Java Bitwise Operators Example
In this post, we feature a comprehensive article about Java Bitwise Operators.
1. Java Bitwise Operators
Bitwise operators compare two variables bit by bit and return a variable whose bits have been set based on whether the two variables being compared had respective bits that were either both “on” (&), one or the other “on” (|), or exactly one “on” (^).
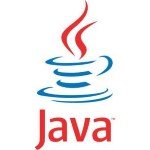
Java defines several bitwise operators, which can be applied to the primitive types like long, int, short, char and byte.
Java provides 4 bitwise and 3 bitshift operators to perform bit operations.
Symbol | Operator | Description |
& | bit-wise AND | If both bits are 1 it gives 1 else gives 0. |
| | bit-wise OR | If either of the bits is 1, it gives 1 else it gives 0. |
^ | bit-wise XOR | If corresponding bits of both operands are different, it gives 1 else it gives 0. |
~ | one’s complement | Inverses all bits, it makes every 0 to 1 and 1 to 0. |
<< | signed left shift | Shifts a bit pattern to the left by certain number of specified bits. |
>> | signed right shift | Shifts a bit pattern to the right by certain number of specified bits. |
>>> | unsigned right shift | The value is moved right by the number of bits specified by the right operand and shifted values are filled by zero. |
2. Examples of bitwise operators
Let us look at an example for each of the bitwise and bitshift operators listed above.
2.1 Example of bitwise AND
The AND (&) operator compares both operands and gives 1 if both bits are 1 and 0 otherwise.
BitwiseANDExample.java
package com.javacodegeeks.basic; public class BitwiseANDExample { public static void main(String[] args) { int a = 5; int b = 7; int result = a & b; System.out.println("Result of a & b is "+result); } }
Output:
Result of a & b is 5
The following is the binary representation of this operation.
5 = 0101 7 = 0111 Bitwise AND operation of 5 and 7 is 0101 & 0111 ---- 0101 = 5 (in decimal) ----
2.2 Example of bitwise OR
The OR (|) operator compares the bits of 2 operands and gives 1 if either of the bit is 1 and 0 otherwise.
BitwiseORExample.java
package com.javacodegeeks.basic; public class BitwiseORExample { public static void main(String[] args) { int a = 5; int b = 7; int result = a | b; System.out.println("Result of a | b is "+result); } }
Output:
Result of a | b is 7
The following is the binary representation of this operation.
5 = 0101 7 = 0111 Bitwise OR operation of 5 and 7 is 0101 | 0111 ---- 0111 = 7 (in decimal) ----
2.3 Example of bitwise XOR
The XOR (^) operator compares the bits of both operands and if both bits are different it gives 1 and 0 otherwise.
BitwiseXORExample.java
package com.javacodegeeks.basic; public class BitwiseXORExample { public static void main(String[] args) { int a = 5; int b = 7; int result = a ^ b; System.out.println("Result of a ^ b is "+result); } }
Output:
Result of a ^ b is 2
The following is the binary representation of this operation.
5 = 0101 7 = 0111 Bitwise XOR operation of 5 and 7 is 0101 ^ 0111 ---- 0010 = 2 (in decimal) ----
2.4 Bitwise complement
The one’s complement (~) operator inverts the bit pattern. It works on only one operand. It makes every 0 to 1 and 1 to 0.
BitwiseComplimentExample.java
package com.javacodegeeks.basic; public class BitwiseComplimentExample { public static void main(String[] args) { int a = 5; int result = ~a; System.out.println("Result of ~a is "+result); } }
Output:
Result of ~a is -6
The following is the binary representation of this operation.
5 = 0101 Bitwise complement operation of 5 is ~ 0101 ---- 1010 = 10 (in decimal) ----
The output of the program is -6 and not 10 because it is printing the 2’s complement of the number which is the negative notation of the binary number.
2.5 Signed Left Shift
The left shift (<<) operator shifts a bit pattern to the left by a certain number of specified bits and fills 0 into the lower order positions.
LeftShiftExample.java
package com.javacodegeeks.basic; public class LeftShiftExample { public static void main(String[] args) { int a =5; int b = -5; System.out.println("Result of a << 1 is "+ (a << 1 )); System.out.println("Result of a << 0 is "+ (a << 0 )); System.out.println("Result of a << 8 is "+ (a << 8 )); System.out.println("Result of b << 1 is "+ (b << 1 )); } }
Output:
Result of a << 1 is 10 Result of a << 0 is 5 Result of a << 8 is 1280 Result of b << 1 is -10
Shifting a number by one is equivalent to multiplying it by 2, or, in general, left shifting a number by n positions is equivalent to multiplication by 2^n
2.6 Signed Right Shift
The right shift(>>) operator shifts a bit pattern to the right by a certain number of specified bits.
RightShiftExample.java
package com.javacodegeeks.basic; public class RightShiftExample { public static void main(String[] args) { int a =5; int b = -5; System.out.println("Result of a >> 1 is "+ (a >> 1 )); System.out.println("Result of a >> 0 is "+ (a >> 0 )); System.out.println("Result of a >> 8 is "+ (a >> 8 )); System.out.println("Result of b >> 1 is "+ (b >> 1 )); } }
Output:
Result of a >> 1 is 2 Result of a >> 0 is 5 Result of a >> 8 is 0 Result of b >> 1 is -3
For the signed right shift, when an input number is negative, where the leftmost bit is 1, then the empty spaces will be filled with 1.
And when an input number is positive, where the leftmost bit is 0, then the empty spaces will be filled with 0
2.7 Unsigned Right Shift
The unsigned right shift(>>>) operator shifts the specified number of bits to the right and the shifted values are filled up with zero irrespective of the leftmost bit is 0 or 1.
UnSignedRightShiftExample.java
package com.javacodegeeks.basic; public class UnSignedRightShiftExample { public static void main(String[] args) { int a =5; int b = -5; System.out.println("Result of a >>> 1 is "+ (a >>> 1 )); System.out.println("Result of a >>> 0 is "+ (a >>> 0 )); System.out.println("Result of a >>> 8 is "+ (a >>> 8 )); System.out.println("Result of b >>> 1 is "+ (b >>> 1 )); } }
Output:
Result of a >>> 1 is 2 Result of a >>> 0 is 5 Result of a >>> 8 is 0 Result of b >>> 1 is 2147483645
For the unsigned right shift, the result will always be a positive integer.
3. Download the source code
This was an example of Java Bitwise Operators.
You can download the full source code of this example here: Java Bitwise Operators Example