Java Assert Keyword Example
In this article, we will explain what is the Assert Keyword in Java using examples. We will also create an example about assertion, that allows testing the correctness of any assumptions that have been made in a program.
1. Introduction
An assertion is achieved using the assert statement in Java. While executing an assertion, it is believed to be true. If it fails, JVM throws an error named AssertionError. It is mainly used for testing purposes during development.
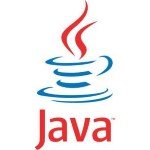
The assert statement is used with a Boolean expression and can be written in two different ways.
First way: assert expression;
Second way: assert expression1; expression2;
2. Java Assertion Example
Now that we have known what the Java Assert is, let us programmatically understand it with the following coding snippet.
AssertionInJava.java
// Java program to demonstrate syntax of assertion import java.util.Scanner; class Test { public static void main( String args[] ) { int a = 15; assert a >= 20 : " Underweight"; System.out.println("value of a is "+a); } }
Before Enabling Assertions:
By default assertions are disabled. When we compile and run the above coding snippet using the commands javac AssertionInJava.java and java Test
Output
value of a is 15
After Enabling Assertions:
When the Assertions are enabled and the output of the above coding snippet is as follows:
Exception in thread "main" java.lang.AssertionError: Underweight at Test.main(AssertionInJava.java:9)
The value of a is 15. The boolean expression along with the assert keyword evaluates to false so the compiler raises Assertion Error along with the message provided with the keyword.
3. Enabling and Disabling Assertions
By default, assertions are disabled. We need to run the code as given. The syntax for enabling the assertion statement in Java source code is:
java –ea Test OR
java –enableassertions Test
where Test is the class name.
The syntax for disabling assertions in java are:
java –da Test OR
java –disableassertions Test
where Test is the class name.
4. When and Where to Use the assert keyword in Java
Wherever a programmer wants to see if his/her assumptions are wrong or not.
- To make sure that an unreachable looking code is actually unreachable.
- To make sure that assumptions written in comments are right.
- To make sure the default switch case is not reached.
- To check the object’s state.
- At the beginning of the method
- After method invocation.
- Arguments to private methods. Private arguments are provided by the developer’s code only and the developer may want to check his/her assumptions about arguments.
- Conditional cases.
- Conditions at the beginning of any method.
5. Where not to Use
- Assertions should not be used to replace error messages
- Assertions should not be used to check arguments in the public methods as they may be provided by the user. Error handling should be used to handle errors provided by the user.
- Assertions should not be used on command line arguments.
6. Download the Source Code
This is an example of how to use assertion in Java.
You can download the full source code of this example here: Java Assert Keyword Example