How to deal with java.lang.outofmemoryerror: java heap space
In this article, we will show you how to deal with java.lang.outofmemoryerror: java heap space. Firstly, we will talk about what is java.lang.outofmemoryerror in general, and for what reason this might be caused. After that, we will show examples of how to solve this problem.
1. Introduction
In every Java application/program dynamic memory binding is required, in order to acquire more memory at execution or to free up space when it’s needed. We should know that java does not release immediately the dynamic memory, in contrast, runs the garbage collector to remove/delete the memory of objects that are no longer in use. In particular, the memory limit used by each program can be as large as the physical memory of a computer, but usually, the limits are much smaller due to other applications running at the same time. So when we create an object in a java class we bind memory and store it into the heap. If these bits of memory are not available at the heap the console will throw an exception which called "java.lang.outofmemoryerror"
. This error is a subclass of java.lang.VirtualMachineError and it has mainly two types of errors :
- The java.lang.OutOfMemoryError: Java heap space
- The java.lang.OutOfMemoryError: PermGen space
2. Technologies used
The example code in this article was built and run using:
- Java 1.8.231(1.8.x will do fine)
- Eclipse IDE for Enterprise Java Developers – Photon
3. What is java.lang.outofmemoryerror: java heap space
To understand more completely what is "java.lang.outofmemoryerror: java heap space"
we must compare the two memory types (java heap space and PermGen). When you write a java program, the objects that you create, are being stored in the heap instead of PremGem space which is used to holds meta-data describing user classes. So if the heap is full, the objects don’t find memory to store, as a result, the console output is: "java.lang.OutOfMemoryError: java heap space"
.
4. What cause java.lang.outofmemoryerror: java heap space
There are several reasons that can lead to this error :
4.1. The size of the heap space is not enough
The first and foremost reason is just like we said, we are trying to store objects whose size in bits is larger than the available heap memory.
ExampleWithError.java
public class ExampleWithError { public static void main(String[] args) { int ArraySize=2*256000*1024; int[] i = new int[ArraySize]; } }
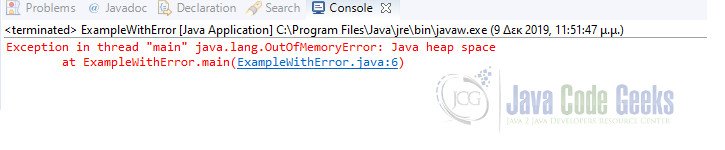
As we have already mentioned this is an example and the output of code with our familiar error.
- Line 2: We create an integer which we want to be bigger than our memory limit (In this example our memory limit is 1Gb).
- Line 5: We add this integer to an array
As a result, we can see at the output that we have exceeded the limits and the console gives us an error.
4.2. Long-standing programs
Another reason is that if the program is long-standing then the garbage collector cannot delete any objects and release some memory chunks because the application unintentionally uses them.
4.3. Too many finalizers
Furthermore, we can find this error at programs that use “finalizers” enormously. This happens because this type of object cannot reclaim their space when the garbage collector is working.
4.4. Memory leaks
We must know that when we see this error, it does not mean that every time we have memory leaks. Sometimes mistakes on objects such as objects which are no longer used by the program and the garbage collector don’t recognize them, can cause memory leaks and then when we use it again it can lead the program to consume constantly more and more memory until the heap space is full.
Here is an example :
MemoryLeak.java
import java.util.HashMap; import java.util.Map; public class MemoryLeak { static class Country { Integer Ccity; Country(Integer Ccity) { this.Ccity = Ccity; } public int hashCode() { return Ccity.hashCode(); } } public static void main(String[] args) { Map map = new HashMap(); while (1==1) { for (int j = 0; j <=500000; j++) { if (!map.containsKey(new Country(j))) { map.put(new Country(j), "capital city:" + j); } } } } }
In this example, we put some objects (data) in a Hashmap with a loop but this loop unexpectedly stops. After the execution (it will take some time), we will see that the console output is :"outofmemoryerror:java heap space"
. Firstly, we expect from the program to run forever assuming that the caching solution store only the 500000 elements but all keys will be stored in "map"
because the Country class which does not have an equality method to control the values.
5. How to deal with java.lang.outofmemoryerror: java heap space
In this section, we will analyze the most interesting part of the article which is how we can deal with the "outofmemoryerror"
. There are two ways to solve this problem.
5.1. Increase the maximum heap size
It is the most simple and fast way to solve this error. The only thing you must do is to open java configuration settings and write two commands.
- Firstly, open the Control Panel and select Programs.
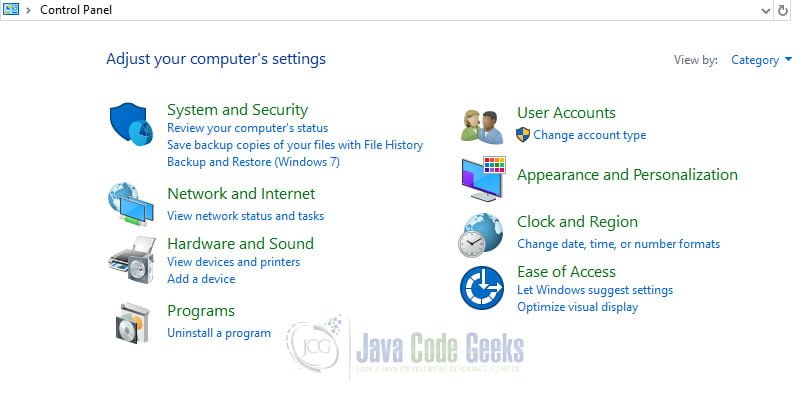
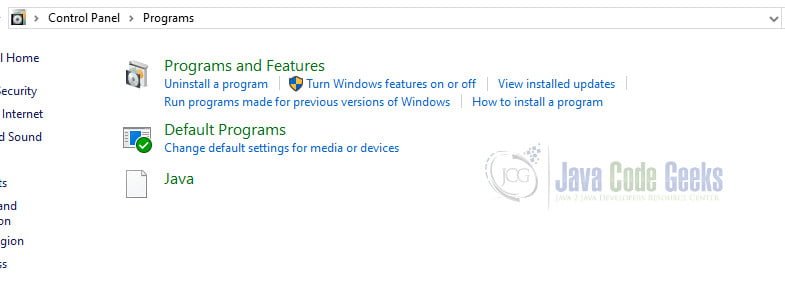
Then click on Java and a window will pop up. On this window click on java and then click on view.
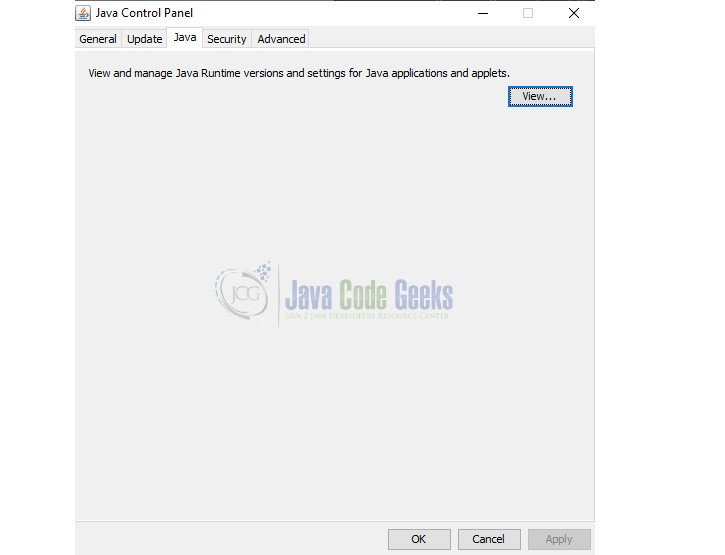

Now you must write on the runtime parameters this: -xms512m. The number of this command declares the limit of the dynamic memory which can be used by the java programs and the last “m” of this command can change to “g” from gigabytes and “k” from kilobytes. Then click OK and OK again.
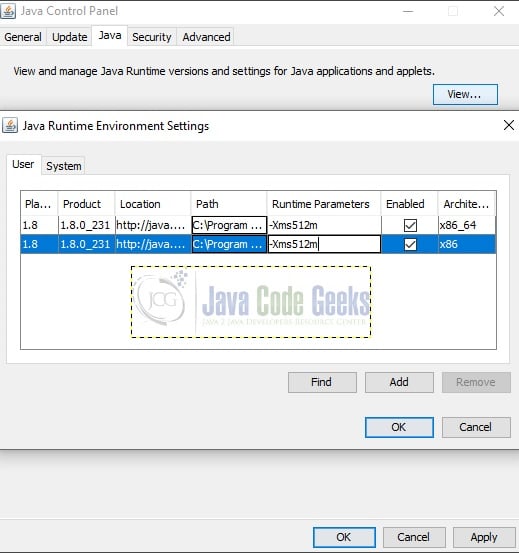
5.2. Use Profilers
if it still appears on your console this error : "java.lang.outofmemoryerror"
then maybe there is a memory leak that causes this problem. The best you can do in this situation is to profile your application. You can use Eclipse Memory Analyzer or any other memory analyzer /profilers such us Java VisualVM, and the Netbeans Profiler.
6. Download the Complete Source Code
Here is the code from the examples we used!
You can download the full source code of this example here: How to deal with java.lang.outofmemoryerror: java heap space