Spring MVC Form Handling Example
With this tutorial we shall explain create and submit a form in Spring MVC. Spring MVC provides tags that are data binding-aware and allow us to handle form elements, compining JSP and Spring Web MVC. Each tag in Spring MVC provides support for the set of attributes of its corresponding HTML tag, thus making the tags familiar and intuitive to use.
In this example we shall make use of a textbox
, a password
, a checkbox
, a dropdown box
and a hidden value
, all tags provided by Spring MVC. They will be used as properties inside a class, which is the MVC model. There is also a validator for all these fields, and a view that contains a form with all these fields to fill and submit.
You may skip project creation and jump directly to the beginning of the example below.
Our preferred development environment is Eclipse. We are using Eclipse Juno (4.2) version, along with Maven Integration plugin version 3.1.0. You can download Eclipse from here and Maven Plugin for Eclipse from here. The installation of Maven plugin for Eclipse is out of the scope of this tutorial and will not be discussed. We are also using JDK 7_u_21. Tomcat 7 is the application server used.
Let’s begin,
1. Create a new Maven project
Go to File -> Project ->Maven -> Maven Project.
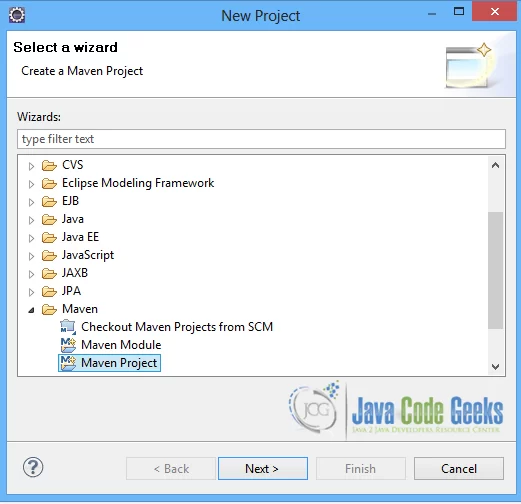
In the “Select project name and location” page of the wizard, make sure that “Create a simple project (skip archetype selection)” option is unchecked, hit “Next” to continue with default values.
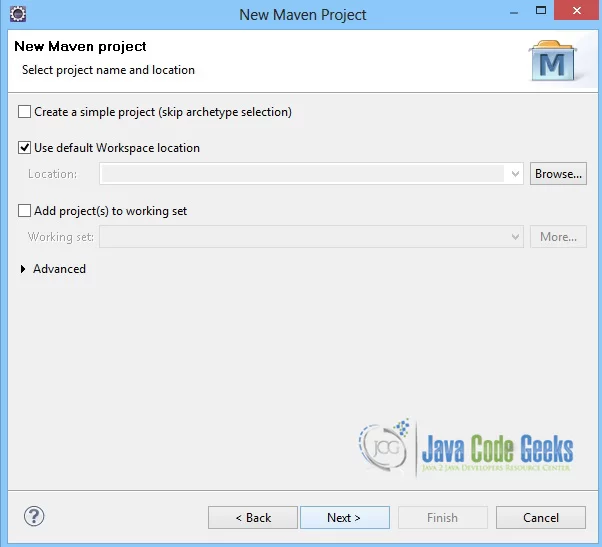
Here the maven archetype for creating a web application must be added. Click on “Add Archetype” and add the archetype. Set the “Archetype Group Id” variable to "org.apache.maven.archetypes"
, the “Archetype artifact Id” variable to "maven-archetype-webapp"
and the “Archetype Version” to "1.0"
. Click on “OK” to continue.
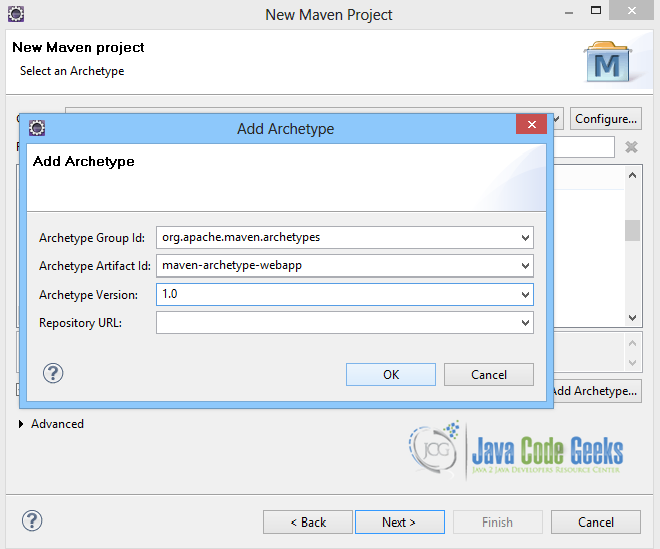
In the “Enter an artifact id” page of the wizard, you can define the name and main package of your project. Set the “Group Id” variable to "com.javacodegeeks.snippets.enterprise"
and the “Artifact Id” variable to "springexample"
. The aforementioned selections compose the main project package as "com.javacodegeeks.snippets.enterprise.springexample"
and the project name as "springexample"
. Set the “Package” variable to "war"
, so that a war file will be created to be deployed to tomcat server. Hit “Finish” to exit the wizard and to create your project.
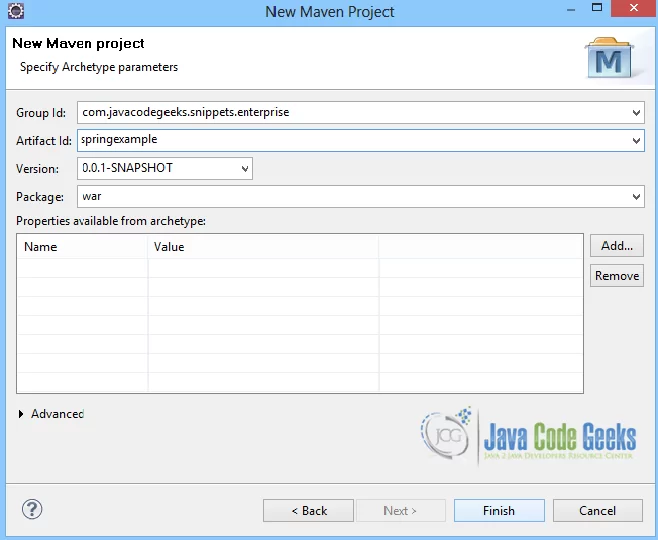
The Maven project structure is shown below:
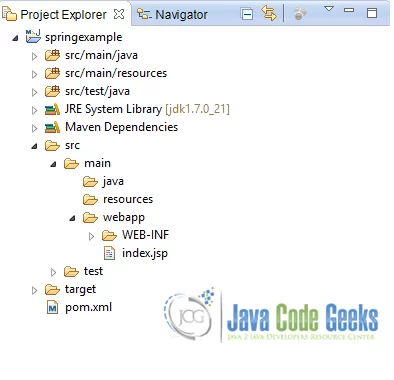
- It consists of the following folders:
- /src/main/java folder, that contains source files for the dynamic content of the application,
- /src/test/java folder contains all source files for unit tests,
- /src/main/resources folder contains configurations files,
- /target folder contains the compiled and packaged deliverables,
- /src/main/resources/webapp/WEB-INF folder contains the deployment descriptors for the Web application ,
- the pom.xml is the project object model (POM) file. The single file that contains all project related configuration.
2. Add Spring-MVC dependencies
Add the dependencies in Maven’s pom.xml
file, by editing it at the “Pom.xml” page of the POM editor. The dependency needed for MVC is the spring-webmvc
package. The javax.validation
and the hibernate-validator
packages will be also used here for validation:
pom.xml
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 | < project xmlns = "http://maven.apache.org/POM/4.0.0" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" < modelVersion >4.0.0</ modelVersion > < groupId >com.javacodegeeks.snippets.enterprise</ groupId > < artifactId >springexample</ artifactId > < packaging >war</ packaging > < version >0.0.1-SNAPSHOT</ version > < name >springexample Maven Webapp</ name > < dependencies > < dependency > < groupId >org.springframework</ groupId > < artifactId >spring-webmvc</ artifactId > < version >${spring.version}</ version > </ dependency > < dependency > < groupId >javax.servlet</ groupId > < artifactId >servlet-api</ artifactId > < version >2.5</ version > </ dependency > < dependency > < groupId >javax.validation</ groupId > < artifactId >validation-api</ artifactId > < version >1.1.0.Final</ version > </ dependency > < dependency > < groupId >org.hibernate</ groupId > < artifactId >hibernate-validator</ artifactId > < version >5.1.0.Final</ version > </ dependency > </ dependencies > < build > < finalName >springexample</ finalName > </ build > < properties > < spring.version >3.2.9.RELEASE</ spring.version > </ properties > </ project > |
3. Create the model
Form.java
is the class that will be used as the model. It has properties for all the fields that will be used in the form, which are a textbox
, a password
, a checkbox
, a dropdown box
and a hidden value
. All fields must have getters and setters to be rendered in the view.
Form.java
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 | package com.javacodegeeks.snippets.enterprise.form.model; import java.util.List; public class Form { // textbox, password, // checkbox, dropdown, hidden value private String name; private String email; private String gender; private String password; private String passwordConf; private List<String> courses; private String tutor; private String hiddenMessage; public String getName() { return name; } public void setName(String name) { this .name = name; } public String getEmail() { return email; } public void setEmail(String email) { this .email = email; } public String getGender() { return gender; } public void setGender(String gender) { this .gender = gender; } public String getPassword() { return password; } public void setPassword(String password) { this .password = password; } public String getPasswordConf() { return passwordConf; } public void setPasswordConf(String passwordConf) { this .passwordConf = passwordConf; } public List<String> getCourses() { return courses; } public void setCourses(List<String> courses) { this .courses = courses; } public String getTutor() { return tutor; } public void setTutor(String tutor) { this .tutor = tutor; } public String getHiddenMessage() { return hiddenMessage; } public void setHiddenMessage(String hiddenMessage) { this .hiddenMessage = hiddenMessage; } } |
4. Create a Validator
The validator is the class that will be used in the controller to check on the values of each field in the form. In order to create a validator class, we are making use of the API provided by Spring MVC. FormValidator.java
below implements the org.springframework.validation.Validator
, and overrides the two methods it provides.
The boolean supports(Class<?> paramClass)
method is used to check if the validator can validate instances of the paramClass
.
In the validate(Object obj, Errors errors)
method, an instance of the class is provided, and an Errors
object. The org.springframework.validation.ValidationUtils
is used here, since it offers validation API methods to check the fields of the object. All error messages are passed in the error
object. A properties
file with error messages is used here to pass various validation messages to the errors
object as shown below:
FormValidator.java
001 002 003 004 005 006 007 008 009 010 011 012 013 014 015 016 017 018 019 020 021 022 023 024 025 026 027 028 029 030 031 032 033 034 035 036 037 038 039 040 041 042 043 044 045 046 047 048 049 050 051 052 053 054 055 056 057 058 059 060 061 062 063 064 065 066 067 068 069 070 071 072 073 074 075 076 077 078 079 080 081 082 083 084 085 086 087 088 089 090 091 092 093 094 095 096 097 098 099 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 | package com.javacodegeeks.snippets.enterprise.form.validator; import java.util.List; import org.springframework.validation.Errors; import org.springframework.validation.ValidationUtils; import org.springframework.validation.Validator; import com.javacodegeeks.snippets.enterprise.form.model.Form; public class FormValidator implements Validator { private static final String EMAIL_PATTERN = "^[_A-Za-z0- 9 -\\+]+(\\.[_A-Za-z0- 9 -]+)*@[A-Za-z0- 9 -]+(\\.[A-Za-z0- 9 ]+)*(\\.[A-Za-z]{ 2 ,}) <pre wp-pre-tag- 2 = "" ></pre><p>quot;;</p><p> public boolean supports(Class<?> paramClass) {<br> return Form. class .equals(paramClass);<br> }</p><p> public void validate(Object obj, Errors errors) {<br> Form form = (Form) obj;<br> ValidationUtils.rejectIfEmptyOrWhitespace(errors, "name" , "valid.name" );<br> if (!form.getEmail().matches(EMAIL_PATTERN)) {<br> errors.rejectValue( "email" , "valid.email" );<br> }<br> ValidationUtils.rejectIfEmptyOrWhitespace(errors, "gender" , "valid.gender" );<br> ValidationUtils.rejectIfEmptyOrWhitespace(errors, "password" , "valid.password" );<br> ValidationUtils.rejectIfEmptyOrWhitespace(errors, "passwordConf" , "valid.passwordConf" );<br> if (!form.getPassword().equals(form.getPasswordConf())) {<br> errors.rejectValue( "passwordConf" , "valid.passwordConfDiff" );<br> }<br> List<String> courses = form.getCourses();<br> if (courses == null || courses.size() < 2 ) {<br> errors.rejectValue( "courses" , "valid.courses" );<br> }<br> ValidationUtils.rejectIfEmptyOrWhitespace(errors, "tutor" , "valid.tutor" );<br> }<br> }<br> The <code>validation.properties</code> file below is the file that contains all the error messages.</p><p><span style= "text-decoration: underline" ><em>validation.properties</em></span></p><pre class = "brush:xml" >valid.name= Please type your name valid.gender = Please select your gender! valid.email=Please type a correct email valid.password=Please select a password valid.passwordConf=Please confirm your password valid.passwordConfDiff=Your password is different valid.courses = Please select at least two courses! valid.tutor=Please choose your tutor! </pre><h2> 5 . Create the Controller</h2><p>The <code>Controller</code> is where the <code>DispatcherServlet</code> will delegate requests. The <code> @Controller </code> annotation indicates that the class serves the role of a Controller. The <code> @RequestMapping </code> annotation is used to map a URL to either an entire class or a particular handler method.</p><p>A <code>org.springframework.validation.Validator</code> is injected here, via the <code> @Autowired </code> annotation, also making use of the <code> @Qualifier </code> annotation to specify that the <code>FormValidator.java</code> implementation of the <code>org.springframework.validation.Validator</code> class is injected.</p><p>The <code> @InitBinder </code> annotation in <code>initBinder(WebDataBinder binder)</code> method allows us to configure web data binding directly within the controller. With <code> @InitBinder </code> we can initialize the <code>WebDataBinder</code>, that is used for data binding from web request parameters to JavaBean objects. Here, the <code>WebDataBinder</code> is where the validator is set.</p><p>The Controller consists of two basic methods, a GET method, which is <code>String initForm(Model model)</code> and a POST method, which is <code>String submitForm(Model model, @Validated Form form, BindingResult result)</code>. The first method creates and returns to the <code> "form" </code> view a new instance of the <code>Form.java</code> class . The second method also gets the <code>Model</code>, and the <code>Form</code> object created in the form. <code>Form</code> is annotated with the <code> @Validated </code> annotation, which allows the form object to be validated with the validator. <code>BindingResult</code> is where all validation errors are automatically passed, so it can be used to decide the next navigation step. If there are no errors, the validation is successful, so the method returns the String representation of the <code>successForm.jsp</code> page, and the form object is passed at the <code>Model</code>. Otherwise, the returned String is the String representation of the <code>form.jsp</code> page, which also has the error messages, as will be shown below.</p><p>The <code> private void initModelList(Model model)</code> method is used to initialize all lists that are passed to the model for the tags that use options, such as the <code>checkbox</code> and the <code>dropdown box</code>. So every time the form is rendered these lists are not null . If the lists are not initialized, then the iteration over the items of the lists leads to a <code>NullPointerException</code>.</p><p><span style= "text-decoration: underline" ><em>FormController.java</em></span></p><pre class = "brush:java" > package com.javacodegeeks.snippets.enterprise.form; import java.util.ArrayList; import java.util.List; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.beans.factory.annotation.Qualifier; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.validation.BindingResult; import org.springframework.validation.Validator; import org.springframework.validation.annotation.Validated; import org.springframework.web.bind.WebDataBinder; import org.springframework.web.bind.annotation.InitBinder; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import com.javacodegeeks.snippets.enterprise.form.model.Form; @Controller @RequestMapping ( "/form.htm" ) public class FormController { @Autowired @Qualifier ( "formValidator" ) private Validator validator; @InitBinder private void initBinder(WebDataBinder binder) { binder.setValidator(validator); } @RequestMapping (method = RequestMethod.GET) public String initForm(Model model) { Form form = new Form(); form.setHiddenMessage( "JavaCodeGeek" ); model.addAttribute( "form" , form); initModelList(model); return "form" ; } @RequestMapping (method = RequestMethod.POST) public String submitForm(Model model, @Validated Form form, BindingResult result) { model.addAttribute( "form" , form); String returnVal = "successForm" ; if (result.hasErrors()) { initModelList(model); returnVal = "form" ; } else { model.addAttribute( "form" , form); } return returnVal; } private void initModelList(Model model) { List<String> courses = new ArrayList<String>(); courses.add( "Maths" ); courses.add( "Physics" ); courses.add( "Geometry" ); courses.add( "Algebra" ); courses.add( "Painting" ); model.addAttribute( "courses" , courses); List<String> genders = new ArrayList<String>(); genders.add( "Male" ); genders.add( "Female" ); model.addAttribute( "genders" , genders); List<String> tutors = new ArrayList<String>(); tutors.add( "Mrs Smith" ); tutors.add( "Mr Johnson" ); tutors.add( "Mr Clarks" ); model.addAttribute( "tutors" , tutors); model.addAttribute( "genders" , genders); } } </pre><h2> 6 . Create the view with a form</h2><p>The view below is a simple example of how to create a form. It is a simple html view consisting of the <code>head</code> and <code>body</code> html tags.<style>.lepopup-progress- 77 div.lepopup-progress-t1>div{background-color:#e0e0e0;}.lepopup-progress- 77 div.lepopup-progress-t1>div>div{background-color:#bd4070;}.lepopup-progress- 77 div.lepopup-progress-t1>div>div{color:#ffffff;}.lepopup-progress- 77 div.lepopup-progress-t1>label{color:# 444444 ;}.lepopup-form- 77 , .lepopup-form- 77 *, .lepopup-progress- 77 {font-size:15px;color:# 444444 ;font-style:normal;text-decoration:none;text-align:left;}.lepopup-form- 77 .lepopup-element div.lepopup-input div.lepopup-signature-box span i{font-size:15px;color:# 444444 ;font-style:normal;text-decoration:none;text-align:left;}.lepopup-form- 77 .lepopup-element div.lepopup-input div.lepopup-signature-box,.lepopup-form- 77 .lepopup-element div.lepopup-input div.lepopup-multiselect,.lepopup-form- 77 .lepopup-element div.lepopup-input input[type= 'text' ],.lepopup-form- 77 .lepopup-element div.lepopup-input input[type= 'email' ],.lepopup-form- 77 .lepopup-element div.lepopup-input input[type= 'password' ],.lepopup-form- 77 .lepopup-element div.lepopup-input select,.lepopup-form- 77 .lepopup-element div.lepopup-input select option,.lepopup-form- 77 .lepopup-element div.lepopup-input textarea{font-size:15px;color:# 444444 ;font-style:normal;text-decoration:none;text-align:left;background-color:rgba( 255 , 255 , 255 , 0.7 );background-image:none;border-width:1px;border-style:solid;border-color:#cccccc;border-radius:0px;box-shadow:none;}.lepopup-form- 77 .lepopup-element div.lepopup-input ::placeholder{color:# 444444 ; opacity: 0.9 ;} .lepopup-form- 77 .lepopup-element div.lepopup-input ::-ms-input-placeholder{color:# 444444 ; opacity: 0.9 ;}.lepopup-form- 77 .lepopup-element div.lepopup-input div.lepopup-multiselect::-webkit-scrollbar-thumb{background-color:#cccccc;}.lepopup-form- 77 .lepopup-element div.lepopup-input>i.lepopup-icon-left, .lepopup-form- 77 .lepopup-element div.lepopup-input>i.lepopup-icon-right{font-size:20px;color:# 444444 ;border-radius:0px;}.lepopup-form- 77 .lepopup-element .lepopup-button,.lepopup-form- 77 .lepopup-element .lepopup-button:visited{font-size:17px;font-weight: 700 ;font-style:normal;text-decoration:none;text-align:center;background-color:rgba( 203 , 169 , 82 , 1 );background-image:linear-gradient(to bottom,rgba( 255 , 255 , 255 ,. 05 ) 0 ,rgba( 255 , 255 , 255 ,. 05 ) 50 %,rgba( 0 , 0 , 0 ,. 05 ) 51 %,rgba( 0 , 0 , 0 ,. 05 ) 100 %);border-width:0px;border-style:solid;border-color:transparent;border-radius:0px;box-shadow:none;}.lepopup-form- 77 .lepopup-element div.lepopup-input .lepopup-imageselect+label{border-width:1px;border-style:solid;border-color:#cccccc;border-radius:0px;box-shadow:none;}.lepopup-form- 77 .lepopup-element div.lepopup-input .lepopup-imageselect+label span.lepopup-imageselect-label{font-size:15px;color:# 444444 ;font-style:normal;text-decoration:none;text-align:left;}.lepopup-form- 77 .lepopup-element div.lepopup-input input[type= 'checkbox' ].lepopup-checkbox-tgl:checked+label:after{background-color:rgba( 255 , 255 , 255 , 0.7 );}.lepopup-form- 77 .lepopup-element div.lepopup-input input[type= 'checkbox' ].lepopup-checkbox-classic+label,.lepopup-form- 77 .lepopup-element div.lepopup-input input[type= 'checkbox' ].lepopup-checkbox-fa-check+label,.lepopup-form- 77 .lepopup-element div.lepopup-input input[type= 'checkbox' ].lepopup-checkbox-square+label,.lepopup-form- 77 .lepopup-element div.lepopup-input input[type= 'checkbox' ].lepopup-checkbox-tgl+label{background-color:rgba( 255 , 255 , 255 , 0.7 );border-color:#cccccc;color:# 444444 ;}.lepopup-form- 77 .lepopup-element div.lepopup-input input[type= 'checkbox' ].lepopup-checkbox-square:checked+label:after{background-color:# 444444 ;}.lepopup-form- 77 .lepopup-element div.lepopup-input input[type= 'checkbox' ].lepopup-checkbox-tgl:checked+label,.lepopup-form- 77 .lepopup-element div.lepopup-input input[type= 'checkbox' ].lepopup-checkbox-tgl+label:after{background-color:# 444444 ;}.lepopup-form- 77 .lepopup-element div.lepopup-input input[type= 'radio' ].lepopup-radio-classic+label,.lepopup-form- 77 .lepopup-element div.lepopup-input input[type= 'radio' ].lepopup-radio-fa-check+label,.lepopup-form- 77 .lepopup-element div.lepopup-input input[type= 'radio' ].lepopup-radio-dot+label{background-color:rgba( 255 , 255 , 255 , 0.7 );border-color:#cccccc;color:# 444444 ;}.lepopup-form- 77 .lepopup-element div.lepopup-input input[type= 'radio' ].lepopup-radio-dot:checked+label:after{background-color:# 444444 ;}.lepopup-form- 77 .lepopup-element div.lepopup-input div.lepopup-multiselect>input[type= 'checkbox' ]+label:hover{background-color:#bd4070;color:#ffffff;}.lepopup-form- 77 .lepopup-element div.lepopup-input div.lepopup-multiselect>input[type= 'checkbox' ]:checked+label{background-color:#a93a65;color:#ffffff;}.lepopup-form- 77 .lepopup-element input[type= 'checkbox' ].lepopup-tile+label, .lepopup-form- 77 .lepopup-element input[type= 'radio' ].lepopup-tile+label {font-size:15px;color:# 444444 ;font-style:normal;text-decoration:none;text-align:center;background-color:#ffffff;background-image:none;border-width:1px;border-style:solid;border-color:#cccccc;border-radius:0px;box-shadow:none;}.lepopup-form- 77 .lepopup-element-error{font-size:15px;color:#ffffff;font-style:normal;text-decoration:none;text-align:left;background-color:#d9534f;background-image:none;}.lepopup-form- 77 .lepopup-element- 2 {background-color:rgba( 226 , 236 , 250 , 1 );background-image:none;border-width:1px;border-style:solid;border-color:rgba( 216 , 216 , 216 , 1 );border-radius:3px;box-shadow: 1px 1px 15px -6px #d7e1eb;}.lepopup-form- 77 .lepopup-element- 3 * {font-family: 'Arial' , 'arial' ;font-size:26px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:center;}.lepopup-form- 77 .lepopup-element- 3 {font-family: 'Arial' , 'arial' ;font-size:26px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:center;background-color:transparent;background-image:none;border-width:1px;border-style:none;border-color:transparent;border-radius:0px;box-shadow:none;padding-top:0px;padding-right:0px;padding-bottom:0px;padding-left:0px;}.lepopup-form- 77 .lepopup-element- 3 .lepopup-element-html-content {min-height:36px;}.lepopup-form- 77 .lepopup-element- 4 * {font-family: 'Arial' , 'arial' ;font-size:19px;color:# 555555 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;}.lepopup-form- 77 .lepopup-element- 4 {font-family: 'Arial' , 'arial' ;font-size:19px;color:# 555555 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;background-color:transparent;background-image:none;border-width:1px;border-style:none;border-color:transparent;border-radius:0px;box-shadow:none;padding-top:0px;padding-right:0px;padding-bottom:0px;padding-left:0px;}.lepopup-form- 77 .lepopup-element- 4 .lepopup-element-html-content {min-height:58px;}.lepopup-form- 77 .lepopup-element- 5 * {font-family: 'Arial' , 'arial' ;font-size:13px;color:# 555555 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;}.lepopup-form- 77 .lepopup-element- 5 {font-family: 'Arial' , 'arial' ;font-size:13px;color:# 555555 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;background-color:transparent;background-image:none;border-width:1px;border-style:none;border-color:transparent;border-radius:0px;box-shadow:none;padding-top:0px;padding-right:0px;padding-bottom:0px;padding-left:0px;}.lepopup-form- 77 .lepopup-element- 5 .lepopup-element-html-content {min-height:70px;}.lepopup-form- 77 .lepopup-element- 6 * {font-family: 'Arial' , 'arial' ;font-size:13px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;}.lepopup-form- 77 .lepopup-element- 6 {font-family: 'Arial' , 'arial' ;font-size:13px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;background-color:transparent;background-image:none;border-width:1px;border-style:none;border-color:rgba( 216 , 216 , 216 , 1 );border-radius:0px;box-shadow:none;padding-top:0px;padding-right:0px;padding-bottom:0px;padding-left:0px;}.lepopup-form- 77 .lepopup-element- 6 .lepopup-element-html-content {min-height:auto;}.lepopup-form- 77 .lepopup-element- 0 * {font-size:15px;color:#ffffff;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;}.lepopup-form- 77 .lepopup-element- 0 {font-size:15px;color:#ffffff;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;background-color:#5cb85c;background-image:none;border-width:0px;border-style:solid;border-color:#ccc;border-radius:5px;box-shadow: 1px 1px 15px -6px # 000000 ;padding-top:40px;padding-right:40px;padding-bottom:40px;padding-left:40px;}.lepopup-form- 77 .lepopup-element- 0 .lepopup-element-html-content {min-height:160px;}</style></p><div class = "lepopup-inline" style= "margin: 0 auto;" ><div class = "lepopup-form lepopup-form-77 lepopup-form-IBMgCloR2eI43yO1 lepopup-form-icon-inside lepopup-form-position-middle-left" data-session= "0" data-id= "IBMgCloR2eI43yO1" data-form-id= "77" data-slug= "7POIYxRf1FUtPpmL" data-title= "Spring Framework Cookbook Inline" data-page= "1" data-xd= "on" data-width= "820" data-height= "356" data-position= "middle-left" data-esc= "off" data-enter= "off" data-disable-scrollbar= "off" style= "display:none;width:820px;height:356px;" onclick= "event.stopPropagation();" ><div class = "lepopup-form-inner" style= "width:820px;height:356px;" ><div class = "lepopup-element lepopup-element-2 lepopup-element-rectangle" data-type= "rectangle" data-top= "0" data-left= "0" data-animation-in= "fadeIn" data-animation-out= "fadeOut" style= "animation-duration:0ms;animation-delay:0ms;z-index:501;top:0px;left:0px;width:820px;height:355px;" ></div><div class = "lepopup-element lepopup-element-3 lepopup-element-html" data-type= "html" data-top= "10" data-left= "0" data-animation-in= "bounceInDown" data-animation-out= "fadeOut" style= "animation-duration:0ms;animation-delay:0ms;z-index:502;top:10px;left:0px;width:800px;height:36px;" ><div class = "lepopup-element-html-content" >Want to master Spring Framework ?</div></div><div class = "lepopup-element lepopup-element-4 lepopup-element-html" data-type= "html" data-top= "53" data-left= "260" data-animation-in= "bounceInDown" data-animation-out= "fadeOut" style= "animation-duration:0ms;animation-delay:0ms;z-index:503;top:53px;left:260px;width:538px;height:58px;" ><div class = "lepopup-element-html-content" >Subscribe to our newsletter and download the <span style= "color: #CAB43D; text-shadow: 1px 1px #835D5D;" >Spring</span> Framework Cookbook <span style= "text-decoration: underline;" >right now!</span></div></div><div class = "lepopup-element lepopup-element-5 lepopup-element-html" data-type= "html" data-top= "135" data-left= "260" data-animation-in= "bounceInDown" data-animation-out= "fadeOut" style= "animation-duration:0ms;animation-delay:0ms;z-index:504;top:135px;left:260px;width:538px;height:70px;" ><div class = "lepopup-element-html-content" >In order to help you master the leading and innovative Java framework, we have compiled a kick-ass guide with all its major features and use cases! Besides studying them online you may download the eBook in PDF format!</div></div><div class = "lepopup-element lepopup-element-6 lepopup-element-html" data-type= "html" data-top= "30" data-left= "7" data-animation-in= "fadeIn" data-animation-out= "fadeOut" style= "animation-duration:0ms;animation-delay:0ms;z-index:505;top:30px;left:7px;width:auto;height:auto;" ><div class = "lepopup-element-html-content" ><img data-lazyloaded= "1" src= "data:image/svg+xml;base64,PHN2ZyB4bWxucz0iaHR0cDovL3d3dy53My5vcmcvMjAwMC9zdmciIHdpZHRoPSIyNTAiIGhlaWdodD0iMzIzIiB2aWV3Qm94PSIwIDAgMjUwIDMyMyI+PHJlY3Qgd2lkdGg9IjEwMCUiIGhlaWdodD0iMTAwJSIgZmlsbD0idHJhbnNwYXJlbnQiLz48L3N2Zz4=" decoding= "async" data-src= "/wp-content/uploads/2017/01/Spring-Framework-Cookbook_book.png.webp" alt= "" width= "250" height= "323" ><noscript><img decoding= "async" src= "/wp-content/uploads/2017/01/Spring-Framework-Cookbook_book.png.webp" alt= "" width= "250" height= "323" /></noscript></div></div><div class = "lepopup-element lepopup-element-8" data-type= "button" data-top= "245" data-left= "375" data-animation-in= "fadeIn" data-animation-out= "fadeOut" style= "animation-duration:1000ms;animation-delay:0ms;z-index:508;top:245px;left:375px;width:160px;height:40px;" ><a class = "lepopup-button lepopup-button-zoom-out " href= "#" onclick= "return lepopup_submit(this);" data-label= "Download NOW!" data-loading= "Sending..." ><span>Download NOW!</span></a></div></div></div><div class = "lepopup-form lepopup-form-77 lepopup-form-IBMgCloR2eI43yO1 lepopup-form-icon-inside lepopup-form-position-middle-left" data-session= "0" data-id= "IBMgCloR2eI43yO1" data-form-id= "77" data-slug= "7POIYxRf1FUtPpmL" data-title= "Spring Framework Cookbook Inline" data-page= "confirmation" data-xd= "on" data-width= "420" data-height= "320" data-position= "middle-left" data-esc= "off" data-enter= "off" data-disable-scrollbar= "off" style= "display:none;width:420px;height:320px;" onclick= "event.stopPropagation();" ><div class = "lepopup-form-inner" style= "width:420px;height:320px;" ><div class = "lepopup-element lepopup-element-0 lepopup-element-html" data-type= "html" data-top= "80" data-left= "70" data-animation-in= "bounceInDown" data-animation-out= "fadeOutUp" style= "animation-duration:1000ms;animation-delay:0ms;z-index:500;top:80px;left:70px;width:280px;height:160px;" ><div class = "lepopup-element-html-content" ><h4 style= "text-align: center; font-size: 18px; font-weight: bold;" >Thank you!</h4><p style= "text-align: center;" >We will contact you soon.</p></div></div></div></div><input type= "hidden" id= "lepopup-logic-IBMgCloR2eI43yO1" value= "[]" ></div><p></p><p>In order to create a form in Spring MVC, we make use of the <code>form:form</code> tag. Its <code>method</code> property is set to POST, and the <code>commandName</code> property is set to the name of the backing bean that is binded to the Model, which is the <code>Form.java</code> class .</p><p>The <code>form:input</code> tag is used to create the textbox, with its <code>path</code> property set to the field binded to it. The <code>form:password</code> tag is used to create the password field. The <code>form:checkboxes</code> tag has another property to configure, appart from the <code>path</code> property. It also provides the <code>items</code> property, where the list of the items to be displayed is set.</p><p>In order to create a <code>hidden value</code>, we are using the simple <code>input</code> tag, with <code>name</code> property set to the <code>hiddenMessage</code>, which is the field bound to it. Its type parameter is set to <code>hidden</code>, so this component is not visible in the view. It also has a <code>value</code> parameter set to a String message.</p><p>The <code>form:errors</code> tag defines where the error message of the specified field will be displayed in the view. Finally, the <code>input</code> tag, with <code>type</code> property set to <code>submit</code> is used for the submit button.</p><p><span style= "text-decoration: underline" ><em>form.jsp</em></span></p><pre class = "brush:xml" ><%@ taglib prefix= "form" uri= "http://www.springframework.org/tags/form" %> <html> <head> <title>Spring MVC form submission</title> </head> <body> <h2>Fill your form!</h2> <form:form method= "POST" commandName= "form" > <table> <tr> <td>Enter your name:</td> <td><form:input path= "name" /></td> <td><form:errors path= "name" cssStyle= "color: #ff0000;" /></td> </tr> <tr> <td>Enter your mail:</td> <td><form:input path= "email" /></td> <td><form:errors path= "email" cssStyle= "color: #ff0000;" /></td> </tr> <tr> <td>Enter your gender</td> <td><form:checkboxes path= "gender" items= "${genders}" /></td> <td><form:errors path= "gender" cssStyle= "color: #ff0000;" /></td> </tr> <tr> <td>Enter a password:</td> <td><form:password path= "password" showPassword= "true" /></td> <td><form:errors path= "password" cssStyle= "color: #ff0000;" /></td> <tr> <td>Confirm your password:</td> <td><form:password path= "passwordConf" showPassword= "true" /></td> <td><form:errors path= "passwordConf" cssStyle= "color: #ff0000;" /></td> </tr> <tr> <td>Choose the courses you like:</td> <td><form:checkboxes path= "courses" items= "${courses}" /></td> <td><form:errors path= "courses" cssStyle= "color: #ff0000;" /></td> </tr> <tr> <td>Please select your tutor:</td> <td><form:select path= "tutor" > <form:option value= "" label= "...." /> <form:options items= "${tutors}" /> </form:select> </td> <td><form:errors path= "tutor" cssStyle= "color: #ff0000;" /></td> </tr> <tr> <form:hidden path= "hiddenMessage" /> </tr> <tr> <td><input type= "submit" name= "submit" value= "Submit" ></td> </tr> <tr> </table> </form:form> </body> </html></pre><p>Below is the page that will be rendered when the validation of the form succeeds:</p><p><span style= "text-decoration: underline" ><em>successForm.jsp</em></span></p><pre class = "brush:xml" ><%@ taglib prefix= "c" uri= "http://java.sun.com/jsp/jstl/core" %> <html> <head> <title>Spring MVC form submission</title> </head> <body> Dear ${form.name}, <br> Your mail is ${form.email} <br> You chose the courses below: <br> <c:forEach var= "course" items= "${form.courses}" > <c:out value= "${course}" /><br> </c:forEach> <br> ${form.tutor} will be your tutor! <br> Your hidden nickname is ${form.hiddenMessage} </body> </html> </pre><h2> 7 . Configure the application</h2><p>The files that we must configure in the application are the <code>web.xml</code> file and the <code>mvc-dispatcher-servlet.xml</code> file.</p><p>The <code>web.xml</code> file is the file that defines everything about the application that a server needs to know. It is placed in the <code>/WEB-INF/</code> directory of the application. The <code><servlet></code> element declares the <code>DispatcherServlet</code>. When the <code>DispatcherServlet</code> is initialized, the framework will try to load the application context from a file named <code>[servlet-name]-servlet.xml</code> located in <code>/WEB-INF/</code> directory. So, we have created the <code>mvc-dispatcher-servlet.xml</code> file, that will be explained below. The <code><servlet-mapping></code> element of <code>web.xml</code> file specifies what URLs will be handled by the <code>DispatcherServlet</code>.</p><p><span style= "text-decoration: underline" ><em>web.xml</em></span></p><pre class = "brush:xml" ><?xml version= "1.0" encoding= "UTF-8" ?> <web-app xmlns:xsi= "http://www.w3.org/2001/XMLSchema-instance" xmlns= "http://java.sun.com/xml/ns/javaee" xmlns:web= "http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation= "http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id= "WebApp_ID" version= "3.0" > <display-name>Archetype Created Web Application</display-name> <servlet> <servlet-name>mvc-dispatcher</servlet-name> <servlet- class > org.springframework.web.servlet.DispatcherServlet </servlet- class > <load-on-startup> 1 </load-on-startup> </servlet> <servlet-mapping> <servlet-name>mvc-dispatcher</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping> </web-app> </pre><p> <br> The <code>mvc-dispatcher-servlet.xml</code> file is also placed in <code>WebContent/WEB-INF</code> directory. The <code>org.springframework.web.servlet.view.InternalResourceViewResolver</code> bean is used as internal resource views resolver, meaning that it will find the <code>jsp</code> and <code>html</code> files in the <code>WebContent/WEB-INF/</code> folder. We can also set properties such as <code>prefix</code> or <code>suffix</code> to the view name to generate the final view page URL. This is the file where all beans created, such as Controllers are placed and defined.</p><p>The <code><context:component-scan></code> tag is used, so that the Spring container will search for all annotated classes under the <code>com.javacodegeeks.snippets.enterprise</code> package . The <code><mvc:annotation-driven></code> tag is used, so that the container searches for annotated classes, to resolve MVC. The <code>FormValidator.java</code> class is also defined here as a bean, with an id.</p><p>Finally, the <code>ResourceBundleMessageSource</code> is used, to provide access to resource bundles using specified basenames. Its <code>basename</code> property is set to <code>validation</code>, thus pointing to the properties file that holds the validation messages.</p><p><span style= "text-decoration: underline" ><em>mvc-dispatcher-servlet.xml</em></span></p><pre class = "brush:xml" ><beans xmlns= "http://www.springframework.org/schema/beans" xmlns:context= "http://www.springframework.org/schema/context" xmlns:mvc= "http://www.springframework.org/schema/mvc" xmlns:xsi= "http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation= " http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.0.xsd" > <context:component-scan base- package = "com.javacodegeeks.snippets.enterprise" /> <mvc:annotation-driven /> <bean id= "messageSource" class = "org.springframework.context.support.ResourceBundleMessageSource" > <property name= "basename" value= "validation" /> </bean> <bean id= "formValidator" class = "com.javacodegeeks.snippets.enterprise.form.validator.FormValidator" /> <bean class = "org.springframework.web.servlet.view.InternalResourceViewResolver" > <property name= "prefix" > <value>/WEB-INF/</value> </property> <property name= "suffix" > <value>.jsp</value> </property> </bean> </beans> </pre><h2> 8 . Run the application</h2><p>Now, let's run the application. We first build the project with Maven. All we have to do is right click on the project and select -> <strong>Run As: Maven build</strong>. The goal must be set to package . The <code>.war</code> file produced must be placed in <code>webapps</code> folder of tomcat. Then, we can start the server.</p><p>Hit on:</p><p><code>http: //localhost:8080/springexample/form.htm</code></p><p></p><figure id="attachment_12489" aria-describedby="caption-attachment-12489" style="width: 660px" class="wp-caption aligncenter"><a href="https://examples.javacodegeeks.com/wp-content/uploads/2014/07/f1.png"><img data-lazyloaded="1" src="data:image/svg+xml;base64,PHN2ZyB4bWxucz0iaHR0cDovL3d3dy53My5vcmcvMjAwMC9zdmciIHdpZHRoPSI2NjAiIGhlaWdodD0iNDI0IiB2aWV3Qm94PSIwIDAgNjYwIDQyNCI+PHJlY3Qgd2lkdGg9IjEwMCUiIGhlaWdodD0iMTAwJSIgZmlsbD0idHJhbnNwYXJlbnQiLz48L3N2Zz4=" decoding="async" data-src="http://examples.javacodegeeks.com/wp-content/uploads/2014/07/f1.png.webp" alt="initial form" width="660" height="424" class="size-full wp-image-12489" data-srcset="https://examples.javacodegeeks.com/wp-content/uploads/2014/07/f1.png.webp 660w, https://examples.javacodegeeks.com/wp-content/uploads/2014/07/f1-300x192.png.webp 300w" data-sizes="(max-width: 660px) 100vw, 660px"><noscript><img decoding="async" src="http://examples.javacodegeeks.com/wp-content/uploads/2014/07/f1.png.webp" alt="initial form" width="660" height="424" class="size-full wp-image-12489" srcset="https://examples.javacodegeeks.com/wp-content/uploads/2014/07/f1.png.webp 660w, https://examples.javacodegeeks.com/wp-content/uploads/2014/07/f1-300x192.png.webp 300w" sizes="(max-width: 660px) 100vw, 660px" /></noscript></a><figcaption id="caption-attachment-12489" class="wp-caption-text">initial form</figcaption></figure><p></p><p>So, here is our form. Press the submit button before having entered any values:</p><p></p><figure id="attachment_12490" aria-describedby="caption-attachment-12490" style="width: 778px" class="wp-caption aligncenter"><a href="https://examples.javacodegeeks.com/wp-content/uploads/2014/07/f2.png"><img data-lazyloaded="1" src="data:image/svg+xml;base64,PHN2ZyB4bWxucz0iaHR0cDovL3d3dy53My5vcmcvMjAwMC9zdmciIHdpZHRoPSI3NzgiIGhlaWdodD0iMzgwIiB2aWV3Qm94PSIwIDAgNzc4IDM4MCI+PHJlY3Qgd2lkdGg9IjEwMCUiIGhlaWdodD0iMTAwJSIgZmlsbD0idHJhbnNwYXJlbnQiLz48L3N2Zz4=" decoding="async" data-src="http://examples.javacodegeeks.com/wp-content/uploads/2014/07/f2.png.webp" alt="validation error messages" width="778" height="380" class="size-full wp-image-12490" data-srcset="https://examples.javacodegeeks.com/wp-content/uploads/2014/07/f2.png.webp 778w, https://examples.javacodegeeks.com/wp-content/uploads/2014/07/f2-300x146.png.webp 300w" data-sizes="(max-width: 778px) 100vw, 778px"><noscript><img decoding="async" src="http://examples.javacodegeeks.com/wp-content/uploads/2014/07/f2.png.webp" alt="validation error messages" width="778" height="380" class="size-full wp-image-12490" srcset="https://examples.javacodegeeks.com/wp-content/uploads/2014/07/f2.png.webp 778w, https://examples.javacodegeeks.com/wp-content/uploads/2014/07/f2-300x146.png.webp 300w" sizes="(max-width: 778px) 100vw, 778px" /></noscript></a><figcaption id="caption-attachment-12490" class="wp-caption-text">validation error messages</figcaption></figure><p></p><p>As a result all validation messages are rendered. Now, press different passwords and a wrong email and press the submit button again:</p><p></p><figure id="attachment_12491" aria-describedby="caption-attachment-12491" style="width: 787px" class="wp-caption aligncenter"><a href="https://examples.javacodegeeks.com/wp-content/uploads/2014/07/f3.png"><img data-lazyloaded="1" src="data:image/svg+xml;base64,PHN2ZyB4bWxucz0iaHR0cDovL3d3dy53My5vcmcvMjAwMC9zdmciIHdpZHRoPSI3ODciIGhlaWdodD0iMzg2IiB2aWV3Qm94PSIwIDAgNzg3IDM4NiI+PHJlY3Qgd2lkdGg9IjEwMCUiIGhlaWdodD0iMTAwJSIgZmlsbD0idHJhbnNwYXJlbnQiLz48L3N2Zz4=" decoding="async" data-src="http://examples.javacodegeeks.com/wp-content/uploads/2014/07/f3.png.webp" alt="more validation error messages" width="787" height="386" class="size-full wp-image-12491" data-srcset="https://examples.javacodegeeks.com/wp-content/uploads/2014/07/f3.png.webp 787w, https://examples.javacodegeeks.com/wp-content/uploads/2014/07/f3-300x147.png.webp 300w" data-sizes="(max-width: 787px) 100vw, 787px"><noscript><img decoding="async" src="http://examples.javacodegeeks.com/wp-content/uploads/2014/07/f3.png.webp" alt="more validation error messages" width="787" height="386" class="size-full wp-image-12491" srcset="https://examples.javacodegeeks.com/wp-content/uploads/2014/07/f3.png.webp 787w, https://examples.javacodegeeks.com/wp-content/uploads/2014/07/f3-300x147.png.webp 300w" sizes="(max-width: 787px) 100vw, 787px" /></noscript></a><figcaption id="caption-attachment-12491" class="wp-caption-text">more validation error messages</figcaption></figure><p></p><p>Again, there are validation messages. Now complete your form correctly and press on submit button:</p><p></p><figure id="attachment_12492" aria-describedby="caption-attachment-12492" style="width: 644px" class="wp-caption aligncenter"><a href="https://examples.javacodegeeks.com/wp-content/uploads/2014/07/f4.png"><img data-lazyloaded="1" src="data:image/svg+xml;base64,PHN2ZyB4bWxucz0iaHR0cDovL3d3dy53My5vcmcvMjAwMC9zdmciIHdpZHRoPSI2NDQiIGhlaWdodD0iMzgwIiB2aWV3Qm94PSIwIDAgNjQ0IDM4MCI+PHJlY3Qgd2lkdGg9IjEwMCUiIGhlaWdodD0iMTAwJSIgZmlsbD0idHJhbnNwYXJlbnQiLz48L3N2Zz4=" decoding="async" data-src="http://examples.javacodegeeks.com/wp-content/uploads/2014/07/f4.png.webp" alt="form submitted" width="644" height="380" class="size-full wp-image-12492" data-srcset="https://examples.javacodegeeks.com/wp-content/uploads/2014/07/f4.png.webp 644w, https://examples.javacodegeeks.com/wp-content/uploads/2014/07/f4-300x177.png.webp 300w" data-sizes="(max-width: 644px) 100vw, 644px"><noscript><img decoding="async" src="http://examples.javacodegeeks.com/wp-content/uploads/2014/07/f4.png.webp" alt="form submitted" width="644" height="380" class="size-full wp-image-12492" srcset="https://examples.javacodegeeks.com/wp-content/uploads/2014/07/f4.png.webp 644w, https://examples.javacodegeeks.com/wp-content/uploads/2014/07/f4-300x177.png.webp 300w" sizes="(max-width: 644px) 100vw, 644px" /></noscript></a><figcaption id="caption-attachment-12492" class="wp-caption-text">form submitted</figcaption></figure><p></p><p>There you go, your form is correctly submitted!</p><h2>9. Download the Eclipse Project</h2><p>This was an example of how handle a form in Spring MVC.</p><div class="download"><strong>Download</strong><br> You can download the full source code of this example here:<a href= "https://examples.javacodegeeks.com/wp-content/uploads/2014/07/SpringMVCFormHandling.zip" ><b>SpringMVCFormHandling</b></a></div><style>.lepopup-progress- 149 div.lepopup-progress-t1>div{background-color:#e0e0e0;}.lepopup-progress- 149 div.lepopup-progress-t1>div>div{background-color:#bd4070;}.lepopup-progress- 149 div.lepopup-progress-t1>div>div{color:#ffffff;}.lepopup-progress- 149 div.lepopup-progress-t1>label{color:# 444444 ;}.lepopup-form- 149 , .lepopup-form- 149 *, .lepopup-progress- 149 {font-size:15px;color:# 444444 ;font-style:normal;text-decoration:none;text-align:left;}.lepopup-form- 149 .lepopup-element div.lepopup-input div.lepopup-signature-box span i{font-family: 'Arial' , 'arial' ;font-size:13px;color:# 555555 ;font-style:normal;text-decoration:none;text-align:left;}.lepopup-form- 149 .lepopup-element div.lepopup-input div.lepopup-signature-box,.lepopup-form- 149 .lepopup-element div.lepopup-input div.lepopup-multiselect,.lepopup-form- 149 .lepopup-element div.lepopup-input input[type= 'text' ],.lepopup-form- 149 .lepopup-element div.lepopup-input input[type= 'email' ],.lepopup-form- 149 .lepopup-element div.lepopup-input input[type= 'password' ],.lepopup-form- 149 .lepopup-element div.lepopup-input select,.lepopup-form- 149 .lepopup-element div.lepopup-input select option,.lepopup-form- 149 .lepopup-element div.lepopup-input textarea{font-family: 'Arial' , 'arial' ;font-size:13px;color:# 555555 ;font-style:normal;text-decoration:none;text-align:left;background-color:rgba( 255 , 255 , 255 , 0.7 );background-image:none;border-width:1px;border-style:solid;border-color:#cccccc;border-radius:0px;box-shadow: inset 0px 0px 15px -7px # 000000 ;}.lepopup-form- 149 .lepopup-element div.lepopup-input ::placeholder{color:# 555555 ; opacity: 0.9 ;} .lepopup-form- 149 .lepopup-element div.lepopup-input ::-ms-input-placeholder{color:# 555555 ; opacity: 0.9 ;}.lepopup-form- 149 .lepopup-element div.lepopup-input div.lepopup-multiselect::-webkit-scrollbar-thumb{background-color:#cccccc;}.lepopup-form- 149 .lepopup-element div.lepopup-input>i.lepopup-icon-left, .lepopup-form- 149 .lepopup-element div.lepopup-input>i.lepopup-icon-right{font-size:20px;color:# 444444 ;border-radius:0px;}.lepopup-form- 149 .lepopup-element .lepopup-button,.lepopup-form- 149 .lepopup-element .lepopup-button:visited{font-family: 'Arial' , 'arial' ;font-size:13px;color:#ffffff;font-weight: 700 ;font-style:normal;text-decoration:none;text-align:center;background-color:# 326693 ;background-image:none;border-width:1px;border-style:solid;border-color:# 326693 ;border-radius:0px;box-shadow:none;}.lepopup-form- 149 .lepopup-element div.lepopup-input .lepopup-imageselect+label{border-width:1px;border-style:solid;border-color:#cccccc;border-radius:0px;box-shadow:none;}.lepopup-form- 149 .lepopup-element div.lepopup-input .lepopup-imageselect+label span.lepopup-imageselect-label{font-size:15px;color:# 444444 ;font-style:normal;text-decoration:none;text-align:left;}.lepopup-form- 149 .lepopup-element div.lepopup-input input[type= 'checkbox' ].lepopup-checkbox-tgl:checked+label:after{background-color:rgba( 255 , 255 , 255 , 0.7 );}.lepopup-form- 149 .lepopup-element div.lepopup-input input[type= 'checkbox' ].lepopup-checkbox-classic+label,.lepopup-form- 149 .lepopup-element div.lepopup-input input[type= 'checkbox' ].lepopup-checkbox-fa-check+label,.lepopup-form- 149 .lepopup-element div.lepopup-input input[type= 'checkbox' ].lepopup-checkbox-square+label,.lepopup-form- 149 .lepopup-element div.lepopup-input input[type= 'checkbox' ].lepopup-checkbox-tgl+label{background-color:rgba( 255 , 255 , 255 , 0.7 );border-color:#cccccc;color:# 555555 ;}.lepopup-form- 149 .lepopup-element div.lepopup-input input[type= 'checkbox' ].lepopup-checkbox-square:checked+label:after{background-color:# 555555 ;}.lepopup-form- 149 .lepopup-element div.lepopup-input input[type= 'checkbox' ].lepopup-checkbox-tgl:checked+label,.lepopup-form- 149 .lepopup-element div.lepopup-input input[type= 'checkbox' ].lepopup-checkbox-tgl+label:after{background-color:# 555555 ;}.lepopup-form- 149 .lepopup-element div.lepopup-input input[type= 'radio' ].lepopup-radio-classic+label,.lepopup-form- 149 .lepopup-element div.lepopup-input input[type= 'radio' ].lepopup-radio-fa-check+label,.lepopup-form- 149 .lepopup-element div.lepopup-input input[type= 'radio' ].lepopup-radio-dot+label{background-color:rgba( 255 , 255 , 255 , 0.7 );border-color:#cccccc;color:# 555555 ;}.lepopup-form- 149 .lepopup-element div.lepopup-input input[type= 'radio' ].lepopup-radio-dot:checked+label:after{background-color:# 555555 ;}.lepopup-form- 149 .lepopup-element div.lepopup-input div.lepopup-multiselect>input[type= 'checkbox' ]+label:hover{background-color:#bd4070;color:#ffffff;}.lepopup-form- 149 .lepopup-element div.lepopup-input div.lepopup-multiselect>input[type= 'checkbox' ]:checked+label{background-color:#a93a65;color:#ffffff;}.lepopup-form- 149 .lepopup-element input[type= 'checkbox' ].lepopup-tile+label, .lepopup-form- 149 .lepopup-element input[type= 'radio' ].lepopup-tile+label {font-size:15px;color:# 444444 ;font-style:normal;text-decoration:none;text-align:center;background-color:#ffffff;background-image:none;border-width:1px;border-style:solid;border-color:#cccccc;border-radius:0px;box-shadow:none;}.lepopup-form- 149 .lepopup-element-error{font-size:15px;color:#ffffff;font-style:normal;text-decoration:none;text-align:left;background-color:#d9534f;background-image:none;}.lepopup-form- 149 .lepopup-element- 2 {background-color:rgba( 226 , 236 , 250 , 1 );background-image:none;border-width:1px;border-style:solid;border-color:rgba( 216 , 216 , 216 , 1 );border-radius:3px;box-shadow: 1px 1px 15px -6px #d7e1eb;}.lepopup-form- 149 .lepopup-element- 3 * {font-family: 'Arial' , 'arial' ;font-size:26px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;}.lepopup-form- 149 .lepopup-element- 3 {font-family: 'Arial' , 'arial' ;font-size:26px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;background-color:transparent;background-image:none;border-width:1px;border-style:none;border-color:transparent;border-radius:0px;box-shadow:none;padding-top:0px;padding-right:0px;padding-bottom:0px;padding-left:0px;}.lepopup-form- 149 .lepopup-element- 3 .lepopup-element-html-content {min-height:73px;}.lepopup-form- 149 .lepopup-element- 4 * {font-family: 'Arial' , 'arial' ;font-size:19px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;}.lepopup-form- 149 .lepopup-element- 4 {font-family: 'Arial' , 'arial' ;font-size:19px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;background-color:transparent;background-image:none;border-width:1px;border-style:none;border-color:transparent;border-radius:0px;box-shadow:none;padding-top:0px;padding-right:0px;padding-bottom:0px;padding-left:0px;}.lepopup-form- 149 .lepopup-element- 4 .lepopup-element-html-content {min-height:23px;}.lepopup-form- 149 .lepopup-element- 5 * {font-family: 'Arial' , 'arial' ;font-size:15px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;}.lepopup-form- 149 .lepopup-element- 5 {font-family: 'Arial' , 'arial' ;font-size:15px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;background-color:transparent;background-image:none;border-width:1px;border-style:none;border-color:transparent;border-radius:0px;box-shadow:none;padding-top:0px;padding-right:0px;padding-bottom:0px;padding-left:0px;}.lepopup-form- 149 .lepopup-element- 5 .lepopup-element-html-content {min-height:24px;}.lepopup-form- 149 .lepopup-element- 6 * {font-family: 'Arial' , 'arial' ;font-size:15px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;}.lepopup-form- 149 .lepopup-element- 6 {font-family: 'Arial' , 'arial' ;font-size:15px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;background-color:transparent;background-image:none;border-width:1px;border-style:none;border-color:transparent;border-radius:0px;box-shadow:none;padding-top:0px;padding-right:0px;padding-bottom:0px;padding-left:0px;}.lepopup-form- 149 .lepopup-element- 6 .lepopup-element-html-content {min-height:18px;}.lepopup-form- 149 .lepopup-element- 7 * {font-family: 'Arial' , 'arial' ;font-size:15px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;}.lepopup-form- 149 .lepopup-element- 7 {font-family: 'Arial' , 'arial' ;font-size:15px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;background-color:transparent;background-image:none;border-width:1px;border-style:none;border-color:transparent;border-radius:0px;box-shadow:none;padding-top:0px;padding-right:0px;padding-bottom:0px;padding-left:0px;}.lepopup-form- 149 .lepopup-element- 7 .lepopup-element-html-content {min-height:18px;}.lepopup-form- 149 .lepopup-element- 8 * {font-family: 'Arial' , 'arial' ;font-size:15px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;}.lepopup-form- 149 .lepopup-element- 8 {font-family: 'Arial' , 'arial' ;font-size:15px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;background-color:transparent;background-image:none;border-width:1px;border-style:none;border-color:transparent;border-radius:0px;box-shadow:none;padding-top:0px;padding-right:0px;padding-bottom:0px;padding-left:0px;}.lepopup-form- 149 .lepopup-element- 8 .lepopup-element-html-content {min-height:18px;}.lepopup-form- 149 .lepopup-element- 9 * {font-family: 'Arial' , 'arial' ;font-size:15px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;}.lepopup-form- 149 .lepopup-element- 9 {font-family: 'Arial' , 'arial' ;font-size:15px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;background-color:transparent;background-image:none;border-width:1px;border-style:none;border-color:transparent;border-radius:0px;box-shadow:none;padding-top:0px;padding-right:0px;padding-bottom:0px;padding-left:0px;}.lepopup-form- 149 .lepopup-element- 9 .lepopup-element-html-content {min-height:18px;}.lepopup-form- 149 .lepopup-element- 10 * {font-family: 'Arial' , 'arial' ;font-size:15px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;}.lepopup-form- 149 .lepopup-element- 10 {font-family: 'Arial' , 'arial' ;font-size:15px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;background-color:transparent;background-image:none;border-width:1px;border-style:none;border-color:transparent;border-radius:0px;box-shadow:none;padding-top:0px;padding-right:0px;padding-bottom:0px;padding-left:0px;}.lepopup-form- 149 .lepopup-element- 10 .lepopup-element-html-content {min-height:18px;}.lepopup-form- 149 .lepopup-element- 11 * {font-family: 'Arial' , 'arial' ;font-size:15px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;}.lepopup-form- 149 .lepopup-element- 11 {font-family: 'Arial' , 'arial' ;font-size:15px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;background-color:transparent;background-image:none;border-width:1px;border-style:none;border-color:transparent;border-radius:0px;box-shadow:none;padding-top:0px;padding-right:0px;padding-bottom:0px;padding-left:0px;}.lepopup-form- 149 .lepopup-element- 11 .lepopup-element-html-content {min-height:18px;}.lepopup-form- 149 .lepopup-element- 12 * {font-family: 'Arial' , 'arial' ;font-size:15px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;}.lepopup-form- 149 .lepopup-element- 12 {font-family: 'Arial' , 'arial' ;font-size:15px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;background-color:transparent;background-image:none;border-width:1px;border-style:none;border-color:transparent;border-radius:0px;box-shadow:none;padding-top:0px;padding-right:0px;padding-bottom:0px;padding-left:0px;}.lepopup-form- 149 .lepopup-element- 12 .lepopup-element-html-content {min-height:18px;}.lepopup-form- 149 .lepopup-element- 13 * {font-family: 'Arial' , 'arial' ;font-size:15px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;}.lepopup-form- 149 .lepopup-element- 13 {font-family: 'Arial' , 'arial' ;font-size:15px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;background-color:transparent;background-image:none;border-width:1px;border-style:none;border-color:transparent;border-radius:0px;box-shadow:none;padding-top:0px;padding-right:0px;padding-bottom:0px;padding-left:0px;}.lepopup-form- 149 .lepopup-element- 13 .lepopup-element-html-content {min-height:18px;}.lepopup-form- 149 .lepopup-element- 14 div.lepopup-input .lepopup-icon-left, .lepopup-form- 149 .lepopup-element- 14 div.lepopup-input .lepopup-icon-right {line-height:36px;}.lepopup-form- 149 .lepopup-element- 15 div.lepopup-input{height:auto;line-height: 1 ;}.lepopup-form- 149 .lepopup-element- 16 * {font-family: 'Arial' , 'arial' ;font-size:14px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;}.lepopup-form- 149 .lepopup-element- 16 {font-family: 'Arial' , 'arial' ;font-size:14px;color:# 333333 ;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;background-color:transparent;background-image:none;border-width:1px;border-style:none;border-color:transparent;border-radius:0px;box-shadow:none;padding-top:0px;padding-right:0px;padding-bottom:0px;padding-left:0px;}.lepopup-form- 149 .lepopup-element- 16 .lepopup-element-html-content {min-height:5px;}.lepopup-form- 149 .lepopup-element- 19 * {font-family: 'Arial' , 'arial' ;font-size:13px;color:# 333333 ;font-style:normal;text-decoration:none;text-align:left;}.lepopup-form- 149 .lepopup-element- 19 {font-family: 'Arial' , 'arial' ;font-size:13px;color:# 333333 ;font-style:normal;text-decoration:none;text-align:left;background-color:transparent;background-image:none;border-width:1px;border-style:none;border-color:transparent;border-radius:0px;box-shadow:none;padding-top:0px;padding-right:0px;padding-bottom:0px;padding-left:0px;}.lepopup-form- 149 .lepopup-element- 19 .lepopup-element-html-content {min-height:363px;}.lepopup-form- 149 .lepopup-element- 0 * {font-size:15px;color:#ffffff;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;}.lepopup-form- 149 .lepopup-element- 0 {font-size:15px;color:#ffffff;font-weight:normal;font-style:normal;text-decoration:none;text-align:left;background-color:#5cb85c;background-image:none;border-width:0px;border-style:solid;border-color:#ccc;border-radius:5px;box-shadow: 1px 1px 15px -6px # 000000 ;padding-top:40px;padding-right:40px;padding-bottom:40px;padding-left:40px;}.lepopup-form- 149 .lepopup-element- 0 .lepopup-element-html-content {min-height:160px;}</style><div class = "lepopup-inline" style= "margin: 0 auto;" ><div class = "lepopup-form lepopup-form-149 lepopup-form-WWIlbsRygplKJhow lepopup-form-icon-inside lepopup-form-position-middle-right" data-session= "0" data-id= "WWIlbsRygplKJhow" data-form-id= "149" data-slug= "7lQM6oyWL5bTm5lw" data-title= "Under the Post Inline" data-page= "1" data-xd= "off" data-width= "820" data-height= "430" data-position= "middle-right" data-esc= "off" data-enter= "on" data-disable-scrollbar= "off" style= "display:none;width:820px;height:430px;" onclick= "event.stopPropagation();" ><div class = "lepopup-form-inner" style= "width:820px;height:430px;" ><div class = "lepopup-element lepopup-element-2 lepopup-element-rectangle" data-type= "rectangle" data-top= "0" data-left= "0" data-animation-in= "fadeIn" data-animation-out= "fadeOut" style= "animation-duration:0ms;animation-delay:0ms;z-index:501;top:0px;left:0px;width:820px;height:430px;" ></div><div class = "lepopup-element lepopup-element-3 lepopup-element-html" data-type= "html" data-top= "7" data-left= "10" data-animation-in= "bounceInDown" data-animation-out= "fadeOut" style= "animation-duration:0ms;animation-delay:0ms;z-index:502;top:7px;left:10px;width:797px;height:73px;" ><div class = "lepopup-element-html-content" >Do you want to know how to develop your skillset to become a <span style= "color: #CAB43D; text-shadow: 1px 1px #835D5D;" >Java Rockstar?</span></div></div><div class = "lepopup-element lepopup-element-4 lepopup-element-html" data-type= "html" data-top= "83" data-left= "308" data-animation-in= "bounceInDown" data-animation-out= "fadeOut" style= "animation-duration:0ms;animation-delay:0ms;z-index:503;top:83px;left:308px;width:473px;height:23px;" ><div class = "lepopup-element-html-content" >Subscribe to our newsletter to start Rocking <span style= "text-decoration: underline;" >right now!</span></div></div><div class = "lepopup-element lepopup-element-5 lepopup-element-html" data-type= "html" data-top= "107" data-left= "308" data-animation-in= "bounceInDown" data-animation-out= "fadeOut" style= "animation-duration:0ms;animation-delay:0ms;z-index:504;top:107px;left:308px;width:473px;height:24px;" ><div class = "lepopup-element-html-content" >To get you started we give you our best selling eBooks for <span style= "color:#e01404; text-shadow: 1px 1px #C99924; font-size: 15px;" >FREE!</span></div></div><div class = "lepopup-element lepopup-element-6 lepopup-element-html" data-type= "html" data-top= "136" data-left= "308" data-animation-in= "bounceInDown" data-animation-out= "fadeOut" style= "animation-duration:0ms;animation-delay:0ms;z-index:505;top:136px;left:308px;width:473px;height:18px;" ><div class = "lepopup-element-html-content" ><span style= "font-weight: bold;" > 1 .</span> JPA Mini Book</div></div><div class = "lepopup-element lepopup-element-7 lepopup-element-html" data-type= "html" data-top= "156" data-left= "308" data-animation-in= "bounceInDown" data-animation-out= "fadeOut" style= "animation-duration:0ms;animation-delay:0ms;z-index:506;top:156px;left:308px;width:473px;height:18px;" ><div class = "lepopup-element-html-content" ><span style= "font-weight: bold;" > 2 .</span> JVM Troubleshooting Guide</div></div><div class = "lepopup-element lepopup-element-8 lepopup-element-html" data-type= "html" data-top= "176" data-left= "308" data-animation-in= "bounceInDown" data-animation-out= "fadeOut" style= "animation-duration:0ms;animation-delay:0ms;z-index:507;top:176px;left:308px;width:473px;height:18px;" ><div class = "lepopup-element-html-content" ><span style= "font-weight: bold;" > 3 .</span> JUnit Tutorial for Unit Testing</div></div><div class = "lepopup-element lepopup-element-9 lepopup-element-html" data-type= "html" data-top= "196" data-left= "308" data-animation-in= "bounceInDown" data-animation-out= "fadeOut" style= "animation-duration:0ms;animation-delay:0ms;z-index:508;top:196px;left:308px;width:473px;height:18px;" ><div class = "lepopup-element-html-content" ><span style= "font-weight: bold;" > 4 .</span> Java Annotations Tutorial</div></div><div class = "lepopup-element lepopup-element-10 lepopup-element-html" data-type= "html" data-top= "216" data-left= "308" data-animation-in= "bounceInDown" data-animation-out= "fadeOut" style= "animation-duration:0ms;animation-delay:0ms;z-index:509;top:216px;left:308px;width:473px;height:18px;" ><div class = "lepopup-element-html-content" ><span style= "font-weight: bold;" > 5 .</span> Java Interview Questions</div></div><div class = "lepopup-element lepopup-element-11 lepopup-element-html" data-type= "html" data-top= "236" data-left= "308" data-animation-in= "bounceInDown" data-animation-out= "fadeOut" style= "animation-duration:0ms;animation-delay:0ms;z-index:510;top:236px;left:308px;width:473px;height:18px;" ><div class = "lepopup-element-html-content" ><span style= "font-weight: bold;" > 6 .</span> Spring Interview Questions</div></div><div class = "lepopup-element lepopup-element-12 lepopup-element-html" data-type= "html" data-top= "256" data-left= "308" data-animation-in= "bounceInDown" data-animation-out= "fadeOut" style= "animation-duration:0ms;animation-delay:0ms;z-index:511;top:256px;left:308px;width:473px;height:18px;" ><div class = "lepopup-element-html-content" ><span style= "font-weight: bold;" > 7 .</span> Android UI Design</div></div><div class = "lepopup-element lepopup-element-13 lepopup-element-html" data-type= "html" data-top= "282" data-left= "308" data-animation-in= "bounceInDown" data-animation-out= "fadeOut" style= "animation-duration:0ms;animation-delay:0ms;z-index:512;top:282px;left:308px;width:473px;height:18px;" ><div class = "lepopup-element-html-content" >and many more ....</div></div><div class = "lepopup-element lepopup-element-14" data-type= "email" data-deps= "" data-id= "14" data-top= "305" data-left= "308" data-animation-in= "fadeIn" data-animation-out= "fadeOut" style= "animation-duration:0ms;animation-delay:0ms;z-index:513;top:305px;left:308px;width:473px;height:36px;" ><div class = "lepopup-input" ><input type= "email" name= "lepopup-14" class = "lepopup-ta-left " placeholder= "Enter your e-mail..." autocomplete= "email" data- default = "" value= "" aria-label= "Email Field" oninput= "lepopup_input_changed(this);" onfocus= "lepopup_input_error_hide(this);" ></div></div><div class = "lepopup-element lepopup-element-15" data-type= "checkbox" data-deps= "" data-id= "15" data-top= "344" data-left= "308" data-animation-in= "fadeIn" data-animation-out= "fadeOut" style= "animation-duration:0ms;animation-delay:0ms;z-index:514;top:344px;left:308px;width:160px;" ><div class = "lepopup-input lepopup-cr-layout-1 lepopup-cr-layout-left" ><div class = "lepopup-cr-container lepopup-cr-container-medium lepopup-cr-container-left" ><div class = "lepopup-cr-box" ><input class = "lepopup-checkbox lepopup-checkbox-classic lepopup-checkbox-medium" type= "checkbox" name= "lepopup-15[]" id= "lepopup-checkbox-PaXNodnZtITlAxfV-14-0" value= "on" data- default = "off" onchange= "lepopup_input_changed(this);" ><label for = "lepopup-checkbox-PaXNodnZtITlAxfV-14-0" onclick= "lepopup_input_error_hide(this);" ></label></div><div class = "lepopup-cr-label lepopup-ta-left" ><label for = "lepopup-checkbox-PaXNodnZtITlAxfV-14-0" onclick= "lepopup_input_error_hide(this);" ></label></div></div></div></div><div class = "lepopup-element lepopup-element-16 lepopup-element-html" data-type= "html" data-top= "344" data-left= "338" data-animation-in= "fadeIn" data-animation-out= "fadeOut" style= "animation-duration:0ms;animation-delay:0ms;z-index:515;top:344px;left:338px;width:350px;height:5px;" ><div class = "lepopup-element-html-content" >I agree to the <a href= "https://www.javacodegeeks.com/about/terms-of-use" target= "_blank" >Terms </a> and <a href= "https://www.javacodegeeks.com/about/privacy-policy" target= "_blank" >Privacy Policy</a></div></div><div class = "lepopup-element lepopup-element-17" data-type= "button" data-top= "372" data-left= "308" data-animation-in= "bounceIn" data-animation-out= "fadeOut" style= "animation-duration:0ms;animation-delay:0ms;z-index:516;top:372px;left:308px;width:85px;height:37px;" ><a class = "lepopup-button lepopup-button-zoom-out " href= "#" onclick= "return lepopup_submit(this);" data-label= "Sign up" data-loading= "Loading..." ><span>Sign up</span></a></div><div class = "lepopup-element lepopup-element-19 lepopup-element-html" data-type= "html" data-top= "67" data-left= "-15" data-animation-in= "fadeIn" data-animation-out= "fadeOut" style= "animation-duration:0ms;animation-delay:0ms;z-index:518;top:67px;left:-15px;width:320px;height:363px;" ><div class = "lepopup-element-html-content" ><img data-lazyloaded= "1" src= "data:image/svg+xml;base64,PHN2ZyB4bWxucz0iaHR0cDovL3d3dy53My5vcmcvMjAwMC9zdmciIHdpZHRoPSIzMjAiIGhlaWdodD0iMzYzIiB2aWV3Qm94PSIwIDAgMzIwIDM2MyI+PHJlY3Qgd2lkdGg9IjEwMCUiIGhlaWdodD0iMTAwJSIgZmlsbD0idHJhbnNwYXJlbnQiLz48L3N2Zz4=" decoding= "async" data-src= "https://examples.javacodegeeks.com/wp-content/uploads/2015/01/books_promo.png.webp" alt= "" width= "320" height= "363" ><noscript><img decoding= "async" src= "https://examples.javacodegeeks.com/wp-content/uploads/2015/01/books_promo.png.webp" alt= "" width= "320" height= "363" /></noscript></div></div></div></div><div class = "lepopup-form lepopup-form-149 lepopup-form-WWIlbsRygplKJhow lepopup-form-icon-inside lepopup-form-position-middle-right" data-session= "0" data-id= "WWIlbsRygplKJhow" data-form-id= "149" data-slug= "7lQM6oyWL5bTm5lw" data-title= "Under the Post Inline" data-page= "confirmation" data-xd= "off" data-width= "420" data-height= "320" data-position= "middle-right" data-esc= "off" data-enter= "on" data-disable-scrollbar= "off" style= "display:none;width:420px;height:320px;" onclick= "event.stopPropagation();" ><div class = "lepopup-form-inner" style= "width:420px;height:320px;" ><div class = "lepopup-element lepopup-element-0 lepopup-element-html" data-type= "html" data-top= "80" data-left= "70" data-animation-in= "bounceInDown" data-animation-out= "fadeOutUp" style= "animation-duration:1000ms;animation-delay:0ms;z-index:500;top:80px;left:70px;width:280px;height:160px;" ><div class = "lepopup-element-html-content" ><h4 style= "text-align: center; font-size: 18px; font-weight: bold;" >Thank you!</h4><p style= "text-align: center;" >We will contact you soon.</p></div></div></div></div><input type= "hidden" id= "lepopup-logic-WWIlbsRygplKJhow" value= "[]" ></div><div class = "post-bottom-meta post-bottom-tags post-tags-classic" ><div class = "post-bottom-meta-title" ><span class = "tie-icon-tags" aria-hidden= "true" ></span> Tags</div><span class = "tagcloud" ><a href= "https://examples.javacodegeeks.com/tag/mvc-2/" rel= "tag" >mvc</a> <a href= "https://examples.javacodegeeks.com/tag/spring/" rel= "tag" >spring</a></span></div> |
This example is not very useful when the page tags are mixed in with the code.
Most of the “code” in FormValidator.java isn’t code, but text.
I’ve seen this on other pages, but this is one of the worst one so far.
I think these things wouldn’t happen so much if the pages were reviewed.