Java State Design Pattern Example
1. Introduction to Java State Design Pattern
In this article, we will introduce java state design pattern in detail. The java State design pattern is one of the behavioural design pattern. When an object changes its behavior based on its internal state, the State design pattern is used. So, we create objects in a State design pattern that represent different states and a context object, the behavior of which varies when the state object changes. The core idea behind the State design pattern is to enable the object without changing its class to modify its behavior. The code should also remain cleaner with its implementation without many if or else declarations.
Let us take an example of TV remote to understand the java state design pattern. Here we will consider only to states ON and OFF. Suppose if the ON button is pressed in the TV remote, it will turn On the TV and if the OFF button is pressed, it will turn Off the TV.
In this article we will discuss the various design pattern that are available and following a real life example of the state design pattern. It will help you to clarify actually when the java state design pattern is used. We will then learn how to plan the java program to implement a state design pattern.
2. Types of Java Design Patterns
More than one design problem can be solved by a single design pattern and more than one design pattern can solve a single design problem. There might be many design issues and solutions for it, but it does depend on your knowledge and knowledge of design patterns to choose the pattern which suits exactly. It depends also on the program that we already have.
Design patterns can be categorized in the following categories:
- Creational patterns
- Structural patterns
- Behavioral patterns
2.1 Creational Pattern
Creational design patterns are used for the design of the objects instantiation process. These design pattern uses the inheritance to change the creation of the object. These patterns contain two recurring steps. First, they all encapsulate the actual knowledge that the system uses in particular classes. Secondly, they stashed the creation and composition of instances of these classes. The whole system knows the objects by defining their interfaces by abstract classes. Therefore, you have a lot of flexibility with the creative patterns in what is created, who creates it, how it is created and when.
2.2 Structural Pattern
Structural design patterns relate to the form of larger systems by classes and objects. Structural class models are used for composing interfaces or implementations by the inheritance. Consider, as a simple example, how two or more classes are combined in one. The result is that the properties of their parent classes are combined. This model is especially helpful for making class libraries that have been developed independently.
2.3 Behavioral Pattern
Behavior patterns are about algorithms and duty assignment between objects. Behavioral patterns describe the patterns of communication not only between objects or classes. These patterns characterize complicated flows which are hard to follow during runtime. You move your focus away from control to focus only on how objects are connected.
In this article, we will discuss the java state design pattern with an example from real world. When an object changes its behavior based on its internal state, state design pattern is used. According to the definition the java state design pattern means “Allows an object to alter its behaviour when its internal state changes. The object will appear to change its class.” If the behavior of an object should be influenced by its state and when complex conditions bind the actions of the object to its state, then use the state design pattern.
3. State Design Pattern – Real life exam
This section explains a real world example based on the java state design pattern in such a way to easily understand this pattern. Here will we take a real life example of developing a web Application for a client. The below image shows the steps required to develop the web application in an efficient and effective way.
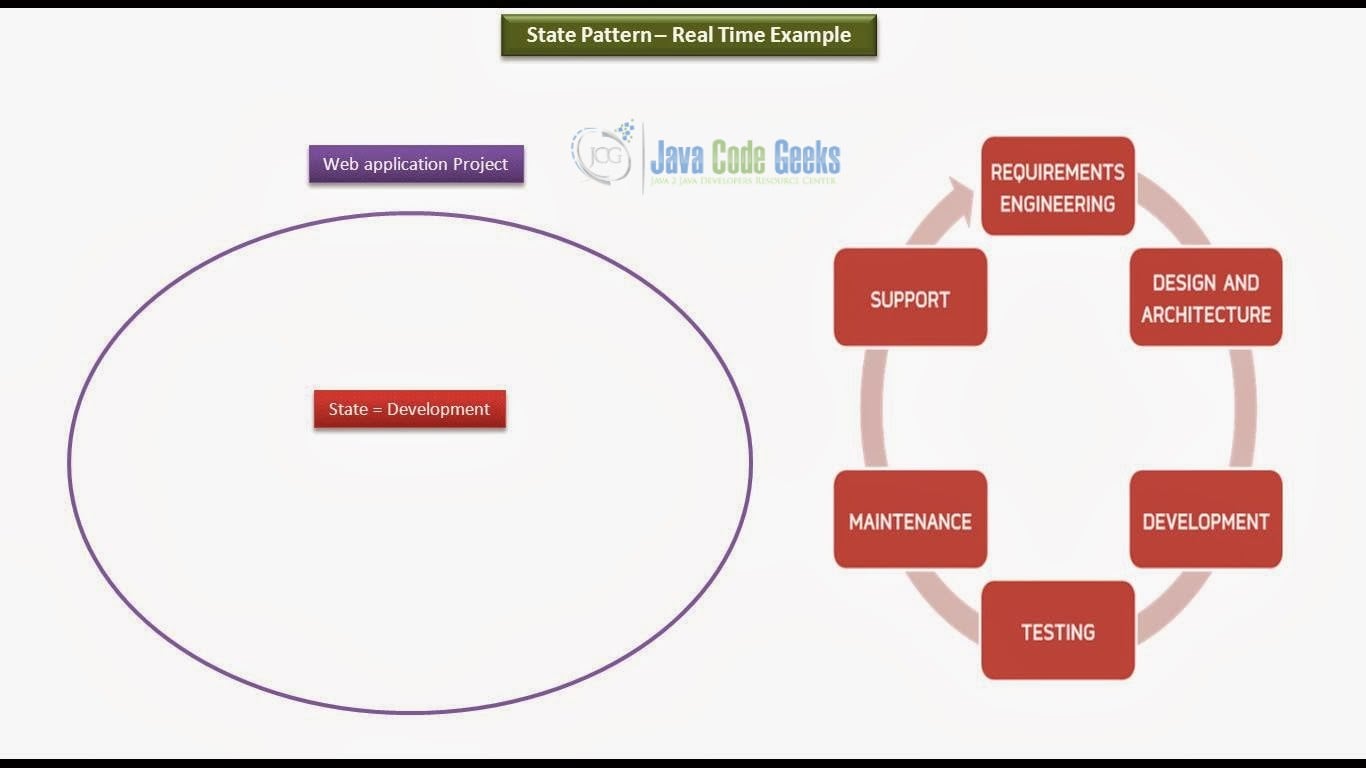
The web application developing consists of six steps as shown in the above image. In web application project developing the first step is Requirement Engineering in this step software engineer gathers all requirement form the client for the web application but we cannot do anything else in this step. And once the requirement gathering is done the state of the project will be moved to the second step. The second step is Design and Architecture in this step the software developer makes class, sequence, activity etc. diagrams related to the software. Similarly, when the second step is done the state of the project is further moved to the Development step. In Development step, the actual implementation of the web application takes place such as coding, programming, styling etc. once this step is finished the state moves to the fourth step.
After the implementation of the project, in Testing step various testing like manual testing, automation testing, integration testinng etc. is performed on the web application. Whenever the testing is completed the state further moves to the next state. The fifth step in web application is Maintenace, in this step the developer maintains the project after specific period of time. Once the Maintenace phase is done the internal state of the project will move to the last step of project that is Support. So, whenever the support step is finished again we can repeat all the six steps for developing any other web applicartion project for the client.
4. Implementing State Design Pattern
In this part we are going to program the java state design pattern using a real life concept. So here, we will discuss the fuctionality of Smart Tv such as Smart Tv switch ON and Smart Tv switch OFF states to understand the state design pattern.
SmartTvState.java
public interface SmartTvState { public void switchOnOffSmartTv(); }
In the above code, we created an interface called a SmartTvState. In this interface we have defined a method known as switchOnOffSmartTv().
SmartTvSwitchOffState.java
public class SmartTvSwitchOffState implements SmartTvState { @Override public void switchOnOffSmartTv() { System.out.println("Smart TV is Switched OFf"); } }
In the above code, we have created a SmartTvSwitchOffState class which implements the SmartTvState interface. Inside this class we implemented the switchOnOffSmartTv() method that we have defined in the SmartTvState interface.
SmartTvSwitchOnState.java
public class SmartTvSwitchOnState implements SmartTvState { @Override public void switchOnOffSmartTv() { System.out.println("Smart TV is Switched On"); } }
In the above code, we have created a SmartTvSwitchOnState class which implements the SmartTvState interface. Inside this class we implemented the switchOnOffSmartTv() method that we have defined in the SmartTvState interface.
SmartTv.java
public class SmartTv implements SmartTvState { private SmartTvState smartTvState; public SmartTvState getSmartTvState() { return smartTvState; } public void setSmartTvState(SmartTvState smartTvState) { this.smartTvState = smartTvState; } @Override public void switchOnOffSmartTv() { System.out.println("Current state Of Smart Tv : " + smartTvState.getClass().getName()); smartTvState.switchOnOffSmartTv(); } }
In the above code, we created a SmartTv class which implements the SmartTvState interface. In this SmartTv class we created a private SmartTvState inteface object called smartTvState. Also we have implemented the getters and setters method for smartTvState like getSmartTvState() and setSmartTvState() methods. Then we have implemented the switchOnOffSmartTv() method.
StateDesignPattern.java
public class StateDesignPattern { public static void main( String[] args ) { SmartTv smartTv= new SmartTv(); SmartTvState smartTvSwitchOnState = new SmartTvSwitchOnState(); SmartTvState smartTvSwitchOffState = new SmartTvSwitchOffState (); smartTv.setSmartTvState(smartTvSwitchOnState); smartTv.switchOnOffSmartTv(); smartTv.setSmartTvState(smartTvSwitchOffState); smartTv.switchOnOffSmartTv(); } }
In the above code, we have created a StateDesignPattern class in which we defined the main() method. In the main() method we create a smartTv object of SmartTv class. Also we have created two objects of SmartTvState interface. Using the smarttv object we set the smartTv states such as switch On state and switch Off state and called their corresponding methods switchOnOffSmartTv() and switchOnOffSmartTv().
Current state Of Smart Tv : SmartTvSwitchOnState Smart TV is Switched On Current state Of Smart Tv : SmartTvSwitchOffState Smart TV is Switched OFf
The above output says that when the State of the Smart Tv is ON then the “Smart TV is Switched On” or when the State of the Smart Tv is OFF then the “Smart TV is Switched OFf”. And the above Java program helps us to understand the state design pattern easily.
5. Benefits of State Design Pattern
Here, we will discuss some of the advantages of the State Design Pattern. The advantages are following:
- The State design pattern has ability to minimize conditional complexity is a major advantage.
- In the state design pattern the behavior of an object is the result of its state function and, depending on the state, the behavior is altered at runtime. This removes reliance on the conditional logic if / else or switch case.
- It negates any need to make statements on objects with various behavioral requirements, distinctive to multiple status transitions.
- The benefits of composable quality are obvious from the state pattern and states are also easier to add to enable additional performance.
- The State design design pattern also enhances cohesion as State behaviours, placed in one place in the code, are added to the ConcreteState classrooms.
- The probability of an error is less, and additional behavioral statements can be added very easily. This enhances the strength, maintenance and flexibility of our code.
6. Conclusion
We saw in this article that this pattern can be implemented very easily. It is much easier to encapsulate each of these statements into separate classes than to write several if-else statements. Moreover, each State’s implementation can differ regardless of the other States. Without changing other states we can easily increase the number of states to make this pattern scalable and adaptable. The State Design Pattern allows us to change the behavior of an object when it changes its internal condition. It is much more easy to track all possible conditions by using state design patterns, and this guarantees a less unspecified behavior for our software applications. Many other ways of implementing a state pattern are available. For designing an efficient state pattern, the designer must list the potential states and pertain each other or define a sequence of states.
7. Download the Project
You can download the project files for the above example from the below link:
You can download the full source code of this example here: Java State Design Pattern Example