Enumeration Java Example
In this tutorial we will discuss about Java Enumeration. The Java programming language contains the enum keyword, which represents a special data type that enables for a variable to belong to a set of predefined constants. The variable must be equal to one of the values that have been predefined for it.
The values defined inside this command are constants and shall be typed in uppercase letters. Also, these values are implicitly static and final and cannot be changed, after their declaration. If this command is a member of a class, then it is implicitly defined as static. Finally, you should use an enum, when you need to define and represent a fixed set of constants.
The most common example of a simple enum includes the days of the week, as shown below:
1 2 3 4 5 6 7 8 9 | public enum Day { SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY } |
Enums in Java are type-safe and thus, the value of an enum variable must be one of the predefined values of the enum itself. Also, enums have their own namespace and they can be used in a switch statement, in the same way as integers.
Furthermore, enums in Java are considered to be reference types, like class or Interface and thus, a programmer can define constructor, methods and variables, inside an enum.
In addition, a programmer can specify values of enum constants at their creation time, as shown in the example below:
1 2 3 4 5 6 7 8 9 | public enum Day { SUNDAY( 1 ), MONDAY( 2 ), TUESDAY( 3 ), WEDNESDAY( 4 ), THURSDAY( 5 ), FRIDAY( 6 ), SATURDAY( 7 ) } |
However, in order for this approach to function properly, a private member variable and a private constructor must be defined, as shown below:
1 2 3 4 5 | private int value; private Day( int value) { this .value = value; } |
In order to retrieve the value of each constant of the enum, you can define a public method inside the enum:
1 2 3 | public int getValue() { return this .value; } |
As we already mentioned, the values inside an enum are constants and thus, you can use them in comparisons using the equals
or compareTo
methods.
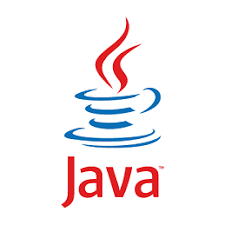
The Java Compiler automatically generates a static method for each enum, called values. This method returns an array of all constants defined inside the enum:
1 2 3 | //Printing all constants of an enum. for (Day day: Day.values()) System.out.println(day.name()); |
Notice that the values are returned in the same order as they were initially defined.
1. Methods: name, valueOf and toString
An enum contains the name and valueOf
methods. The valueOf
method can be used to map from a name to the corresponding enum constant, while the name method returns the name of an enum’s constant, exactly as declared in its declaration. Also, an enum can override the toString
method, just like any other Java class. A simple example is shown below:
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 | @Override public String toString() { switch ( this ) { case FRIDAY: return "Friday: " + value; case MONDAY: return "Monday: " + value; case SATURDAY: return "Saturday: " + value; case SUNDAY: return "Sunday: " + value; case THURSDAY: return "Thursday: " + value; case TUESDAY: return "Tuesday: " + value; case WEDNESDAY: return "Wednesday: " + value; default : return null ; } } |
An enum in Java can implement an Interface or extend a class, just like any other normal class. Also, an enum implicitly implements both the Serializable
and Comparable
interfaces.
Finally, you can define abstract methods inside this command. Each constant of the enum implements each abstract method independently. For example:
Car.java:
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 | public enum Car { AUDI { @Override public int getPrice() { return 25000 ; } }, MERCEDES { @Override public int getPrice() { return 30000 ; } }, BMW { @Override public int getPrice() { return 20000 ; } }; public abstract int getPrice(); } |
2. The EnumMap and EnumSet Collections
An EnumMap
is a specialized Map
implementation. All of the keys in an EnumMap
must come from a single enum type that is specified, explicitly or implicitly, when the map is created. EnumMaps
are represented internally as arrays. Also, EnumMaps
are maintained in the natural order of their keys.
A sample example that uses an EnumMap
is shown below:
EnumMapExample.java:
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 | import java.util.EnumMap; public class EnumMapExample { public static void main(String[] args) { // Create an EnumMap that contains all constants of the Car enum. EnumMap cars = new EnumMap(Car. class ); // Put some values in the EnumMap. cars.put(Car.BMW, Car.BMW.getPrice()); cars.put(Car.AUDI, Car.AUDI.getPrice()); cars.put(Car.MERCEDES, Car.MERCEDES.getPrice()); // Print the values of an EnumMap. for (Car c: cars.keySet()) System.out.println(c.name()); System.out.println(cars.size()); // Remove a Day object. cars.remove(Car.BMW); System.out.println( "After removing Car.BMW, size: " + cars.size()); // Insert a Day object. cars.put(Car.valueOf( "BMW" ), Car.BMW.getPrice()); System.out.println( "Size is now: " + cars.size()); } } |
A sample execution is shown below:
AUDI
MERCEDES
BMW
3
After removing Car.BMW, size: 2
Size is now: 3
An EnumSet
is a specialized Set
implementation. All of the elements in an EnumSet
must come from a single enum type that is specified, explicitly or implicitly, when the set is created. EnumSet
are represented internally as bit vectors. Also, an iterator traverses the elements in their natural order.
A sample example that uses an EnumSet
is shown below:
EnumSetExample.java:
01 02 03 04 05 06 07 08 09 10 11 12 13 14 15 16 17 18 19 20 21 22 | import java.util.EnumSet; public class EnumSetExample { public static void main(String[] args) { // Create an EnumSet that contains all days of the week. EnumSet week = EnumSet.allOf(Day. class ); // Print the values of an EnumSet. for (Day d: week) System.out.println(d.name()); System.out.println(week.size()); // Remove a Day object. week.remove(Day.FRIDAY); System.out.println( "After removing Day.FRIDAY, size: " + week.size()); // Insert a Day object. week.add(Day.valueOf( "FRIDAY" )); System.out.println( "Size is now: " + week.size()); } } |
A sample execution is shown below:
SUNDAY
MONDAY
TUESDAY
WEDNESDAY
THURSDAY
FRIDAY
SATURDAY
7
After removing Day.FRIDAY, size: 6
Size is now: 7
3. Download the Eclipse Project
This was a tutorial about Java Enumeration.
The Eclipse project of this Java Enumeration Example: Enumeration Java Example
Last updated on Dec. 24, 2019
Thanks. Very thorough.