Java Finally keyword Example
1. Introduction
In this post, we will discuss the Finally keyword in Java. This keyword is used for a block as part of a try-catch. Java finally block is a block that is used to execute important codes such as closing connection, stream, etc.
Java finally block is always executed whether an exception is handled or not. This ensures that the code inside the finally block is executed irrespective of an exception being raised or handled inside a try block. Any code which we want to get executed irrespective of an exception is written inside a finally block. Usually, closing database connections, streams or resources ensures that there is no resource leak even though if any mishap occurs during our program execution is done inside a finally block.
2. Java Finally Keyword Examples
Now let us understand using this keyword block under various conditions so that we have a good understanding of it. We shall see the usage of this keyword block under various cases like there is no exception inside a try block, an exception occurred inside a try block but it is not handled, an exception occurred inside a try block and it is handled.
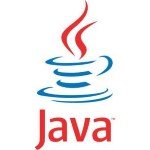
2.1 Without any Exception
In this example, we shall use the this keyword block when there is no exception inside a try block.
public class Example1 { public static void main(String[] args) { try { int result=4/2; System.out.println("The result is:"+result); System.out.println("This is inside a try-block"); } catch(Exception e) { System.out.println("Inside a catch-block"); } finally { System.out.println("Inside a finally-block"); } } }
Output
The result is:2 This is inside a try-block Inside a finally-block
In the above example, there was no exception inside a try block. All the statements were executed inside a try block. Once the execution is finished, the execution of the finally block took place. The print statement as part of the finally block is seen in the output.
2.2 With a Handled Exception
In this example, we shall use the finally block when there is an exception inside a try block and is handled.
public class Example2 { public static void main(String[] args) { try { int result=5/0; System.out.println("The result is"+result); System.out.println("Inside a try-block"); } catch( ArithmeticException ae) { System.out.println("Please check the denominator"); System.out.println("Inside a catch-block"); } finally { System.out.println("Inside a finally-block"); } } }
Output
Please check the denominator Inside a catch-block Inside a finally-block
In the above example, at line 6 an exception has occurred as part of the try block. The execution immediately moves to the catch block as the catch block handles corresponding Arithmetic Exception which was occurred in the try block. All the statements inside the catch block were executed. Once the execution of the catch block was finished, the finally block was executed at the end.
2.3 With an Exception that is not handled
In this example, we shall use the finally block when there is an exception inside a try block and is not handled.
public class Example3 { public static void main(String[] args) { try { int result=5/0; System.out.println("The result is"+result); System.out.println("Inside a try-block"); } catch( NullPointerException ne) { System.out.println("Please check the denominator"); System.out.println("Inside a catch-block"); } finally { System.out.println("Inside a finally-block"); } } }
Output
Inside a finally-block Exception in thread "main" java.lang.ArithmeticException: / by zero at Test.main(Example3.java:6)
In this example the exception was raised in try block was not handled as the catch block was handling NullPointerException. So the exception message was seen in the output and the finally block was still executed.
Need to use the Finally Block: As part of our programming requirements, we interact with databases by opening connections, use input and output streams, resources inside a try block. As a good programmer, to ensure the safety of resources and to make sure that there is no resource leak as part of our program, it is advisable to use the finally block along with try-catch blocks.
3. Download the Source Code
This is an example of Java Finally keyword Example.
You can download the full source code of this example here: Java Finally keyword Example