How to Create and Run Your First Java Program
1. Introduction
This is an in-depth article on how to create and run your first java program. Java compiler is used to compile java code. Java class is the output of the compilation. To execute the program you need java runtime virtual machine.
2. Run Your First Java Program
2.1 Prerequisites
Java 7 or 8 is required on the linux, windows or mac operating system.
2.2 Download
You can download Java 7 from Oracle site. On the other hand, You can use Java 8. Java 8 can be downloaded from the Oracle web site .
2.3 Setup
You can set the environment variables for JAVA_HOME and PATH. They can be set as shown below:
Environmental Variables setup
JAVA_HOME=”/desktop/jdk1.8.0_73″ export JAVA_HOME PATH=$JAVA_HOME/bin:$PATH export PATH
2.4 First Program
2.4.1 Checking the running of the program
You need to create a java class "FirstJavaProgram"
. The class needs to have static method "main"
which takes string array as the arguments. The first program in java is shown below. It prints “Checking First java program”.
First Java Program
public class FirstJavaProgram { public static void main(String[] args) { System.out.println("checking First java "); } }
The command below executes the above code snippet:
Run Command
javac FirstJavaProgram.java java FirstJavaProgram
The output of the executed command is shown below.
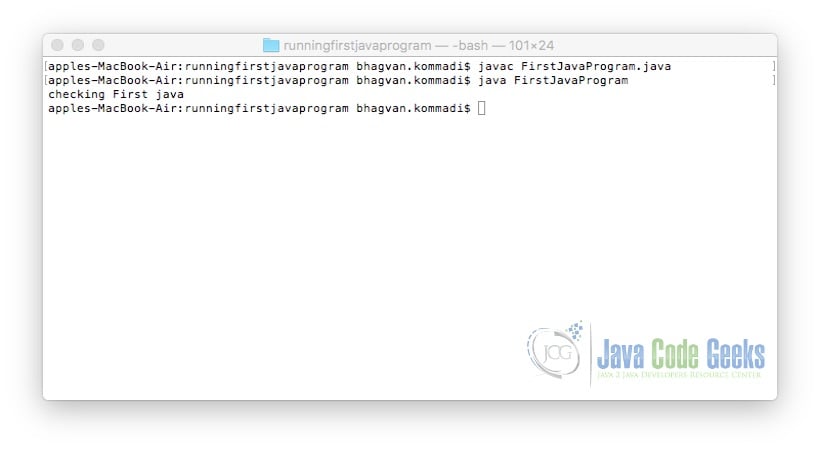
2.4.2 Command Line Arguments
You can pass the command line arguments to the java program. The static method "main"
has the string array which will have the command line arguments. The code is modified to handle command line arguments.
JavaProgramArguments
public class JavaProgramArguments { public static void main(String[] args) { System.out.println("checking the arguments "); int i=0; for(String arg: args) { i++; System.out.println("argument "+i+" value "+ arg); } } }
The command below executes the above code snippet:
Run Command
javac JavaProgramArguments.java java JavaProgramArguments 11 22 33
The output of the executed command is shown below.
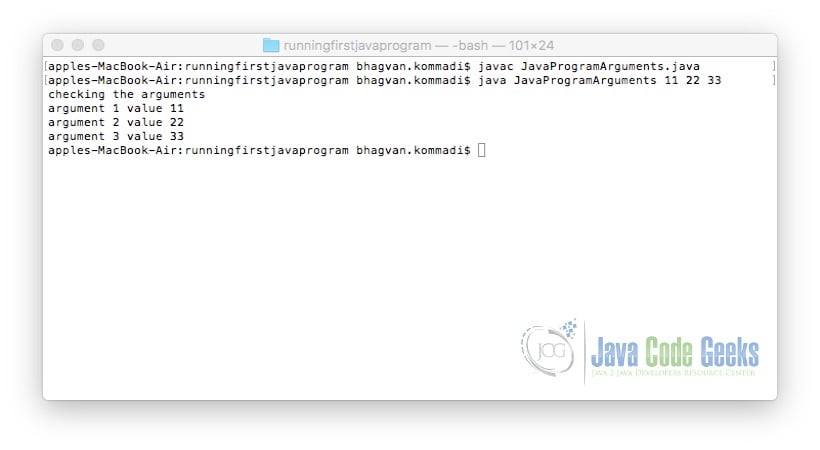
2.4.3 Java Class with Constructor
The java program can have a constructor. The "Program"
class can have a public method "output"
. In the "main"
method, program is instantiated and the method on the object is invoked. The code is shown below.
Program Class
public class Program { public Program() { } public void output(String value) { System.out.println(value); } public static void main(String[] args) { Program program = new Program(); program.output("checking class with constructor"); } }
The command below executes the above code snippet:
Run Command
javac Program.java java Program
The output of the executed command is shown below.
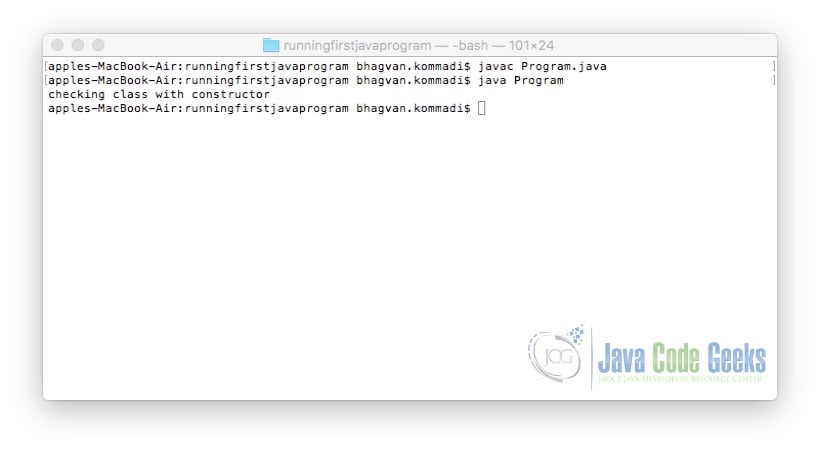
2.4.4 Java Class with setter and getter
You can create a java class Person
which has id as a private member. It can have a default constructor with setter and getter methods for "id"
. The code for the java class is shown below.
Person Class
public class Person { private String id; public Person() { } public String getId() { return id; } public void setId(String id) { this.id = id; } public static void main(String[] args) { Person person = new Person(); person.setId("34567"); System.out.println("Person's id" + person.getId()); } }
The command below executes the above code snippet:
Run Command
javac Person.java java Person
The output of the executed command is shown below.
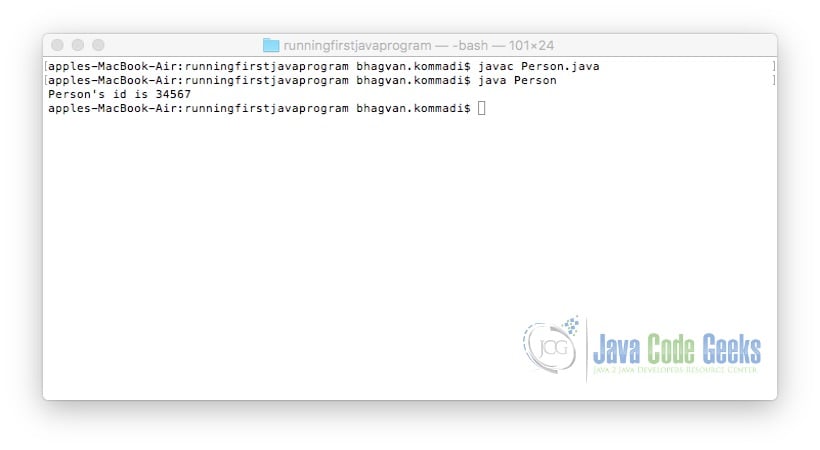
2.5 Error Handling
Errors can happen during compilation or runtime. Compile time errors happen during the compilation of the program. Run-time errors can happen when you run the program. Logical errors are related to the code and the wrong results.
2.6 Input
You can use the "java.util.Scanner"
class to input values to the java program. The "java.util.Scanner"
has methods to handle long, float, double and String types. The methods are "nextLong()"
, "nextFloat()"
, "nextDouble()"
and "next()"
. The code below shows how "java.util.Scanner"
class can be used.
Java Input
import java.util.Scanner; public class JavaInput { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.print("Enter a number: "); double number = scanner.nextDouble(); System.out.println("The number inputted is " + number); } }
The command below executes the above code snippet:
Run Command
javac JavaInput.java java JavaInput
The output of the executed command is shown below.
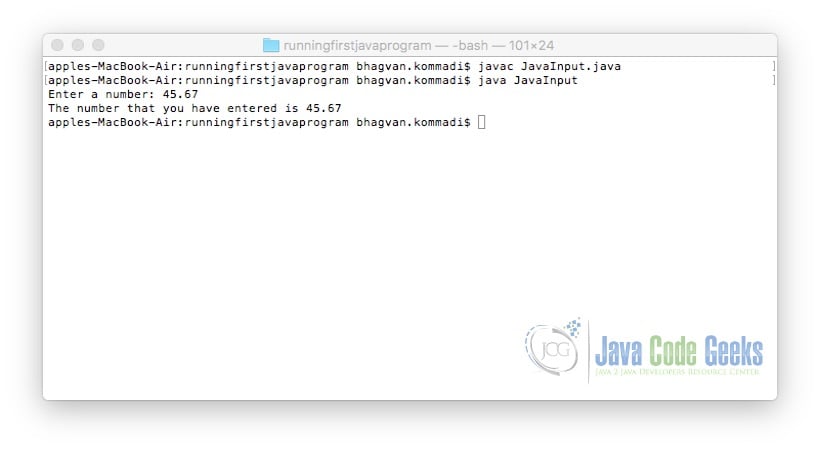
2.7 Expressions
You can use expressions in the code. Expressions are variables, operators, literals and method calls. They evaluate to one value. The sample code is shown below
Expressions
public class JavaExpressions { public static void main(String[] args) { double number; number = 65.0; System.out.println(number); double newNumber; newNumber = 45.0; if(newNumber < number) { System.out.println("45 is less than 65"); } } }
The command below executes the above code snippet:
Run Command
javac JavaExpressions.java java JavaExpressions
The output of the executed command is shown below.
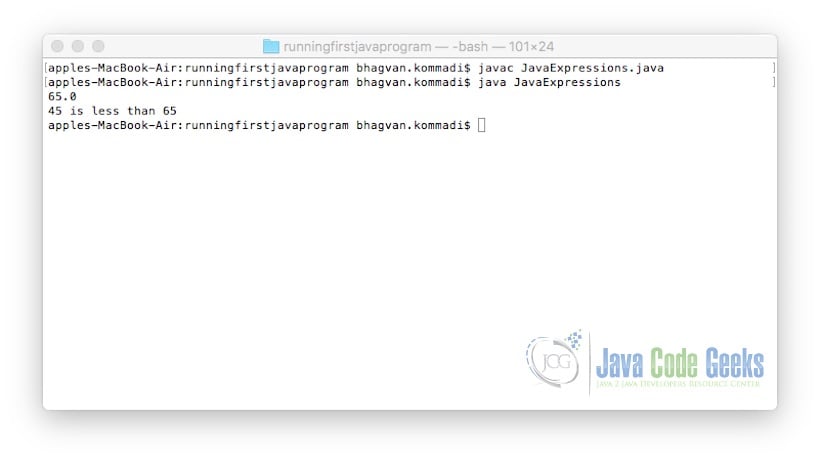
2.8 Blocks
A block of code consists of one or more statements. These statements are enclosed in curly braces { } in if condition, for, do-while and while loops.
2.9 Comments
In the program, you can add comments at the class level and the methods. You can use block comments or single line comments. The suggested best practices for commenting code can be accessed at the oracle website.
start command
/** Person class */ public class Person { // id property private String id; /** * default constructor */ public Person() { } /** * getter method for Id */ public String getId() { return id; } /** * setter method for Id */ public void setId(String id) { this.id = id; } /** * static method main */ public static void main(String[] args) { Person person = new Person(); person.setId("34567"); System.out.println("Person's id is " + person.getId()); } }
3. Download the Source Code
You can download the full source code of this example here: How to Create and Run Your First Java Program