Java Bean Example
In this example, we are going to demonstrate how to use and configure the Java Bean.
1. Introduction
In computing based on the Java Platform, JavaBeans are classes that encapsulate many objects into a single object (the bean). They are serializable, have a zero-argument constructor, and allow access to properties using getter and setter methods. The name “Bean” was given to encompass this standard, which aims to create reusable software components for Java.
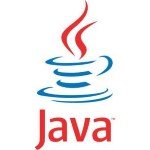
2. JavaBean Features
According to Java white paper, it is a reusable software component. A bean encapsulates many objects into one object so that we can access this object from multiple places. Moreover, it provides easy maintenance.
Features:
1. Introspection – Introspection is a process of analyzing a Bean to determine its capabilities. This is an essential feature of the Java Beans API because it allows another application such as a design tool, to obtain information about a component.
2. Properties – A property is a subset of a Bean’s state. The values assigned to the properties determine the behaviour and appearance of that component. They are set through a setter method and can be obtained by a getter method.
3. Customization – A customizer can provide a step-by-step guide that the process must follow to use the component in a specific context.
4. Events – Beans may interact with the EventObject EventListener model.
5. Persistence – Persistence is the ability to save the current state of a Bean, including the values of a Bean’s properties and instance variables, to nonvolatile storage and to retrieve them at a later time.
6. Methods – A bean should use accessor methods to encapsulate the properties. A bean can provide other methods for business logic not related to the access to the properties.
3. Structure of Java Bean class
Syntax for setter methods:
- It should be public in nature.
- The return-type should be void.
- The setter method should be prefixed with set.
- It should take some argument i.e. it should not be no-arg method.
Syntax for getter methods:
- It should be public in nature.
- The return-type should not be void i.e. according to our requirement we have to give return-type.
- The getter method should be prefixed with get.
- It should not take any argument.
public class TestBean { private String name; //setter method public void setName(String name) { this.name = name; } //getter method public String getName() { return name; } }
For Boolean properties getter method name can be prefixed with either “get” or “is”. But recommended to use “is”.
public class Test { private boolean empty; public boolean getName() { return empty; } public boolean isempty() { return empty; } }
4. JavaBean Example
Before going to write a JavaBean, here are some basic rules. A JavaBean should be public, should has no argument default constructor and should implement serializable interface. Keep these basic rules in mind before writing a JavaBean
The example program shown below demonstrates how to implement JavaBeans.
// Java Program of JavaBean class package com.javacodegeeks.javabean; public class Student implements java.io.Serializable { private int id; private String name; public Student() { } public void setId(int id) { this.id = id; } public int getId() { return id; } public void setName(String name) { this.name = name; } public String getName() { return name; } }
The following program is written in order to access the JavaBean class that we created above:
// Java program to access JavaBean class package com.javacodegeeks.javabean; public class Test { public static void main(String args[]) { Student s = new Student(); // object is created s.setName("JavaCodeGeeks"); // setting value to the object System.out.println(s.getName()); } }
Output
JavaCodeGeeks
5. Advantages and Disadvantages of JavaBean
Pros:
- The properties, events, and methods of a bean can be exposed to another application.
- A bean may register to receive events from other objects and can generate events that are sent to those other objects.
- Auxiliary software can be provided to help configure a bean.
- The configuration settings of a bean can be saved to persistent storage and restored.
Cons:
- A class with a zero-argument constructor is subject to being instantiated in an invalid state. If such a class is instantiated manually by a developer (rather than automatically by some kind of framework), the developer might not realize that the class has been improperly instantiated. The compiler cannot detect such a problem, and even if it is documented, there is no guarantee that the developer will see the documentation.
- JavaBeans are inherently mutable and so lack the advantages offered by immutable objects.
- Having to create getters for every property and setters for many, most, or all of them can lead to an immense quantity of boilerplate code.
6. Download the Source Code
You can download the full source code of this example here: Java Bean Example