Java DecimalFormat Example
In this example, we will see how we can use the Java DecimalFormat class to format decimal numbers.
1. Java DecimalFormat – Introduction
 This class is useful when we want to format the decimal numbers in the following ways:
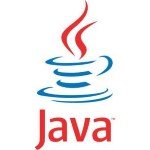
- As per a defined specific string pattern: We can specify a pattern string to define the display of the decimal.
- Specific to a locale: We can use locale-specific settings to control the application of patterns for the display of decimals.
2. Technologies used
The example code in this article was built and run using:
- Java 1.8.231(1.8.x will do fine)
- Eclipse IDE for Enterprise Java Developers-Photon
3. Available characters for the pattern
Here you can see the available character that you can use inside the patterns.
Symbol | Meaning |
0 | Digit |
# | Digit, zero shows as absent |
. | Decimal separator |
% | Multiply by 100 and show as a percentage |
, | Grouping separator |
; | Separates negative and positive subpatterns |
\ | Multiply by 1000 and show as per mille value |
¤ | Currency sign, replaced by currency symbol. If doubled, replaced by the international currency symbol. |
‘ | Used to quote special characters in a prefix or suffix. |
E | Separates mantissa and exponent in scientific notation. |
– | Minus sign |
4. Using a decimal format java pattern string
import java.text.DecimalFormat; public class JavaDecimalFormatExample { private static final String COMMA_SEPERATED = "###,###.###"; private static double number = 12345.6; public static void main(String[] args) { DecimalFormat decimalFormat = new DecimalFormat(COMMA_SEPERATED); System.out.println(decimalFormat.format(number)); } }
Output
1 | 12,345.6 |
In the above example we have defined a String PATERN which is passed as a parameter to the constructor of class DecimalFormat
. Then we used the method format
, in which we passed the decimal and it returned the desired formatted decimal string.
We can change this pattern later by using the method applyPattern()
.
Lets suppose we want show the number with two decimal places. Lets see an example of how to do it:
1 2 3 4 5 | ... private static final String TWO_DECIMAL_PLACES_WITH_COMMA = "###,###.00" ; System.out.println( "After another pattern" ); decimalFormat.applyPattern(TWO_DECIMAL_PLACES_WITH_COMMA); System.out.println(decimalFormat.format(number)); |
Output
1 2 | After another pattern 12,345.60 |
So, different patterns can be used to achieve different patterns, below are a few examples:
import java.text.DecimalFormat; public class JavaDecimalFormatExample { private static final String FIXED_PLACES = "000,000.00"; private static final String BEGIN_WITH_DOLLAR = "$###,###.00"; private static double number = 12345.6; public static void main(String[] args) { applyCustomFormat(BEGIN_WITH_DOLLAR, number); applyCustomFormat(FIXED_PLACES, number); } private static void applyCustomFormat(String pattern, double value) { DecimalFormat decimalFormat = new DecimalFormat(pattern); decimalFormat.applyPattern(pattern); System.out.println(decimalFormat.format(value)); } }
Output
1 2 | $12,345.60 012,345.60 |
5. Using locale-specific pattern
In the previous examples we created a DecimalFormat for the default Locale of the JVM, where the code is running. In case we want to display the numbers as per the locale, we would create an object of the class NumberFormat
passing it the locale and then cast it to DecimalFormat
.
Lets see an example:
1 2 3 4 5 | ..... Locale UK_LOCALE = new Locale( "en" , "UK" ); Locale US_LOCALE = new Locale( "en" , "US" ); applyCustomFormat(COMMA_SEPERATED, number, UK_LOCALE); applyCustomFormat(BEGIN_WITH_DOLLAR, number, US_LOCALE); |
1 2 3 4 5 | private static void applyCustomFormat(String pattern, double value, Locale locale) { DecimalFormat decimalFormat = (DecimalFormat) NumberFormat.getNumberInstance(locale); System.out.println(decimalFormat.format(value)); } |
Output
1 2 | 12,345.6 12,345.6 |
Here, the system locale is set to UK so the output is been formatted as per the UK locale.
There are few other methods and scenarios which can be useful, lets see them :
5.1 Grouping
We can group the digits using the method setGroupingSize(integer
). This method groups the numbers starting from the decimal.
Lets see an example:
1 2 3 4 5 6 | ... //setting group DecimalFormat groupeddecimalFormat = new DecimalFormat( "###,###.###" ); groupeddecimalFormat.setGroupingSize( 4 ); System.out.println(groupeddecimalFormat.format( 13243534.32 )); .... |
Output
1 | 13,243,534.32 |
In the above example we can see that the large number is grouped into 4 integers, also observe that the pattern applied is overwritten by the group set method.
6. Example for DecimalFormatSymbols
DecimalFormatSymbols is an object from the DecimalFormat class that is used to change any of the available symbols that we use for patterns to another symbol if needed.
Here you can see an example about DecimalFormatSymbols.
import java.text.DecimalFormatSymbols; public class DFSymbols { public static void main(String[] args) { DecimalFormatSymbols dfsymbol=new DecimalFormatSymbols(); System.out.println("Decimal separator current character:" + dfsymbol.getDecimalSeparator()); char decSep = '*'; dfsymbol.setDecimalSeparator(decSep); System.out.println("The updated character:"+ dfsymbol.getDecimalSeparator()); } }
Output
Decimal separator current character:. The updated character:*
7. Download the source code
You can download the full source code of this example here: Java DecimalFormat Example
Last updated on Apr. 23rd, 2020