Java Exercises
In this post, we feature an article with Java Exercises. In this tutorial, we will see the Java features in detail through code examples.
1. Overview
We look at the features of Java in this article. Java is used for developing software and executing the code. The exercises help to demonstrate the java language concepts.
Table Of Contents
- 1. Overview
- 2. Java Exercises
- 2.1. Prerequisites
- 2.2. Download
- 2.3. Setup
- 2.4. IDE
- 2.5. Launching IDE
- 2.6. Hello World Program
- 2.7. Data Types
- 2.8. Primitive Data Types
- 2.9. Operators
- 2.10. If Else
- 2.11. Loops
- 2.12. Arrays
- 2.13. Objects
- 2.14. Inheritance
- 2.15. Encapsulation
- 2.16. Keywords
- 2.17. Classes
- 2.18. Annotations
- 2.19. Exceptions
- 2.20. Methods
- 2.21. Constructors
- 3. Download the Source Code
2. Java Exercises
Java language is an object-oriented language. It is platform-independent and architectural neutral. Java exercises are useful for programmers who are preparing for interviews and certification. They help in understanding the java language concepts from the code samples.
2.1 Prerequisites
Java 8 is required on the linux, windows or mac operating system. Eclipse Oxygen can be used for this example.
2.2 Download
You can download Java 8 from the Oracle web site . Eclipse Oxygen can be downloaded from the eclipse web site.
2.3 Setup
Below is the setup commands required for the Java Environment.
Setup
JAVA_HOME="/desktop/jdk1.8.0_73" export JAVA_HOME PATH=$JAVA_HOME/bin:$PATH export PATH
2.4 IDE
The ‘eclipse-java-oxygen-2-macosx-cocoa-x86_64.tar’ can be downloaded from the eclipse website. The tar file is opened by double click. The tar file is unzipped by using the archive utility. After unzipping, you will find the eclipse icon in the folder. You can move the eclipse icon from the folder to applications by dragging the icon.
2.5 Launching IDE
Eclipse has features related to language support, customization, and extension. You can click on the eclipse icon to launch eclipse. You can select the workspace from the screen which pops up. You can see the eclipse workbench on the screen.
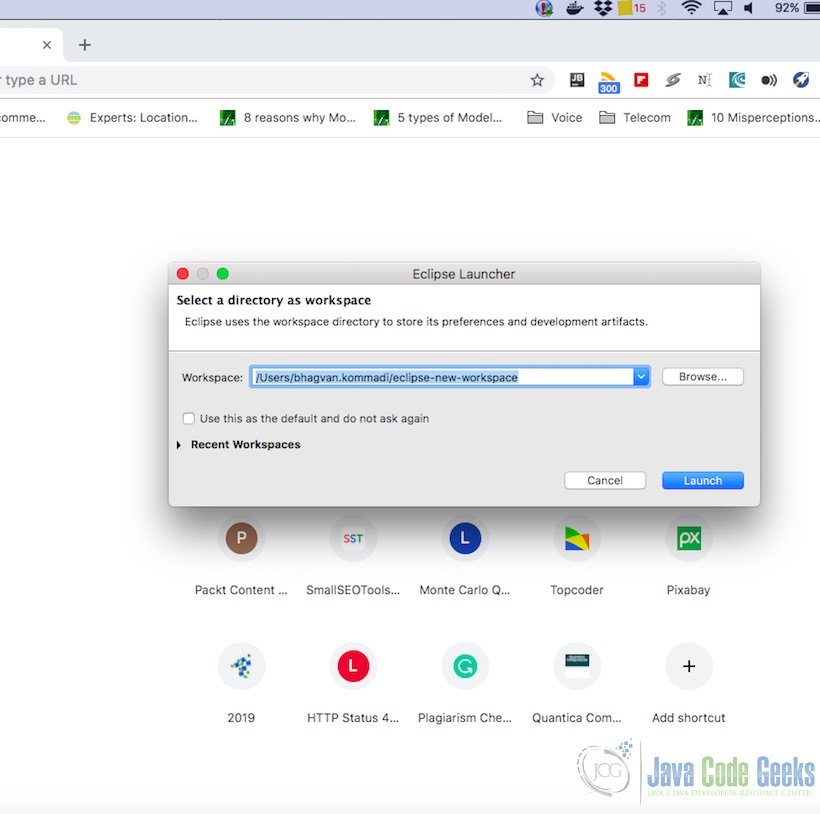
2.6 Hello World Program
Java Hello World
program code is presented below. The class has main
method which prints out the greetings message. System.out.println
is used for printing the messages.Hello World
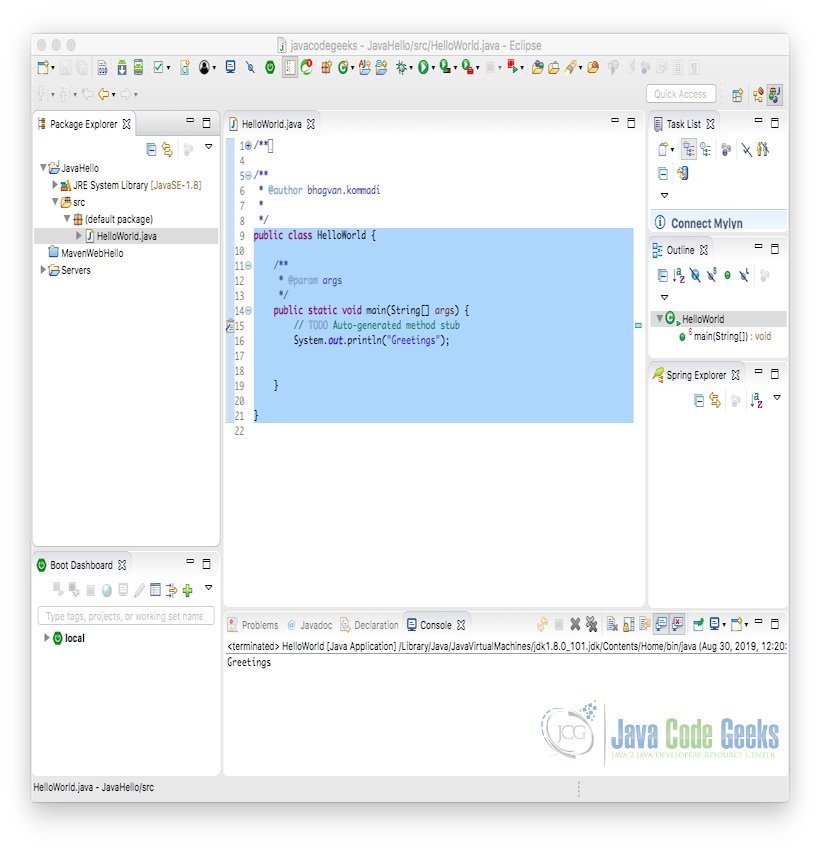
2.7 Data Types
You can look at the code below before answering the multiple-choice. Which of the multiple-choice answers will be the output of the below code ?
Data Types Exercise
class DataTypesExercise { public static void main(String args[]) { int integervariable; System.out.println(integervariable); } }
- NaN
- 0
- runtime error
- compilation error
The answer is 4. The integer variable is not initialized. When you compile the code, you will get an error message.
2.8 Primitive Data Types
You can look at the code below before answering the multiple-choice. Which of the multiple-choice answers will be the output of the below code?
Primitive Data Types Exercise
class PrimitiveExercise { public static void main(String[] args) { Double doubleInstance = new Double("4.4"); int integervar = doubleInstance.intValue(); System.out.println(integervar); } }
- 0
- 4
- 0.4
- 3
The answer is 2. The output will be 4. The integer value of the double instance is 4.
2.9 Operators
You can look at the code below before answering the multiple-choice. Which of the multiple-choice answers will be the output of the below code?
Operators Exercise
class OperatorExercise { public static void main(String args[]) { int negativeInteger = -2; System.out.println(negativeInteger >>1); int positiveInteger = 2; System.out.println(positiveInteger>>1); } }
- 1, -1
- -1, 1
- 0,0
- compilation error
The answer is 2. The output will be -1 and 1. Right shift operator takes two numbers as input. It right shifts the bits of the first operand. The next operand is used for shifting the number of places.
2.10 If else
You can look at the code below before answering the multiple-choice. Which of the multiple-choice answers will be the output of the below code?
If Else Exercise
class IfElseExercise { public static void main(String[] args) { boolean boolvar = false; if (boolvar = false) { System.out.println("FALSE"); } else { System.out.println("TRUE"); } } }
- FALSE
- TRUE
- Compilation Error
- Runtime Error
The answer is 2. The output value is TRUE. In the condition of if statement, we are assigning false to boolvar. The assignment returns false. Hence the control goes to the else section. The output is TRUE.
2.11 Loops
You can look at the code below before answering the multiple-choice. Which of the multiple-choice answers will be the output of the below code ?
Loops Exercise
class LoopExercise { public static void main(String[] args) { int firstvar = 0; int secondvar = 4; do { firstvar++; if (secondvar-- < firstvar++) { break; } } while (firstvar < 3); System.out.println(firstvar + "" + secondvar); } }
- 45
- 54
- 42
- 34
Answer is 3. The output is 42. firstvar
keeps increasing till it reaches 3. Once it reaches the value 2. The secondvar
will be 2 after decrement and break. The firstvar
is incremented twice before break. The firstvar
value will be 4 and secondvar
will be 2.
2.12 Arrays
You can look at the code below before answering the multiple-choice. Which of the multiple-choice answers will be the output of the below code ?
Arrays Exercise
public class ArraysExercise { public static void main(String args[]) { int arrayInstance[] = {11, 27, 31, 84, 94}; for(int j=0; j < arrayInstance.length; j++) { System.out.print( j+ " "+ arrayInstance[j]+ " "); } } }
- 1 11 2 27 3 31 4 84 5 94
- 0 11 1 27 2 31 3 84 4 94
- compilation error
- run time error
The Answer is 2. The output will be index starting from 0 and elements starting from 11. j starts from 0.
2.13 Objects
You can look at the code below before answering the multiple-choice. Which of the multiple-choice answers will be the output of the below code ?
Data Types Exercise
class Parallelogram { int length, width; Parallelogram() { length = 25; width = 46; } public void draw() { System.out.println ("length = " + length + " width = " + width); } } class ObjectsExercise { public static void main(String[] args) { Parallelogram shape1 = new Parallelogram(); Parallelogram shape2 = shape1; shape1.length += 2; shape1.width += 2; System.out.println ("Parallelogram 1 length and width "); shape1.draw(); System.out.println ("Parallelogram 2 length and width"); shape2.draw(); } }
a) Parallelogram 1 length and width
length = 27 width = 48
Parallelogram 2 length and width
length = 25 width = 46
b) Parallelogram 1 length and width
length = 27 width = 48
Parallelogram 2 length and width
length = 27 width = 48
c) Runtime Error
d) Compilation Error
The answer is b. The output will be the same for Parallelolgram1 and Parallelolgram2. The length
and width
will be incremented by 2.
2.14 Inheritance
You can look at the code below before answering the multiple-choice. Which of the multiple-choice answers will be the output of the below code?
Inheritance Exercise
class Shape { public void draw() { System.out.println("draw method invoked"); } } class Rectangle extends Shape { public void draw() { System.out.println("Rectangle draw method invoked"); } } public class InheritanceExercise { public static void main(String[] args) { Shape shape = new Rectangle(); shape.draw(); } }
- draw method invoked
- Rectangle draw method invoked
- throws a compilation error
- throws a runtime error
The answer is 2. The output is “Rectangle draw method invoked”. shape object is created using the derived class Rectangle
constructor. When the draw
method is invoked, shape object picks the Rectangle
draw
method.
This is related to polymorphism. A java object can take multiple forms. When a parent class object refers to a child class, the object takes the form of child class. getClass
method of the parent class object will return the child class.
Let us look at another exercise. You can look at the code below before answering the multiple-choice. Which of the multiple-choice answers will be the output of the below code?
Polymorphism Exercise
class Figure { public final void draw() { System.out.println("draw method invoked"); } } class RectangleFigure extends Figure { public void draw() { System.out.println("Rectangle draw method invoked"); } } public class PolymorphismExercise { public static void main(String[] args) { Figure figure = new RectangleFigure(); figure.draw(); } }
- draw method invoked
- Rectangle draw method invoked
- throws a compilation error
- throws a runtime error
The Answer is 3. Compilation error is thrown as Shape
has a final method that is overridden in Rectangle
. Rectangle
is the derived class of Shape
.
2.15 Encapsulation
You can look at the code below before answering the multiple-choice. Which of the multiple-choice answers will be the output of the below code?
Encapsulation Exercise
class Square { int width; } class EncapsulationExercise { public static void main(String args[]) { Square square; System.out.println(square.width); } }
- 0
- Compilation error – width is not accessible
- Runtime error
- Compilation error – square is not initialized
The answer is 4. Compilation Error is thrown because Square instance is not initialized.
2.16 Keywords
You can look at the code below before answering the multiple-choice. Which of the multiple-choice answers will be the output of the below code?
KeyWords Exercise
class KeyWordsExercise { public static void main(String args[]){ final int integervar; integervar = 34; System.out.println(integervar); } }
- 0
- 34
- compilation error
- runtime error
The answer is 2. The output will be 34. The final integervar is first time initialized to 34. Hence the output will be 34.
2.17 Classes
You can look at the code below before answering the multiple-choice. Which of the multiple-choice answers will be the output of the below code?
Classes Exercise
abstract class Vehicle { public int speed; Vehicle() { speed = 10; } abstract public void setSpeed(); abstract final public void getSpeed(); } class Truck extends Vehicle { public void setSpeed(int speed) { this.speed = speed; } final public void getSpeed() { System.out.println("Speed = " + speed); } public static void main(String[] args) { Truck truck = new Truck(); truck.setSpeed(30); truck.getSpeed(); } }
- Speed = 30
- Speed = 0
- Compilation error
- Runtime error
The answer is 3. A Compilation error occurs because abstract and final are used in the method getSpeed
declaration.
2.18 Annotations
Select one of the following which is not a predefined java annotation :
- @Deprecated
- @FunctionInterface
- @Overriden
- @SafeVarags
The answer is 3. @Overriden is not a pre-defined java annotation. @Deprecated
, @Override
, @SuppressWarnings
, @SafeVarags
and @FunctionInterface
are the predefined java annotations.
2.19 Exceptions
You can look at the code below before answering the multiple-choice. Which of the multiple-choice answers will be the output of the below code?
Exceptions Exercise
class CheckException extends Exception { } class ExceptionExercise { public static void main(String args[]) { try { throw new CheckException(); } catch(CheckException exception) { System.out.println("Check Exception Occured"); } finally { System.out.println("finally block executed"); } } }
- Compilation Error
- CheckException Occurred
- CheckException Occurred
finally block executed - finally block executed
The answer is 3. The output message will be “Check exception Occured” and “finally block executed”. In the try block, CheckException
is thrown. Catch block catches and prints the output. The finally block gets executed to print the output from the finally block.
2.20 Methods
You can look at the code below before answering the multiple-choice. Which of the multiple-choice answers will be the output of the below code?
Methods Exercise
class MethodExercise { public static void main(String args[]) { System.out.println(method()); } static int method() { int intvar = 4; return intvar; } }
- NaN
- 4
- Runtime error
- Compilation error
The answer is 2. The output is 4. The static method is invoked and the intvar value is returned. The output printed is intvar value 4.
2.21 Packages
You can look at the code below before answering the multiple-choice. Which of the multiple-choice answers will be the output of the below code?
Packages Exercise
import static java.lang.System.out; class PackageExercise { public static void main(String args[]) { out.println("packages"); } }
- Runtime error
- Compiler Error
- packages
- None of the above
The answer is 3. The output is packages. The static import has the package java.lang.System.out
out can be used for invoking the method println
. The output is printed.
2.22 Constructors
You can look at the code below before answering the multiple-choice. Which of the multiple-choice answers will be the output of the below code?
Constructors Exercise
class Coordinates { int xcoord, ycoord; public Coordinates() { xcoord = 5; ycoord = 5; } public int getXCoord() { return xcoord; } public int getYCoord() { return ycoord; } public static void main(String args[]) { Coordinates coordinates = new Coordinates(); System.out.println(coordinates.getYCoord()); } }
- 0
- 5
- Compilation error
- Runtime error
The answer is 2. The output is 5. The default constructor of the Coordinates
class sets the xcoord
and ycoord
to value 5. The output will be the ycoord
value.
3. Download the Source Code
You can download the full source code of this example here: Java Exercises