Java Date Format Example
In this article, we will check the options available on Java Date Format. We are going to create a Java date formatter example.
1. Introduction
Java has multiple packages providing various utility functions to make developer’s job easier. One such is the java.text package which includes utility classes for parsing and formatting numbers and dates, along with utility classes for building other kinds of parsers. The java.text.DateFormat
class and its concrete subclass java.text.SimpleDateFormat
provide a convenient way to convert strings with the date and/or time info to and from java.util.Date
objects.
You can also check the Java Date and Calendar Tutorial in the following video:
2. Java Date Format
This is an abstract class used to provide an interface over most of the date related utilities. The most common implementation is SimpleDateFormat
. The way we initialize the class is by calling the method as below
Before understanding the various ways of initializing, we can look at three different flags which control the display format of date and time
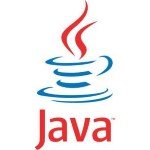
- SHORT – This displays the time or date in the shortest form visible. For example, Time is displayed as 10:14 pm while the Date is displayed as 22/6/19
- MEDIUM – This is the default mode of display. In this mode, Time is displayed as 10:14:53 pm while the Date is displayed as 22 Jun, 2019
- LONG – This displays the time or date in the clearest form available. For example, Time is displayed as 10:14:53 PM IST including the timezone while the Date is displayed as 22 June, 2019. In Medium, Month is abbreviated to 3 characters while here it is expanded.
- FULL – This involves no change for Time from LONG but the Date is displayed as Saturday, 22 June, 2019 including the week of the day.
Date Initialization
DateFormat format = DateFormat.getInstance(); DateFormat dateTimeFormat = DateFormat.getDateTimeInstance(); DateFormat timeFormat = DateFormat.getTimeInstance();
- The first one initializes both the date and time instance with value as
SHORT
- The second one initializes both the date and time instance with value as
MEDIUM
- The final one initializes only the time instance with value as
MEDIUM
We can see the result by running the example which might make it clear
Date With default format
Date now = new Date(); System.out.println(" String version of Date. " + now.toString()); System.out.println(" DateTimeWithShort. " + DateFormat.getInstance().format(now)); System.out.println(" TimeWithMedium. " + DateFormat.getTimeInstance().format(now)); System.out.println(" DateTimeWithMedium. " + DateFormat.getDateTimeInstance().format(now));
This produces the following output
String version of Date. Sat Jun 22 22:32:24 IST 2019 DateTimeWithShort. 22/6/19 10:32 PM TimeWithMedium. 10:32:24 PM DateTimeWithMedium. 22 Jun, 2019 10:32:24 PM
The format takes in a date parameter which can be any valid date. For our example, We have considered the current time using new Date()
. Also we can provide the various flags discussed above as input to the methods getTimeInstance
and getDateTimeInstance
. We will see couple of examples of the same with their corresponding outputs below.
Date With predefined formats
System.out.println("TimeWithShort . " + DateFormat.getTimeInstance(DateFormat.SHORT).format(now)); System.out.println("TimeWithLong. " + DateFormat.getTimeInstance(DateFormat.LONG).format(now)); System.out.println("DTML. " + DateFormat.getDateTimeInstance(DateFormat.MEDIUM, DateFormat.LONG).format(now)); System.out.println("DTMF. " + DateFormat.getDateTimeInstance(DateFormat.MEDIUM, DateFormat.FULL).format(now)); System.out.println("DTLL. " + DateFormat.getDateTimeInstance(DateFormat.LONG, DateFormat.LONG).format(now)); System.out.println("DTLF. " + DateFormat.getDateTimeInstance(DateFormat.LONG, DateFormat.FULL).format(now));
TimeWithShort . 10:43 PM TimeWithLong. 10:43:27 PM IST DTML. 22 Jun, 2019 10:43:27 PM IST DTMF. 22 Jun, 2019 10:43:27 PM IST DTLL. 22 June, 2019 10:43:27 PM IST DTLF. 22 June, 2019 10:43:27 PM IST
The textFormat
package also provides utility for extracting date from a string or other types. Let us see a simple example of parsing date with a string.
Date Parsing
try { DateFormat format = DateFormat.getDateTimeInstance(DateFormat.SHORT,DateFormat.SHORT); Date parsedDate = format.parse("22/6/19 10:43 PM"); Calendar cal = Calendar.getInstance(); cal.setTime(parsedDate); cal.add(Calendar.DATE,5); System.out.println("Date Result. "+cal.getTime().toString()); } catch (ParseException e) { e.printStackTrace(); }
- We are creating
DateFormat
instance withSHORT
flag. - We parse the input string using the
parse
method. - After parsing, the extracted date is set to calendar instance.
- 5 days are added to the original date
- Final result is printed back to the console.
A thing to note is that the date string should adhere to the format pattern specified. If not parse exception is thrown, when the string is being parsed..
3. SimpleDateFormat
In the above example, the format flags specified is very limited and cannot handle a variety of other different formats. Even date.toString()
cannot be handled with the existing format flags. This is where SimpleDateFormat
kicks in and provides other formatting options. The class can be initialized as shown below
Date With default format
Date now = new Date(); String pattern = "G"; DateFormat simpleDateFormat = new SimpleDateFormat(pattern); System.out.println(simpleDateFormat.format(now));
- We specify a pattern format to be used. We can see all the patterns in table below
- In next step, we provide the pattern to be compiled by passing the pattern to an instance of
SimpleDateFormat
. - The final step is formatting the date. Date is displayed based on the string format specified.
The following are the other format specifiers available in SimpleDateFormat
to extend the functionality of the date parsing and formatting.
Pattern (substitute in code above) | Description | Output |
G | Used to specify the era | AD |
y | specifies the year | 2019 |
yy | specifies the year in 2 digit | 19 |
yyyyy | pads with zero for the extra y | 02019 |
M (or) MM | Month specifier in number | 7 (or) 07 (or) 12 |
MMM | Month specifier in abbreviated string | Jul |
MMMM | Full name of the month | July |
m (or) mm | Displays the minute | 09 (or) 43 |
d (or) dd | Displays the day of the month | 7 (or) 07 (or) 23 |
h (or) hh | Displays the hour(1-12) | 5 (or) 05 (or) 11 |
H (or) HH | Displays the hour(0-23) | 17 (or) 01 (or) 1 |
k (or) kk | Displays the hour(1-24) | 1 (or) 01 (or) 24 |
K (or) KK | Displays the hour (0-11) | 1 (or) 01 (or) 11 |
S (or) SS (or) SSS | Millisecond (0-999) | 7 (or) 07 (or) 007 |
E (or) EEEE | Day in Week | Thu (or) Thursday |
D | Day in Year | 178 |
F | Day of Week in number | 1 indicates Monday |
w (or) ww | Week for an year | 26 |
W | Week in month | 5 |
a | AM/PM | AM |
z(or)zzzzz | Display the current time zone | IST (or) Indian Standard Time |
We can follow the entire format specifiers with an example.
Date With Full Custom format
try { DateFormat simpleDateFormat = new SimpleDateFormat("dd MMMM yyyy G hh:mm:SS zzz"); String result = simpleDateFormat.format(now); System.out.println("Date String "+result); System.out.println("ParsedResult "+simpleDateFormat.parse(result)); } catch (ParseException e) { e.printStackTrace(); }
The above displays the date in the format 25 June 2019 AD 05:17:960 IST. We can parse the string date back into original date form. Both of them have been illustrated with above example
In this article, we saw the various ways date can be formatted and also the ways of parsing a string back to date.
4. Download the Source code
You can download the full source code of this example here: Java Date Format Example
Last updated on Aug. 29, 2019
Seriously? An article written five years after the release of java 8 with the new date-time API which talks about the pre java 8 date and time classes?