Introduction to Groovy Language
1.Introduction
This is an in-depth article on Groovy language. The examples presented are developed using Groovy. Groovy is an opensource framework for developing and prototyping applications. It is based on java language.
2. Groovy Language Introduction
2.1 Prerequisites
Java 8 is required on the Linux, windows, or Mac operating systems. Groovy version 2.5.7 is used for this article.
2.2 Download
You can download Java 8 from the Oracle web site . Likewise, Groovy 2.5.7 can be downloaded from this website.
2.3 Setup
2.3.1 Java Setup
You can set the environment variables for JAVA_HOME and PATH. They can be set as shown below:
Setup
JAVA_HOME="/desktop/jdk1.8.0_73" export JAVA_HOME PATH=$JAVA_HOME/bin:$PATH export PATH
2.3.2 Groovy Setup
You can set the Groovy home in the PATH as shown below:
Setup
GROOVY_HOME="/usr/local/bin/groovy" export GROOVY_HOME PATH=$GROOVY_HOME/bin:$PATH export PATH
2.4 Running Groovy
You can check the version of the groovy by using the command groovy –version. The command for running Groovy is as below:
Groovy Version
groovy -version
The output of the executed Groovy command is shown below.
Groovy Version Output
apples-MacBook-Air:~ bhagvan.kommadi$ groovy -version Groovy Version: 2.5.7 JVM: 1.8.0_101 Vendor: Oracle Corporation OS: Mac OS X
2.5 Hello World in Groovy
You can build a helloworld class as shown below in the sample code.
Groovy HelloWorld
package org.javacodegeeks class HelloWorld { static void main( String [] args) { println("Greetings... ") } }
The code above can be executed by using the command below:
Groovy Compilation Command
groovy HelloWorld.groovy
The output of the executed Groovy command is shown below.
Groovy Execution output
apples-MacBook-Air:groovy bhagvan.kommadi$ groovy HelloWorld.groovy Greetings...
2.6 Groovy IDE Integration
You can configure the Groovy Eclipse plugin from the distribution site. The screen shot below shows the configuration of Groovy Eclipse plugin from Help-> Install-> New Software.
The groovy version is set from Eclipse’s Preferences -> Groovy ->Compiler. The setting of the groovy version 2.4.16 is shown below:
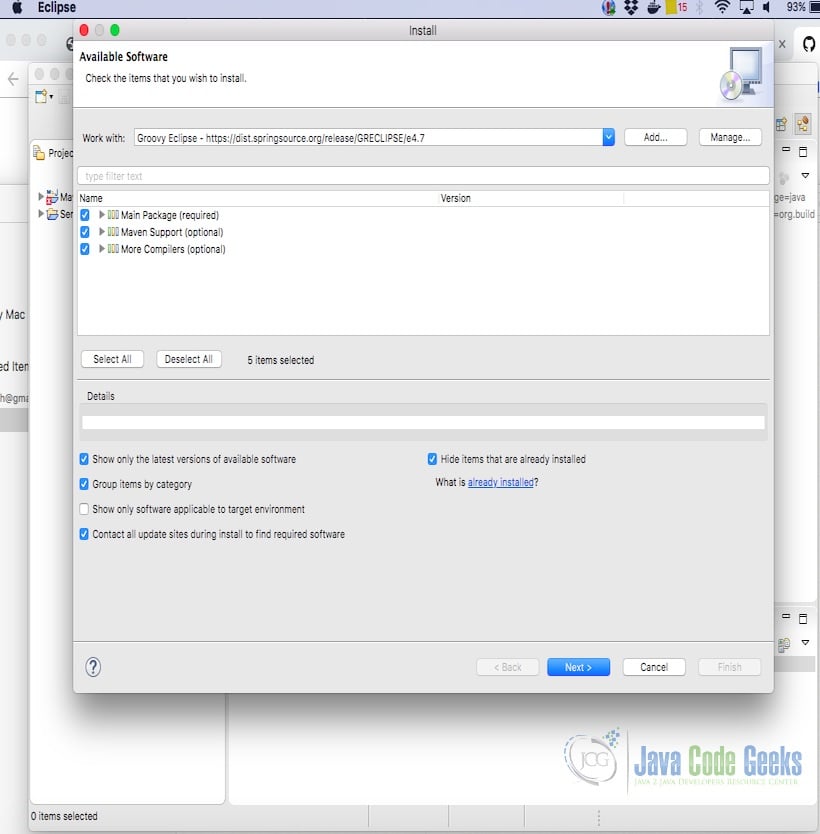
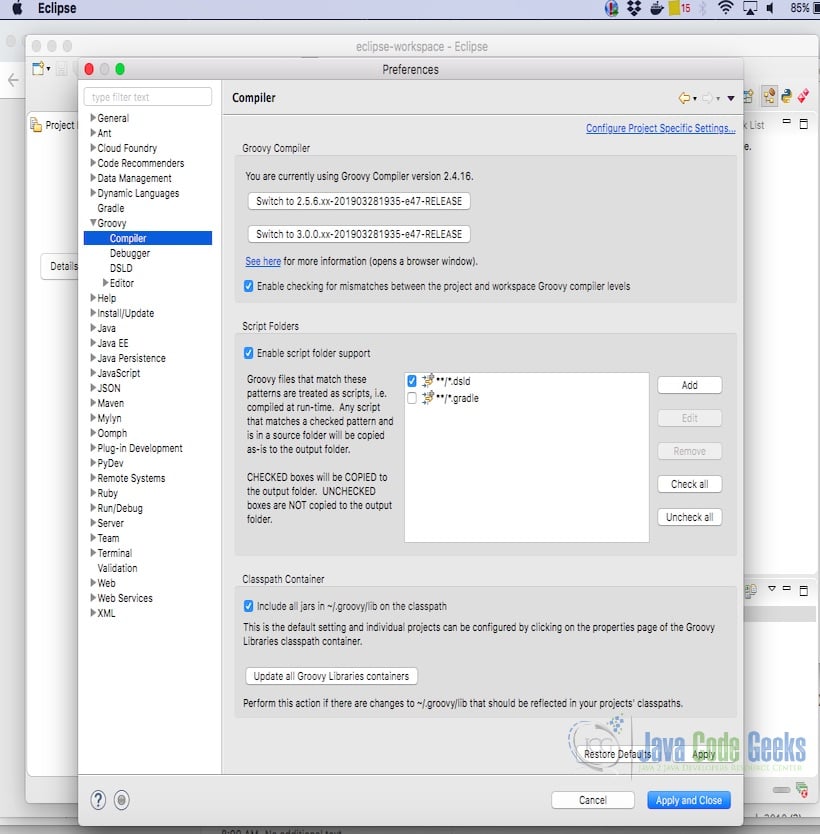
2.7 Groovy Data Types
Groovy has different data types such as :
- Byte
- Short
- int
- long
- float
- double
- char
- Boolean
- String
You can try out different data types as shown below in the sample code.
Groovy DataTypes
package org.javacodegeeks class DataTypes { static void main(String[] args) { int x = 6; long y = 200L; float a = 30.56f; double b = 20.5e40; BigInteger bi = 20g; BigDecimal bd = 4.5g; println(x); println(y); println(a); println(b); println(bi); println(bd); } }
The code above can be executed by using the command below:
Groovy Compilation Command
groovy DataTypes.groovy
The output of the executed Groovy command is shown below.
Groovy Execution output
apples-MacBook-Air:groovy bhagvan.kommadi$ groovy DataTypes.groovy 6 200 30.56 2.05E41 20 4.5
2.8 Groovy Variables
In groovy, variables are declared using def keyword. Sample code is attached below.
Groovy Variables
class VariableExample { static void main(String[] args) { int y = 7; int Y = 8; def _Person = "John"; println(y); println(Y); println(_Person); } }
The code above can be executed by using the command below:
Groovy Compilation Command
groovy VariableExample.groovy
The output of the executed Groovy command is shown below.
Groovy Execution output
apples-MacBook-Air:groovy bhagvan.kommadi$ groovy VariableExample.groovy 7 8 John
2.9 Groovy Methods
2.9.1 Groovy Static Methods
In groovy, static methods are defined using static and def keywords. The sample code is shown below.
Groovy Methods
class MethodsExample { static def ExecuteMethod() { println("Executing in groovy"); println(" a static method"); } static void main(String[] args) { ExecuteMethod(); } }
The code above can be executed by using the command below:
Groovy Compilation Command
groovy MethodsExample.groovy
The output of the executed Groovy command is shown below.
Groovy Execution output
apples-MacBook-Air:groovy bhagvan.kommadi$ groovy MethodsExample.groovy Executing in groovy a static method
2.9.2 Groovy Instance Methods
In groovy, instance methods are created like in java. The example below shows how to create the instance methods.
Groovy Methods
class InstanceMethods { int y; public int getY() { return y; } public void setY(int pY) { y = pY; } static void main(String[] args) { InstanceMethods instance = new InstanceMethods(); instance.setY(200); println(instance.getY()); } }
The code above can be executed by using the command below:
Groovy Compilation Command
groovy InstanceMethods.groovy
The output of the executed Groovy command is shown below.
Groovy Execution output
apples-MacBook-Air:groovy bhagvan.kommadi$ groovy InstanceMethods.groovy 200 apples-MacBook-Air:groovy bhagvan.kommadi$
2.9.3 Groovy Method Parameters
Method parameters in groovy are defined as presented in the code below.
Groovy Methods
class MethodParamExample { static void product(int a,int b) { int c = a*b; println(c); } static void main(String[] args) { product(20,6); } }
The code above can be executed by using the command below:
Groovy Compilation Command
groovy MethodParametersExample.groovy
The output of the executed Groovy command is shown below.
Groovy Execution output
apples-MacBook-Air:groovy bhagvan.kommadi$ groovy MethodParametersExample.groovy 120 apples-MacBook-Air:groovy bhagvan.kommadi$
2.10 Groovy File I/O
Groovy has features to read, write, and traverse files. The sample code shows how to read a file in groovy.
Groovy File I/O
import java.io.File class FileIO { static void main(String[] args) { new File("input.txt").eachLine { line -> println "line : $line"; } } }
The code above can be executed by using the command below:
Groovy Compilation Command
groovy FileIO.groovy
The input file (input.txt) contents are shown below.
input.txt
line1 line2 line3
The output of the executed Groovy command is shown below.
Groovy Execution output
apples-MacBook-Air:groovy bhagvan.kommadi$ groovy FileIO.groovy line : line1 line : line2 line : line3
2.11 Groovy Strings
In groovy, a String literal is defined as text in quotes. The example code is shown below.
Groovy File I/O
class StringOperations { static void main(String[] args) { String c = "Christmas"; String d = "Greetings"; println(d.length()); println(c + d); } }
The code above can be executed by using the command below:
Groovy Compilation Command
groovy StringOperations.groovy
The output of the executed Groovy command is shown below.
Groovy Execution output
apples-MacBook-Air:groovy bhagvan.kommadi$ groovy StringOperations.groovy 9 ChristmasGreetings
3. Download the Source Code
That was an introduction to Groovy Language.
You can download the full source code of this example here: Introduction to Groovy Language