Core Java
Java Heap and Stack
Hello readers, in this tutorial, we will learn about Heap space and Stack in Java.
1. Introduction
In Java, the Java Virtual Machine (JVM) divides the memory into two parts i.e. Java Heap Space and Java Stack Memory.
1.1 Java Heap Space?
- Created by the JVM when it starts and the memory is allocated until the application is running
- Memory is allocated to Java Runtime (JRE) classes and the objects
- The objects created in the heap space has global access and can be accessed from anywhere in the application
- Managed by Garbage collection to perform the memory cleaning activity by removing the objects that don’t have any reference
1.2 Java Stack Memory?
- Used for the execution of threads
- Memory is allocated to methods, local variables, and reference variables
- Memory is referenced in LIFO (Last in first out) order and allows fast access to those values
- On invoking a method, a block is created in the Stack memory to hold the local variables and reference to other objects in the method. As the method ends its execution, the created block becomes unused and is available for the next method
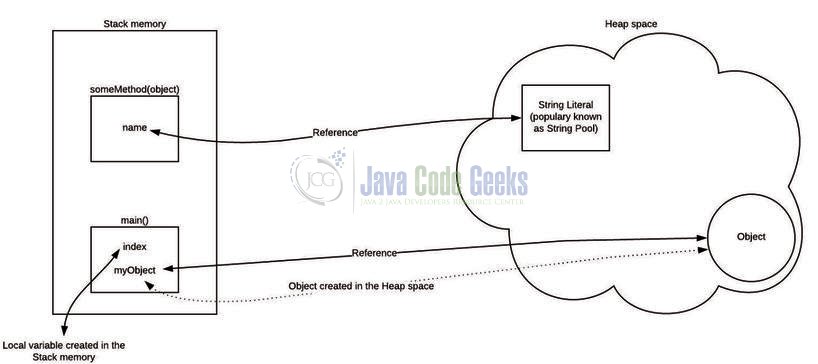
2. Heap Space vs. Stack Memory?
Heap Space | Stack Memory | |
---|---|---|
Basic | Allocates memory to JRE classes and objects. Starting JDK7 String pool is also a part of this space | Allocates memory to methods, local variables (like int, boolean, float, etc.), and reference variables |
Memory | Offers dynamic allocation of memory and is stored in any order | References in LIFO (Last in first out) order and thus offer recursion |
Size | Much bigger than Stack Memory | |
Memory Resizing | Memory size can be adjusted by using the JVM -Xms and -Xmx parameters | Memory size can be increased by readjusting the JVM -XSS parameter |
Visibility | Visible to all threads as it offers global access for the objects | Visible only to the owner’s thread |
Exception | Throws java.lang.OutOfMemoryError if no Heap space is left to allocate memory to the new object | Throws java.lang.StackOverFlowError when Stack memory is filled up with an infinite recursive class |
3. Sample program
Let us understand the different memory allocations through the sample code.
Employee.java
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 | package java.memoryallocation; // As soon as this program run it loads the runtime classes into the Heap space. public class Employee { // The main() found creates a Stack memory that will be used by the main() method thread. public static void main(String[] args) { // This local variable is created and stored in the stack memory of the main() method. int index = 1 ; // This object creation is created in the Heap space and the Stack memory contains a reference for it. Object myObject = new Object(); // This object creation is created in the Heap space and the Stack memory contains a reference for it. Employee myEmployee = new Employee(); // Now calling the "someMethod()", a block in the top of the Stack memory is created and is used by // the "someMethod()" method. Since Java is pass-by-value in nature, a new reference to Object is created // in the "someMethod" stack block. myEmployee.someMethod(myObject); } // At this point the "main()" terminates as it has done it job and the Stack space created for // "main()" method is destroyed. Also, the program ends and hence the JRE frees all the memory // and ends the program execution. private void someMethod(Object object) { // The string created goes to the "String Pool" that residers in the heap space and the reference of it // is created in the "someMethod()" stack space. String name = object.toString(); System.out.println( "Name= " + name); } // At this point the "someMethod()" terminates and the memory block allocated for "someMethod()" // in the Stack space becomes free. } |
4. StackOverFlowErrror and OutOfMemoryError?
StackOverflowError | OutOfMemoryError |
---|---|
Related to Stack memory | Related to Heap space |
Occurs when Stack memory is full | Occurs when Heap space is full |
Thrown when we call a method and the Stack memory is full | Thrown we create a new object and the Heap space is full |
Can occur due to infinite recursions of a method | Can occur due to the creation of multiple objects |
Ensuring method execution is completed and the Stack memory is reclaimed | Garbage collection helps in reclaiming Heap space and avoid OutOfMemory error |
5. Summary
In this tutorial, we learned about Heap and Stack in Java. We saw the Heap Space vs. Stack Memory comparison. We have also created a sample program to understand the different memory allocations. You can download the sample class from the Downloads section.
That is all for this tutorial and I hope the article served you with whatever you were looking for. Happy Learning and do not forget to share!
6. Additional Knowledge
7. Download the Eclipse Project
This was an example of Java Heap and Stack.
Download
You can download the full source code of this example here: Java Heap and Stack
You can download the full source code of this example here: Java Heap and Stack
Fantastic article