How to initialize an ArrayList in Java
In this article we will explain how to initialize an ArrayList in Java through example.
1. Introduction
ArrayList is a part of the Collection Framework and is present in java.util
package. It provides us dynamic arrays in Java. Though, it may be slower than standard arrays but can be helpful in programs where lots of manipulation in the array is needed.
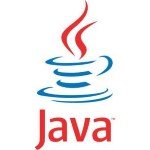
- ArrayList inherits the AbstractList class and implements the List interface.
- ArrayList is initialized by size, however, the size can increase if collection grows or shrunk if objects are removed from the collection.
- Java ArrayList allows us to randomly access the list.
- ArrayList can not be used for primitive types, like int, char, etc. We need a wrapper class for such cases (see this for details).
- ArrayList in Java can be seen as similar to a vector in C++.
You can also check our video on How to use ArrayList:
An ArrayList is a dynamically sized array, we do not have to specify the size while creating it. We can dynamically add and remove items. It automatically resizes itself.
2. Ways to Initialize an ArrayList in Java
In the above section, we have understood an ArrayList. Now let us see, how we can initialize an ArrayList. We can initialize an ArrayList using add()
method, asList()
method, List.of()
method, and using another collection. Let us get into each way programmatically and understand in detail.
2.1 Initialization with add()
In this way, we use add()
method to initialize an ArrayList. The following coding example illustrates the initialization of an ArrayList using add()
method.
// Java code to illustrate initialization // of ArrayList using add() method import java.util.*; public class Example1{ public static void main(String args[]) { // create a ArrayList String type ArrayList<String> al = new ArrayList<String>(); // Initialize an ArrayList with add() al.add("Java"); al.add("Code"); al.add("Geeks"); // print ArrayList System.out.println("ArrayList : " + al); } }
Output
ArrayList : [Java, Code, Geeks]
2.2 Initialization using asList()
In this way, we use asList()
method to initialize an ArrayList. The following coding example illustrates the initialization of an ArrayList using asList()
method.
// Java code to illustrate initialization // of ArrayList using asList method import java.util.*; public class Example2 { public static void main(String args[]) { // create a ArrayList String type // and Initialize an ArrayList with asList() ArrayList<String> al = new ArrayList<String>( Arrays.asList("Java", "Code", "Geeks")); // print ArrayList System.out.println("ArrayList : " + al); } }
Output
ArrayList : [Java, Code, Geeks]
2.3. Initialization using List.of() method
In this way, we use List.of()
method to initialize an ArrayList. The following coding example illustrates the initialization of an ArrayList using List.of()
method.
// Java code to illustrate initialization // of ArrayList using List.of() method import java.util.*; public class Example3 { public static void main(String args[]) { // create a ArrayList String type // and Initialize an ArrayList with List.of() List<String> al = new ArrayList<>( List.of("Java", "Code", "Geeks")); // print ArrayList System.out.println("ArrayList : " + al); } }
Output
ArrayList : [Java, Code, Geeks]
Note: To execute this program, use Java 9 or above versions.
2.4 Initialization using another Collection
In this way, we use some collection to initialize an ArrayList. The following coding example illustrates the initialization of an ArrayList using a collection.
import java.util.*; public class Example4 { public static void main(String args[]) { // create another collection List<Integer> arr = new ArrayList<>(); arr.add(1); arr.add(2); arr.add(3); arr.add(4); arr.add(5); // create a ArrayList Integer type // and Initialize an ArrayList with arr List<Integer> al = new ArrayList<Integer>(arr); // print ArrayList System.out.println("ArrayList : " + al); } }
Output
ArrayList : [1, 2, 3, 4, 5]
3. Download the Source Code
That was an example on how to initialize an ArrayList in Java.
You can download the full source code of this example here: How to initialize an ArrayList in Java
Good Article