Java Visitor Design Pattern Example
1. Introduction to Java Visitor Design Pattern
In this post we are going to discuss about one of the behavioral design patterns that is Java Visitor design pattern. Visitor design pattern is perhaps the most strong and efficient of all the design pattern that we have learned so far. The motive of a visitor pattern is to invent a new procedure without modifying the existing paradigm of an object. Just imagine we’ve got a composite object made of modules. The paradigm of the object is fixed, either we can not modify the structure, or we do not plan to add any new component varieties to it. In other words, Visitor design pattern is used for operations in a group of objects of the same kind. We can simply move the operating sort of logic from objects to a another class by using the visitor pattern.
Visitor design pattern enables one or more operations to be used on a set of objects in run time, which decouples them from the paradigm of an object. The Visitor Interface is the backbone of this pattern. The ConcreteVisitor also performs the Visitor Interface operations. The ConcreteVisitor also performs the Visitor Interface operations. Typically the concrete visitor saves the regional state, as it passes through the components. The interface for the component describes a technique of acceptation to enable the visitor to perform some action over this element. In fact, the visitor pattern creates an external class which includes data in the other classes. If a showcase of objects requires you to perform operations, visitor may be your pattern.
This article begins by showing the different design patterns available and follows the real world example of the visitor design pattern. This might help you to comprehend exactly when to use the visitor design pattern. We will then learn how to arrange classes to implement the java visitor pattern.
2. Types of Java Design Patterns
A design pattern is represented as a reusable remedy to a issue of design. You can report a solution to a design issue by means of a design pattern. A sensitive application of designs leads to increased maintenance to the coding, as some kind of recognize design patterns as an effective remedy to a prevalent problem and thus eliminate the shape of a certain component of computer software. Design patterns serve as a prevalent language for software developers to grasp the basic concept feasibly while working on common issues.
2.1 Creational Pattern
The instantiation method is demonstrated by creation design patterns. The composition and inheritance are mainly the basis of this design. It allows us to relocate from the rough coding of a particular set of traits to the reasoning of a much more complex set of shared behaviors. This includes a class that creates objects with a specific behaviour. The five design patterns are available: Abstract Factory, Prototype, Method of Factory, Builder and Singleton.
2.2 Structural Pattern
In order to build larger structures in classes and objects, structural design patterns actually contribute. Inherence in structural design patterns was used for the composition of interfaces or different implementations. Inheritance feature classes may be combined into one class. This allows structural object patterns to determine how new objects are developed. The mobility of the composition of the object enables us to change the structure over time. The flexibility of the structure of the object enables us to modify the shape over time.
2.3 Behavioral Pattern
Behavioral Design Pattern addresses the interaction of objects. It explains how objects interact between themselves and how the work steps are torn between various objects, in order to create the software more flexible and verifiable. The purpose of the pattern is to encapsulate an object request. Allows you to parameterize customers with various queries, queues or log requests and endorse unfeasible procedures.
If you have separate and different procedures to conduct over an object structure, you would use the visitor design pattern. This prevents you from introducing code in your object structure, which is way better maintained separately, so that code is cleaner. Maybe you want to operate on a variety of possible interface objects. If you have to conduct a number of unrelated procedures throughout the class, visiters are also worthwhile.
3. Visitor Design Pattern – Real life example
An example of the real world often makes it easier to completely understand any design pattern. An good example I saw for the visitor design pattern in action is a Uber/Ola taxi example, where a employee calls a Uber taxi to his doorstep. The Uber taxi visitor is in charge of transportation for that person after the person sits in.
Below we will discuss one more real life example in context of visitor design pattern. Let’s consider an example of school were many small childrens are studying and one day the school management decides for the health checkup of students and the school management approachs a child specialist doctor. After a couple of days doctor visit the school for regular checkup of students. In the below image you can see some students on the left side of the inage and visitor doctor on the right side.
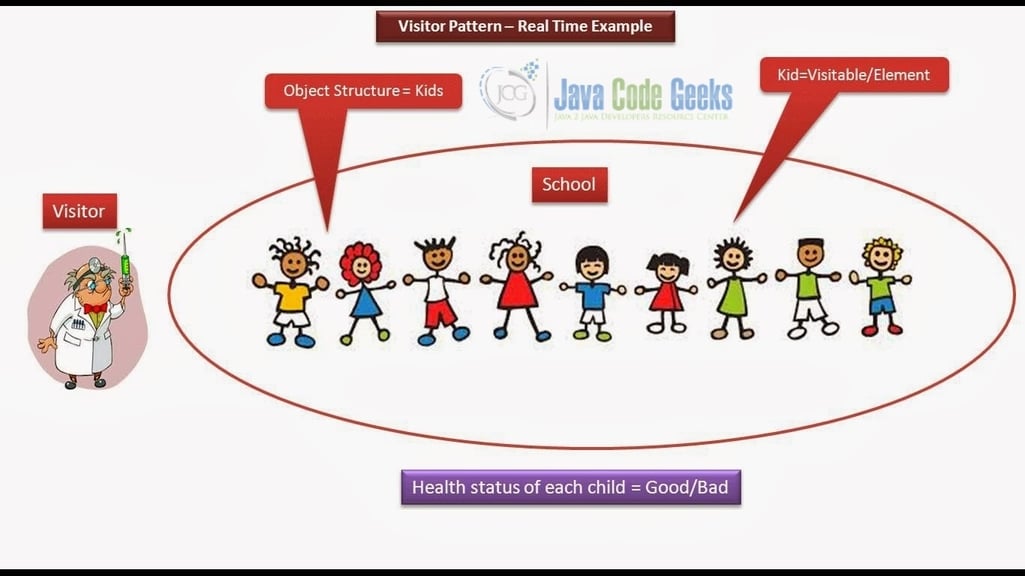
As shown in the above image the child specialist doctor visits and then checks health status of each and every students and gives report card or review of each students to the school management. So, here the child specialist doctor acts as a visitor and the students are the object structure for the example. Also the students are acts as a visitable element for the visitor doctor.
4. Implementing Visitor Design Pattern
The following scenario demonstrates a basic implementation of the Java visitor design pattern. An example that we understand in the above section as real world example is the one we use here in java programming.
Visitable.java
public interface Visitable { public void accept(Visitor visitor); }
In the above example, we have created an interface called Visitable. In this interface we have defined a accept() public method with Visitor object as a parameter but not implemented.
Student.java
public class Student implements Visitable { private String nameOfStudent; private String healthStatus; public Student( String nameOfStudent ) { super(); this.nameOfStudent = nameOfStudent; } public String getName() { return nameOfStudent; } public String getHealthStatus() { return healthStatus; } public void setHealthStatus( String healthStatus ) { this.healthStatus = healthStatus; } @Override public void accept( Visitor visitor ) { visitor.visit(this); } }
In the above code, we have created a class Student which implements Visitable interface inside we created two private variables nameOfStudent and healthStatus namely. Then we implemented the Student() method inside which we called the super() method and set the name of student. Then we implemented the getter and setter methods for both the variables like getName(), getHealthStatus(), setHealthStatus(). In the last step we implemented the accept() method with the parameter visitor.
Visitor.java
public interface Visitor { public void visit(Visitable visitable); }
In the above code, we have created an interface known as Visitor. In this interface we created the method visit() with the parameter of Visitable object.
Doctor.java
public class Doctor implements Visitor { private String nameOfDoctor; public Doctor( String nameOfDoctor ) { super(); this.nameOfDoctor = nameOfDoctor; } public String getName() { return nameOfDoctor; } @Override public void visit( Visitable visitable ) { Student student = (Student) visitable; student.setHealthStatus("Bad"); System.out.println("Doctor: '" + this.getName() + "' does the checkup of the student: '" + student.getName() + "' and Reported health is not good so updated the health status as 'Bad'\n"); } }
In the above code, we have created a class called Doctor which implements Visitor interface. In this class we defined a private vairable nameOfDoctor which represents the name of the visited doctor. Then we created a Doctor() method with the parameter name of Doctor and called super() inside this method. Then we defined a getter method for doctor name and returns the same. Then we override the visit() method with parameter Visitable inside this method we created the Student object and using this object we generate the health status report for each and every students that is good health or bad health.
School.java
import java.util.ArrayList; public class School { static ArrayList studentList; static { studentList = new ArrayList(); Student kishan = new Student("Kishan"); Student karan = new Student("Karan"); Student vishal = new Student("Vishal"); Student kapil = new Student("Kapil"); studentList.add(kishan); studentList.add(karan); studentList.add(vishal); studentList.add(kapil); } public static void doHealthCheckup() { Doctor doctor = new Doctor("Dr.Sumit"); for( Student student : studentList ) { student.accept(Doctor); } } }
In the above code we have created a class known as School. In this class we created a array list of student. Then we add some dummy names of the student in the student list one by one and then we implemented the doHealthCheckup() method in which doctor object is created and each student’s health is checked one after anthor.
VisitorDesignPattern.java
public class VisitorDesignPattern { public static void main( String[] args ) { School.doHealthCheckup(); } }
In the above code, we have created a VisitorDesignPattern class inside which we implemented the main() method. In this method we called the doHealthCheckup() method using the school object.
Doctor: 'Dr.Sumit' does the checkup of the student: 'Kishan' and Reported health is not good so updated the health status as 'Bad' Doctor: 'Dr.Sumit' does the checkup of the student: 'Karan' and Reported health is not good so updated the health status as 'Bad' Doctor: 'Dr.Sumit' does the checkup of the student: 'Vishal' and Reported health is not good so updated the health status as 'Bad' Doctor: 'Dr.Sumit' does the checkup of the student: 'Kapil' and Reported health is not good so updated the health status as 'Bad'
In the output we can see that Dr. Sumit do Health checkup for every students and reported the health status of each students whether it is good or bad. For example, ” ‘Dr.Sumit’ does the checkup of the student: ‘Kapil’ and Reported health is not good so updated the health status as ‘Bad “.
5. Benefits of Visitor Design Pattern
In this section, we are looking at several advantages of the visitot design pattern. The advantages of the visitor design pattern are as follows:
- The inclusion of a new item to the scheme is simple and only the visitor interface and execution will need to change, with no impact on extant item classes.
- Anthor advantage of the pattern is that if the operating reasoning alters, we only have to modify the visitor integration in all item classes, instead of doing it.
- In a single visitor class, you group decisions prevalent to several elements. In this class of visitors is the script only for that action. Makes reading the code simpler if you like to understand the code explicitly for one action.
- Since each component is visited, visitors can collect state masking the algorithm and all its information.
- The classes visited do not have to share a similar base class.
- Various concrete components do not need a certain algorithm to enact their part.
- A visitor object can collect valuable information while working with different objects. This could be useful when traversing a complicated object hierarchy, such as an object tree, and whenever you use the visitor for all of these objects.
- You can enter a new behaviour, which can actually work without modifying those classes with different objects classes.
- The same class may be used for several variants of the same kind of behavior.
- Visitor can be treated as a advanced variant of the Command Pattern. Its objects can perform operations over different class objects.
6. Conclusion
The visitor pattern is excellent to distinguish the dataset from its classes. In addition, it makes it much easier to introduce new operations only by offering the visitor with a new implementation. We also don’t rely on interfaces of modules and, if they are different, it is all well, because we have a distinct computation algorithm per concrete component. You can heighten the Visitor’s design pattern through Java reflection to provide a strong way to work on object systems and to make it flexible to introduce new sorts of visitors if necessary. The Visitor pattern involves the original programmer to predict possible future fully functional modifications in most computer languages. This is achieved with techniques that acknowledge a visitor and allow it to work with the original object collection.
7. Download the Project
You can download the project files for the above example from the below link:
You can download the full source code of this example here: Java Visitor Design Pattern Example
hey mate. do you have a error here.
Doctor doctor = new Doctor(“Dr.Sumit”);
for( Student student : studentList )
{
student.accept(Doctor);//HERE.
}