How to Generate a Random Int in Java
This article shows how to generate a random Int or integer in Java using different approaches.
1. Introduction
Java has several ways to generate a random Integer, primitive or wrapped object, on its environment.
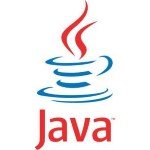
Here we’ll see some of these methods listed below:
- Random class
- Math class
- ThreadLocalRandom class
2. Pre-requisites
The minimum Java version for execute this article’s example is JDK 8 (find here), but we can use the most recent released Java version (JDK 15).
Also, I’m using IntelliJ 2020.2, but you can use any IDE with support for versions recommended above.
3. Random class
The Random class is part of java.util
package and is used to generate pseudo-random numbers in Java. Therefore, this is one simple approach to generates any type of number in Java as integer, double, float and so on.
Following we’ll see some methods that can be used to create a random integer:
Random.nextInt()
int firstCounter = 0; System.out.println("Generating without range"); while(firstCounter <3) { System.out.println(new Random().nextInt()); firstCounter++; }
Random.nextInt() result
Generating without range 1042399262 579286117 -1461817437
Literally, this is the most simple way to generate a random integer with Random class. So, the method nextInt()
will use inner Random class next()
method implementation to catch a integer for us.
A disadvantage (or advantage, depends on the use) of this method is that you can have any value for integer, varying from -2147483648 to 2147483647. However, if we want a more controlled range of integer, we can use the same method with a bound argument
Random.nextInt(int bound)
int secondCounter = 0; System.out.println("Generating with range"); while(secondCounter <3) { System.out.println(new Random().nextInt(10)); secondCounter++; }
Random.nextInt(int bound) result
Generating with range 3 6 4
As we can notice, the number does not vary above 10, how we determined in the method’s argument.
Note: The bound of nextInt() method must be positive (above zero)!
4. Math class
Here is what I think is the most famous way to generate a random number: the Math class.
Math class own a method random()
that generates a pseudo-random double
type number. Yes, I said double, that’s not the focus of this article.
But, We can write some lines of code as below to adapt our necessities:
Math.random() example
int max = 10; int min = -10; int range = (max - min) + 1; System.out.println("Generating integer numbers with Math.random():"); for (int i = 0; i < 5; i++) { int random = (int)(Math.random() * range) + min; System.out.println(random); }
As we notice, first is created a range of maximum and minimum numbers. Further, we establish a range to convert the random result of Math class that we can control our double result.
Finally, we put a cast to the integer primitive (could be the Integer wrapper class too) to make the result stay in integer. The output of this code will be 5 random numbers between -10 and +10 as following:
Math.random() output
Generating integer numbers with Math.random(): -1 6 4 -6 -2
5. ThreadLocalRandom class
ThreadLocalRandom class is a combination of the ThreadLocal and Random classes and is isolated to the current thread.
The difference between Random and ThreadLocalRandom is that Random class doesn’t perform well in a multi-thread environment. So, that’s why the ThreadLocalRandom was created.
ThreadLocalRandom simple example
System.out.println("Generating without range"); while(firstCounter <3) { System.out.println(ThreadLocalRandom.current().nextInt()); firstCounter++; }
We see above that we need to use ThreadLocalRandom.current()
method to call the current thread. In addition, we use the nextInt()
present in ThreadLocalRandom class and the result is some random numbers generated:
ThreadLocalRandom simple output
Generating without range 656287093 1502019411 850155572
Another advantage for the use of ThreadLocalRandom is that we can use a range with negative numbers now:
ThreadLocalRandom with range example
int secondCounter = 0; System.out.println("Generating with range"); while(secondCounter <3) { System.out.println(ThreadLocalRandom.current().nextInt(-10,10)); secondCounter++; }
And the result is as below:
ThreadLocalRandom with range output
Generating with range -5 2 8
6. Summary
In conclusion, we see some classes that we can use to generate random integer numbers in Java: Random, Math, and ThreadLocalRandom.
Moreover, we see what is needed to work with the generation properly and notice the different approaches and the kind of scenarios that we can use these generating methods.
7. Download the source code
You can download the full source code of this example here: How to Generate a Random Int in Java