Java 12 New Features Tutorial
In this tutorial, I will demonstrate how to install JDK12 and dive into several API changes with Java examples.
Table Of Contents
1. Introduction
JDK 12 is released on March 19, 2019. It includes several JDK Enhancement Proposals (JEPs). Here are the JEPs:
- 189 – Shenandoah: A Low-Pause-Time Garbage Collector (Experimental)
- 230 – Microbenchmark Suite
- 325 – Switch Expressions (Preview)
- 334 – JVM Constants API
- 340 – One AArch64 Port, Not Two
- 341 – Default CDS Archives
- 344 – Abortable Mixed Collections for G1
- 346 – Promptly Return Unused Committed Memory from G1
In this tutorial, I will use Oracle OpenJDK 12 to demonstrate the following API changes:
- The
switch
expression - The
java.util.stream.Collectors
‘s new method –teeing
- The
java.text.NumberFormat
‘s new formatting styles - The
java.lang.String
class’s new methods:indent
andtransform
- Support Unicode 11, including chess symbols
2. Technologies Used
The example code in this article was built and run using:
- Java 12
- Maven 3.6.0
- Eclipse 4.11
- Junit 4.12
3. Set Up
3.1 Install Oracle Open JDK 12
In this step, I will install Oracle OpenJDK 12 in my Windows 10 PC. Oracle documents the installation steps.
First, download the zip file – openjdk-12.0.1_windows-x64_bin.zip
from http://jdk.java.net/12/ and extract it to a folder.
Secondly, follow the steps here to configure JAVA_HOME
and PATH
environmental variables.
Lastly, verify that JDK12 is installed correctly by executing the command java -version
C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures>java -version openjdk version "12.0.1" 2019-04-16 OpenJDK Runtime Environment (build 12.0.1+12) OpenJDK 64-Bit Server VM (build 12.0.1+12, mixed mode, sharing) C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures>
3.2 Install Eclipse 4.11
In this step, I will install Eclipse 4.11 which supports JDK 12 with the following steps.
First, download eclipse-inst-win64.exe
from https://www.eclipse.org/downloads/ and run the installer.
Secondly, launch Eclipse after the installation is completed, then click Help->Install New Software...
to install “Java 12 Support for Eclipse 2019-03 (4.11)“.
Lastly, configure the JDK 12 as the installed JRE.
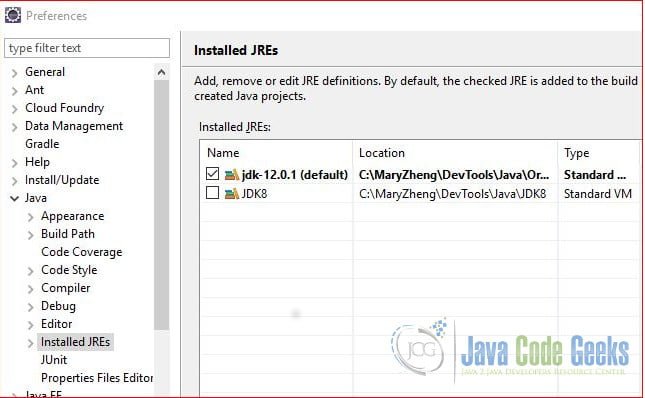
3.3 Maven Project
Create a new Maven project and configure its compiler level to Java 12 and check the “Enable preview features” checkbox.
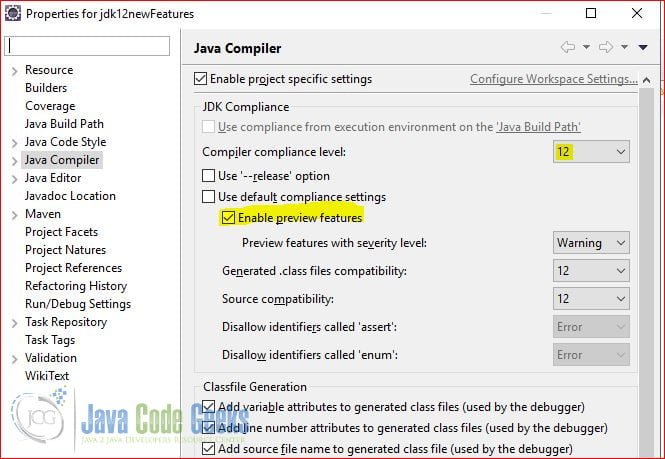
Set up the Java 12 and enable the preview feature in the pom.xml file.
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org-jcg-zheng-demo</groupId> <artifactId>jdk12newFeatures</artifactId> <version>0.0.1-SNAPSHOT</version> <properties> <maven.compiler.target>12</maven.compiler.target> <maven.compiler.source>12</maven.compiler.source> <argLine>--enable-preview</argLine> </properties> <build> <sourceDirectory>src</sourceDirectory> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.8.0</version> <configuration> <release>12</release> <compilerArgs> <arg>--enable-preview</arg> </compilerArgs> </configuration> </plugin> </plugins> </build> <dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>4.12</version> </dependency> </dependencies> </project>
4. Changes in String
The java.lang.String
class added two new methods in JDK12:
indent()
: adds a number of leading white-space characters to the String. If the parameter is negative, that number of leading white-space characters will be removed (if possible).transform()
: applies the provided function to the String. The result does not need to be a String.
In this step, I will create a Junit test class to demonstrate how to use the indent
method to print out a message with 5 white-spaces indented and remove the leading 2 white-spaces. I will also demonstrate how to use the transform
method to convert a string value into a Name
object.
StringTest.java
package org.jcg.zheng.demo; import static org.junit.Assert.assertEquals; import java.util.function.Function; import org.junit.Test; class Name { private String firstName; private String lastName; public Name(String firstName, String lastName) { super(); this.firstName = firstName; this.lastName = lastName; } @Override public String toString() { return "Name [firstName=" + firstName + ", lastName=" + lastName + "]"; } } public class StringTest { @Test public void test_indent_postive() { String message = "This is some text message"; System.out.println(message); String indentedStr = message.indent(5); System.out.println(indentedStr); } @Test public void test_indent_nagative() { String str = " first line\n 2nd line\n 3rd line"; System.out.println(str); String indentedStr = str.indent(-2); System.out.println("-- negatively indented string --"); System.out.println(indentedStr); } @Test public void test_transform() { String str = "100"; Integer integer = str.transform(Integer::parseInt); assertEquals(100, integer.intValue()); } @Test public void test_transform_pojo() { Function convertName = str -> { int i = str.indexOf(" "); String firstNm = str.substring(0, i); String lastNm = str.substring(i + 1); Name nm = new Name(firstNm, lastNm); return nm; }; String str = "Mary Zheng"; Name toName = str.transform(convertName); assertEquals("Name [firstName=Mary, lastName=Zheng]", toName.toString()); } }
Execute the Junit tests and capture the output as the following:
C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures>mvn test -Dtest=StringTest [INFO] Scanning for projects... [INFO] [INFO] ----------------< org-jcg-zheng-demo:jdk12newFeatures >----------------- [INFO] Building jdk12newFeatures 0.0.1-SNAPSHOT [INFO] --------------------------------[ jar ]--------------------------------- [INFO] [INFO] --- maven-resources-plugin:2.6:resources (default-resources) @ jdk12newFeatures --- [WARNING] Using platform encoding (Cp1252 actually) to copy filtered resources, i.e. build is platform dependent! [INFO] skip non existing resourceDirectory C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures\src\main\resources [INFO] [INFO] --- maven-compiler-plugin:3.8.0:compile (default-compile) @ jdk12newFeatures --- [INFO] Changes detected - recompiling the module! [WARNING] File encoding has not been set, using platform encoding Cp1252, i.e. build is platform dependent! [INFO] Compiling 5 source files to C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures\target\classes [INFO] /C:/MaryZheng/Workspaces/jdk12/jdk12newFeatures/src/test/java/org/jcg/zheng/demo/preview/SwitchTest.java: C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures\src\test\java\org\jcg\zheng\demo\preview\SwitchTest.java uses preview language features. [INFO] /C:/MaryZheng/Workspaces/jdk12/jdk12newFeatures/src/test/java/org/jcg/zheng/demo/preview/SwitchTest.java: Recompile with -Xlint:preview for details. [INFO] [INFO] --- maven-resources-plugin:2.6:testResources (default-testResources) @ jdk12newFeatures --- [WARNING] Using platform encoding (Cp1252 actually) to copy filtered resources, i.e. build is platform dependent! [INFO] skip non existing resourceDirectory C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures\src\test\resources [INFO] [INFO] --- maven-compiler-plugin:3.8.0:testCompile (default-testCompile) @ jdk12newFeatures --- [INFO] Nothing to compile - all classes are up to date [INFO] [INFO] --- maven-surefire-plugin:2.12.4:test (default-test) @ jdk12newFeatures --- [INFO] Surefire report directory: C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures\target\surefire-reports ------------------------------------------------------- T E S T S ------------------------------------------------------- Running org.jcg.zheng.demo.StringTest first line 2nd line 3rd line -- negatively indented string -- first line 2nd line 3rd line This is some text message This is some text message Tests run: 4, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.19 sec Results : Tests run: 4, Failures: 0, Errors: 0, Skipped: 0 [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 9.986 s [INFO] Finished at: 2019-05-08T21:14:17-05:00 [INFO] ------------------------------------------------------------------------ C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures>
5. Changes in NumberFormat
The java.text.NumberFormat
class has a new enum – NumberFormat.Style and new subclass – CompactNumberFormat
which will format a decimal number in a compact form. An example of a short compact form would be writing 1000000 as 1M
; writing 1000 as 1K
.
In this step, I will create a Junit class to demonstrate how to format “1000” and “1000000” as “1 thousand”, “1 million”, “1K”, and “1M”.
CompactNumberFormatTest.java
package org.jcg.zheng.demo; import static org.junit.Assert.*; import java.text.NumberFormat; import java.util.Locale; import org.junit.Test; public class CompactNumberFormatTest { @Test public void test_default() { NumberFormat fmt = NumberFormat.getCompactNumberInstance(); String formatedNumber = fmt.format(1000); assertEquals("1K", formatedNumber); } @Test public void test_short_format_1k() { NumberFormat fmt = NumberFormat.getCompactNumberInstance(Locale.US, NumberFormat.Style.SHORT); String formatedNumber = fmt.format(1000); assertEquals("1K", formatedNumber); } @Test public void test_short_format_1m() { NumberFormat fmt = NumberFormat.getCompactNumberInstance(Locale.US, NumberFormat.Style.SHORT); String formatedNumber = fmt.format(1000000); assertEquals("1M", formatedNumber); } @Test public void test_long_format_1m() { NumberFormat fmt = NumberFormat.getCompactNumberInstance(Locale.US, NumberFormat.Style.LONG); String formatedNumber = fmt.format(1000000); assertEquals("1 million", formatedNumber); } @Test public void test_long_format_1k() { NumberFormat fmt = NumberFormat.getCompactNumberInstance(Locale.US, NumberFormat.Style.LONG); String formatedNumber = fmt.format(1000); assertEquals("1 thousand", formatedNumber); } }
Execute the Junit tests and capture the output as the following:
C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures>mvn test -Dtest=CompactNumberFormatTest [INFO] Scanning for projects... [INFO] [INFO] ----------------< org-jcg-zheng-demo:jdk12newFeatures >----------------- [INFO] Building jdk12newFeatures 0.0.1-SNAPSHOT [INFO] --------------------------------[ jar ]--------------------------------- [INFO] [INFO] --- maven-resources-plugin:2.6:resources (default-resources) @ jdk12newFeatures --- [WARNING] Using platform encoding (Cp1252 actually) to copy filtered resources, i.e. build is platform dependent! [INFO] skip non existing resourceDirectory C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures\src\main\resources [INFO] [INFO] --- maven-compiler-plugin:3.8.0:compile (default-compile) @ jdk12newFeatures --- [INFO] Changes detected - recompiling the module! [WARNING] File encoding has not been set, using platform encoding Cp1252, i.e. build is platform dependent! [INFO] Compiling 5 source files to C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures\target\classes [INFO] /C:/MaryZheng/Workspaces/jdk12/jdk12newFeatures/src/test/java/org/jcg/zheng/demo/preview/SwitchTest.java: C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures\src\test\java\org\jcg\zheng\demo\preview\SwitchTest.java uses preview language features. [INFO] /C:/MaryZheng/Workspaces/jdk12/jdk12newFeatures/src/test/java/org/jcg/zheng/demo/preview/SwitchTest.java: Recompile with -Xlint:preview for details. [INFO] [INFO] --- maven-resources-plugin:2.6:testResources (default-testResources) @ jdk12newFeatures --- [WARNING] Using platform encoding (Cp1252 actually) to copy filtered resources, i.e. build is platform dependent! [INFO] skip non existing resourceDirectory C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures\src\test\resources [INFO] [INFO] --- maven-compiler-plugin:3.8.0:testCompile (default-testCompile) @ jdk12newFeatures --- [INFO] Nothing to compile - all classes are up to date [INFO] [INFO] --- maven-surefire-plugin:2.12.4:test (default-test) @ jdk12newFeatures --- [INFO] Surefire report directory: C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures\target\surefire-reports ------------------------------------------------------- T E S T S ------------------------------------------------------- Running org.jcg.zheng.demo.CompactNumberFormatTest Tests run: 5, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.136 sec Results : Tests run: 5, Failures: 0, Errors: 0, Skipped: 0 [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 9.738 s [INFO] Finished at: 2019-05-08T21:23:37-05:00 [INFO] ------------------------------------------------------------------------ C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures>
6. Changes in Collectors
The java.util.stream.Collectors
interface has a new teeing
method which takes two Collectors
and one BiFunction
to return a new Collector
. It’s useful to process a single stream with two collectors and then merge them into one result.
Here is the syntax:
public static <T,R1,R2,R > Collector <T,?,R > teeing(Collector <? super T,?,R1 > downstream1, Collector <? super T,?,R2 > downstream2, BiFunction <? super R1,? super R2,R > merger)
In this step, I will create a Junit test class to demonstrate how to find two lists: one contains "Zheng"
, the other contains "Mary"
from the same list of names. I will also demonstrate how to find the minimum and maximum integer from a list.
CollectorTeeingTest.java
package org.jcg.zheng.demo; import static org.junit.Assert.assertEquals; import java.util.Comparator; import java.util.List; import java.util.function.BiFunction; import java.util.stream.Collector; import java.util.stream.Collectors; import java.util.stream.Stream; import org.junit.Test; public class CollectorTeeingTest { @Test public void filter_two_list() { List<List<String>> twoLists = Stream .of("Mary Zheng", "Alex Zheng", "Java Code Geeks", "Allen Zheng", "Software Developer", "Mary Johnshon") .collect(Collectors.teeing(filterList("Zheng"), filterList("Mary"), mergeTwoList())); assertEquals(3, twoLists.get(0).size()); assertEquals(2, twoLists.get(1).size()); } private Collector<String, ?, List<String>> filterList(String matchingStr) { return Collectors.filtering(n -> n.contains(matchingStr), Collectors.toList()); } private BiFunction<List<String>, List<String>, List<List<String>>> mergeTwoList() { return (List<String> list1, List<String> list2) -> List.of(list1, list2); } @Test public void find_min_max_with_teeing() { var minMaxList = Stream.of(1, 3, 6, 7, 12) .collect(Collectors.teeing(Collectors.minBy(Comparator.comparing(Integer::intValue)), Collectors.maxBy(Comparator.comparing(Integer::intValue)), List::of)); assertEquals(1, minMaxList.get(0).get().intValue()); assertEquals(12, minMaxList.get(1).get().intValue()); } }
Execute the Junit tests and capture the output as the following:
C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures>mvn test -Dtest=CollectorTeeingTest [INFO] Scanning for projects... [INFO] [INFO] ----------------< org-jcg-zheng-demo:jdk12newFeatures >----------------- [INFO] Building jdk12newFeatures 0.0.1-SNAPSHOT [INFO] --------------------------------[ jar ]--------------------------------- [INFO] [INFO] --- maven-resources-plugin:2.6:resources (default-resources) @ jdk12newFeatures --- [WARNING] Using platform encoding (Cp1252 actually) to copy filtered resources, i.e. build is platform dependent! [INFO] skip non existing resourceDirectory C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures\src\main\resources [INFO] [INFO] --- maven-compiler-plugin:3.8.0:compile (default-compile) @ jdk12newFeatures --- [INFO] Changes detected - recompiling the module! [WARNING] File encoding has not been set, using platform encoding Cp1252, i.e. build is platform dependent! [INFO] Compiling 5 source files to C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures\target\classes [INFO] /C:/MaryZheng/Workspaces/jdk12/jdk12newFeatures/src/test/java/org/jcg/zheng/demo/preview/SwitchTest.java: C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures\src\test\java\org\jcg\zheng\demo\preview\SwitchTest.java uses preview language features. [INFO] /C:/MaryZheng/Workspaces/jdk12/jdk12newFeatures/src/test/java/org/jcg/zheng/demo/preview/SwitchTest.java: Recompile with -Xlint:preview for details. [INFO] [INFO] --- maven-resources-plugin:2.6:testResources (default-testResources) @ jdk12newFeatures --- [WARNING] Using platform encoding (Cp1252 actually) to copy filtered resources, i.e. build is platform dependent! [INFO] skip non existing resourceDirectory C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures\src\test\resources [INFO] [INFO] --- maven-compiler-plugin:3.8.0:testCompile (default-testCompile) @ jdk12newFeatures --- [INFO] Nothing to compile - all classes are up to date [INFO] [INFO] --- maven-surefire-plugin:2.12.4:test (default-test) @ jdk12newFeatures --- [INFO] Surefire report directory: C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures\target\surefire-reports ------------------------------------------------------- T E S T S ------------------------------------------------------- Running org.jcg.zheng.demo.CollectorTeeingTest Tests run: 2, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.204 sec Results : Tests run: 2, Failures: 0, Errors: 0, Skipped: 0 [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 9.681 s [INFO] Finished at: 2019-05-08T21:25:23-05:00 [INFO] ------------------------------------------------------------------------ C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures>
7. Support Unicode 11
JDK 12 supports Unicode 11 which includes chess symbols, Chinese, emoji characters etc. In this step, I will create a Junit test to print out the chess symbols.
Unicode11Test.java
package org.jcg.zheng.demo; import org.junit.Test; public class Unicode11Test { private static final String BLACK_BISHOP = "\u265D"; private static final String BLACK_KING = "\u265A"; private static final String BLACK_KNIGHT = "\u265E"; private static final String BLACK_PAWN = "\u265F"; private static final String BLACK_QUEEN = "\u265B"; private static final String BLACK_ROOK = "\u265C"; private static final String WHITE_BISHOP = "\u2657"; private static final String WHITE_KING = "\u2654"; private static final String WHITE_KNIGHT = "\u2658"; private static final String WHITE_PAWN = "\u2659"; private static final String WHITE_QUEEN = "\u2655"; private static final String WHITE_ROOK = "\u2656"; @Test public void test_chess_symbol() { System.out.println("Chess Symbol:"); System.out .println("white: " + WHITE_KING + WHITE_QUEEN + WHITE_ROOK + WHITE_BISHOP + WHITE_KNIGHT + WHITE_PAWN); System.out .println("black: " + BLACK_KING + BLACK_QUEEN + BLACK_ROOK + BLACK_BISHOP + BLACK_KNIGHT + BLACK_PAWN); } }
Execute the Junit tests in Eclipse and capture the output as the following:
Chess Symbol: white: ♔♕♖♗♘♙ black: ♚♛♜♝♞♟
8. Preview – Switch Expression
JDK12 introduces the preview language feature which provides a way of including beta version of new features. The preview language features are not included in the Java SE specification.
Preview feature – raw string literals (JEP 326) is removed from JDK 12 general release. It may be restored in JDK 13.
Preview feature – switch expressions (JEP 325) is included in JDK 12 general release. JEP 325 enhances the switch
statement to be used as both statement or expression.
Prior to JDK 12, switch
was a statement. in JDK 12, it has become an expression which evaluates the contents of the switch
to produce a result. It also supports the comma separated list on the case
statement to make the code looks clearer and cleaner.
SwitchTest.java
package org.jcg.zheng.demo.preview; import static org.junit.Assert.assertEquals; import org.junit.Test; public class SwitchTest { @Test public void test_Switch_statement() { Day day = Day.FRIDAY; switch (day) { case MONDAY, TUESDAY -> System.out.println("Back to work."); case WEDNESDAY -> System.out.println("Wait for the end of week..."); case THURSDAY, FRIDAY -> System.out.println("Plan for the weekend?"); case SATURDAY, SUNDAY -> System.out.println("Enjoy the holiday!"); } } @Test public void test_Switch_expression_2() { Day day = Day.FRIDAY; String message = switch (day) { case MONDAY, TUESDAY -> "Back to work."; case WEDNESDAY -> "Wait for the end of week..."; case THURSDAY, FRIDAY -> "Plan for the weekend?"; case SATURDAY, SUNDAY -> "Enjoy the holiday!"; default -> throw new IllegalArgumentException("Seriously?!"); }; assertEquals("Plan for the weekend?", message); } @Test public void test_switch_before_jdk12() { int nameLetterCounts = 0; String testName = "MARY"; switch (testName) { case "MARY": nameLetterCounts = 4; break; case "ZHENG": nameLetterCounts = 5; break; } assertEquals(4, nameLetterCounts); } } enum Day { MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY }
Execute the Junit tests and capture the output as the following:
C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures>mvn test -Dtest=SwitchTest [INFO] Scanning for projects... [INFO] [INFO] ----------------< org-jcg-zheng-demo:jdk12newFeatures >----------------- [INFO] Building jdk12newFeatures 0.0.1-SNAPSHOT [INFO] --------------------------------[ jar ]--------------------------------- [INFO] [INFO] --- maven-resources-plugin:2.6:resources (default-resources) @ jdk12newFeatures --- [WARNING] Using platform encoding (Cp1252 actually) to copy filtered resources, i.e. build is platform dependent! [INFO] skip non existing resourceDirectory C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures\src\main\resources [INFO] [INFO] --- maven-compiler-plugin:3.8.0:compile (default-compile) @ jdk12newFeatures --- [INFO] Changes detected - recompiling the module! [WARNING] File encoding has not been set, using platform encoding Cp1252, i.e. build is platform dependent! [INFO] Compiling 5 source files to C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures\target\classes [INFO] /C:/MaryZheng/Workspaces/jdk12/jdk12newFeatures/src/test/java/org/jcg/zheng/demo/preview/SwitchTest.java: C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures\src\test\java\org\jcg\zheng\demo\preview\SwitchTest.java uses preview language features. [INFO] /C:/MaryZheng/Workspaces/jdk12/jdk12newFeatures/src/test/java/org/jcg/zheng/demo/preview/SwitchTest.java: Recompile with -Xlint:preview for details. [INFO] [INFO] --- maven-resources-plugin:2.6:testResources (default-testResources) @ jdk12newFeatures --- [WARNING] Using platform encoding (Cp1252 actually) to copy filtered resources, i.e. build is platform dependent! [INFO] skip non existing resourceDirectory C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures\src\test\resources [INFO] [INFO] --- maven-compiler-plugin:3.8.0:testCompile (default-testCompile) @ jdk12newFeatures --- [INFO] Nothing to compile - all classes are up to date [INFO] [INFO] --- maven-surefire-plugin:2.12.4:test (default-test) @ jdk12newFeatures --- [INFO] Surefire report directory: C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures\target\surefire-reports ------------------------------------------------------- T E S T S ------------------------------------------------------- Running org.jcg.zheng.demo.preview.SwitchTest Plan for the weekend? Tests run: 3, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.125 sec Results : Tests run: 3, Failures: 0, Errors: 0, Skipped: 0 [INFO] ------------------------------------------------------------------------ [INFO] BUILD SUCCESS [INFO] ------------------------------------------------------------------------ [INFO] Total time: 9.495 s [INFO] Finished at: 2019-05-08T21:27:37-05:00 [INFO] ------------------------------------------------------------------------ C:\MaryZheng\Workspaces\jdk12\jdk12newFeatures>
9. Summary
In this tutorial, I demonstrated five API changes in Java 12:
- The
java.lang.String
class – new indent and transform methods - The
java.text.NumberFormat
class – new format styles - The
java.util.stream.Collectors
– new teeing method - The preview feature –
switch
- Unicode support, including Chess symbols
Please visit Oracle web site for more more changes in Java 12. Java 13 is planned to release on September 2019.
10. Download the Source Code
This was a Java 12 new features tutorial.
You can download the full source code of this example here: Java 12 New Features Tutorial