Java Interpreter Design Pattern Example
1. Introduction to Java Interpreter Design Pattern
The interpreter design pattern is one of the behavioral design patterns that indicate how the words can be evaluated in a language. For an example, we will take one of our day to day used application Google Translator. Google Translator is an Interpreter design pattern example, in which the input can be read in any other language and the output can be read in another language. According to the definition the Interpreter design pattern simply means “Given a language, define a representation for its grammar along with an interpreter that uses the representation to interpret sentences in the language.”
Design patterns are demonstrated alternatives to common issues in software design. They include the right and evaluated solution for what has been frequently found in software platforms. Such a solution is the interpreter deisgn pattern. The interpreter design model shows how customized dialects are evaluated. For an instance, the Spring spel language and even SQL are unlimited examples. We may say that the interpreter design pattern is similar to the pattern of the composite design pattern. When the consequent Hierarchical framework embodies grammar, Composite design generally works as an interpreter design pattern so we got the interpreter who accepts and interprets a set of rules. This pattern basically evaluates grammar and language phrases.
This article begins with a response to various available design patterns, which we understand through a real-world of the Interpreter Design scenario. It would help you understand exactly when you will use the Interpreter design pattern. When we understand this, we will exactly know how to code classes and try to implement the Interpreter design pattern.
2. Types of Java Design Patterns
As the name suggests, design patterns are the solutions for problems that most often occur while designing a software and more frequently. Most of these are evolved instead of discovered. Many computer program learners have been summed up in these design patterns by many professionals. Neither of these patterns will compel you to do much about the enactment; they are simply the regulations to fix a specific problem in general contexts. Our prior responsibility is the implementation of the code. Let’s learn more about these design patterns, as they are so important in the context of java.
- Creational patterns
- Structural patterns
- Behavioral patterns
2.1 Creational Pattern
Here the design patterns are subdivided into six different kinds of design. Creative design patterns are used in class instantiation. These design patterns also provide flexible methods of constructing objects, significantly reducing class binaries and improve the scalability of code. In particular, creative design patterns can offer a complete adjustment upon which objects are produced, how and how these objects are created. These design patterns are however broken down into design classes and objects. They use inheritance efficiently in the installation procedure when working with class creation patterns, but object generation patterns efficiently use a delegation to complete the work. These design models are used to create objects that can be separated from their performance structure.
2.2 Structural Pattern
The Structural Design Pattern contains seven different pattern types. These design patterns show how different elements of an application can be integrated flexibly and extensively. Structural patterns potentially help us to ensure that the entire structure is not necessarily changed when one of the elements or system components changes. These patterns are mainly concerned with the integration of class and object.
2.3 Behavioural Pattern
The behavioral design consists of eleven different design models to determine the interaction mechanism between the objects. The behavioral design pattern recognizes the interaction of the objects. This pattern sends or pushes messages to each other for communication purposes, as different objects and classes. The behavioral design model focuses on how problems can be fixed and how duties can be allocated between objects. These design patterns do not include specification, but rather communication.
In this article, we are going to explain the Interpreter design pattern by understanding an example of a real world and then use java programming to implement this pattern. The interpreter design pattern shows programmatically how to evaluate phrases in a language. It helps to create a grammar in a simple language, so that phrases can be interpreted in the language.
3. Interpreter Design Pattern – Real world example
In this section, you will find an explanation of real life example of the Interpreter design pattern. This example will explain how the the Interpreter design pattern can be used in simple grammar expressions and sometimes in simple rule engines. Here we would take the example of Google Translator which is a language translation application and we will undersatand how Google Translator is a real world example of Interpreter design pattern.
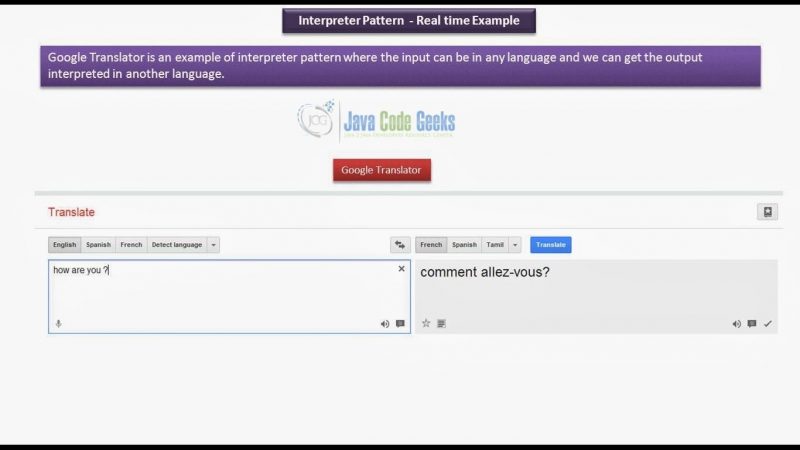
Google Translator Application offers a Web interface, Mobile app and ios app and a software-enabled API. Google Translate supports more than 100 languages at different levels, serving up over 500 million users each day as of May 2016 and will help developers build browser extensions and software applications. Google Translator is a really great example of an interpreter design pattern so that we can offer input in every language in the Google Translator, then what the Google Translator does it reads the data and it will provide the output in a different language. So, considering all this we may say that Google Translator is a very good example of interpreter design pattern.
4. Implementing Interpreter Design Pattern
Upto now we understand the idea of the Interpreter design pattern and the real world example of it. So in this section, the Interpreter design pattern will be put into action in Java progam and how the classes are defined based on the Interpreter Design pattern will be understandable. We take an example of date conversion into various formats such as MM-DD-YYYY or DD-MM-YYYY to understand the interpreter design pattern.
Format.java
import java.util.Date; public class Format { public String format; public Date date; public String getFormat() { return format; } public void setFormat( String format ) { this.format = format; } public Date getDate() { return date; } public void setDate( Date date ) { this.date = date; } }
In the above code, we have created a class called Format in which we have defined two public variables such as format, date of type Date. Then we defined the setters and getters method for Format and Date like getFormat(), setFormat(), getDate() and setDate().
AbstractFormat.java
public abstract class AbstractFormat { public abstract void execute( Format format); }
In the above code, we have created a abstract class known as AbstractFormat. In this abstract class we have defined a public abstract method called execute() which has a parameter of type Format class.
DayFormat.java
import java.util.Date; public class DayFormat extends AbstractFormat { @Override public void execute( Format format ) { String format1 = format.getFormat(); Date date = format.getDate(); Integer day = new Integer(date.getDate()); String tempFormat = format1.replaceAll("DD", day.toString()); format.setFormat(tempFormat); } }
In the above code, we have created a class DayFormat which extends the AbstractFormat class. In this class we implemented the execute() method that we have just defined in the AbstractFormat class. In this method we create two variables format, date and day and called getFormat() and getDate() methods.
MonthFormat.java
import java.util.Date; public class MonthFormat extends AbstractFormat { @Override public void execute( Format format ) { String format1 = format.getFormat(); Date date = format.getDate(); Integer month = new Integer(date.getMonth()+1); String tempFormat = format1.replaceAll("MM", month.toString()); format.setFormat(tempFormat); } }
In the above code, we have created a class MonthFormat which extends the AbstractFormat class. In this class we implemented the execute() method that we have just defined in the AbstractFormat class. In this method we create two variables format, date and month and called getFormat() and getDate() methods.
YearFormat.java
import java.util.Date; public class YearFormat extends AbstractFormat { @Override public void execute( Format format ) { String format1 = format.getFormat(); Date date = format.getDate(); Integer year = new Integer(date.getYear() + 2000); String tempFormat = format1.replaceAll("YYYY", year.toString()); format.setFormat(tempFormat); } }
In the above code, we have created a class YearFormat which extends the AbstractFormat class. In this class we implemented the execute() method that we have just defined in the AbstractFormat class. In this method we create two variables format, date and year and called getFormat() and getDate() methods.
InterpreterDesignPattern.java
import java.util.ArrayList; import java.util.Date; import java.util.Scanner; public class InterpreterDesignPattern { public static void main( String[] args ) { System.out.println("Please select the Date Format: 'MM-DD-YYYY' or 'YYYY-MM-DD' or 'DD-MM-YYYY' "); Scanner scanner = new Scanner(System.in); String inputDate = scanner.next(); Format format = new Format(); format.setFormat(format); format.setDate(new Date()); ArrayList formatOrderList = getFormatOrder(format); System.out.println("Input : " + format.getFormat() + " : " + new Date()); for( AbstractFormat abstractFormat : formatOrderList ) { abstractFormat.execute(format); System.out.println(abstractFormat.getClass().getName() + " Executed: " + format.getFormat()); } System.out.println("Output : " + format.getFormat()); } private static ArrayList getFormatOrder( Format format) { ArrayList formatOrderList = new ArrayList(); String[] strArray = format.getFormat().split("-"); for( String string : strArray ) { if( string.equalsIgnoreCase("MM") ) { formatOrderList.add(new MonthFormat()); } else if( string.equalsIgnoreCase("DD") ) { formatOrderList.add(new DayFormat()); } else { formatOrderList.add(new YearFormat()); } } return formatOrderList; } }
In this above code, we imported ArrayList, Date and Scanner modules form java library then we have created InterpreterDesignPattern class and inside this class we implemented the main() method. Then we have created a Scanner class variable scanner that will be used to take the input from the user. And we created a Format class variable format and called the setFormat() and setDate() methods. Then we have created a Array List of AbstractFormat class then we use for loop to get the name and class of the format of the date. In the last step we implemented the getFormatOrder() method of Array List of type AbstractFormat class. In this method we compare the user entered date format with the month format, day format and year format using the classes DayFormat(), MonthFormat() and YearFormat() and we return formatOrderList.
Please select the Date Format : 'MM-DD-YYYY' or 'YYYY-MM-DD' or 'DD-MM-YYYY' MM-DD-YYYY Input : MM-DD-YYYY : THUR Feb 14 22:40:45 IST 2019 MonthFormat Executed : 2-DD-YYYY DayFormat Executed : 2-14-YYYY YearFormat Executed : 2-14-2019 Output : 2-14-2019 Please select the Date Format : 'MM-DD-YYYY' or 'YYYY-MM-DD' YYYY-MM-DD Input : YYYY-MM-DD : THUR Feb 14 22:40:55 IST 2014 YearFormat Executed : 2019-MM-DD MonthFormat Executed : 2019-2-DD DayFormat Executed : 2019-2-9 Output : 2019-2-14
In the above output, we have provided the date format “MM-DD-YYYY” and the date output is 2-14-2019 and when we provided the date format “YYYY-MM-DD” the output will be 2019-2-14. Similarly for the date format “DD-MM-YYYY”.
5. Benefits of Interpreter Design Pattern
Here we are discussing some of the benefits of the Interpreter design pattern in this section. The benefits are as follows:
- Changing and expanding grammar is easy. Because the Interpreter design pattern uses grammar classes, then you also can change or extend your grammar with inheritance. Incremental modification of existing expressions can be made, and new ones defined as changes to old ones.
- The grammar is also easy to implement. Keys defining endpoints have similar rollout in the abstract syntax tree. Such classes are easier to write and can often be automated by a compiler or parser generator.
- It’s difficult to maintain complex grammars. For all the grammatical rules the interpreter design defines at least one class. Thus it is difficult to administer and maintain grammars containing many rules. Additional patterns of design can be used to alleviate this problem. And if the grammar is highly complex, other techniques, like parsers or compilers, are better suited.
- Introduce new different ways to translate words. The model of the interpreter facilitates a new evaluation of the expression. For instance, by defining new operations in the expression classes you can endorse fairly publishing or type-checking. Though if you continue to create new ways of interpreting a term, consider using visitor patterns so that grammar classes are not modified.
6. Conclusion
The interpreter pattern may appear only to be applicable in situations. Many developers therefore tend to overlook it. You will realize how important this design pattern is as you grow as a developer. When we can create a syntax tree for our grammar, the interpreter pattern can be used. The interpreter design pattern requires numerous bug checks and a number of expressions and codes. When grammar gets more complex and therefore difficult to maintain and provide efficiency, it becomes complicated. A common problem, for instance, is looking for strings which match a pattern. The standard language to specify string patterns is regular expressions. Instead of building individual string algorithms, searches algorithms can interpret a regexp which stipulates a set of strings that suit each pattern.
7. Download the Project
You can download the project files for the above example from the below link:
You can download the full source code of this example here:
Java Interpreter Design Pattern Example