Java Exceptions List Example
In this article we will discuss the Java exceptions list. We will discuss what are exceptions, when they occur and their types.
You can also check this tutorial in the following video:
1. What is An Exception in Java?
Exception is mechanism which Java uses to handle any unforeseen use-case/scenario. Basically an exception is thrown when either something unexpected happened during code execution which is not covered in any code block.
Table Of Contents
- 1. What is An Exception in Java?
- 2. Java Error Handling Mechanisms
- 3. Java Exceptions List
-
- 3.1 CloneNotSupportedException
- 3.2 InterruptedException
- 3.3 ReflectiveOperationException
- 3.4 RuntimeException
-
- 3.4.1. ArithmeticException
- 3.4.2. ArrayStoreException
- 3.4.3. ClassCastException
- 3.4.4. EnumConstantNotPresentException
- 3.4.5. IllegalArgumentException
- 3.4.6. IllegalMonitorStateException
- 3.4.7. IllegalStateException
- 3.4.8. IndexOutOfBoundsException
- 3.4.9. NegativeArraySizeException
- 3.4.10. NullPointerException
- 3.4.11. SecurityException
- 3.4.12. TypeNotPresentException
- 3.4.13. UnsupportedOperationException
- 4. Summary
- 5. Download Source Code
2. Java Error Handling Mechanisms
In this section we will cover the Java error handling mechanisms.
2.1 Throwable Class
The Throwable
class is the superclass of all errors and exceptions in the Java language. Only objects that are instances of this class (or one of its subclasses) are thrown by the Java Virtual Machine or can be thrown by the Java throw
statement. Similarly, only this class or one of its subclasses can be the argument type in a catch
clause.
Instances of two subclasses, Error
and Exception
, are conventionally used to indicate that exceptional situations have occurred. Typically, these instances are freshly created in the context of the exceptional situation so as to include relevant information (such as stack trace data).
2.2 Error Class
An Error
is a subclass of Throwable
that indicates serious problems that a reasonable application should not try to catch. Most such errors are abnormal conditions.
A method is not required to declare in its throws
clause any subclasses of Error
that might be thrown during the execution of the method but not caught, since these errors are abnormal conditions that should never occur.
Hence, Error
and its subclasses are regarded as unchecked exceptions for the purposes of compile-time checking of exceptions.
2.3 Exception Class
The class Exception
and any subclasses that doesn’t descend from RuntimeException
are called checked exceptions.
Checked exceptions need to be declared in a method or constructor’s throws
clause so that they can be thrown by the execution of the method or constructor and propagate outside the method or constructor boundary.
In this article we will be focused mostly on the Exception
hierarchy.
3. Java Exceptions List
In this section we will cover all the exception classes defined in Java.
3.1 CloneNotSupportedException
Thrown to indicate that the clone
method in any class has been called to clone an object, but that the class does not implement the Cloneable
interface.
Can be used to handle exception around the clone
method while overriding to implement custom cloning. More details can be found here.
Example is shown in the code snippet below.
CloneException.java
public class CloneException {
String name;
CloneException(String name) {
this.name = name;
}
public static void main(String[] args) {
try {
CloneException expOne = new CloneException("CloneException");
CloneException expTwo = (CloneException) expOne.clone();
System.out.println(expTwo.name);
} catch (CloneNotSupportedException c) {
c.printStackTrace();
}
}
}
Output of CloneException.java is shown in Fig. 1 below.
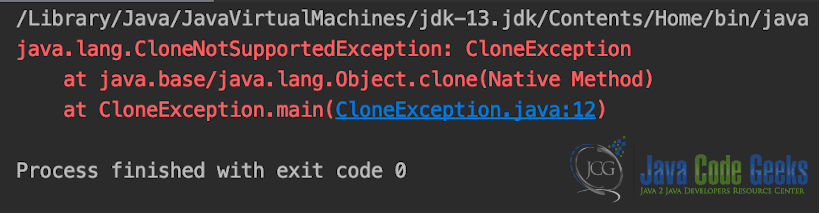
3.2 InterruptedException
Thrown when a thread is waiting, sleeping, or otherwise occupied, and the thread is interrupted, either before or during the activity. More details can be found here.
Example of this exception is shown in the code snippet below.
InteruptExcetption.java
public class InteruptExcetption extends Thread {
public void run() {
for (int i = 0; i < 10; i++) {
try {
Thread.sleep(1000);
if (i == 7) {
throw new InterruptedException(); // to simulate the interrupt exception
}
} catch (InterruptedException e) {
System.err.println("Sleep is disturbed. " + e);
e.printStackTrace();
}
System.out.println("Iteration: " + i);
}
}
public static void main(String args[]) {
InteruptExcetption exceptionOne = new InteruptExcetption();
exceptionOne.start();
try {
exceptionOne.join();
} catch (InterruptedException e) {
System.err.println("Properly not joined with parent thread. " + e);
e.printStackTrace();
}
}
}
Output of the InteruptExcetption.java is shown in Fig.2 below.
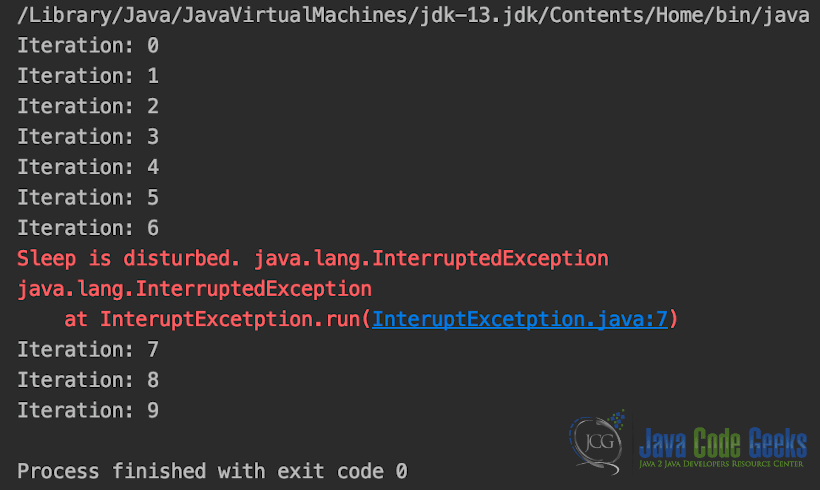
3.3 ReflectiveOperationException
Common superclass of exceptions thrown by reflective operations in core reflection. This exception class is not used directly, it is used via it subclasses. More details can be found here.
3.3.1 ClassNotFoundException
Thrown when an application tries to load in a class through its string name using:
- The
forName
method in classClass
. - The
findSystemClass
orloadClass
method in classClassLoader
.
but no definition for the class with the specified name could be found. More details can be found here.
Example of this is shown in the snippet below.
ClassNotFoundException.java
public class ClassNotFoundException {
public static void main(String[] args) {
try {
Class.forName("example.javacodegeeks.MyInvisibleClass");
} catch (java.lang.ClassNotFoundException e) {
e.printStackTrace();
}
}
}
Output of the ClassNotFoundException.java is shown in Fig. 3 below.

3.3.2 IllegalAccessException
An IllegalAccessException is thrown when an application tries to reflectively create an instance (other than an array), set or get a field, or invoke a method, but the currently executing method does not have access to the definition of the specified class, field, method or constructor. More details can be found here.
Example is shown in the code snippet below.
IllegalAccessException.java
public class IllegalAccessException {
public static void main(String[] args) throws InstantiationException, java.lang.IllegalAccessException {
Class<?> classVar = ClassWithPrivateConstructor.class;
ClassWithPrivateConstructor t = (ClassWithPrivateConstructor) classVar.newInstance();
t.sampleMethod();
}
}
class ClassWithPrivateConstructor {
private ClassWithPrivateConstructor() {}
public void sampleMethod() {
System.out.println("Method 'sampleMethod' Called");
}
}
Output of IllegalAccessException.java is shown in the Fig. 4 below.

3.3.3 InstantiationException
Thrown when an application tries to create an instance of a class using the newInstance
method in class Class
, but the specified class object cannot be instantiated. The instantiation can fail for a variety of reasons including but not limited to:
- the class object represents an abstract class, an interface, an array class, a primitive type, or
void
- the class has no nullary constructor
More details can be found here.
3.3.4 NoSuchFieldException
Signals that the class doesn’t have a field of a specified name.
Details can be found here.
Example is shown in the code snippet below.
NoSuchFieldException.java
class SampleClass {
int age;
SampleClass(int age) {
age = age;
}
}
public class NoSuchFieldException {
public static void main(String[] args) {
try {
String propertyName = "name";
SampleClass.class.getDeclaredField(propertyName);
} catch (java.lang.NoSuchFieldException e) {
e.printStackTrace();
}
}
}
Output is shown in the code Fig. 5 below.
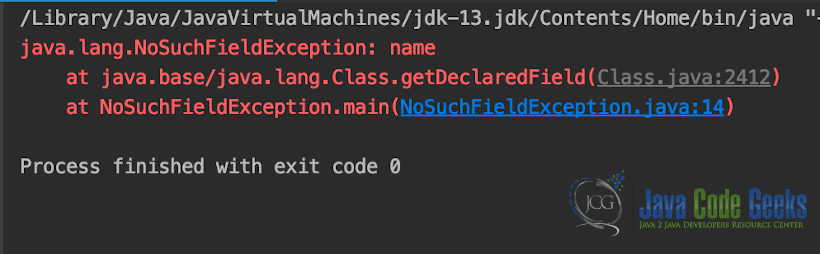
3.3.5 NoSuchMethodException
Thrown when a particular method cannot be found.
Details can be found here.
Example is shown in the code snippet below.
NoSuchMethodFoundException.java
public class NoSuchMethodFoundException {
public static void main(String[] args) {
try {
String propertyName = "getAge";
SampleClass.class.getDeclaredMethod(propertyName);
} catch (java.lang.NoSuchMethodException e) {
e.printStackTrace();
}
}
}
class SampleMethodClass {
int age;
SampleMethodClass(int age) {
age = age;
}
}
Output is shown in the Fig. 6 below.

3.4 RuntimeException
RuntimeException
is the superclass of those exceptions that can be thrown during the normal operation of the Java Virtual Machine.
RuntimeException
and its subclasses are unchecked exceptions. Unchecked exceptions do not need to be declared in a method or constructor’s throws
clause.
Further details can be found here.
3.4.1 ArithmeticException
Thrown when an exceptional arithmetic condition has occurred. For example, an integer “divide by zero” throws an instance of this class.
More details can be found here.
Example is shown in the code snippet below.
ArithemeticExceptionExample.java
public class ArithemeticExceptionExample {
public static void main(String[] args) {
try {
int a = 12 / 0;
} catch (ArithmeticException e) {
e.printStackTrace();
}
}
}
Output is shown in Fig. 7. below.

3.4.2 ArrayStoreException
Thrown to indicate that an attempt has been made to store the wrong type of object into an array of objects.
More details can be found here.
Example is shown in the code snippet below
ArrayStoreException.java
public class ArrayStoreException {
public static void main(String[] args) {
try{
Object sampleArray[] = new Integer[3];
sampleArray[0] = new String("a");
}
catch (java.lang.ArrayStoreException e){
e.printStackTrace();
}
}
}
Output of the ArrayStoreException.java is shown in Fig.8 below.
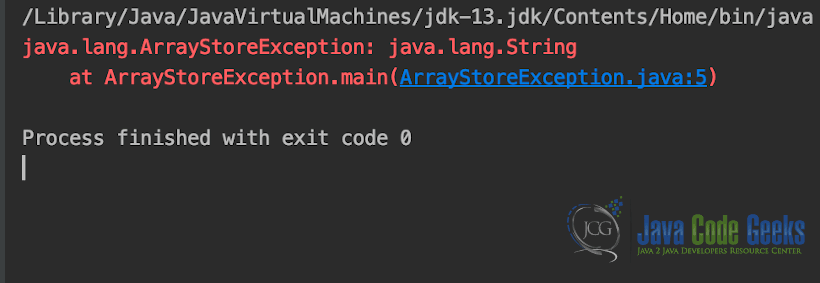
3.4.3 ClassCastException
Thrown to indicate that the code has attempted to cast an object to a subclass of which it is not an instance.
More details can be found here.
Example is shown in the code snippet below.
ClassCastException.java
public class ClassCastException {
public static void main(String[] args) {
try{
Object newObject = new Integer(0);
System.out.println((String)newObject);
}catch (java.lang.ClassCastException e){
e.printStackTrace();
}
}
}
Output of ClassCastException is shown in Fig.9 below.
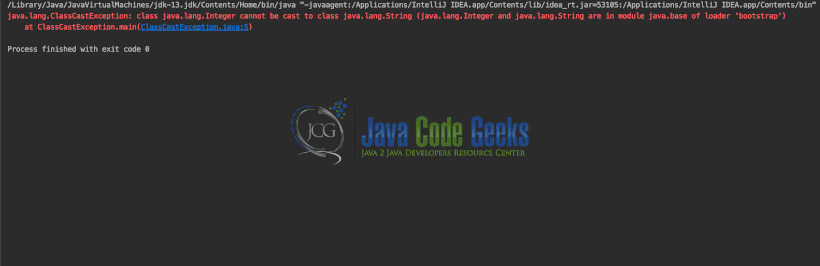
3.4.4 EnumConstantNotPresentException
Thrown when an application tries to access an enum constant by name and the enum type contains no constant with the specified name.
Further details can be found here.
3.4.5 IllegalArgumentException
Thrown to indicate that a method has been passed an illegal or inappropriate argument.
Further details can be found here.
3.4.5.1 IllegalThreadStateException
Thrown to indicate that a thread is not in an appropriate state for the requested operation. See, for example, the suspend
and resume
methods in class Thread
.
More details can be found here.
Example is shown in the code snippet below.
IllegalThreadStateException.java
public class IllegalThreadStateException extends Thread {
public static void main(String[] args) {
try {
IllegalThreadStateException d1 = new IllegalThreadStateException();
d1.start();
d1.start();
} catch (java.lang.IllegalThreadStateException e) {
e.printStackTrace();
}
}
}
Output of above code snippet is shown in Fig. 10 below.

3.4.5.2 NumberFormatException
Thrown to indicate that the application has attempted to convert a string to one of the numeric types, but that the string does not have the appropriate format.
Further details can be found here.
Example is shown in the code snippet below.
NumberFormatException.java
public class NumberFormatException {
public static void main(String args[]) {
try {
int num = Integer.parseInt("XYZ");
System.out.println(num);
} catch (java.lang.NumberFormatException e) {
e.printStackTrace();
}
}
}
Output of NumberFormatException.java is shown in Fig.11 below.

3.4.6 IllegalMonitorStateException
Thrown to indicate that a thread has attempted to wait on an object’s monitor or to notify other threads waiting on an object’s monitor without owning the specified monitor.
Further details can be found here.
Example code is shown in snippet below.
IllegalMonitorStateException.java
import java.util.concurrent.TimeUnit;
public class IllegalMonitorStateException {
public static void main(String[] args) {
try {
Utility.syncObject.wait();
} catch (InterruptedException ex) {
System.err.println("An InterruptedException was caught: " + ex.getMessage());
ex.printStackTrace();
}
}
}
class Utility {
public final static Object syncObject = new Object();
public static class HaltThread extends Thread {
@Override
public void run() {
synchronized (syncObject) {
try {
System.out.println("[HaltThread]: Waiting for another thread "
+ "to notify me...");
syncObject.wait();
System.out.println("[HaltThread]: Successfully notified!");
} catch (InterruptedException ex) {
System.err.println("[HaltThread]: An InterruptedException was caught: "
+ ex.getMessage());
ex.printStackTrace();
}
}
}
}
public static class StartingThread extends Thread {
@Override
public void run() {
synchronized (syncObject) {
try {
System.out.println("[StartingThread]: Sleeping for some time...");
TimeUnit.SECONDS.sleep(5);
System.out.println("[StartingThread]: Woke up!");
System.out.println("[StartingThread]: About to notify another thread...");
syncObject.notify();
System.out.println("[StartingThread]: Successfully notified some other thread!");
} catch (InterruptedException ex) {
System.err.println("[HaltThread]: An InterruptedException was caught: "
+ ex.getMessage());
ex.printStackTrace();
}
}
}
}
}
Output of IllegalMonitorStateException.java is shown in Fig.12 below.
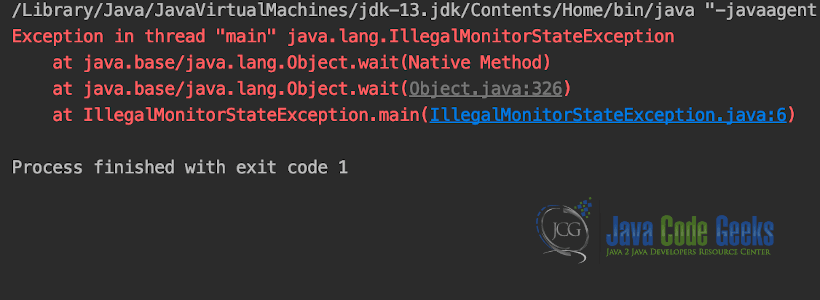
3.4.7 IllegalStateException
Signals that a method has been invoked at an illegal or inappropriate time. In other words, the Java environment or Java application is not in an appropriate state for the requested operation.
More details can be found here.
Example is shown in the code snippet below.
IllegalStateException.java
import java.util.Iterator;
import java.util.Vector;
public class IllegalStateException {
public static void main(String[] args) {
Vector<Integer> intVect = new Vector<Integer>(3);
intVect.add(1);
intVect.add(2);
intVect.add(3);
Iterator vectIter = intVect.iterator();
while (vectIter.hasNext()) {
Object obj1 = vectIter.next();
vectIter.remove();
vectIter.remove();
}
}
}
The next()
method of Iterator places the cursor on the element to return. If remove()
method is called, the element where the cursor is positioned is removed. If remove() method is called without calling next() method, which element is to be removed by the JVM because cursor will be pointing no element. At this point calling remove()
is an illegal operation.
Output is shown in the Fig.13 below.
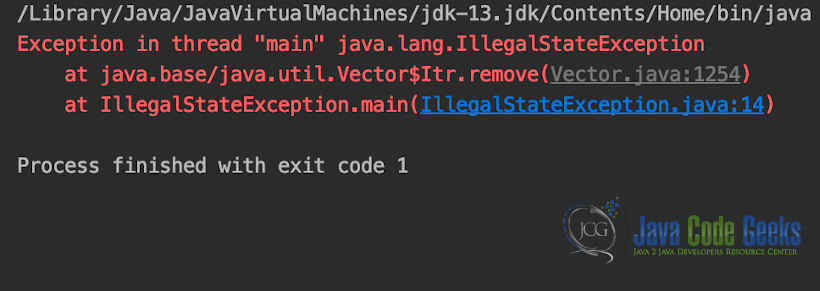
3.4.8 IndexOutOfBoundsException
Thrown to indicate that an index of some sort (such as to an array, to a string, or to a vector) is out of range.
Further details can be found here.
3.4.8.1 ArrayIndexOutOfBoundsException
Thrown to indicate that an array has been accessed with an illegal index. The index is either negative or greater than or equal to the size of the array.
for details check here.
Example is shown in code snippet below.
ArrayIndexOutOfBoundException.java
public class ArrayIndexOutOfBoundException {
public static void main(String[] args) {
int[] arr = new int[3];
try{
arr[10] = 12;
}catch (ArrayIndexOutOfBoundsException e){
e.printStackTrace();
}
}
}
Output is shown in Fig.14 below.
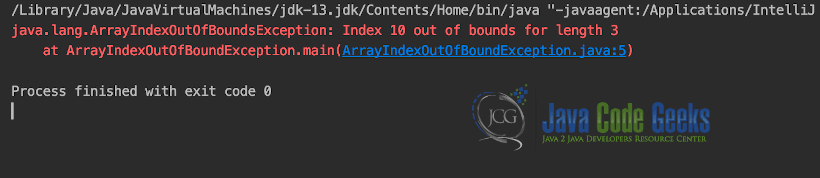
3.4.8.2 StringIndexOutOfBoundsException
Thrown by String
methods to indicate that an index is either negative or greater than the size of the string. For some methods such as the charAt method, this exception also is thrown when the index is equal to the size of the string.
For details check here.
Example is shown in the code snippet below.
StringIndexOutOfBoundsException.java
public class StringIndexOutOfBoundsException {
public static void main(String[] args) {
String sampleStr = "JavaCodeGeeks";
try{
System.out.println(sampleStr.charAt(100));
}catch (java.lang.StringIndexOutOfBoundsException e){
e.printStackTrace();
}
}
}
Output is shown in Fig.15 below.
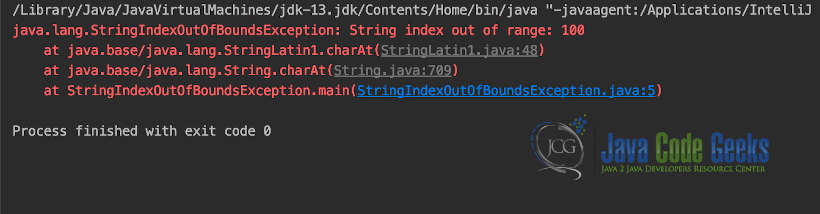
3.4.9 NegativeArraySizeException
Thrown if an application tries to create an array with negative size.
For details visit here.
Example is shown in code snippet below.
NegativeArraySizeException.java
public class NegativeArraySizeException {
public static void main(String[] args) {
try{
int[] sampleArr = new int[-1];
}catch (java.lang.NegativeArraySizeException e){
e.printStackTrace();
}
}
}
Output of the NegativeArraySizeException.java is shown in Fig. 16 below.
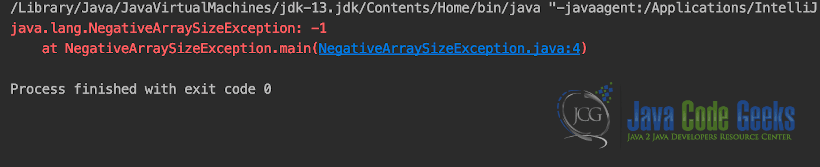
3.4.10 NullPointerException
Thrown when an application attempts to use null
in a case where an object is required. These include:
- Calling the instance method of a
null
object. - Accessing or modifying the field of a
null
object. - Taking the length of
null
as if it were an array. - Accessing or modifying the slots of
null
as if it were an array. - Throwing
null
as if it were aThrowable
value.
Applications should throw instances of this class to indicate other illegal uses of the null
object.
For further details check here.
Example is shown in the code snippet below.
NullPointerException.java
public class NullPointerException {
public static void main(String[] args) {
try{
String abc=null;
System.out.println(abc.toLowerCase());
}catch(java.lang.NullPointerException e){
e.printStackTrace();
}
}
}
Output of NullPointerException.java is shown in Fig.17 below.
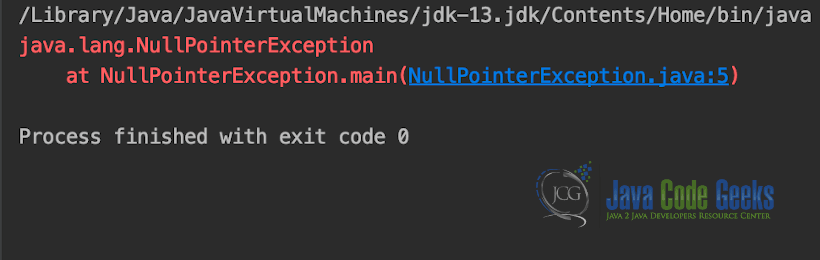
3.4.11 SecurityException
The SecurityException
indicates that a security violation has occurred and thus, the application cannot be executed. It is thrown by the security manager to indicate a security violation.
A simple example is to use a package name that is already defined in Java. Other use-case would be that if JVM determines that package name as invalid.
For further details check here.
3.4.12 TypeNotPresentException
Thrown when an application tries to access a type using a string representing the type’s name, but no definition for the type with the specified name can be found.
This exception differs from ClassNotFoundException
in that ClassNotFoundException is a checked exception, whereas this exception is unchecked.
Note that this exception may be used when undefined type variables are accessed as well as when types (e.g., classes, interfaces or annotation types) are loaded.
For further details visit here.
3.4.13 UnsupportedOperationException
Thrown to indicate that the requested operation is not supported.
This class is a member of the Java Collections Framework.
For further details check here.
Example is shown in the code snippet below.
UnsupportedOperationException.java
import java.util.Arrays;
import java.util.List;
public class UnsupportedOperationException {
public static void main(String[] args) {
String[] flowersAsArrays = { "Ageratum", "Allium", "Poppy", "Catmint" };
List<String> flowersAsArrayList = Arrays.asList(flowersAsArrays);
try{
flowersAsArrayList.add("Celosia");
} catch (java.lang.UnsupportedOperationException e){
e.printStackTrace();
}
}
}
Output of UnsupportedOperationException.java is shown in Fig.18 below.
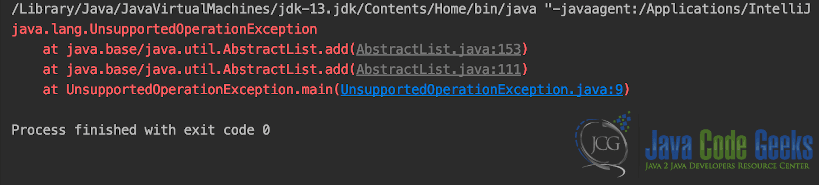
4. Summary
In summary, we have discussed all the pre-defined exceptions in java, with the relevant code examples. I hope this will give you an idea about what exception in java are and how to use the pre-defined exceptions in java.
5. Download the Source Code
You can download the full source code of this example here: Java Exceptions List Example