Java Convert String toUpperCase
With this example, we are going to demonstrate how to convert a String toUpperCase in Java. The String class represents character strings. All string literals in Java programs, such as "abc"
, are implemented as instances of this class.
1. Java Convert String toUpperCase
In short, to convert a String to UpperCase you should:
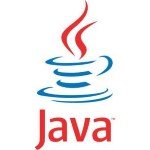
- Create a new String.
- Use
toUpperCase()
API method of String. This method converts all of the characters in this String to upper case using the rules of the default locale. This method is equivalent totoUpperCase(Locale.getDefault())
.
Note that in case you’d like to do the opposite, there is the toLowerCase method. The toLowerCase method works exactly like the toUpperCase, but converts the string to only contain lower-case letters instead of capital letters.
Let’s take a look at the code snippet that follows:
public class ConvertStringToUpperCase { public static void main(String[] args) { String s = "Java Code Geeks"; System.out.println("Original String: " + s); String su = s.toUpperCase(); System.out.println("String to upper case: " + su); } }
Output:
Original String: Java Code Geeks
String to upper case: JAVA CODE GEEKS
Here we just create a String s, then we print it. Afterwards, we create a new string and we assign the value that is returned from toUpperCase method when called by the previously created string. In the end, we print the result of the “su” string. The result is the same string but all the letters are in upper case, exactly what we expected.
2. Download the Source code
This was an example of how to convert a String to UpperCase in Java.
You can download the full source code of this example here: Java Convert String toUpperCase
Last update on May 5th, 2020