not necessary that read will actually read 30 bytes. It will read as many bytes as possible, thus it will make an attempt to read up to 50 bytes, but it will actually read as many bytes are available up to 50. In this case, 23 bytes were available. The number of bytes it actually read is returned from read()
as an integer. If the the byte array is of length zero, then no bytes are read, and read()
will return immediately ‘0’.
You can also choose to read a number of bytes and place them in an arbitrary position in you buffer array, instead of filling up your array. To do that you can use public int read(byte[] b, int off, int len)
, where in off
you specify the offset from the start of the buffer that you want to start placing the read bytes, and len
is the number of bytes you wish to read from the stream.
InputStreamExample.java
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
package com.javacodegeeks.core.io.inputstream; import java.io.IOException; import java.util.Arrays; public class InputStreamExample { public static void main(String[] args){ byte [] bytes = new byte [ 30 ]; try { System.out.println( "Available bytes :" +System.in.available()); System.out.print( "Write something :" ); int bytesread = System.in.read(bytes, 5 , 14 ); System.out.println( "I've read :" +bytesread + " bytes from the InputStream" ); System.out.println(Arrays.toString(bytes)); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } } |
If you run the program, it will output:
Available bytes :0 Write something :posjdpojsdpocjspojdcopsjcdpojspodcjpsjdocjpsdca[spdc I've read :14 bytes from the InputStream [0, 0, 0, 0, 0, 112, 111, 115, 106, 100, 112, 111, 106, 115, 100, 112, 111, 99, 106, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
As you can see, the bytes we places from bytes[6] to bytes[19]. All other bytes are unaffected.
2. Reading characters form InputStream
When you’re dealing with binary data, it is usually fine to read bytes from the input stream. But, as you might agree, reading bytes is not always handy, especially when reading streams of characters, like we did in the example. For that, Java offers special Reader
classes, that convert byte streams to character streams. It does that by simply parsing the bytes and encoding them according to character set encoding (you can do that on your own, but don’t even bother). Such a Reader
is InputStreamReader
. To create an InputStreamReader
, you give it an InputStream
as an argument in its constructor, optionally along with a character set (or else the default will be used to encode the characters).
Let’s see how you can use it to read characters from the console:
InputStreamExample.java
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
|
package com.javacodegeeks.core.io.inputstream; import java.io.IOException; import java.io.InputStreamReader; import java.util.Arrays; public class InputStreamExample { public static void main(String[] args){ char [] characters = new char [ 30 ]; try { InputStreamReader inputReader = new InputStreamReader(System.in, "utf-8" ); System.out.print( "Write some characters :" ); int bytesread = inputReader.read(characters); System.out.println( "I've read :" +bytesread + " characters from the InputStreamReader" ); System.out.println(Arrays.toString(characters)); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } } |
If you run the program, it will output :
Write some characters :JavaCodeGeeks I've read :15 characters from the InputStreamReader [J, a, v, a, C, o, d, e, G, e, e, k, s, , , , , , , , , , , , , , , , , , ]
So as you can see, I can now read characters instead of bytes. Of course public int read(char[] cbuf, int offset, int length)
method is also available from the Reader
that offers the same basic functionality as we’ve described before in the case of InpuStream
. Same goes for read()
, but instead of reading one bytes, it reads one character.
3. Using BufferedReader
You can also buffer a Reader
, mainly for efficiency. But, you can also take advantage of it when reading character streams, as you can pack characters in Strings
. Thus, you can read a text input stream line by line.
Let’s see how :
InputStreamExample.java
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
|
package com.javacodegeeks.core.io.inputstream; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; public class InputStreamExample { public static void main(String[] args){ try { InputStreamReader inputReader = new InputStreamReader(System.in, "utf-8" ); BufferedReader buffReader = new BufferedReader(inputReader); System.out.print( "Write a line :" ); String line = buffReader.readLine(); System.out.println( "Line read :" +line); } catch (IOException e) { e.printStackTrace(); } } } |
If you run the program, it will output:
Write a line :Java Code Geeks Rock ! Line read :Java Code Geeks Rock !
You can still use public int read(char[] cbuf, int off, int len)
methods to read characters to buffers, if you want. Buffered reader will efficiently read bytes, using an internal buffer. It appends the input in that buffer and performs its conversions there. The size of that internal buffer can be specified, if the default of 512 characters is not enough for you, using public BufferedReader(Reader in, int sz)
constructor, in the sz
argument.
4. Read files using FileInputStream
FileInputStream
is a sub class of InputStream
that is used to read files. Let’s see how you can use it :
InputStreamExample.java:
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
|
package com.javacodegeeks.core.io.inputstream; import java.io.FileInputStream; import java.io.IOException; import java.io.InputStream; import java.util.Arrays; public class InputStreamExample { private static final String FILE_PATH= "F:\\nikos7\\Desktop\\testFiles\\textFile.txt" ; public static void main(String[] args){ InputStream fileInputStream = null ; byte [] bytes = new byte [ 20 ]; try { fileInputStream = new FileInputStream(FILE_PATH); System.out.println( "Available bytes of file:" +fileInputStream.available()); int bytesread = fileInputStream.read(bytes, 0 , 15 ); System.out.println( "Bytes read :" +bytesread); System.out.println(Arrays.toString(bytes)); } catch (IOException e) { e.printStackTrace(); } finally { try { fileInputStream.close(); } catch (IOException e) { e.printStackTrace(); } } } } |
If you run the program, it will output:
Available bytes of file:173 Bytes read :15 [111, 112, 97, 112, 111, 115, 106, 99, 100, 111, 97, 115, 100, 118, 111, 0, 0, 0, 0, 0]
If you want to read buffered binary data, thus no need to use a Reader
, you can use BufferedInputStream
.
InputStreamExample.java
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
|
package com.javacodegeeks.core.io.inputstream; import java.io.BufferedInputStream; import java.io.FileInputStream; import java.io.IOException; import java.io.InputStream; import java.util.Arrays; public class InputStreamExample { private static final String FILE_PATH= "F:\\nikos7\\Desktop\\testFiles\\textFile.txt" ; public static void main(String[] args){ InputStream fileInputStream = null ; BufferedInputStream bufferedInputStream = null ; byte [] bytes = new byte [ 512 ]; try { fileInputStream = new FileInputStream(FILE_PATH); bufferedInputStream = new BufferedInputStream(fileInputStream, 1024 ); int bytesread = bufferedInputStream.read(bytes, 0 , 512 ); System.out.println( "Bytes read :" +bytesread); System.out.println(Arrays.toString(bytes)); } catch (IOException e) { e.printStackTrace(); } finally { try { bufferedInputStream.close(); } catch (IOException e) { e.printStackTrace(); } } } } |
As you can see here, we’ve specified the internal buffer to be 1024 bytes.
If you run the program, it will output:
Bytes read :173 [111, 112, 97, 112, 111, 115, 106, 99, 100, 111, 97, 115, 100, 118, 111, 112, 97, 115, 100, 118, 13, 10, 97, 115, 100, 118, 111, 112, 97, 115, 111, 100, 106, 118, 111, 112, 106, 97, 112, 115, 111, 118, 91, 97, 115, 100, 118, 13, 10, 112, 111, 97, 115, 100, 118, 112, 111, 106, 97, 115, 100, 118, 91, 97, 115, 107, 100, 118, 91, 112, 107, 91, 13, 10, 115, 97, 100, 118, 112, 115, 111, 106, 100, 118, 111, 106, 115, 112, 111, 100, 118, 106, 13, 10, 115, 100, 118, 111, 106, 112, 111, 106, 118, 112, 97, 111, 115, 106, 100, 112, 118, 106, 112, 111, 97, 115, 106, 100, 118, 13, 10, 97, 115, 106, 100, 118, 111, 106, 112, 97, 111, 115, 106, 100, 112, 118, 106, 112, 97, 111, 115, 106, 100, 118, 97, 115, 100, 118, 13, 10, 97, 111, 115, 100, 98, 102, 112, 106, 97, 111, 115, 106, 100, 111, 98, 106, 97, 115, 112, 111, 100, 98, 106, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
Of course you can use all the above methods to bridge the byte stream to a character streams. So let’s see how you can read a text file,line by line
InputStreamExample.java
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
|
package com.javacodegeeks.core.io.inputstream; import java.io.BufferedInputStream; import java.io.BufferedReader; import java.io.FileInputStream; import java.io.IOException; import java.io.InputStream; import java.io.InputStreamReader; public class InputStreamExample { private static final String FILE_PATH= "F:\\nikos7\\Desktop\\testFiles\\textFile.txt" ; public static void main(String[] args){ InputStream fileInputStream = null ; BufferedReader bufferedReader = null ; try { fileInputStream = new FileInputStream(FILE_PATH); bufferedReader = new BufferedReader( new InputStreamReader(fileInputStream)); String line= "" ; while ( (line = bufferedReader.readLine()) != null ){ System.out.println(line); } } catch (IOException e) { e.printStackTrace(); } finally { try { bufferedReader.close(); } catch (IOException e) { e.printStackTrace(); } } } } |
If you run the program, it will output:
opaposjcdoasdvopasdv asdvopasodjvopjapsoveasdv poasdvpojasdvwaskdvepke sadvpsojdvojspodvj sdvojpojvpaosjdpvjpoasjdv asjdvojpaosjdpvjpaosjdvasdv aosdbfpjaosjdobjaspodbj
5. Read data from memory
To read data form memory, you can use a different subclass of InputStream
, ByteArrayInputStream
.
Let’s see how you can use it:
InputStreamExample.java:
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
|
package com.javacodegeeks.core.io.inputstream; import java.io.BufferedReader; import java.io.ByteArrayInputStream; import java.io.IOException; import java.io.InputStream; import java.io.InputStreamReader; import java.util.Arrays; public class InputStreamExample { public static void main(String[] args){ String str1 = "aosdjfopsdjpojsdovjpsojdvpjspdvjpsjdv" ; String str2 = "aosdjfopsdjpojsdovjpsojdvpjspdvjpsjdv \n" + "sidjvijsidjvisjdvjiosjdvijsiodjv \n" + "ajsicjoaijscijaisjciajscijaiosjco \n" + "asicoaisjciajscijascjiajcsioajsicjioasico" ; byte [] bytes = new byte [ 512 ]; InputStream inputStream = new ByteArrayInputStream(str1.getBytes()); BufferedReader bufReader = new BufferedReader( new InputStreamReader ( new ByteArrayInputStream(str2.getBytes()))); try { int bytesread = inputStream.read(bytes, 0 , 50 ); System.out.println( "Bytes read from str1 :" +bytesread); System.out.println( "Bytes read from str1 :" +Arrays.toString(bytes)); String line = "" ; while ( (line = bufReader.readLine()) != null ){ System.out.println( "Line of str1 :" + line); } } catch (IOException e) { e.printStackTrace(); } finally { try { bufReader.close(); inputStream.close(); } catch (IOException e) { e.printStackTrace(); } } } } |
If you run the program, it will output:
Bytes read from str1 :37 Bytes read from str1 :[97, 111, 115, 100, 106, 102, 111, 112, 115, 100, 106, 112, 111, 106, 115, 100, 111, 118, 106, 112, 115, 111, 106, 100, 118, 112, 106, 115, 112, 100, 118, 106, 112, 115, 106, 100, 118, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0] Line of str1 :aosdjfopsdjpojsdovjpsojdvpjspdvjpsjdv Line of str1 :sidjvijsidjvisjdvjiosjdvijsiodjv Line of str1 :ajsicjoaijscijaisjciajscijaiosjco Line of str1 :asicoaisjciajscijascjiajcsioajsicjioasico
6. Reading from other sources
As you might image an sublclass of InputStream
is present to help you read from all aforementioned sources. Most IO classes have an interface method that enables you to obtain an InputStream
connected to a particular source. From then on, you can use all the above methods to enable buffering, or to simply bridge a byte stream to a character stream.
7. Mark and Reset
Java io InputStream
offers to methods mark
and reset
. mark
is used to place a marker in the current position of the stream. You can think of it as a pointer
to the present point of the stream. As you scan though the stream you can place markers at arbitrary points. After a while, if you call reset, the “cursor” of the stream will go to the last marker you’ve placed, so you can re-read the same data, particularly useful to perform error correction e.t.c.
Let’s see an example here with BufferedReader
. Using them with InputStream
and its subclasses is the exact same thing.
InputStreamExample.java
01
02
03
04
05
06
07
08
09
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
|
package com.javacodegeeks.core.io.inputstream; import java.io.BufferedReader; import java.io.ByteArrayInputStream; import java.io.IOException; import java.io.InputStreamReader; import java.util.Arrays; public class InputStreamExample { public static void main(String[] args){ String str1 = "Java Code Geeks Rock!" ; char [] cbuf = new char [ 10 ]; BufferedReader bufReader = new BufferedReader( new InputStreamReader ( new ByteArrayInputStream(str1.getBytes()))); try { int charsread = bufReader.read(cbuf, 0 , 5 ); System.out.println(Arrays.toString(cbuf)); bufReader.mark( 120 ); charsread = bufReader.read(cbuf, 0 , 5 ); System.out.println(Arrays.toString(cbuf)); bufReader.reset(); charsread = bufReader.read(cbuf, 0 , 5 ); System.out.println(Arrays.toString(cbuf)); } catch (IOException e) { e.printStackTrace(); } finally { try { bufReader.close(); } catch (IOException e) { e.printStackTrace(); } } } } |
The integer “120” argument you see in the mark
method is a threshold for the maximum number of bytes that can be read before releasing the marker. So, here if we read more than 120 characters the marker will be removed.
If you run the program, it will output:
[J, a, v, a, , , , , ] [C, o, d, e, , , , , ] [C, o, d, e, , , , , ]
8. Download Source Code
This was a java.io.InputStream Example.
You can download the source code of this example here: java.io.InputStream – InputStream Java Example
Last updated on May 28th, 2020