Apache Camel Sample Application Example
1. Introduction
What is Apache Camel? Even the Apache Camel site does not
seem to have a succinct example of this. They do point to a Stack Overflow
article which provides some insight. To me though Camel is all about mediation,
enrichment and transformation.
It is a well defined API to implement common Enterprise Integration
Patterns (EIP). The idea is send and receive messages and let the messages do
the work. It is lightweight and effective. Either standalone or coupled with
containers like Tomcat or Spring, Camel offers you the developer a number of
ways decouple services and use it for mediation.
While it certainly takes a while to get the gist of basic
examples, I am going to show you how to use Maven to build and deploy Camel and
then we will create a very simple example to demonstrate how it works.
1.1 Required Software
This article only covers the basics. However if you want to delve further a quick look at the examples folder that is part of the Camel install offers loads of different examples for the user and I highly recommend going through at least some of these to expedite your Camel learning adventure.
First, we need Java installed and then Maven. I am using the following software:
Java java version “1.8.0_181”
Maven Apache Maven 3.6.0
Apache Camel version 3.0.0-M1
1.3 Setup
Once you have java and Maven up and running, we can use Maven to generate the Camel archetype. The installation is interactive and you need to provide the necessary info to proceed..
mvn archetype:generate -DarchetypeGroupId=org.apache.camel.archetypes -DarchetypeArtifactId=camel-archetype-java
1.3.1 Confirm properties configuration:
The camel-core dependency, allows us to create a Camel Context
and build routes.
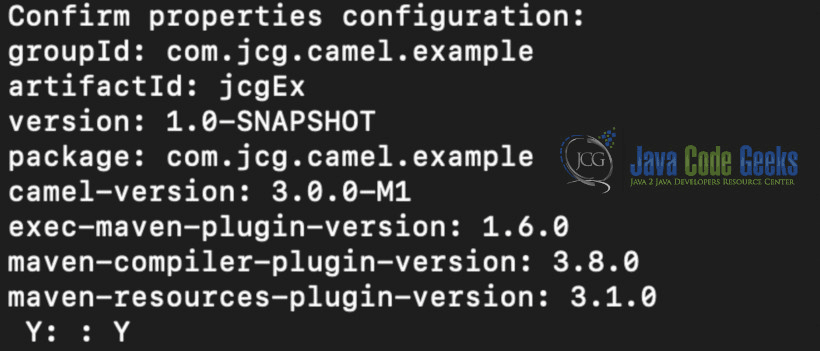
Edit the newly created pom.xml and add the camel-core Maven dependency if needed. My generated pom.xml already had this. if you are using a different version you can simply use the snippet below:
pom.xml
<dependency> <groupId>org.apache.camel</groupId> <artifactId>camel-core</artifactId> </dependency>
You now have a bare bones install of Apache Camel as your
project.
1.4 Next we want to install the Camel Maven Plugin.
This allows us to run our routes from Maven and as of Camel 2.19 provides Route validation: “The Camel Maven Plugin now provides the camel:validate
goal to parse your Java and XML source code for any Camel routes and report invalid Camel endpoint uri and simple expression errors. You can run this at code time (not runtime). ”
For more details of the goals provided, run:
mvn help:describe -Dplugin=org.apache.camel:camel-maven-plugin -Ddetail
In order to generate the Camel Maven Plugin run the following Maven Command:
mvn archetype:generate -DarchetypeGroupId=org.apache.camel.archetypes -DarchetypeArtifactId=camel-archetype-java
The the following is added to your pom.xml
pom.xml
<dependency> <groupId>org.apache.camel</groupId> <artifactId>camel-maven-plugin</artifactId> </dependency>
Installation completed. You can use the command: mvn compile exec:java to run the example app in standalone mode. You should see the following output if everything is setup correctly:
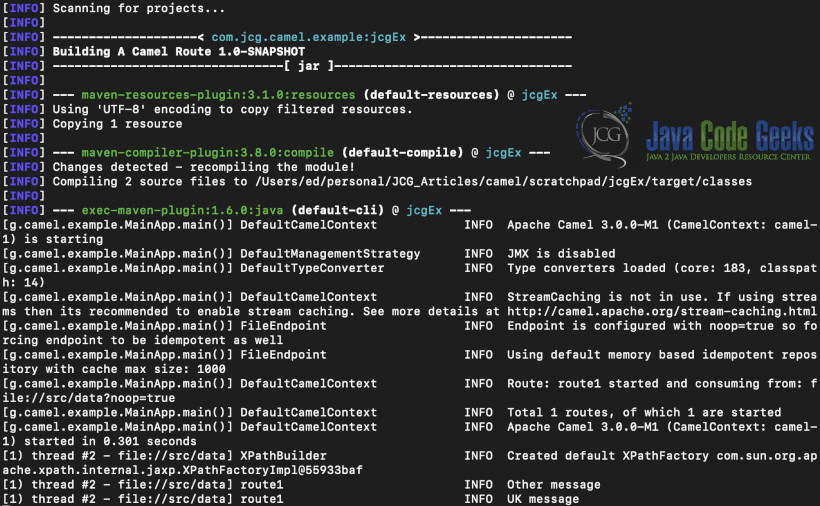
This standalone example is installed when the camel-maven-plugin
was installed. That’s great, but really it’s just a sanity check, to make sure
that everything is running smoothly.
Now let’s get to the implementation!
2. The Example Solution
Now that we have completed a successful installation, we need to build our first simple example application.
You are welcome to use the IDE of your choice and configure accordingly. Maven has loads of nifty tips and tricks to make our lives easier. If we want to build the necessary project files for our favourite IDE, all we need to do is type:
mvn idea:idea
for IntelliJ or mvn eclipse:eclipse
for Eclipse IDE users.
Camel either uses a Java DSL (Domain Specific Language) or an XML configuration for routing and mediation rules. These rules are added to the CamelContext in order to implement the various Enterprise Integration Patterns (EIP) mentioned earlier.
While we are not going into this topic in depth here, it is important to remember that Camel is made up of loads of components from simple request/reply, publish/subscribe, web services and many more that cover more than 60 message based EIP’s. For further reading checkout Enterprise Integration Patterns by Gregor Hohpe and Bobby Woolf here: https://www.enterpriseintegrationpatterns.com/
When you are looking for solutions to common orchestration
and mediation design challenges EIP’s are a world of help particularly when it
comes to messaging.
For the example here and in the interests of keeping it simple, I will focus on the File Transfer EIP. Here is a link that will explain this pattern in greater detail, but you have no doubt already dealt with similar solutions in the past.
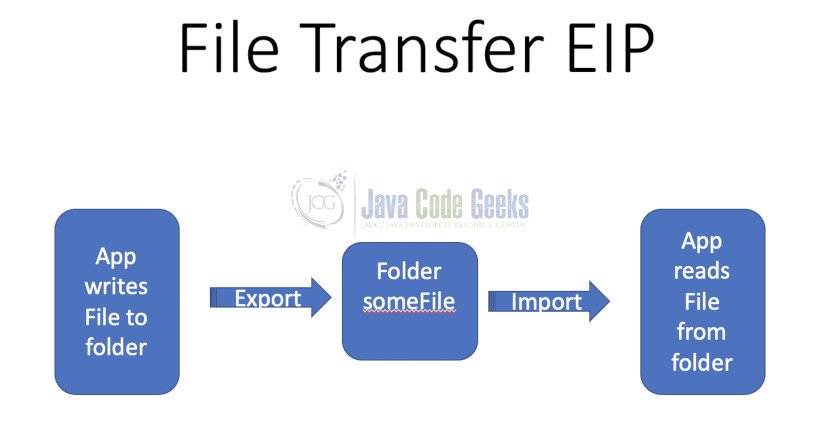
The above diagram represents a basic file transfer pattern representation
adopted from https://www.enterpriseintegrationpatterns.com/patterns/messaging/FileTransferIntegration.html
We will be implementing a single class that will demonstrate
the core concepts of CamelContext and Routing. There are numerous other
examples supplied with the camel installation that are excellent at helping developers
to fast understand a concept or idea. I urge you to explore them.
The following diagram essentially just represents a file copy from one folder to another. We will use leave the original file alone and simply add a copy to an output folder. We will configure Camel to ensure that the process is idempotent. Copying a file only once and then leaving it unchanged.
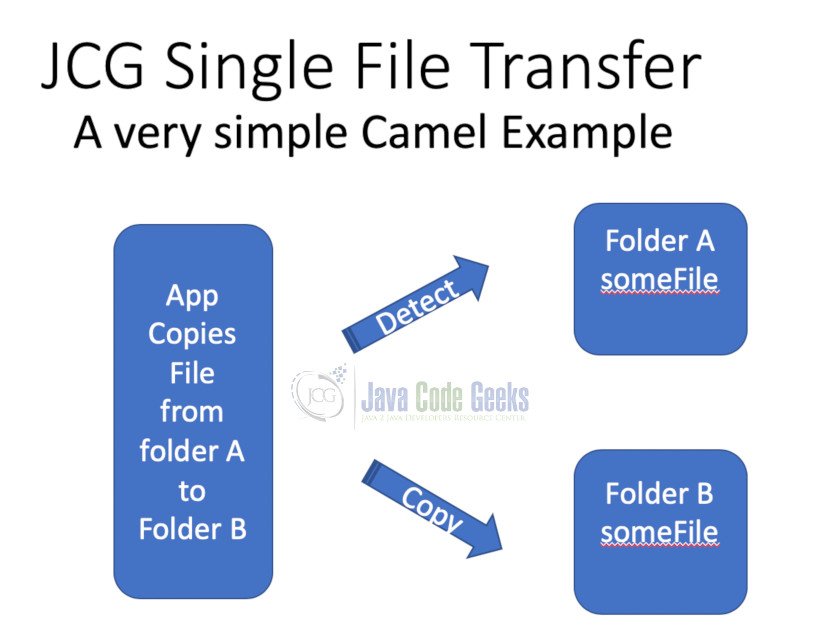
First we need to generate our Maven Archetype:
mvn archetype:generate -DarchetypeGroupId=org.apache.camel.archetypes -DarchetypeArtifactId=camel-archetype-java
Provide the required values
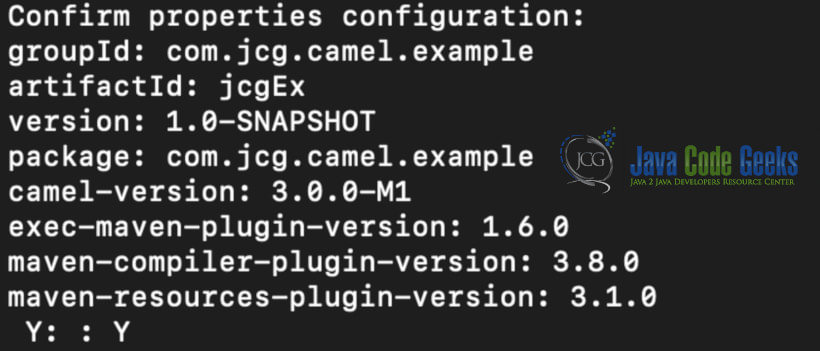
Hit Enter!!!
Note the camel version here is 3.0.0-M1
I’ve created a single class to handle everything.
CamelFileApp.java
import org.apache.camel.builder.RouteBuilder; import org.apache.camel.impl.DefaultCamelContext; import org.apache.camel.CamelContext; public class CamelFileApp { public static void main(final String[] args) throws Exception { String folderA = "file:demo/copyFrom"; String folderB= "file:demo/copyTo"; //First we get the CamelContext CamelContext camelContext = new DefaultCamelContext(); //Next we provide the Route info and tell Camel to set idempotent=true //by adding "?noop=true" to the URI camelContext.addRoutes(new RouteBuilder() { @Override public void configure() { from(folderA+"?noop=true").to(folderB); } }); //initiate Camel camelContext.start(); Thread.sleep(60000); //remember to terminate!!! camelContext.stop(); } }
Note the "?noop=true"
value added to the URI. This tells Camel that this operation is idempotent. The original file will be left unchanged in folder and a copy placed in folder B. The file will only be copied once. You can verify this by checking the file is in both the copyFrom and copyTo folders.
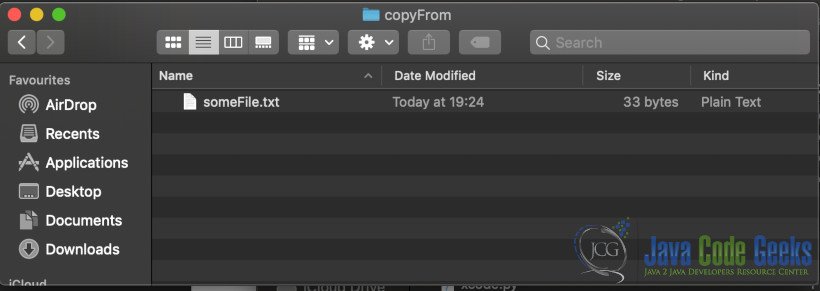
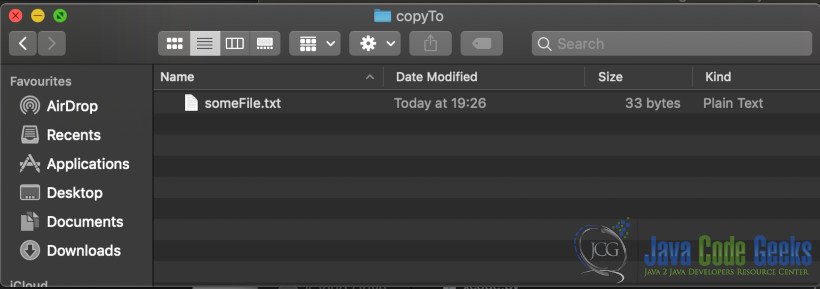
If you remove "?noop=true"
the original file is in fact moved from the copyTo folder and there is only a copy in the copyTo folder.
This is just one small example of Apache Camel. You can read
up more about noop=true and other powerful URI options provided by Camel here: http://camel.apache.org/file2.html
This example while simple, serves its purpose and I deliberately put the routing and context functionality in one class. Ideally though these would be two separate classes if you want a better design, but then in the real world this would also be run in container. I hope I have piqued your interest in Apache Camel. It is a very powerful tool for developers to have in their arsenal when it comes to Enterprise Integration Patterns.
3. Download the Source Code
This was an Apache Camel Sample Application Example
You can download the full source code of this example here: Apache Camel Sample Application Example
Do I need Thread.sleep and context.stop()? If I want to monitor the directory 24 / 7. And have my server running for 365 days?