Simple if else Java Example
In this post, we feature a simple if else Java Example. The Java if statement is used to test a boolean condition i.e. true or false.
1. Introduction
The logic inside the if condition executes when the condition is true otherwise the else block is executed. Java supports various types of if statements.
- if statement
- if else statement
- if else if statement
- nested if statement
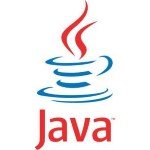
To start with this tutorial, we are hoping that users at present have their preferred IDE and JDK 1.8 installed on their machines. For easy usage, I am using Eclipse IDE.
2. Java If else Example
Now let us start with different code snippets to under the if else example in Java programming language.
2.1 Java if Statement
The if statement tests the condition and executes the conditional block only if the condition evaluates to true. Let us understand this with a simple code snippet.
Snippet
package com.jcg.ifelse; public class Example1 { public static void main(String[] args) { // Defining a salary variable for the employee. int salary= 2000; // Checking the employee salary. if(salary > 1000) { System.out.println("Salary is greater than 1000."); } } }
In this snippet, the output will only be printed if the employee salary is greater than 1000.
2.2 Java if else Statement
The if else statement tests the condition and executes the if block if the condition evaluates to true otherwise the else block, is executed. Let us understand this with a simple code snippet.
Snippet
package com.jcg.ifelse; public class Example2 { public static void main(String[] args) { boolean b = false; if (b) { System.out.println("Variable value is true."); } else { System.out.println("Variable value is NOT true."); } } }
In this snippet, the output will be printed based on the boolean value.
2.3 Java if else if Statement
The if else if
statement test one condition from multiple statements. Let us understand this with a simple code snippet.
Snippet
package com.jcg.ifelse; public class Example3 { public static void main(String[] args) { // Defining a salary variable for the employee. int salary= 1250; // Checking the employee salary. if(salary < 500) { System.out.println("Salary is less than 500."); } else if (salary >= 500 && salary < 1000) { System.out.println("Salary is greater than 500 but less than 1000."); } else if (salary >= 1000 && salary < 2000) { System.out.println("Salary is greater than 1000 but less than 2000."); } else if (salary > 2000) { System.out.println("Salary is greater than 2000."); } else { System.out.println("Invalid!"); } } }
In this snippet, the output will be printed based on the employee salary.
2.4 Java nested-if Statement
The nested-if statement consists of if block within another if block. Here the inner if condition executes only when the outer if the condition evaluates to true. Let us understand this with a simple code snippet.
Snippet
package com.jcg.ifelse; public class Example4 { public static void main(String[] args) { // Defining a salary and designation variable for the employee. int salary= 1250; String department= "Technology"; // Checking the employee salary. if(salary > 1000) { if(department.equalsIgnoreCase("technology")) { System.out.println("Employee is in technology department."); } else { System.out.println("Employee is not in technology department."); } } else { System.out.println("Invalid!"); } } }
In this snippet, the inner block will execute only when the outer if condition evaluates to true.
3. Switch Case
The switch case in Java language executes one statement from multiple conditions. It is like an if else if
statement. Make note,
- Duplicate case values are not allowed
- The
default
case is optional - The
break
keyword is used to end the case statement sequence. If omitted, the execution goes onto the next case
Let us understand this with a simple code snippet.
Snippet
package com.jcg.ifelse; public class Example5 { public static void main(String[] args) { int number= 5; switch(number) { case 2: System.out.println("2"); break; case 5: System.out.println("5"); break; case 8: System.out.println("8"); break; default: System.out.println("Invalid choice!"); break; } } }
In this snippet, the output will be printed based on the passed switch case condition. That is all for this tutorial and I hope the article served you whatever you were looking for. Happy Learning and do not forget to share!
4. Conclusion
In this tutorial, we had an in-depth look at the if else
statement. Developers can download the sample application as an Eclipse project in the Downloads section.
5. Download the Eclipse Project
This was an example of the if else statement.
You can download the full source code of this example here: Simple if-else Java Example
Last updated on Aug. 22, 2019