How to call a method in Java
In this article, we will show you how to call a method in Java. A method in java is a collection of statements that are grouped together to perform an operation. You can pass data, known as parameters, into a method. Methods are also known as functions.
You can also check this tutorial in the following video:
Each method has its own name. When that name is encountered in a program, the execution of the program branches to the body of that method. When the method is finished, execution returns to the area of the program code from which it was called, and the program continues on to the next line of code.
When you call the System.out.println() method, for example, the system actually executes several statements in the background which is already stored in the library, in order to display a message on the console.
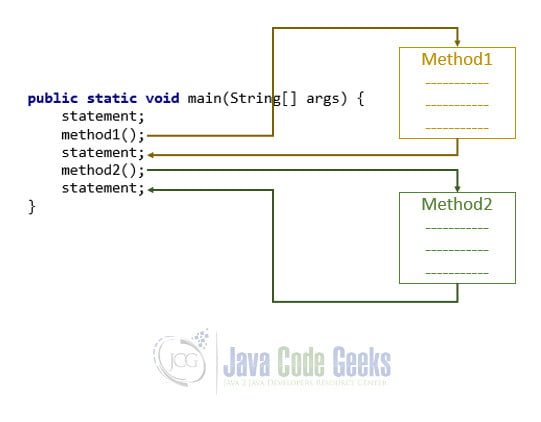
Why use methods? To reuse code: define the code once, and use it many times. Actually modular fashion allows for several programmers to work independently on separate concepts which can be assembled later to create the entire project. The use of methods will be our first step in the direction of modular programming.
Now you will learn how to create your own methods with or without return
values, invoke a method with or without parameters and apply method abstraction in the program design.
1. Create a Method
A method must be declared within a class. It is defined with the name of the method, followed by parentheses ().
Method definition consists of a method header and a method body.
Example:
public class MyClass { public static void myMethod() { // code to be executed } }
Code explanation:
public static
: modifier, it defines the access type of the methodmyMethod()
: name of the methodstatic
: means that the method belongs to the MyClass class and not an object of the MyClass class.void
: means that this method does not have a return value.
Note: The word public
before the method name means that the method itself can be called from anywhere which includes other classes, even from different packages (files) as long as you import the class. There are three other words that can replace public
. They are protected
and private
. If a method is protected
, then only this class and subclasses (classes that use this as a basis to build off of) can call the method. If a method is private
, then the method can only be called inside the class. The last keyword is really not even a word. This is if you had nothing in the place of public
, protected
, or private
. This is called the default, or package-private. This means that only the classes in the same package can call the method. If you are interested to see more examples you can read this article.
2. How to call a method in Java
To call a method in Java, write the method’s name followed by two parentheses () and a semicolon;
The process of method calling is simple. When a program invokes a method, the program control gets transferred to the called method.
In the following example, myMethod()
is used to print a text (the action), when it is called:
MyClass.java
public class MyClass { static void myMethod() { System.out.println("You have called me! My name is: myMethod!"); } public static void main(String[] args) { myMethod(); } }
Output:
You have called me! My name is: myMethod!
In the above example if you remove the static
keyword, java complains that you can’t call a method from a static function:
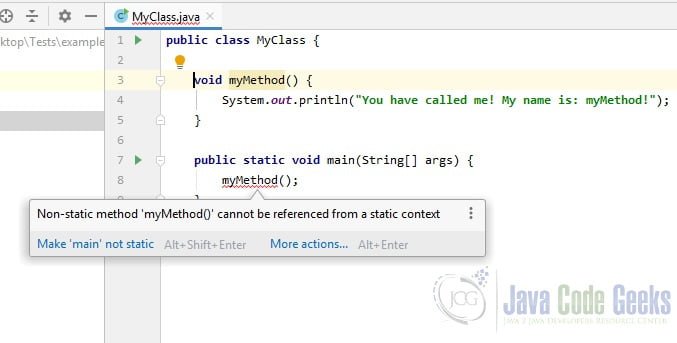
If you don’t want to use static
, you can only call instance method:
public class MyClass { void myMethod() { System.out.println("You have called me! My name is: myMethod!"); } public static void main(String[] args) { new MyClass().myMethod(); } }
A method can also be called multiple times:
MyClass.java
public class MyClass { static void myMethod() { System.out.println("You have called me! My name is: myMethod!"); } public static void main(String[] args) { myMethod(); myMethod(); myMethod(); } }
Output:
You have called me! My name is: myMethod! You have called me! My name is: myMethod! You have called me! My name is: myMethod!
3. Java Method Parameters
3.1 Parameters and Arguments
Information can be passed to methods as parameter. Parameters act as variables inside the method.
Parameters are specified after the method name, inside the parentheses. You can add as many parameters as you want, just separate them with a comma.
The following example has a method that takes an int
called num as parameter. When the method is called, we pass along a number, which is used inside the method to be multiplied by 2:
Example03
public class Math { static int multiplyBytwo(int number) { return number * 2; } public static void main(String[] args) { int result = multiplyBytwo(2); System.out.println("The output is: " + result); } }
Output:
The result of multiplication is: 4
3.2 Multiple Parameters
You can have as many parameters as you like. See the following example:
Example04
public class Math { static int sum(int num1, int num2) { return num1 + num2; } public static void main(String[] args) { int result = sum(2,3); System.out.println("Sum of numbers is: " + result); } }
Output:
Sum of numbers is: 5
Note: when you are working with multiple parameters, the method call must have the same number of arguments as there are parameters, and the arguments must be passed in the same order.
In examples 03 and 04 we have passed parameters by value. In fact, the values of the arguments remain the same even after the method invocation. Let’s see another example, example05:
Swapp.java
public class Swapp { public static void swapFunction(int a, int b) { System.out.println("In swapFunction at the begining: a = " + a + " , b = " + b); // Swap n1 with n2 int c = a; a = b; b = c; System.out.println("In swapFunction at the end: a = " + a + " , b = " + b); } public static void main(String[] args) { int a = 10; int b = 20; System.out.println("Before swapping: a = " + a + " , b = " + b); // Invoke the swap method swapFunction(a, b); System.out.println("\n**Now, Before and After swapping values will be same here**:"); System.out.println("After swapping: a = " + a + " , b = " + b); } }
Output:
Before swapping: a = 10 , b = 20 In swapFunction at the begining: a = 10 , b = 20 In swapFunction at the end: a = 20 , b = 10 **Now, Before and After swapping values will be same here**: After swapping: a = 10 , b = 20
Now if we pass an object and change any of its fields, the values will also be changed, example06:
Student.java
public class Student { String firstName; String familyName; int age; public Student(){ } public Student(String firstName, String familyName){ this.firstName = firstName; this.familyName = familyName; } public String getFirstName() { return firstName; } public void setFirstName(String firstName) { this.firstName = firstName; } public String getFamilyName() { return familyName; } public void setFamilyName(String familyName) { this.familyName = familyName; } public int getAge() { return age; } public void setAge(int age) { this.age = age; } }
Now we create an instance of the Student
class and initialize its fields by its constructor, after that we will rechange the field value by its method:
public class Main { public static void main(String[] args) { Student student = new Student("Jack", "Smith"); System.out.println("Student's name is set (Constructor): " + student.getFirstName()); student.setFirstName("John"); System.out.println("Student's name is set (Main): " + student.getFirstName()); } }
Output:
Student's name is set (Constructor): Jack Student's name is set (Main): John
4. Method Overloading
When a class has two or more methods by the same name but different parameters, it is known as method overloading. It is different from overriding. In overriding, a method has the same method name, type, number of parameters, etc. Consider the following example, which has one method that adds numbers of different type, example07:
Sum.java
public class Sum { static int sum(int x, int y) { return x + y; } static double sum(double x, double y) { return x + y; } public static void main(String[] args) { int int_sum = sum(10, 20); double double_sum = sum(10.5, 23.67); System.out.println("int: " + int_sum); System.out.println("double: " + double_sum); } }
Output:
int: 30 double: 34.17
Note: Multiple methods can have the same name as long as the number and/or type of parameters are different.
5. Method Overriding
Overriding is a feature that allows a subclass or child class to provide a specific implementation of a method that is already provided by one of its super-classes or parent classes. When a method in a subclass has the same name, same parameters or signature and same return type(or sub-type) as a method in its super-class, then the method in the subclass is said to override the method in the super-class.
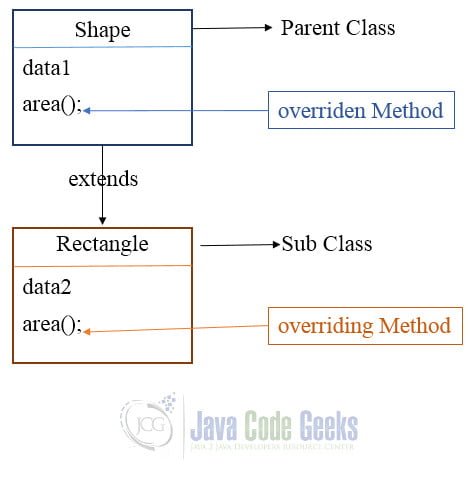
If an object of a parent class is used to invoke the method, then the version in the parent class will be executed, but if an object of the subclass is used to invoke the method, then the version in the child class will be executed. In other words, it is the type of the object being referred to (not the type of the reference variable) that determines which version of an overridden method will be executed.
5.1 Rules for Java Method Overriding
- The method must have the same name as in the parent class
- The method must have the same parameter as in the parent class.
- There must be an IS-A relationship (inheritance).
Let us see an example, example08:
Human.java
public class Human { //Overridden method public void walk() { System.out.println("Human is walking"); } }
Now we extend the Human
class and create a subclass from it:
HumanChild.java
public class HumanChild extends Human{ //Overriding method public void walk(){ System.out.println("Child is walking"); } public static void main( String args[]) { HumanChild obj = new HumanChild(); obj.walk(); } }
As you see, obj.walk();
will call the child class version of walk()
:
Output:
Child is walking
6. Calling an abstract method
Calling an abstract method in java is a way of providing implementation to the abstract superclass method in the child class. The subclass must override the inherited method to provide its implementation. To declare a method abstract we simply specify the abstract
keyword in the method signature.
package com.practice; abstract class Vehicle { abstract void shape(); void engine() { System.out.println("whatever"); } } class Audi extends Vehicle { void shape() { System.out.println("Audi is the shape of vehicle"); } } public class CallingAbstractMethodInJava { public static void main(String[] args) { Audi audi = new Audi(); audi.shape(); audi.engine(); } }
Output:
Audi is the shape of vehicle Whatever
7. this keyword in java
this
keyword in java is a reference variable that refers to the current object
package com.practice; class Student { int id; String name; Student() { } Student(int id, String name) { this.id = id; this.name = name; } public void display() { System.out.println("Id= " + this.id + ", Name= " + this.name); } } public class ThisKeyWordInJava { public static void main(String[] args) { Student s1 = new Student(101, "Rakesh"); s1.display(); Student s2 = new Student(102, "Suresh"); s2.display(); } }
Output:
Id= 101, Name= Rakesh Id= 102, Name= Suresh
8. More articles
- Best Way to Learn Java Programming Online
- Java Tutorial for Beginners (with video)
- Java Constructor Example (with video)
- Printf Java Example (with video)
9. Download the Source Code
You can download the full source code of this example here: How to call a method in Java