Java Iterator Design Pattern Example
1. Introduction
In this article, we will discuss the Java Iterator Design Pattern in detail. Java Iterator Design Pattern is one of the most famous design pattern that is used in Java as well as .NET programming. It is being used frequently due to the need of iterators in almost every project. Iterator Design pattern focuses on exposing ways to retrieve an iterator and perform tasks using it. Java Iterator Design pattern is used precisely where there is need of collection of objects frequently.
The Java Iterator Design pattern is used primarily to conceal the implementation of how the objects are being iterated. It deals with creation of classes that provides variety of ways to Iterative through the collection of objects. Before we proceed with the understanding of Java Iterator Design Pattern in depth, let us understand the different types of design patterns that Java has to offer and understand where Java Iterator Design Pattern stands.
This pattern masks the real transversal rollout of the collection. For various reasons the application systems only use iterator methods. The iterator pattern enables sequential, unknown access to the components of a collection object. The important points on this interface to recollect are:
- This pattern can be used when a collection is predicted to be traversed with different standards.
- Without revealing its data structure, the collection object should also be obtained and traversed.
- For the collection object, new transverse procedures without altering its functionality should be defined.
2. Types of Design Pattern
Design patterns are a recent innovation for software development. A prevalent and well-portrayed solution is a design pattern for a powerful software problem. A delicate use of design patterns results in increased programming repairs, as design patterns can be acknowledged by some as a perfect solution to a common issue and thus reduce the contour in the conduct of a particular piece of software. Design patterns comprise of design patterns such as creational, structural and behavioral design pattern.
2.1 Creational Pattern
Creational design patterns illustrate the instantiation process. This design pattern relies primarily on the composition and inheritance. They enable us to move from the tough programming of a specific set of behaviors to the logic of a smaller set of common behaviors which can be made up of much more complicated ones. This involves something more than a class to create objects with a particular behavior. There are five creational designs pattern: Abstract Factory, Prototype, Factory Method, Builder and Singleton.
2.2 Structural Pattern
Structural patterns contribute to the setup of larger systems in classes and objects. For the composition of interfaces or different implementations, inhertance in structural design patterns is used. For example, two or more classes of inheritance functionalities can be coupled into one class. This allows two or more class libraries to operate freely together. This enables structural object patterns to determine how to develop new objects. The mobility of the object’s composition allows us to change the structure during run-time.
2.3 Behavioral Pattern
The communication of objects is stated by a behavioral design pattern. It discusses how different objects and classes send one another information so that things are happening and how the relevant task steps are split into various objects. When creative patterns define a time and structural patterns define a hierarchy that is almost static, behavioral patterns define a mechanism or flow.
We will explore one of the structural design patterns below in this article-the Iterator design pattern. The Iterator design pattern is a type of structural design pattern. This pattern generates a class of that gives out iterators that envelops the original class iteration logic and provides extra features, keeping the fingerprint of class methods undamaged.
3. Java Iterator Design Pattern – Real Life Example
The best way to understand any design pattern is to map it to a real life example and try to code a solution accordingly. A perfect example of an iterator design patterm is the MP3 player control. The user can’t worry about viewing their play list when they see it. This was accomplished with basic forward and back buttons in old mp3 players. This altered to the idea of wheel interface with the iPod. The iPhone is moving this to use swipe motions further. However, all devices offer similar idea-a way to iterate out of your complete set of music.
Before understanding the real world example, Let’s get a another practical example to comprehend iterator pattern. Assuming that we have a list of radio channels, and the user system wants to traverse them one after another or on a channel- For instance, some user systems want only Spanish channels to function and do not want to process other kinds of channels. Thus we can supply the client with a collection of channels and let them publish the channels through it and determine if they will be processed.
We will now discuss an another real life example of Iterator design pattern to understand the concept well. Here we will look into an example of notification bar/alert bar in our mobile application/ web application. We could use Iterator pattern here and supply Notification/Alert – based iteration. We should ensure that the customer system only has iterator access to the list of Notification/Alert.
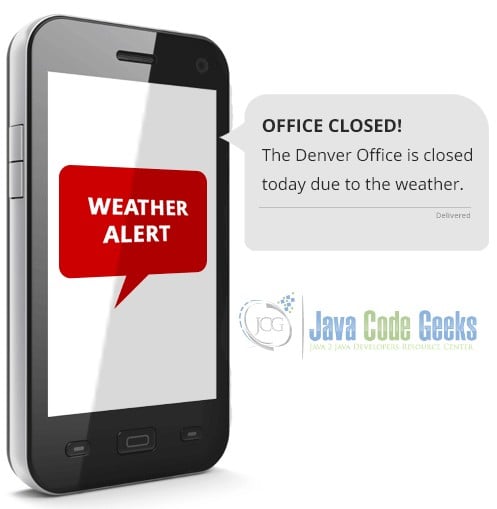
Let’s get in detail to understand this. Assume that in our application we create a notification bar displaying all the alerts held within a stash. Notification/Alert Collection offers an iterator for the iteration of its components all without showing the Customer how it is instituted the collection. The iterator functionality contains a number of techniques for traversing or altering a collection that can also provide search functions, remove functions etc.
Iterator pattern isn’t just about traversing a stash, we can also provide various kinds of iterators premised on our needs. Iterator design pattern conceals the real traversal enactment via the collection and customer initiatives simply use iterator techniques.
4. Implementing Iterator Design Pattern
In this section, we will understand the java iterator design pattern in detail by implementing it in java program. For the implementation we will take the above discussed real life example of Notification/ Alert bar in our mobile or web application.
Alert.java
public class Alert { String alert; public Alert(String alert) { this.alert = alert; } public String getAlert() { return alert; } }
In the above code, we have created a class called Alert in which we defined a string variable alert. And we created two methods such as Alert() with one parameter alert and getAlert() method which returns the alert object.
CollectionSet.java
public interface CollectionSet { public Iterator createIterator(); }
In the above code, we have created an interface known as CollectionSet. In this interface we defined an Iterator method called createIterator().
AlertCollection.java
public class AlertCollection implements CollectionSet { static final int MAX_ALERTS = 8; int numberOfAlerts = 0; Alert[] alertList; public AlertCollection() { alertList = new Alert[MAX_ALERTS]; addAlert("Alert 1"); addAlert("Alert 2"); addAlert("Alert 3"); addAlert("Alert 4"); addAlert("Alert 5"); } public void addAlert(String str) { Alert alert = new Alert(str); if (numberOfAlerts >= MAX_ALERTS) System.err.println("COMPLETED"); else { alertList[numberOfAlerts] = alert; numberOfAlerts = numberOfAlerts + 1; } } public Iterator createIterator() { return new AlertIterator(alertList); } }
In the above code, we have created a AlertCollection class which implements CollectionSet interface. In this class we defined a final variable MAX_ALERTS and a list namely alertList. Then we have created a AlertCollection method in which we add dummy notification or alert using addAlert method(). Then we implemented the addAlert() and createIterator() method.
Iterator.java
public interface Iterator { boolean hasNext(); Object next(); }
In the above code, we have created an interface called Iterator. In this interface we implement boolean hasNext() which indicates whether there are more elements to iterate over or not and also we implement Object next() that returns the next element in the collection.
AlertIterator.java
pubilc class AlertIterator implements Iterator { Alert[] alertList; int position = 0; public AlertIterator(Alert[] alertList) { this.alertList = alertList; } public Object next() { Alert alert = alertList[position]; position += 1; return alert; } public boolean hasNext() { if (position >= alertList.length || alertList[position] == null) return false; else return true; } }
In the above code, we have created a class called AlertIterator which implements the Iterator interface. In this class we a alertList and a position variable which maintains the current position of iterator over the array. Then we create AlertIterator constructor takes the array of alertList are going to iterate over. After then we created the next() and hasNext() method. The next() method return the next element in the array and increament the position whereas the hasNext() method checks whether there is element in the next position or not and verifies.
AlertBar.java
public class AlertBar { AlertCollection alerts; public AlertBar(AlertCollection alerts) { this.alerts = alerts; } public void printAlerts() { Iterator iterator = alerts.createIterator(); System.out.println("-------Alert BAR------------"); while (iterator.hasNext()) { Alert n = (Alert)iterator.next(); System.out.println(n.getAlert()); } } }
In the above code, we have created AlertBar class in which we have implemented the AlertBar constructor which takes the parameter of alerts. Then we created a method called printAlerts() inside this method we create object of Iterator interface and called createIterator() method. At last we print the alerts.
IteratorDesignPattern.java
class IteratorDesignPattern { public static void main(String args[]) { AlertCollection alertCollection = new AlertCollection(); AlertBar alertBar = AlertBar(alertCollection); alertBar.printAlerts(); } }
In the above code, we have created an IteratorDesignPattern class inside this class we implemented the main() method. In the main() method we created an object of AlertCollection and an object of alertBar. Then we print all the alerts using the alertBar object.
-------Alert BAR------------ Alert 1 Alert 2 Alert 3 Alert 4 Alert 5
From the output we can assume that, the Iterator pattern is beneficial if you wish to provide a proper way to iterate a collection and conceal the client program’s application logic. The iteration logic is integrated in the collection and can feasibly be used by the customer.
5. Benefits of Iterator Design Pattern
In this section, we explore several advantages of the iterator design pattern. The advantages of the iterator design pattern listed here are as follows:
- You can impose or subclassify an iterator in such a way that the regular ones don’t do anything without needing to change the real object iterates over.
- Iterator design pattern conceals the real traversal implementation via the collection and customer initiatives simply use iterator techniques.
- Objects which can be crossed over should not clog their workflows with traversal techniques, especially any highly skilled techniques.
- The Iterator pattern includes interfaces of iterations, so we don’t have to see which collection objects like an ArrayList or a HashTable are being used from now on.
- You can distribute Iterators to as many customers as you like and every customer could pass by his own pace in his own time.
- In Java, you can customize the iterator design pattern as needed. Also on the same collection we can use many iterators.
- The generic Iterator only requires a loop to cope with any kind of stash of products polymorphically, as only the Iterator is deployed.
- In particular, Java Iterators from the java.util package will throw an exception if you modify the storage space that supports them when you still have an Iterator out. This exception allows you to understand that incorrect objects may now return back to the Iterator.
6. Conclusion
The Iterator pattern enables synchronous direct access to an aggregate components without its inherent formulation being exposed. The Iterator pattern is indeed liable for the complete job iteration and thus removes extra burden and simplifies the functionality and its implementation, having left it to be responsible. Lastly, the Iterator relates to two basic principles initiatives which are highly cohesive in classes designed across a set of tasks pertaining to one another and the Single Obligation, where the class has a specific purpose or duty of care. Iterator pattern is a design pattern that is fairly straightforward and often used. In each language there are a great deal of data structures and collections. Every collection needs to include an iterator to iterate its objects. In doing so, however, it should ensure that its enactment does not become known. The use of the Iterator Design pattern in Java has been very clean in the coding of all of the data collections: collections, list and Iterator interfaces, and classes such as lists, maps, sets and all their sub – classes.
7. Download the Project
You can download the project files for the above example from the below link:
You can download the full source code of this example here: Java Iterator Design Pattern Example