How to create an Array in Java
In this article, we will see How to create an Array in Java.
You can check how to use arrays in Java in the following video:
1. What is an array?
An array is a data structure used as a container to store a collection of elements with the same type. The size of an array is fixed once created. Elements stored in an array can be primitive values or variables but they are all of a single type.
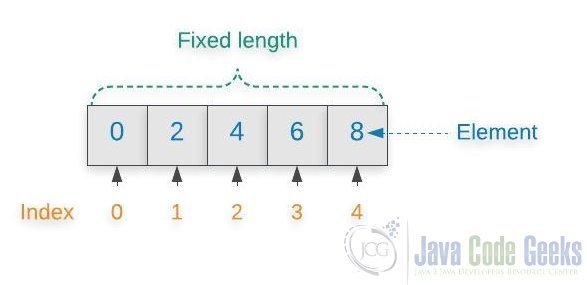
In this example, we are going to show you how to create an array in Java. The JDK version we use to compile the source code in this example is OpenJDK 13 and the IDE we use is Eclipse IDE 2020-03.
2. How to create an array in Java
To create an array in Java, there are a few steps we need to follow.
2.1 Declaring an array
Actually we don’t declare an array. We declare a variable to refer to
an array. The following line declares a variable referring to an array of integers:
// declaring an array of integers int[] arrayOfIntegers;
There are two parts in the declaration:
- type[]: type is the data type of elements stored in an array
- array name: it can be any valid variable name
We can declare arrays of other types in the same way:
// declaring an array of longs long[] arrayOfLongs; // declaring an array of Strings String[] arrayOfStrings;
It is valid to declare an array as below but it is not recommended:
// declaring an array of integers in another way int arrayOfIntegers[];
You might be surprised that we cannot use the array variable we have just declared above directly. For example, if we want to print the size of the array to standard output,
public class ArrayExample { /** * @param args */ public static void main(String[] args) { // declaring an array of integers int[] arrayOfIntegers; // print the size of the array System.out.println(arrayOfIntegers.length); } }
the following error will occur when compiling:
Exception in thread "main" java.lang.Error: Unresolved compilation problem: The local variable arrayOfIntegers may not have been initialized
So before we can use the array, we need to initialize it.
2.2 Initializing an array
Firstly we create an array of integers:
// create an array of integers containing 5 elements arrayOfIntegers = new int[5];
then we assign values to the elements of the array:
arrayOfIntegers[0] = 0; arrayOfIntegers[1] = 2; arrayOfIntegers[2] = 4; arrayOfIntegers[3] = 6; arrayOfIntegers[4] = 8;
You may have already noticed that we access an element of an array by its index number. Note that the index begins with 0. So, for example, the index number of the third element is 2.
Of course, if you are familiar with the loop syntax in Java, in some cases you can initialize the array by using a for
loop:
// initialize the array with a for loop for (int i = 0; i < arrayOfIntegers.length; i++) { // the value is the index number multiples 2 arrayOfIntegers[i] = i * 2; }
There is another way to initialize an array by providing the elements between a pair of curly brackets:
// another way to create and initialize the array arrayOfIntegers = new int[] {0, 2, 4, 6, 8};
2.3 A shortcut
There is a shortcut to declare and initialize an array in one line as below:
// a shortcut to declare and initialize an array int[] arrayOfIntegers = {0, 1, 2, 3, 4, 5, 6, 7, 8, 9};
Comparing the ways of creating an array, we need to note that the new
keyword is required when creating and initializing an array for a previously declared variable. The new
keyword creates a new array at first, then the reference of the new array is assigned back to the variable declared previously.
// declaring an array of integers int[] arrayOfIntegers; // create an array of integers containing 5 elements arrayOfIntegers = new int[5]; // another way to initialize the array arrayOfIntegers = new int[] {0, 2, 4, 6, 8};
2.4 Multi-dimensional array
In real-world coding scenarios, we may need to describe something more complex than a collection of elements such as a matrix. Is it possible to describe a matrix with an array? The answer is yes. A multi-dimensional array can serve this purpose very well. For instance, we would like to print the matrix below to standard out:
0 1 2 3 4 5 6 7 8 9
We can use a multi-dimensional array to implement it:
public class MultiDimensionArrayExample { /** * @param args */ public static void main(String[] args) { // declaring and initializing an multi-dimensional array int[][] matrix = { { 0, 1, 2 }, { 3, 4, 5, 6 }, { 7, 8, 9 } }; // using two loops to print all elements to standard output // the row loop for (int i = 0; i < matrix.length; i++) { // the column loop for (int j = 0; j < matrix[i].length; j++) { // print the value followed by a tab character System.out.printf("%d\t", matrix[i][j]); } // print a new line after each row System.out.println(); } } }
Basically, a multi-dimensional array is an array of arrays. As we can see from the example above, each element of the first dimension of the matrix is an array.
3. Download the Source Code
You can download the full source code of this example here: How to create an Array in Java